Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / xsp / System / Web / UI / HtmlControls / HtmlTableRow.cs / 1305376 / HtmlTableRow.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.HtmlControls { using System; using System.Collections; using System.ComponentModel; using System.Globalization; using System.Web; using System.Web.UI; using System.Security.Permissions; ////// [ ParseChildren(true, "Cells") ] public class HtmlTableRow : HtmlContainerControl { HtmlTableCellCollection cells; public HtmlTableRow() : base("tr") { } ////// The ////// class defines the properties, methods, and events for the HtmlTableRow control. /// This class allows programmatic access on the server to individual HTML /// <tr> elements enclosed within an control. /// /// [ WebCategory("Layout"), DefaultValue(""), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public string Align { get { string s = Attributes["align"]; return((s != null) ? s : String.Empty); } set { Attributes["align"] = MapStringAttributeToString(value); } } /* * Collection of child TableCells. */ ////// Gets or sets the horizontal alignment of the cells contained in an /// ///control. /// /// [ Browsable(false), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), ] public virtual HtmlTableCellCollection Cells { get { if (cells == null) cells = new HtmlTableCellCollection(this); return cells; } } ////// Gets or sets the group of table cells contained within an /// ///control. /// /// [ WebCategory("Appearance"), DefaultValue(""), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public string BgColor { get { string s = Attributes["bgcolor"]; return((s != null) ? s : String.Empty); } set { Attributes["bgcolor"] = MapStringAttributeToString(value); } } ////// Gets or sets the background color of an ////// control. /// /// [ WebCategory("Appearance"), DefaultValue(""), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public string BorderColor { get { string s = Attributes["bordercolor"]; return((s != null) ? s : String.Empty); } set { Attributes["bordercolor"] = MapStringAttributeToString(value); } } ////// Gets or sets the border color of an ///control. /// /// [ WebCategory("Layout"), DefaultValue(""), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public string Height { get { string s = Attributes["height"]; return((s != null) ? s : String.Empty); } set { Attributes["height"] = MapStringAttributeToString(value); } } ////// Gets or sets the height of an ///control. /// /// public override string InnerHtml { get { throw new NotSupportedException(SR.GetString(SR.InnerHtml_not_supported, this.GetType().Name)); } set { throw new NotSupportedException(SR.GetString(SR.InnerHtml_not_supported, this.GetType().Name)); } } ///[To be supplied.] ////// public override string InnerText { get { throw new NotSupportedException(SR.GetString(SR.InnerText_not_supported, this.GetType().Name)); } set { throw new NotSupportedException(SR.GetString(SR.InnerText_not_supported, this.GetType().Name)); } } ///[To be supplied.] ////// [ WebCategory("Layout"), DefaultValue(""), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public string VAlign { get { string s = Attributes["valign"]; return((s != null) ? s : String.Empty); } set { Attributes["valign"] = MapStringAttributeToString(value); } } ////// Gets or sets the vertical alignment of of the cells contained in an /// ///control. /// /// /// protected internal override void RenderChildren(HtmlTextWriter writer) { writer.WriteLine(); writer.Indent++; base.RenderChildren(writer); writer.Indent--; } ////// /// protected override void RenderEndTag(HtmlTextWriter writer) { base.RenderEndTag(writer); writer.WriteLine(); } ////// protected override ControlCollection CreateControlCollection() { return new HtmlTableCellControlCollection(this); } ///[To be supplied.] ////// protected class HtmlTableCellControlCollection : ControlCollection { internal HtmlTableCellControlCollection (Control owner) : base(owner) { } ///[To be supplied.] ////// public override void Add(Control child) { if (child is HtmlTableCell) base.Add(child); else throw new ArgumentException(SR.GetString(SR.Cannot_Have_Children_Of_Type, "HtmlTableRow", child.GetType().Name.ToString(CultureInfo.InvariantCulture))); // throw an exception here } ///Adds the specified ///object to the collection. The new control is added /// to the end of the array. /// public override void AddAt(int index, Control child) { if (child is HtmlTableCell) base.AddAt(index, child); else throw new ArgumentException(SR.GetString(SR.Cannot_Have_Children_Of_Type, "HtmlTableRow", child.GetType().Name.ToString(CultureInfo.InvariantCulture))); // throw an exception here } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //Adds the specified ///object to the collection. The new