Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Framework / System / Windows / Markup / XamlInt32CollectionSerializer.cs / 1 / XamlInt32CollectionSerializer.cs
//---------------------------------------------------------------------------- // // File: XamlInt32CollectionSerializer.cs // // Description: // XamlSerializer used to persist collections of integer indices in Baml // // Copyright (C) 2006 by Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System; using System.Collections; using System.ComponentModel; using System.ComponentModel.Design; using System.ComponentModel.Design.Serialization; using System.Diagnostics; using System.Globalization; using System.IO; using System.Xml; using MS.Utility; using MS.Internal; #if PBTCOMPILER using System.Reflection; using System.Collections.Generic; namespace MS.Internal.Markup #else using System.Windows; using System.Windows.Media; using System.Windows.Media.Media3D; using MS.Internal.Media; namespace System.Windows.Markup #endif { ////// XamlInt32CollectionSerializer is used to persist collections of integer indices in Baml /// internal class XamlInt32CollectionSerializer : XamlSerializer { // // Internal only class. // We actually create a class here - to avoid jitting for use of struct/value type // #if PBTCOMPILER internal class IntegerMarkup { internal IntegerMarkup( int value) { _value = value; } internal int Value { get { return _value; } } int _value; } #endif #region Construction ////// Constructor for XamlInt32CollectionSerializer /// public XamlInt32CollectionSerializer() { } #endregion Construction #region Conversions ////// Convert a string into a compact binary representation and write it out /// to the passed BinaryWriter. /// public override bool ConvertStringToCustomBinary ( BinaryWriter writer, // Writer into the baml stream string stringValue) // String to convert { #if PBTCOMPILER Listints = Parse( stringValue); #else Int32Collection ints = Int32Collection.Parse( stringValue ); #endif int cur, last , count, max = 0 ; count = ints.Count ; // loop through the collection testing for // if the numbers are consecutive, and what's the max. bool consecutive = true; bool allPositive = true ; for ( int i = 1; i < count; i++) { #if PBTCOMPILER last = ints[i-1].Value; cur = ints[i].Value; #else last = ints.Internal_GetItem(i-1); cur = ints.Internal_GetItem(i); #endif if ( consecutive && ( last + 1 != cur )) { consecutive = false; } // // If any number is negative - we will just use Integer type. // // We could handle this by encoding the min/max and creating a different number of bit encoding. // For now - we're seeing enough gains with this change. // if ( cur < 0 ) { allPositive = false ; } if ( cur > max ) { max = cur; } } if ( consecutive ) { writer.Write( (byte) IntegerCollectionType.Consecutive ); writer.Write( count ); // Write the count // Write the first number. #if PBTCOMPILER writer.Write( ints[0].Value ); #else writer.Write( ints.Internal_GetItem(0)); #endif } else { IntegerCollectionType type; if ( allPositive && max <= 255 ) { type = IntegerCollectionType.Byte; } else if ( allPositive && max <= UInt16.MaxValue ) { type = IntegerCollectionType.UShort; } else { type = IntegerCollectionType.Integer; } writer.Write( (byte) type ); writer.Write( count ); // Write the count switch( type ) { case IntegerCollectionType.Byte: { for( int i = 0; i < count; i++ ) { writer.Write( (byte) #if PBTCOMPILER ints[i].Value #else ints.Internal_GetItem(i) #endif ) ; } } break; case IntegerCollectionType.UShort: { for( int i = 0; i < count; i++ ) { writer.Write( (ushort) #if PBTCOMPILER ints[i].Value #else