Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / xsp / System / Web / Configuration / HttpRuntimeSection.cs / 1477467 / HttpRuntimeSection.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Configuration { using System; using System.Xml; using System.Configuration; using System.Collections.Specialized; using System.Collections; using System.Globalization; using System.IO; using System.Text; using System.Web.Util; using System.ComponentModel; using System.Security.Permissions; public sealed class HttpRuntimeSection : ConfigurationSection { #if !FEATURE_PAL // FEATURE_PAL-specific timeout values internal const int DefaultExecutionTimeout = 110; #else // !FEATURE_PAL // The timeout needs to be extended, since Coriolis/Rotor is much slower // especially platforms like Solaris wan't be able to process complicated // requests if in debug mode. // Remove or change this once the real timeout is known #if DEBUG internal const int DefaultExecutionTimeout = 110 * 10; #else // DEBUG internal const int DefaultExecutionTimeout = 110 * 5; #endif // DEBUG #endif // !FEATURE_PAL internal const int DefaultMaxRequestLength = 4096 * 1024; // 4MB internal const int DefaultRequestLengthDiskThreshold = 80 * 1024; // 80KB internal const int DefaultMinFreeThreads = 8; internal const int DefaultMinLocalRequestFreeThreads = 4; internal const int DefaultAppRequestQueueLimit = 100; internal const int DefaultShutdownTimeout = 90; internal const int DefaultDelayNotificationTimeout = 0; internal const int DefaultWaitChangeNotification = 0; internal const int DefaultMaxWaitChangeNotification = 0; internal const bool DefaultEnableKernelOutputCache = true; internal const bool DefaultRequireRootedSaveAsPath = true; internal const bool DefaultSendCacheControlHeader = true; internal const string DefaultEncoderType = "System.Web.Util.HttpEncoder"; internal static readonly Version DefaultRequestValidationMode = VersionUtil.Framework40; internal const string DefaultRequestValidationModeString = "4.0"; internal const string DefaultRequestValidationType = "System.Web.Util.RequestValidator"; internal const string DefaultRequestPathInvalidCharacters = "<,>,*,%,&,:,\\,?"; internal const int DefaultMaxUrlLength = 260; internal const int DefaultMaxQueryStringLength = 2048; internal const bool DefaultRelaxedUrlToFileSystemMapping = false; private bool enableVersionHeaderCache = true; private bool enableVersionHeaderCached = false; private TimeSpan executionTimeoutCache; private bool executionTimeoutCached = false; private bool sendCacheControlHeaderCached = false; private bool sendCacheControlHeaderCache; private static ConfigurationPropertyCollection _properties; private static readonly ConfigurationProperty _propExecutionTimeout = new ConfigurationProperty("executionTimeout", typeof(TimeSpan), TimeSpan.FromSeconds((double)DefaultExecutionTimeout), StdValidatorsAndConverters.TimeSpanSecondsConverter, StdValidatorsAndConverters.PositiveTimeSpanValidator, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propMaxRequestLength = new ConfigurationProperty("maxRequestLength", typeof(int), 4096, null, StdValidatorsAndConverters.NonZeroPositiveIntegerValidator, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propRequestLengthDiskThreshold = new ConfigurationProperty("requestLengthDiskThreshold", typeof(int), 80, null, StdValidatorsAndConverters.NonZeroPositiveIntegerValidator, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propUseFullyQualifiedRedirectUrl = new ConfigurationProperty("useFullyQualifiedRedirectUrl", typeof(bool), false, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propMinFreeThreads = new ConfigurationProperty("minFreeThreads", typeof(int), 8, null, StdValidatorsAndConverters.PositiveIntegerValidator, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propMinLocalRequestFreeThreads = new ConfigurationProperty("minLocalRequestFreeThreads", typeof(int), 4, null, StdValidatorsAndConverters.PositiveIntegerValidator, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propAppRequestQueueLimit = new ConfigurationProperty("appRequestQueueLimit", typeof(int), 5000, null, StdValidatorsAndConverters.NonZeroPositiveIntegerValidator, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propEnableKernelOutputCache = new ConfigurationProperty("enableKernelOutputCache", typeof(bool), true, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propEnableVersionHeader = new ConfigurationProperty("enableVersionHeader", typeof(bool), true, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propRequireRootedSaveAsPath = new ConfigurationProperty("requireRootedSaveAsPath", typeof(bool), true, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propEnable = new ConfigurationProperty("enable", typeof(bool), true, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propShutdownTimeout = new ConfigurationProperty("shutdownTimeout", typeof(TimeSpan), TimeSpan.FromSeconds((double)DefaultShutdownTimeout), StdValidatorsAndConverters.TimeSpanSecondsConverter, null, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propDelayNotificationTimeout = new