control is added /// to the array at the specified index location. // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.HtmlControls { using System; using System.Collections; using System.ComponentModel; using System.Globalization; using System.Web; using System.Web.UI; using System.Security.Permissions; ////// [ ParseChildren(true, "Cells") ] public class HtmlTableRow : HtmlContainerControl { HtmlTableCellCollection cells; public HtmlTableRow() : base("tr") { } ////// The ////// class defines the properties, methods, and events for the HtmlTableRow control. /// This class allows programmatic access on the server to individual HTML /// <tr> elements enclosed within an control. /// /// [ WebCategory("Layout"), DefaultValue(""), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public string Align { get { string s = Attributes["align"]; return((s != null) ? s : String.Empty); } set { Attributes["align"] = MapStringAttributeToString(value); } } /* * Collection of child TableCells. */ ////// Gets or sets the horizontal alignment of the cells contained in an /// ///control. /// /// [ Browsable(false), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), ] public virtual HtmlTableCellCollection Cells { get { if (cells == null) cells = new HtmlTableCellCollection(this); return cells; } } ////// Gets or sets the group of table cells contained within an /// ///control. /// /// [ WebCategory("Appearance"), DefaultValue(""), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public string BgColor { get { string s = Attributes["bgcolor"]; return((s != null) ? s : String.Empty); } set { Attributes["bgcolor"] = MapStringAttributeToString(value); } } ////// Gets or sets the background color of an ////// control. /// /// [ WebCategory("Appearance"), DefaultValue(""), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public string BorderColor { get { string s = Attributes["bordercolor"]; return((s != null) ? s : String.Empty); } set { Attributes["bordercolor"] = MapStringAttributeToString(value); } } ////// Gets or sets the border color of an ///control. /// /// [ WebCategory("Layout"), DefaultValue(""), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public string Height { get { string s = Attributes["height"]; return((s != null) ? s : String.Empty); } set { Attributes["height"] = MapStringAttributeToString(value); } } ////// Gets or sets the height of an ///control. /// /// public override string InnerHtml { get { throw new NotSupportedException(SR.GetString(SR.InnerHtml_not_supported, this.GetType().Name)); } set { throw new NotSupportedException(SR.GetString(SR.InnerHtml_not_supported, this.GetType().Name)); } } ///[To be supplied.] ////// public override string InnerText { get { throw new NotSupportedException(SR.GetString(SR.InnerText_not_supported, this.GetType().Name)); } set { throw new NotSupportedException(SR.GetString(SR.InnerText_not_supported, this.GetType().Name)); } } ///[To be supplied.] ////// [ WebCategory("Layout"), DefaultValue(""), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public string VAlign { get { string s = Attributes["valign"]; return((s != null) ? s : String.Empty); } set { Attributes["valign"] = MapStringAttributeToString(value); } } ////// Gets or sets the vertical alignment of of the cells contained in an /// ///control. /// /// /// protected internal override void RenderChildren(HtmlTextWriter writer) { writer.WriteLine(); writer.Indent++; base.RenderChildren(writer); writer.Indent--; } ////// /// protected override void RenderEndTag(HtmlTextWriter writer) { base.RenderEndTag(writer); writer.WriteLine(); } ////// protected override ControlCollection CreateControlCollection() { return new HtmlTableCellControlCollection(this); } ///[To be supplied.] ////// protected class HtmlTableCellControlCollection : ControlCollection { internal HtmlTableCellControlCollection (Control owner) : base(owner) { } ///[To be supplied.] ////// public override void Add(Control child) { if (child is HtmlTableCell) base.Add(child); else throw new ArgumentException(SR.GetString(SR.Cannot_Have_Children_Of_Type, "HtmlTableRow", child.GetType().Name.ToString(CultureInfo.InvariantCulture))); // throw an exception here } ///Adds the specified ///object to the collection. The new control is added /// to the end of the array. /// public override void AddAt(int index, Control child) { if (child is HtmlTableCell) base.AddAt(index, child); else throw new ArgumentException(SR.GetString(SR.Cannot_Have_Children_Of_Type, "HtmlTableRow", child.GetType().Name.ToString(CultureInfo.InvariantCulture))); // throw an exception here } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.Adds the specified ///object to the collection. The new control is added /// to the array at the specified index location.