ints.Internal_GetItem(i) #endif ); } } break; case IntegerCollectionType.Integer: { for( int i = 0; i < count; i++ ) { writer.Write( #if PBTCOMPILER ints[i].Value #else ints.Internal_GetItem(i) #endif ); } } break; } } return true; } #if PBTCOMPILER public static List Parse(string source) { IFormatProvider formatProvider = CultureInfo.GetCultureInfo("en-us"); TokenizerHelper th = new TokenizerHelper(source, formatProvider); List resource = new List (); int value; while (th.NextToken()) { value = Convert.ToInt32(th.GetCurrentToken(), formatProvider); resource.Add( new IntegerMarkup(value) ); } return resource; } #endif #if !PBTCOMPILER /// /// Convert a compact binary representation of a collection /// into a Point3DCollection into and instance /// ////// This is called ONLY from the Parser and is not a general public method. /// public override object ConvertCustomBinaryToObject( BinaryReader reader) { return DeserializeFrom( reader ); } ////// Convert a compact binary representation of a collection /// into a Point3DCollection into and instance /// ////// This is called ONLY from the Parser and is not a general public method. /// public static object StaticConvertCustomBinaryToObject( BinaryReader reader) { return DeserializeFrom( reader ); } private static Int32Collection DeserializeFrom( BinaryReader reader ) { Int32Collection theCollection; IntegerCollectionType type; type = (IntegerCollectionType) reader.ReadByte(); int count = reader.ReadInt32(); if ( count < 0 ) { throw new ArgumentException(SR.Get(SRID.IntegerCollectionLengthLessThanZero)); } theCollection = new Int32Collection( count ); if ( type == IntegerCollectionType.Consecutive ) { // Get the first integer int first = reader.ReadInt32(); for( int i = 0; i < count; i ++) { theCollection.Add( first + i ); } } else { switch( type ) { case IntegerCollectionType.Byte : { for ( int i = 0; i < count; i++ ) { theCollection.Add( (int) reader.ReadByte()); } } break; case IntegerCollectionType.UShort : { for ( int i = 0; i < count; i++ ) { theCollection.Add( (int) reader.ReadUInt16()); } } break; case IntegerCollectionType.Integer : { for ( int i = 0; i < count; i++ ) { int value = reader.ReadInt32(); theCollection.Add( value); } } break; default: throw new ArgumentException(SR.Get(SRID.UnknownIndexType)); } } return theCollection; } #endif #endregion Conversions internal enum IntegerCollectionType : byte { Unknown = 0, Consecutive = 1, Byte = 2, UShort= 3, Integer= 4 } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // File: XamlInt32CollectionSerializer.cs // // Description: // XamlSerializer used to persist collections of integer indices in Baml // // Copyright (C) 2006 by Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System; using System.Collections; using System.ComponentModel; using System.ComponentModel.Design; using System.ComponentModel.Design.Serialization; using System.Diagnostics; using System.Globalization; using System.IO; using System.Xml; using MS.Utility; using MS.Internal; #if PBTCOMPILER using System.Reflection; using System.Collections.Generic; namespace MS.Internal.Markup #else using System.Windows; using System.Windows.Media; using System.Windows.Media.Media3D; using MS.Internal.Media; namespace System.Windows.Markup #endif { ////// XamlInt32CollectionSerializer is used to persist collections of integer indices in Baml /// internal class XamlInt32CollectionSerializer : XamlSerializer { // // Internal only class. // We actually create a class here - to avoid jitting for use of struct/value type // #if PBTCOMPILER internal class IntegerMarkup { internal IntegerMarkup( int value) { _value = value; } internal int Value { get { return _value; } } int _value; } #endif #region Construction ////// Constructor for XamlInt32CollectionSerializer /// public XamlInt32CollectionSerializer() { } #endregion Construction #region Conversions ////// Convert a string into a compact binary representation and write it out /// to the passed BinaryWriter. /// public override bool ConvertStringToCustomBinary ( BinaryWriter writer, // Writer into the baml stream string stringValue) // String to convert { #if PBTCOMPILER Listints = Parse( stringValue); #else Int32Collection ints = Int32Collection.Parse( stringValue ); #endif int cur, last , count, max = 0 ; count = ints.Count ; // loop through the collection testing for // if the numbers are consecutive, and what's the max. bool consecutive = true; bool allPositive = true ; for ( int i = 1; i < count; i++) { #if PBTCOMPILER last = ints[i-1].Value; cur = ints[i].Value; #else last = ints.Internal_GetItem(i-1); cur = ints.Internal_GetItem(i); #endif if ( consecutive && ( last + 1 != cur )) { consecutive = false; } // // If any number is negative - we will just use Integer type. // // We could handle this by encoding the min/max and creating a different number of bit encoding. // For now - we're seeing enough gains with this change. // if ( cur < 0 ) { allPositive = false ; } if ( cur > max ) { max = cur; } } if ( consecutive ) { writer.Write( (byte) IntegerCollectionType.Consecutive ); writer.Write( count ); // Write the count // Write the first number. #if PBTCOMPILER writer.Write( ints[0].Value ); #else writer.Write( ints.Internal_GetItem(0)); #endif } else { IntegerCollectionType type; if ( allPositive && max <= 255 ) { type = IntegerCollectionType.Byte; } else if ( allPositive && max <= UInt16.MaxValue ) { type = IntegerCollectionType.UShort; } else { type = IntegerCollectionType.Integer; } writer.Write( (byte) type ); writer.Write( count ); // Write the count switch( type ) { case IntegerCollectionType.Byte: { for( int i = 0; i < count; i++ ) { writer.Write( (byte) #if PBTCOMPILER ints[i].Value #else ints.Internal_GetItem(i) #endif ) ; } } break; case IntegerCollectionType.UShort: { for( int i = 0; i < count; i++ ) { writer.Write( (ushort) #if PBTCOMPILER ints[i].Value #else ints.Internal_GetItem(i) #endif ); } } break; case IntegerCollectionType.Integer: { for( int i = 0; i < count; i++ ) { writer.Write( #if PBTCOMPILER ints[i].Value #else ints.Internal_GetItem(i) #endif ); } } break; } } return true; } #if PBTCOMPILER public static List Parse(string source) { IFormatProvider formatProvider = CultureInfo.GetCultureInfo("en-us"); TokenizerHelper th = new TokenizerHelper(source, formatProvider); List resource = new List (); int value; while (th.NextToken()) { value = Convert.ToInt32(th.GetCurrentToken(), formatProvider); resource.Add( new IntegerMarkup(value) ); } return resource; } #endif #if !PBTCOMPILER /// /// Convert a compact binary representation of a collection /// into a Point3DCollection into and instance /// ////// This is called ONLY from the Parser and is not a general public method. /// public override object ConvertCustomBinaryToObject( BinaryReader reader) { return DeserializeFrom( reader ); } ////// Convert a compact binary representation of a collection /// into a Point3DCollection into and instance /// ////// This is called ONLY from the Parser and is not a general public method. /// public static object StaticConvertCustomBinaryToObject( BinaryReader reader) { return DeserializeFrom( reader ); } private static Int32Collection DeserializeFrom( BinaryReader reader ) { Int32Collection