ConfigurationProperty("delayNotificationTimeout", typeof(TimeSpan), TimeSpan.FromSeconds((double)DefaultDelayNotificationTimeout), StdValidatorsAndConverters.TimeSpanSecondsConverter, null, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propWaitChangeNotification = new ConfigurationProperty("waitChangeNotification", typeof(int), 0, null, StdValidatorsAndConverters.PositiveIntegerValidator, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propMaxWaitChangeNotification = new ConfigurationProperty("maxWaitChangeNotification", typeof(int), 0, null, StdValidatorsAndConverters.PositiveIntegerValidator, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propEnableHeaderChecking = new ConfigurationProperty("enableHeaderChecking", typeof(bool), true, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propSendCacheControlHeader = new ConfigurationProperty("sendCacheControlHeader", typeof(bool), DefaultSendCacheControlHeader, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propApartmentThreading = new ConfigurationProperty("apartmentThreading", typeof(bool), false, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propEncoderType = new ConfigurationProperty("encoderType", typeof(string), DefaultEncoderType, null, StdValidatorsAndConverters.NonEmptyStringValidator, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propRequestValidationMode = new ConfigurationProperty("requestValidationMode", typeof(Version), DefaultRequestValidationMode, StdValidatorsAndConverters.VersionConverter, null, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propRequestValidationType = new ConfigurationProperty("requestValidationType", typeof(string), DefaultRequestValidationType, null, StdValidatorsAndConverters.NonEmptyStringValidator, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propRequestPathInvalidCharacters = new ConfigurationProperty("requestPathInvalidCharacters", typeof(string), DefaultRequestPathInvalidCharacters, StdValidatorsAndConverters.WhiteSpaceTrimStringConverter, null, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propMaxUrlLength = new ConfigurationProperty("maxUrlLength", typeof(int), DefaultMaxUrlLength, null, new IntegerValidator(0, 2097151), // Max from VS 330766 ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propMaxQueryStringLength = new ConfigurationProperty("maxQueryStringLength", typeof(int), DefaultMaxQueryStringLength, null, new IntegerValidator(0, 2097151), // Max from VS 330766 ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propRelaxedUrlToFileSystemMapping = new ConfigurationProperty("relaxedUrlToFileSystemMapping", typeof(bool), DefaultRelaxedUrlToFileSystemMapping, ConfigurationPropertyOptions.None); private int _MaxRequestLengthBytes; private int _RequestLengthDiskThresholdBytes; private static String s_versionHeader = null; private Version _requestValidationMode; /**/ static HttpRuntimeSection() { // Property initialization _properties = new ConfigurationPropertyCollection(); _properties.Add(_propExecutionTimeout); _properties.Add(_propMaxRequestLength); _properties.Add(_propRequestLengthDiskThreshold); _properties.Add(_propUseFullyQualifiedRedirectUrl); _properties.Add(_propMinFreeThreads); _properties.Add(_propMinLocalRequestFreeThreads); _properties.Add(_propAppRequestQueueLimit); _properties.Add(_propEnableKernelOutputCache); _properties.Add(_propEnableVersionHeader); _properties.Add(_propRequireRootedSaveAsPath); _properties.Add(_propEnable); _properties.Add(_propShutdownTimeout); _properties.Add(_propDelayNotificationTimeout); _properties.Add(_propWaitChangeNotification); _properties.Add(_propMaxWaitChangeNotification); _properties.Add(_propEnableHeaderChecking); _properties.Add(_propSendCacheControlHeader); _properties.Add(_propApartmentThreading); _properties.Add(_propEncoderType); _properties.Add(_propRequestValidationMode); _properties.Add(_propRequestValidationType); _properties.Add(_propRequestPathInvalidCharacters); _properties.Add(_propMaxUrlLength); _properties.Add(_propMaxQueryStringLength); _properties.Add(_propRelaxedUrlToFileSystemMapping); } public HttpRuntimeSection() { _MaxRequestLengthBytes = -1; _RequestLengthDiskThresholdBytes = -1; } protected override ConfigurationPropertyCollection Properties { get { return _properties; } } [ConfigurationProperty("executionTimeout", DefaultValue = "00:01:50")] [TypeConverter(typeof(TimeSpanSecondsConverter))] [TimeSpanValidator(MinValueString="00:00:00", MaxValueString=TimeSpanValidatorAttribute.TimeSpanMaxValue)] public TimeSpan ExecutionTimeout { get { if (executionTimeoutCached == false) { executionTimeoutCache = (TimeSpan)base[_propExecutionTimeout]; executionTimeoutCached = true; } return executionTimeoutCache; } set { base[_propExecutionTimeout] = value; executionTimeoutCache = value; } } [ConfigurationProperty("maxRequestLength", DefaultValue = 4096)] [IntegerValidator(MinValue = 0)] public int MaxRequestLength { get { return (int)base[_propMaxRequestLength]; } set { if (value < RequestLengthDiskThreshold) { throw new ConfigurationErrorsException(SR.GetString( SR.Config_max_request_length_smaller_than_max_request_length_disk_threshold), ElementInformation.Properties[_propMaxRequestLength.Name].Source, ElementInformation.Properties[_propMaxRequestLength.Name].LineNumber); } base[_propMaxRequestLength] = value; } // } [ConfigurationProperty("requestLengthDiskThreshold", DefaultValue = 80)] [IntegerValidator(MinValue = 1)] public int RequestLengthDiskThreshold { get { return (int)base[_propRequestLengthDiskThreshold]; } set { if (value > MaxRequestLength) { throw new ConfigurationErrorsException(SR.GetString( SR.Config_max_request_length_disk_threshold_exceeds_max_request_length), ElementInformation.Properties[_propRequestLengthDiskThreshold.Name].Source, ElementInformation.Properties[_propRequestLengthDiskThreshold.Name].LineNumber); } base[_propRequestLengthDiskThreshold] = value; } } [ConfigurationProperty("useFullyQualifiedRedirectUrl", DefaultValue = false)] public bool UseFullyQualifiedRedirectUrl { get { return (bool)base[_propUseFullyQualifiedRedirectUrl]; } set { base[_propUseFullyQualifiedRedirectUrl] = value; } } [ConfigurationProperty("minFreeThreads", DefaultValue = 8)] [IntegerValidator(MinValue = 0)] public int MinFreeThreads { get { return (int)base[_propMinFreeThreads]; } set { base[_propMinFreeThreads] = value; } } [ConfigurationProperty("minLocalRequestFreeThreads", DefaultValue = 4)] [IntegerValidator(MinValue = 0)] public int MinLocalRequestFreeThreads { get { return (int)base[_propMinLocalRequestFreeThreads]; } set { base[_propMinLocalRequestFreeThreads] = value; } } [ConfigurationProperty("appRequestQueueLimit", DefaultValue = 5000)] [IntegerValidator(MinValue = 1)] public int AppRequestQueueLimit { get { return (int)base[_propAppRequestQueueLimit]; } set { base[_propAppRequestQueueLimit] = value; } } [ConfigurationProperty("enableKernelOutputCache", DefaultValue = true)] public bool EnableKernelOutputCache { get { return (bool)base[_propEnableKernelOutputCache]; } set { base[_propEnableKernelOutputCache] = value; } } [ConfigurationProperty("enableVersionHeader", DefaultValue = true)] public bool EnableVersionHeader { get { if (enableVersionHeaderCached == false) { enableVersionHeaderCache = (bool)base[_propEnableVersionHeader]; enableVersionHeaderCached = true; } return enableVersionHeaderCache; } set { base[_propEnableVersionHeader] = value; enableVersionHeaderCache = value; } } [ConfigurationProperty("apartmentThreading", DefaultValue = false)] public bool ApartmentThreading { get { return (bool)base[_propApartmentThreading]; } set { base[_propApartmentThreading] = value; } } [ConfigurationProperty("requireRootedSaveAsPath", DefaultValue = true)] public bool RequireRootedSaveAsPath { get { return (bool)base[_propRequireRootedSaveAsPath]; } set { base[_propRequireRootedSaveAsPath] = value; } } [ConfigurationProperty("enable", DefaultValue = true)] public bool Enable { get { return (bool)base[_propEnable]; } set { base[_propEnable] = value; } } [ConfigurationProperty("sendCacheControlHeader", DefaultValue = DefaultSendCacheControlHeader)] public bool SendCacheControlHeader { get { if (sendCacheControlHeaderCached == false) { sendCacheControlHeaderCache = (bool)base[_propSendCacheControlHeader]; sendCacheControlHeaderCached = true; } return sendCacheControlHeaderCache; } set { base[_propSendCacheControlHeader] = value; sendCacheControlHeaderCache = value; } } [ConfigurationProperty("shutdownTimeout", DefaultValue = "00:01:30")] [TypeConverter(typeof(TimeSpanSecondsConverter))] public TimeSpan ShutdownTimeout { get { return (TimeSpan)base[_propShutdownTimeout]; } set { base[_propShutdownTimeout] = value; } } [ConfigurationProperty("delayNotificationTimeout", DefaultValue = "00:00:00")] [TypeConverter(typeof(TimeSpanSecondsConverter))] public TimeSpan DelayNotificationTimeout { get { return (TimeSpan)base[_propDelayNotificationTimeout]; } set { base[_propDelayNotificationTimeout] = value; } } [ConfigurationProperty("waitChangeNotification", DefaultValue = 0)] [IntegerValidator(MinValue = 0)] public int WaitChangeNotification { get { return (int)base[_propWaitChangeNotification]; } set { base[_propWaitChangeNotification] = value; } } [ConfigurationProperty("maxWaitChangeNotification", DefaultValue = 0)] [IntegerValidator(MinValue = 0)] public int MaxWaitChangeNotification { get { return (int)base[_propMaxWaitChangeNotification]; } set { base[_propMaxWaitChangeNotification] = value; } } [ConfigurationProperty("enableHeaderChecking", DefaultValue = true)] public bool EnableHeaderChecking { get { return (bool)base[_propEnableHeaderChecking]; } set { base[_propEnableHeaderChecking] = value; } } [ConfigurationProperty("encoderType", DefaultValue = DefaultEncoderType)] [StringValidator(MinLength = 1)] public string EncoderType { get { return (string)base[_propEncoderType]; } set { base[_propEncoderType] = value; } } [ConfigurationProperty("requestValidationMode", DefaultValue = DefaultRequestValidationModeString)] [TypeConverter(typeof(VersionConverter))] public Version RequestValidationMode { get { if (_requestValidationMode == null) { _requestValidationMode = (Version)base[_propRequestValidationMode]; } return _requestValidationMode; } set { _requestValidationMode = value; base[_propRequestValidationMode] = value; } } [ConfigurationProperty("requestValidationType", DefaultValue = DefaultRequestValidationType)] [StringValidator(MinLength = 1)] public string RequestValidationType { get { return (string)base[_propRequestValidationType]; } set { base[_propRequestValidationType] = value; } } [ConfigurationProperty("requestPathInvalidCharacters", DefaultValue = DefaultRequestPathInvalidCharacters)] public string RequestPathInvalidCharacters { get { return (string)base[_propRequestPathInvalidCharacters]; } set { base[_propRequestPathInvalidCharacters] = value; _RequestPathInvalidCharactersArray = null; } } private int _MaxUrlLength = 0; [ConfigurationProperty("maxUrlLength", DefaultValue = DefaultMaxUrlLength)] [IntegerValidator(MinValue = 0)] public int MaxUrlLength { get { if (_MaxUrlLength == 0) _MaxUrlLength = (int)base[_propMaxUrlLength]; return _MaxUrlLength; } set { _MaxUrlLength = value; base[_propMaxUrlLength] = value; } } private int _MaxQueryStringLength = 0; [ConfigurationProperty("maxQueryStringLength", DefaultValue = DefaultMaxQueryStringLength)] [IntegerValidator(MinValue = 0)] public int MaxQueryStringLength { get { if (_MaxQueryStringLength == 0) _MaxQueryStringLength = (int)base[_propMaxQueryStringLength]; return _MaxQueryStringLength; } set { _MaxQueryStringLength = value; base[_propMaxQueryStringLength] = value; } } [ConfigurationProperty("relaxedUrlToFileSystemMapping", DefaultValue = DefaultRelaxedUrlToFileSystemMapping)] public bool RelaxedUrlToFileSystemMapping { get { return (bool)base[_propRelaxedUrlToFileSystemMapping]; } set { base[_propRelaxedUrlToFileSystemMapping] = value; } } private int BytesFromKilobytes(int kilobytes) { long maxLength = kilobytes * 1024L; return ((maxLength < Int32.MaxValue) ? (int)maxLength : Int32.MaxValue); } internal int MaxRequestLengthBytes { get { if (_MaxRequestLengthBytes < 0) { _MaxRequestLengthBytes = BytesFromKilobytes(MaxRequestLength); } return _MaxRequestLengthBytes; } } internal int RequestLengthDiskThresholdBytes { get { if (_RequestLengthDiskThresholdBytes < 0) { _RequestLengthDiskThresholdBytes = BytesFromKilobytes(RequestLengthDiskThreshold); } return _RequestLengthDiskThresholdBytes; } } internal String VersionHeader { get { if (!EnableVersionHeader) { return null; } if (s_versionHeader == null) { String header = null; // construct once (race condition here doesn't matter) try { String version = VersionInfo.SystemWebVersion; int i = version.LastIndexOf('.'); if (i > 0) { header = version.Substring(0, i); } } catch { } if (header == null) { header = String.Empty; } s_versionHeader = header; } return s_versionHeader; } } ////////////////////////////////////////////////////////////////// ////////////////////////////////////////////////////////////////// ////////////////////////////////////////////////////////////////// private char [] _RequestPathInvalidCharactersArray = null; internal char [] RequestPathInvalidCharactersArray { get { if (_RequestPathInvalidCharactersArray != null) return _RequestPathInvalidCharactersArray; _RequestPathInvalidCharactersArray = DecodeAndThenSplitString(RequestPathInvalidCharacters); if (_RequestPathInvalidCharactersArray == null) { // Maybe comma was one of the invalid chars, which can cause DecodeAndThenSplitString to fail // Split using the comma and then decode each part _RequestPathInvalidCharactersArray = SplitStringAndThenDecode(RequestPathInvalidCharacters); } if (_RequestPathInvalidCharactersArray == null) { // failed to construct invalid chars throw new ConfigurationErrorsException(SR.GetString(SR.Config_property_generic), ElementInformation.Properties[_propRequestPathInvalidCharacters.Name].Source, ElementInformation.Properties[_propRequestPathInvalidCharacters.Name].LineNumber); } return _RequestPathInvalidCharactersArray; } } ////////////////////////////////////////////////////////////////// ////////////////////////////////////////////////////////////////// private static char [] DecodeAndThenSplitString(string invalidCharString) { if (string.IsNullOrEmpty(invalidCharString)) { return new char[0]; } string [] stringsDecoded = HttpUtility.UrlDecode(invalidCharString, Encoding.UTF8).Split(','); char [] charsDecoded = new char[stringsDecoded.Length]; for(int iter=0; iter // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Configuration { using System; using System.Xml; using System.Configuration; using System.Collections.Specialized; using System.Collections; using System.Globalization; using System.IO; using System.Text; using System.Web.Util; using System.ComponentModel; using System.Security.Permissions; public sealed class HttpRuntimeSection : ConfigurationSection { #if !FEATURE_PAL // FEATURE_PAL-specific timeout values internal const int DefaultExecutionTimeout = 110; #else // !FEATURE_PAL // The timeout needs to be extended, since Coriolis/Rotor is much slower // especially platforms like Solaris wan't be able to process complicated // requests if in debug mode. // Remove or change this once the real timeout is known #if DEBUG internal const int DefaultExecutionTimeout = 110 * 10; #else // DEBUG internal const int DefaultExecutionTimeout = 110 * 5; #endif // DEBUG #endif // !FEATURE_PAL internal const int DefaultMaxRequestLength = 4096 * 1024; // 4MB internal const int DefaultRequestLengthDiskThreshold = 80 * 1024; // 80KB internal const int DefaultMinFreeThreads = 8; internal const int DefaultMinLocalRequestFreeThreads = 4; internal const int DefaultAppRequestQueueLimit = 100; internal const int DefaultShutdownTimeout = 90; internal const int DefaultDelayNotificationTimeout = 0; internal const int DefaultWaitChangeNotification = 0; internal const int DefaultMaxWaitChangeNotification = 0; internal const bool DefaultEnableKernelOutputCache = true; internal const bool DefaultRequireRootedSaveAsPath = true; internal const bool DefaultSendCacheControlHeader = true; internal const string DefaultEncoderType = "System.Web.Util.HttpEncoder"; internal static readonly Version DefaultRequestValidationMode = VersionUtil.Framework40; internal const string DefaultRequestValidationModeString = "4.0"; internal const string DefaultRequestValidationType = "System.Web.Util.RequestValidator"; internal const string DefaultRequestPathInvalidCharacters = "<,>,*,%,&,:,\\,?"; internal const int DefaultMaxUrlLength = 260; internal const int DefaultMaxQueryStringLength = 2048; internal const bool DefaultRelaxedUrlToFileSystemMapping = false; private bool enableVersionHeaderCache = true; private bool enableVersionHeaderCached = false; private TimeSpan executionTimeoutCache; private bool executionTimeoutCached = false; private bool sendCacheControlHeaderCached = false; private bool sendCacheControlHeaderCache; private static ConfigurationPropertyCollection _properties; private static readonly ConfigurationProperty _propExecutionTimeout = new ConfigurationProperty("executionTimeout", typeof(TimeSpan), TimeSpan.FromSeconds((double)DefaultExecutionTimeout), StdValidatorsAndConverters.TimeSpanSecondsConverter, StdValidatorsAndConverters.PositiveTimeSpanValidator, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propMaxRequestLength = new ConfigurationProperty("maxRequestLength", typeof(int), 4096, null, StdValidatorsAndConverters.NonZeroPositiveIntegerValidator, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propRequestLengthDiskThreshold = new ConfigurationProperty("requestLengthDiskThreshold", typeof(int), 80, null, StdValidatorsAndConverters.NonZeroPositiveIntegerValidator, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propUseFullyQualifiedRedirectUrl = new ConfigurationProperty("useFullyQualifiedRedirectUrl", typeof(bool), false, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propMinFreeThreads = new ConfigurationProperty("minFreeThreads", typeof(int), 8, null, StdValidatorsAndConverters.PositiveIntegerValidator, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propMinLocalRequestFreeThreads = new ConfigurationProperty("minLocalRequestFreeThreads", typeof(int), 4, null, StdValidatorsAndConverters.PositiveIntegerValidator, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propAppRequestQueueLimit = new ConfigurationProperty("appRequestQueueLimit", typeof(int), 5000, null, StdValidatorsAndConverters.NonZeroPositiveIntegerValidator, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propEnableKernelOutputCache = new ConfigurationProperty("enableKernelOutputCache", typeof(bool), true, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propEnableVersionHeader = new ConfigurationProperty("enableVersionHeader", typeof(bool), true, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propRequireRootedSaveAsPath = new ConfigurationProperty("requireRootedSaveAsPath", typeof(bool), true, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propEnable = new ConfigurationProperty("enable", typeof(bool), true, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propShutdownTimeout = new ConfigurationProperty("shutdownTimeout", typeof(TimeSpan), TimeSpan.FromSeconds((double)DefaultShutdownTimeout), StdValidatorsAndConverters.TimeSpanSecondsConverter, null, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propDelayNotificationTimeout = new ConfigurationProperty("delayNotificationTimeout", typeof(TimeSpan), TimeSpan.FromSeconds((double)DefaultDelayNotificationTimeout), StdValidatorsAndConverters.TimeSpanSecondsConverter, null, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propWaitChangeNotification = new ConfigurationProperty("waitChangeNotification", typeof(int), 0, null, StdValidatorsAndConverters.PositiveIntegerValidator, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propMaxWaitChangeNotification = new ConfigurationProperty("maxWaitChangeNotification", typeof(int), 0, null, StdValidatorsAndConverters.PositiveIntegerValidator, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propEnableHeaderChecking = new ConfigurationProperty("enableHeaderChecking", typeof(bool), true, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propSendCacheControlHeader = new ConfigurationProperty("sendCacheControlHeader", typeof(bool), DefaultSendCacheControlHeader, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propApartmentThreading = new ConfigurationProperty("apartmentThreading", typeof(bool), false, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propEncoderType = new ConfigurationProperty("encoderType", typeof(string), DefaultEncoderType, null, StdValidatorsAndConverters.NonEmptyStringValidator, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propRequestValidationMode = new