Link Menu
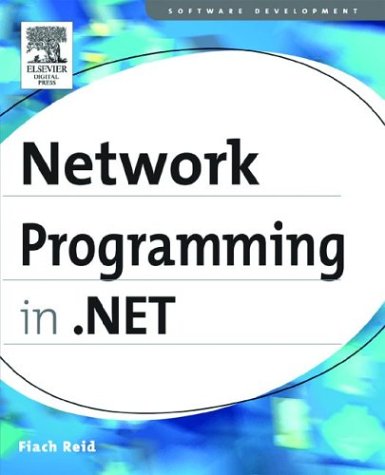
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Matrix.cs
- SqlUDTStorage.cs
- CodeCatchClauseCollection.cs
- ScrollableControl.cs
- ManipulationStartingEventArgs.cs
- CollectionViewProxy.cs
- TargetException.cs
- StylusButton.cs
- WebPartsSection.cs
- Attachment.cs
- Soap.cs
- ThreadAttributes.cs
- ApplicationDirectoryMembershipCondition.cs
- __ComObject.cs
- Part.cs
- XmlNode.cs
- TreeNodeStyle.cs
- SqlPersistenceWorkflowInstanceDescription.cs
- RepeaterItemEventArgs.cs
- PropertyNames.cs
- SelfSignedCertificate.cs
- RowUpdatingEventArgs.cs
- VisemeEventArgs.cs
- GatewayIPAddressInformationCollection.cs
- LinearQuaternionKeyFrame.cs
- Identity.cs
- securitycriticaldataClass.cs
- AttributeQuery.cs
- EllipseGeometry.cs
- EntityContainerEntitySet.cs
- OdbcConnectionPoolProviderInfo.cs
- SecurityTokenTypes.cs
- CollectionConverter.cs
- _ListenerResponseStream.cs
- BrowserCapabilitiesFactoryBase.cs
- ValueQuery.cs
- ClientTargetSection.cs
- MatrixUtil.cs
- WebPartManager.cs
- IUnknownConstantAttribute.cs
- CacheDict.cs
- TreeViewAutomationPeer.cs
- HtmlEncodedRawTextWriter.cs
- RangeValidator.cs
- EdmConstants.cs
- HttpCacheParams.cs
- ObjectViewEntityCollectionData.cs
- StrongTypingException.cs
- CertificateElement.cs
- WeakEventTable.cs
- RbTree.cs
- SequenceNumber.cs
- PersonalizationProviderHelper.cs
- XPathConvert.cs
- FormDesigner.cs
- AutoCompleteStringCollection.cs
- FolderLevelBuildProviderCollection.cs
- XmlSubtreeReader.cs
- Pens.cs
- SqlHelper.cs
- HttpWebRequest.cs
- XslNumber.cs
- _UriTypeConverter.cs
- DockPattern.cs
- DetailsViewUpdateEventArgs.cs
- ResourceLoader.cs
- _ConnectOverlappedAsyncResult.cs
- PrintingPermission.cs
- SqlException.cs
- RuntimeConfigLKG.cs
- InitiatorSessionSymmetricMessageSecurityProtocol.cs
- RepeatButton.cs
- XmlStringTable.cs
- XPathDocument.cs
- APCustomTypeDescriptor.cs
- ZipIOCentralDirectoryDigitalSignature.cs
- MsmqIntegrationAppDomainProtocolHandler.cs
- IssuerInformation.cs
- ValueUtilsSmi.cs
- SymmetricCryptoHandle.cs
- ASCIIEncoding.cs
- ReadOnlyPermissionSet.cs
- MultiAsyncResult.cs
- ConnectionOrientedTransportChannelListener.cs
- IndentedWriter.cs
- ValueChangedEventManager.cs
- ListBox.cs
- ZipArchive.cs
- ImportFileRequest.cs
- ScriptingWebServicesSectionGroup.cs
- FlowDocumentPaginator.cs
- MessageTraceRecord.cs
- HandlerFactoryCache.cs
- NetTcpBindingElement.cs
- dtdvalidator.cs
- UnknownWrapper.cs
- DesignerVerbCollection.cs
- BooleanAnimationUsingKeyFrames.cs
- DrawingState.cs
- AttributeProviderAttribute.cs