theCollection; IntegerCollectionType type; type = (IntegerCollectionType) reader.ReadByte(); int count = reader.ReadInt32(); if ( count < 0 ) { throw new ArgumentException(SR.Get(SRID.IntegerCollectionLengthLessThanZero)); } theCollection = new Int32Collection( count ); if ( type == IntegerCollectionType.Consecutive ) { // Get the first integer int first = reader.ReadInt32(); for( int i = 0; i < count; i ++) { theCollection.Add( first + i ); } } else { switch( type ) { case IntegerCollectionType.Byte : { for ( int i = 0; i < count; i++ ) { theCollection.Add( (int) reader.ReadByte()); } } break; case IntegerCollectionType.UShort : { for ( int i = 0; i < count; i++ ) { theCollection.Add( (int) reader.ReadUInt16()); } } break; case IntegerCollectionType.Integer : { for ( int i = 0; i < count; i++ ) { int value = reader.ReadInt32(); theCollection.Add( value); } } break; default: throw new ArgumentException(SR.Get(SRID.UnknownIndexType)); } } return theCollection; } #endif #endregion Conversions internal enum IntegerCollectionType : byte { Unknown = 0, Consecutive = 1, Byte = 2, UShort= 3, Integer= 4 } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
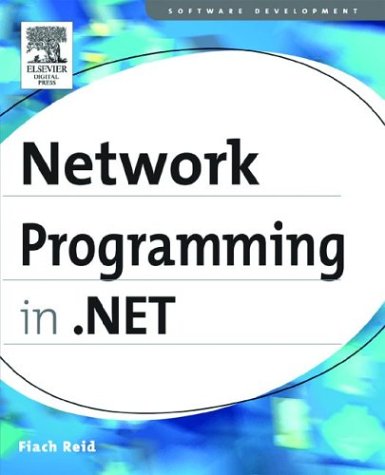
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- HMACSHA1.cs
- PrefixHandle.cs
- CodeDirectoryCompiler.cs
- FormsAuthenticationConfiguration.cs
- DecoderExceptionFallback.cs
- OptionUsage.cs
- AssociationType.cs
- NonValidatingSecurityTokenAuthenticator.cs
- KeyTime.cs
- DependencyPropertyKey.cs
- SimplePropertyEntry.cs
- XmlSigningNodeWriter.cs
- EntityTemplateFactory.cs
- EntityContainerEmitter.cs
- CanonicalizationDriver.cs
- SpeakCompletedEventArgs.cs
- AttachedAnnotation.cs
- CallbackHandler.cs
- BoundPropertyEntry.cs
- RangeBase.cs
- BoundField.cs
- ToolStripContentPanelRenderEventArgs.cs
- ResourceSetExpression.cs
- QilStrConcat.cs
- Misc.cs
- CaseKeyBox.ViewModel.cs
- ValueTypeFixupInfo.cs
- ReadWriteObjectLock.cs
- DataViewSettingCollection.cs
- QueryContinueDragEvent.cs
- BamlTreeUpdater.cs
- InplaceBitmapMetadataWriter.cs
- Rotation3D.cs
- SqlUtils.cs
- PackageRelationship.cs
- PassportAuthenticationModule.cs
- Int64Animation.cs
- OracleEncoding.cs
- CollectionViewGroupRoot.cs
- TypeUtils.cs
- TypefaceMetricsCache.cs
- FixedSOMTextRun.cs
- XmlSchemaFacet.cs
- OleDbStruct.cs
- XmlQuerySequence.cs
- XmlCharCheckingReader.cs
- Clipboard.cs
- SystemUnicastIPAddressInformation.cs
- HttpContext.cs
- DocumentXPathNavigator.cs
- Span.cs
- Base64Stream.cs
- AsyncStreamReader.cs
- Visual.cs
- Region.cs
- _BaseOverlappedAsyncResult.cs
- ItemCheckedEvent.cs
- Soap.cs
- SymLanguageType.cs
- Inline.cs
- AffineTransform3D.cs
- GlyphInfoList.cs
- OutKeywords.cs
- TablePatternIdentifiers.cs
- ResourceReferenceExpressionConverter.cs
- AdapterUtil.cs
- SpecialNameAttribute.cs
- ComponentSerializationService.cs
- JapaneseLunisolarCalendar.cs
- GeneralTransform.cs
- RegularExpressionValidator.cs
- ZipFileInfoCollection.cs
- HttpRuntimeSection.cs
- LinqDataSourceInsertEventArgs.cs
- SqlCharStream.cs
- PrinterResolution.cs
- HelpKeywordAttribute.cs
- WebServiceHandlerFactory.cs
- Content.cs
- XamlFigureLengthSerializer.cs
- TimeSpan.cs
- XmlNodeReader.cs
- DebugHandleTracker.cs
- ColorKeyFrameCollection.cs
- BindingCollection.cs
- SafeThreadHandle.cs
- FontConverter.cs
- GenericIdentity.cs
- ThemeDirectoryCompiler.cs
- AudioDeviceOut.cs
- HttpCapabilitiesBase.cs
- ConsumerConnectionPointCollection.cs
- VectorCollectionValueSerializer.cs
- ElementHost.cs
- ButtonColumn.cs
- ArrangedElement.cs
- CompositeActivityCodeGenerator.cs
- DateBoldEvent.cs
- SizeKeyFrameCollection.cs
- PackWebRequest.cs