ConfigurationProperty("requestValidationMode", typeof(Version), DefaultRequestValidationMode, StdValidatorsAndConverters.VersionConverter, null, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propRequestValidationType = new ConfigurationProperty("requestValidationType", typeof(string), DefaultRequestValidationType, null, StdValidatorsAndConverters.NonEmptyStringValidator, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propRequestPathInvalidCharacters = new ConfigurationProperty("requestPathInvalidCharacters", typeof(string), DefaultRequestPathInvalidCharacters, StdValidatorsAndConverters.WhiteSpaceTrimStringConverter, null, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propMaxUrlLength = new ConfigurationProperty("maxUrlLength", typeof(int), DefaultMaxUrlLength, null, new IntegerValidator(0, 2097151), // Max from VS 330766 ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propMaxQueryStringLength = new ConfigurationProperty("maxQueryStringLength", typeof(int), DefaultMaxQueryStringLength, null, new IntegerValidator(0, 2097151), // Max from VS 330766 ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propRelaxedUrlToFileSystemMapping = new ConfigurationProperty("relaxedUrlToFileSystemMapping", typeof(bool), DefaultRelaxedUrlToFileSystemMapping, ConfigurationPropertyOptions.None); private int _MaxRequestLengthBytes; private int _RequestLengthDiskThresholdBytes; private static String s_versionHeader = null; private Version _requestValidationMode; /* */ static HttpRuntimeSection() { // Property initialization _properties = new ConfigurationPropertyCollection(); _properties.Add(_propExecutionTimeout); _properties.Add(_propMaxRequestLength); _properties.Add(_propRequestLengthDiskThreshold); _properties.Add(_propUseFullyQualifiedRedirectUrl); _properties.Add(_propMinFreeThreads); _properties.Add(_propMinLocalRequestFreeThreads); _properties.Add(_propAppRequestQueueLimit); _properties.Add(_propEnableKernelOutputCache); _properties.Add(_propEnableVersionHeader); _properties.Add(_propRequireRootedSaveAsPath); _properties.Add(_propEnable); _properties.Add(_propShutdownTimeout); _properties.Add(_propDelayNotificationTimeout); _properties.Add(_propWaitChangeNotification); _properties.Add(_propMaxWaitChangeNotification); _properties.Add(_propEnableHeaderChecking); _properties.Add(_propSendCacheControlHeader); _properties.Add(_propApartmentThreading); _properties.Add(_propEncoderType); _properties.Add(_propRequestValidationMode); _properties.Add(_propRequestValidationType); _properties.Add(_propRequestPathInvalidCharacters); _properties.Add(_propMaxUrlLength); _properties.Add(_propMaxQueryStringLength); _properties.Add(_propRelaxedUrlToFileSystemMapping); } public HttpRuntimeSection() { _MaxRequestLengthBytes = -1; _RequestLengthDiskThresholdBytes = -1; } protected override ConfigurationPropertyCollection Properties { get { return _properties; } } [ConfigurationProperty("executionTimeout", DefaultValue = "00:01:50")] [TypeConverter(typeof(TimeSpanSecondsConverter))] [TimeSpanValidator(MinValueString="00:00:00", MaxValueString=TimeSpanValidatorAttribute.TimeSpanMaxValue)] public TimeSpan ExecutionTimeout { get { if (executionTimeoutCached == false) { executionTimeoutCache = (TimeSpan)base[_propExecutionTimeout]; executionTimeoutCached = true; } return executionTimeoutCache; } set { base[_propExecutionTimeout] = value; executionTimeoutCache = value; } } [ConfigurationProperty("maxRequestLength", DefaultValue = 4096)] [IntegerValidator(MinValue = 0)] public int MaxRequestLength { get { return (int)base[_propMaxRequestLength]; } set { if (value < RequestLengthDiskThreshold) { throw new ConfigurationErrorsException(SR.GetString( SR.Config_max_request_length_smaller_than_max_request_length_disk_threshold), ElementInformation.Properties[_propMaxRequestLength.Name].Source, ElementInformation.Properties[_propMaxRequestLength.Name].LineNumber); } base[_propMaxRequestLength] = value; } // } [ConfigurationProperty("requestLengthDiskThreshold", DefaultValue = 80)] [IntegerValidator(MinValue = 1)] public int RequestLengthDiskThreshold { get { return (int)base[_propRequestLengthDiskThreshold]; } set { if (value > MaxRequestLength) { throw new ConfigurationErrorsException(SR.GetString( SR.Config_max_request_length_disk_threshold_exceeds_max_request_length), ElementInformation.Properties[_propRequestLengthDiskThreshold.Name].Source, ElementInformation.Properties[_propRequestLengthDiskThreshold.Name].LineNumber); } base[_propRequestLengthDiskThreshold] = value; } } [ConfigurationProperty("useFullyQualifiedRedirectUrl", DefaultValue = false)] public bool UseFullyQualifiedRedirectUrl { get { return (bool)base[_propUseFullyQualifiedRedirectUrl]; } set { base[_propUseFullyQualifiedRedirectUrl] = value; } } [ConfigurationProperty("minFreeThreads", DefaultValue = 8)] [IntegerValidator(MinValue = 0)] public int MinFreeThreads { get { return (int)base[_propMinFreeThreads]; } set { base[_propMinFreeThreads] = value; } } [ConfigurationProperty("minLocalRequestFreeThreads", DefaultValue = 4)] [IntegerValidator(MinValue = 0)] public int MinLocalRequestFreeThreads { get { return (int)base[_propMinLocalRequestFreeThreads]; } set { base[_propMinLocalRequestFreeThreads] = value; } } [ConfigurationProperty("appRequestQueueLimit", DefaultValue = 5000)] [IntegerValidator(MinValue = 1)] public int AppRequestQueueLimit { get { return (int)base[_propAppRequestQueueLimit]; } set { base[_propAppRequestQueueLimit] = value; } } [ConfigurationProperty("enableKernelOutputCache", DefaultValue = true)] public bool EnableKernelOutputCache { get { return (bool)base[_propEnableKernelOutputCache]; } set { base[_propEnableKernelOutputCache] = value; } } [ConfigurationProperty("enableVersionHeader", DefaultValue = true)] public bool EnableVersionHeader { get { if (enableVersionHeaderCached == false) { enableVersionHeaderCache = (bool)base[_propEnableVersionHeader]; enableVersionHeaderCached = true; } return enableVersionHeaderCache; } set { base[_propEnableVersionHeader] = value; enableVersionHeaderCache = value; } } [ConfigurationProperty("apartmentThreading", DefaultValue = false)] public bool ApartmentThreading { get { return (bool)base[_propApartmentThreading]; } set { base[_propApartmentThreading] = value; } } [ConfigurationProperty("requireRootedSaveAsPath", DefaultValue = true)] public bool RequireRootedSaveAsPath { get { return (bool)base[_propRequireRootedSaveAsPath]; } set { base[_propRequireRootedSaveAsPath] = value; } } [ConfigurationProperty("enable", DefaultValue = true)] public bool Enable { get { return (bool)base[_propEnable]; } set { base[_propEnable] = value; } } [ConfigurationProperty("sendCacheControlHeader", DefaultValue = DefaultSendCacheControlHeader)] public bool SendCacheControlHeader { get { if (sendCacheControlHeaderCached == false) { sendCacheControlHeaderCache = (bool)base[_propSendCacheControlHeader]; sendCacheControlHeaderCached = true; } return sendCacheControlHeaderCache; } set { base[_propSendCacheControlHeader] = value; sendCacheControlHeaderCache = value; } } [ConfigurationProperty("shutdownTimeout", DefaultValue = "00:01:30")] [TypeConverter(typeof(TimeSpanSecondsConverter))] public TimeSpan ShutdownTimeout { get { return (TimeSpan)base[_propShutdownTimeout]; } set { base[_propShutdownTimeout] = value; } } [ConfigurationProperty("delayNotificationTimeout", DefaultValue = "00:00:00")] [TypeConverter(typeof(TimeSpanSecondsConverter))] public TimeSpan DelayNotificationTimeout { get { return (TimeSpan)base[_propDelayNotificationTimeout]; } set { base[_propDelayNotificationTimeout] = value; } } [ConfigurationProperty("waitChangeNotification", DefaultValue = 0)] [IntegerValidator(MinValue = 0)] public int WaitChangeNotification { get { return (int)base[_propWaitChangeNotification]; } set { base[_propWaitChangeNotification] = value; } } [ConfigurationProperty("maxWaitChangeNotification", DefaultValue = 0)] [IntegerValidator(MinValue = 0)] public int MaxWaitChangeNotification { get { return (int)base[_propMaxWaitChangeNotification]; } set { base[_propMaxWaitChangeNotification] = value; } } [ConfigurationProperty("enableHeaderChecking", DefaultValue = true)] public bool EnableHeaderChecking { get { return (bool)base[_propEnableHeaderChecking]; } set { base[_propEnableHeaderChecking] = value; } } [ConfigurationProperty("encoderType", DefaultValue = DefaultEncoderType)] [StringValidator(MinLength = 1)] public string EncoderType { get { return (string)base[_propEncoderType]; } set { base[_propEncoderType] = value; } } [ConfigurationProperty("requestValidationMode", DefaultValue = DefaultRequestValidationModeString)] [TypeConverter(typeof(VersionConverter))] public Version RequestValidationMode { get { if (_requestValidationMode == null) { _requestValidationMode = (Version)base[_propRequestValidationMode]; } return _requestValidationMode; } set { _requestValidationMode = value; base[_propRequestValidationMode] = value; } } [ConfigurationProperty("requestValidationType", DefaultValue = DefaultRequestValidationType)] [StringValidator(MinLength = 1)] public string RequestValidationType { get { return (string)base[_propRequestValidationType]; } set { base[_propRequestValidationType] = value; } } [ConfigurationProperty("requestPathInvalidCharacters", DefaultValue = DefaultRequestPathInvalidCharacters)] public string RequestPathInvalidCharacters { get { return (string)base[_propRequestPathInvalidCharacters]; } set { base[_propRequestPathInvalidCharacters] = value; _RequestPathInvalidCharactersArray = null; } } private int _MaxUrlLength = 0; [ConfigurationProperty("maxUrlLength", DefaultValue = DefaultMaxUrlLength)] [IntegerValidator(MinValue = 0)] public int MaxUrlLength { get { if (_MaxUrlLength == 0) _MaxUrlLength = (int)base[_propMaxUrlLength]; return _MaxUrlLength; } set { _MaxUrlLength = value; base[_propMaxUrlLength] = value; } } private int _MaxQueryStringLength = 0; [ConfigurationProperty("maxQueryStringLength", DefaultValue = DefaultMaxQueryStringLength)] [IntegerValidator(MinValue = 0)] public int MaxQueryStringLength { get { if (_MaxQueryStringLength == 0) _MaxQueryStringLength = (int)base[_propMaxQueryStringLength]; return _MaxQueryStringLength; } set { _MaxQueryStringLength = value; base[_propMaxQueryStringLength] = value; } } [ConfigurationProperty("relaxedUrlToFileSystemMapping", DefaultValue = DefaultRelaxedUrlToFileSystemMapping)] public bool RelaxedUrlToFileSystemMapping { get { return (bool)base[_propRelaxedUrlToFileSystemMapping]; } set { base[_propRelaxedUrlToFileSystemMapping] = value; } } private int BytesFromKilobytes(int kilobytes) { long maxLength = kilobytes * 1024L; return ((maxLength < Int32.MaxValue) ? (int)maxLength : Int32.MaxValue); } internal int MaxRequestLengthBytes { get { if (_MaxRequestLengthBytes < 0) { _MaxRequestLengthBytes = BytesFromKilobytes(MaxRequestLength); } return _MaxRequestLengthBytes; } } internal int RequestLengthDiskThresholdBytes { get { if (_RequestLengthDiskThresholdBytes < 0) { _RequestLengthDiskThresholdBytes = BytesFromKilobytes(RequestLengthDiskThreshold); } return _RequestLengthDiskThresholdBytes; } } internal String VersionHeader { get { if (!EnableVersionHeader) { return null; } if (s_versionHeader == null) { String header = null; // construct once (race condition here doesn't matter) try { String version = VersionInfo.SystemWebVersion; int i = version.LastIndexOf('.'); if (i > 0) { header = version.Substring(0, i); } } catch { } if (header == null) { header = String.Empty; } s_versionHeader = header; } return s_versionHeader; } } ////////////////////////////////////////////////////////////////// ////////////////////////////////////////////////////////////////// ////////////////////////////////////////////////////////////////// private char [] _RequestPathInvalidCharactersArray = null; internal char [] RequestPathInvalidCharactersArray { get { if (_RequestPathInvalidCharactersArray != null) return _RequestPathInvalidCharactersArray; _RequestPathInvalidCharactersArray = DecodeAndThenSplitString(RequestPathInvalidCharacters); if (_RequestPathInvalidCharactersArray == null) { // Maybe comma was one of the invalid chars, which can cause DecodeAndThenSplitString to fail // Split using the comma and then decode each part _RequestPathInvalidCharactersArray = SplitStringAndThenDecode(RequestPathInvalidCharacters); } if (_RequestPathInvalidCharactersArray == null) { // failed to construct invalid chars throw new ConfigurationErrorsException(SR.GetString(SR.Config_property_generic), ElementInformation.Properties[_propRequestPathInvalidCharacters.Name].Source, ElementInformation.Properties[_propRequestPathInvalidCharacters.Name].LineNumber); } return _RequestPathInvalidCharactersArray; } } ////////////////////////////////////////////////////////////////// ////////////////////////////////////////////////////////////////// private static char [] DecodeAndThenSplitString(string invalidCharString) { if (string.IsNullOrEmpty(invalidCharString)) { return new char[0]; } string [] stringsDecoded = HttpUtility.UrlDecode(invalidCharString, Encoding.UTF8).Split(','); char [] charsDecoded = new char[stringsDecoded.Length]; for(int iter=0; iter
Link Menu
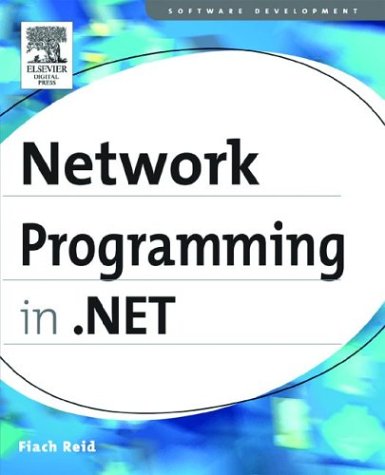
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ReaderWriterLockWrapper.cs
- ProcessThread.cs
- ClientSponsor.cs
- PrintPreviewDialog.cs
- InternalBufferOverflowException.cs
- OleDbSchemaGuid.cs
- ImagingCache.cs
- XsdCachingReader.cs
- TemplatedMailWebEventProvider.cs
- TreeViewAutomationPeer.cs
- DataGridViewToolTip.cs
- Environment.cs
- DataGridViewImageColumn.cs
- X509AsymmetricSecurityKey.cs
- StylusSystemGestureEventArgs.cs
- ComponentEditorPage.cs
- ResourceLoader.cs
- ColorContext.cs
- BaseHashHelper.cs
- Compress.cs
- PenThreadPool.cs
- FixedSOMContainer.cs
- AuthenticatingEventArgs.cs
- TableHeaderCell.cs
- CollectionViewProxy.cs
- _ChunkParse.cs
- FileDialog.cs
- UnmanagedMemoryStreamWrapper.cs
- DataGridViewCellValidatingEventArgs.cs
- Int64Converter.cs
- RestClientProxyHandler.cs
- ToolConsole.cs
- TextElementEnumerator.cs
- DataGridHeadersVisibilityToVisibilityConverter.cs
- AttachedPropertyInfo.cs
- PolicyUnit.cs
- QuaternionIndependentAnimationStorage.cs
- login.cs
- X509ThumbprintKeyIdentifierClause.cs
- HtmlInputFile.cs
- HttpListenerException.cs
- SecurityTokenProviderContainer.cs
- RepeaterDataBoundAdapter.cs
- safelinkcollection.cs
- AccessibleObject.cs
- BitmapPalette.cs
- ClientRuntimeConfig.cs
- TriggerBase.cs
- TripleDES.cs
- DrawToolTipEventArgs.cs
- SchemaComplexType.cs
- ControlDesignerState.cs
- Clock.cs
- SystemGatewayIPAddressInformation.cs
- ErrorRuntimeConfig.cs
- UidManager.cs
- DetailsView.cs
- HashMembershipCondition.cs
- WindowsEditBox.cs
- TreeNodeStyle.cs
- DesignTimeSiteMapProvider.cs
- StateRuntime.cs
- XmlUnspecifiedAttribute.cs
- AudioFileOut.cs
- Trustee.cs
- Marshal.cs
- ResourceLoader.cs
- ContextMenu.cs
- NonParentingControl.cs
- CodeAttributeDeclaration.cs
- TextEditorSpelling.cs
- BamlRecordWriter.cs
- TraceListener.cs
- DeclaredTypeElement.cs
- TableCellCollection.cs
- ISSmlParser.cs
- SqlRowUpdatedEvent.cs
- TreeView.cs
- SemanticAnalyzer.cs
- TableLayoutCellPaintEventArgs.cs
- GridItemCollection.cs
- PropertyGridEditorPart.cs
- ObjectNotFoundException.cs
- ZoneLinkButton.cs
- SortedDictionary.cs
- NativeMethods.cs
- ToolStripScrollButton.cs
- TypeInfo.cs
- CacheRequest.cs
- RandomNumberGenerator.cs
- QueryConverter.cs
- MobileControlsSectionHandler.cs
- Size3D.cs
- MouseEventArgs.cs
- FrameworkElementAutomationPeer.cs
- ToolStripDropDownMenu.cs
- ValueProviderWrapper.cs
- ListViewItem.cs
- MailMessageEventArgs.cs
- NativeMethodsOther.cs