Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / Xml / System / Xml / schema / XmlSchemaFacet.cs / 1 / XmlSchemaFacet.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace System.Xml.Schema { using System.ComponentModel; using System.Xml.Serialization; internal enum FacetType { None, Length, MinLength, MaxLength, Pattern, Whitespace, Enumeration, MinExclusive, MinInclusive, MaxExclusive, MaxInclusive, TotalDigits, FractionDigits, } ///public abstract class XmlSchemaFacet : XmlSchemaAnnotated { string value; bool isFixed; FacetType facetType; /// [XmlAttribute("value")] public string Value { get { return this.value; } set { this.value = value; } } /// [XmlAttribute("fixed"), DefaultValue(false)] public virtual bool IsFixed { get { return isFixed; } set { if (!(this is XmlSchemaEnumerationFacet) && !(this is XmlSchemaPatternFacet)) { isFixed = value; } } } internal FacetType FacetType { get { return facetType; } set { facetType = value; } } } /// public abstract class XmlSchemaNumericFacet : XmlSchemaFacet { } /// public class XmlSchemaLengthFacet : XmlSchemaNumericFacet { public XmlSchemaLengthFacet() { FacetType = FacetType.Length; } } /// public class XmlSchemaMinLengthFacet : XmlSchemaNumericFacet { public XmlSchemaMinLengthFacet() { FacetType = FacetType.MinLength; } } /// public class XmlSchemaMaxLengthFacet : XmlSchemaNumericFacet { public XmlSchemaMaxLengthFacet() { FacetType = FacetType.MaxLength; } } /// public class XmlSchemaPatternFacet : XmlSchemaFacet { public XmlSchemaPatternFacet() { FacetType = FacetType.Pattern; } } /// public class XmlSchemaEnumerationFacet : XmlSchemaFacet { public XmlSchemaEnumerationFacet() { FacetType = FacetType.Enumeration; } } /// public class XmlSchemaMinExclusiveFacet : XmlSchemaFacet { public XmlSchemaMinExclusiveFacet() { FacetType = FacetType.MinExclusive; } } /// public class XmlSchemaMinInclusiveFacet : XmlSchemaFacet { public XmlSchemaMinInclusiveFacet() { FacetType = FacetType.MinInclusive; } } /// public class XmlSchemaMaxExclusiveFacet : XmlSchemaFacet { public XmlSchemaMaxExclusiveFacet() { FacetType = FacetType.MaxExclusive; } } /// public class XmlSchemaMaxInclusiveFacet : XmlSchemaFacet { public XmlSchemaMaxInclusiveFacet() { FacetType = FacetType.MaxInclusive; } } /// public class XmlSchemaTotalDigitsFacet : XmlSchemaNumericFacet { public XmlSchemaTotalDigitsFacet() { FacetType = FacetType.TotalDigits; } } /// public class XmlSchemaFractionDigitsFacet : XmlSchemaNumericFacet { public XmlSchemaFractionDigitsFacet() { FacetType = FacetType.FractionDigits; } } /// public class XmlSchemaWhiteSpaceFacet : XmlSchemaFacet { public XmlSchemaWhiteSpaceFacet() { FacetType = FacetType.Whitespace; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace System.Xml.Schema { using System.ComponentModel; using System.Xml.Serialization; internal enum FacetType { None, Length, MinLength, MaxLength, Pattern, Whitespace, Enumeration, MinExclusive, MinInclusive, MaxExclusive, MaxInclusive, TotalDigits, FractionDigits, } ///public abstract class XmlSchemaFacet : XmlSchemaAnnotated { string value; bool isFixed; FacetType facetType; /// [XmlAttribute("value")] public string Value { get { return this.value; } set { this.value = value; } } /// [XmlAttribute("fixed"), DefaultValue(false)] public virtual bool IsFixed { get { return isFixed; } set { if (!(this is XmlSchemaEnumerationFacet) && !(this is XmlSchemaPatternFacet)) { isFixed = value; } } } internal FacetType FacetType { get { return facetType; } set { facetType = value; } } } /// public abstract class XmlSchemaNumericFacet : XmlSchemaFacet { } /// public class XmlSchemaLengthFacet : XmlSchemaNumericFacet { public XmlSchemaLengthFacet() { FacetType = FacetType.Length; } } /// public class XmlSchemaMinLengthFacet : XmlSchemaNumericFacet { public XmlSchemaMinLengthFacet() { FacetType = FacetType.MinLength; } } /// public class XmlSchemaMaxLengthFacet : XmlSchemaNumericFacet { public XmlSchemaMaxLengthFacet() { FacetType = FacetType.MaxLength; } } /// public class XmlSchemaPatternFacet : XmlSchemaFacet { public XmlSchemaPatternFacet() { FacetType = FacetType.Pattern; } } /// public class XmlSchemaEnumerationFacet : XmlSchemaFacet { public XmlSchemaEnumerationFacet() { FacetType = FacetType.Enumeration; } } /// public class XmlSchemaMinExclusiveFacet : XmlSchemaFacet { public XmlSchemaMinExclusiveFacet() { FacetType = FacetType.MinExclusive; } } /// public class XmlSchemaMinInclusiveFacet : XmlSchemaFacet { public XmlSchemaMinInclusiveFacet() { FacetType = FacetType.MinInclusive; } } /// public class XmlSchemaMaxExclusiveFacet : XmlSchemaFacet { public XmlSchemaMaxExclusiveFacet() { FacetType = FacetType.MaxExclusive; } } /// public class XmlSchemaMaxInclusiveFacet : XmlSchemaFacet { public XmlSchemaMaxInclusiveFacet() { FacetType = FacetType.MaxInclusive; } } /// public class XmlSchemaTotalDigitsFacet : XmlSchemaNumericFacet { public XmlSchemaTotalDigitsFacet() { FacetType = FacetType.TotalDigits; } } /// public class XmlSchemaFractionDigitsFacet : XmlSchemaNumericFacet { public XmlSchemaFractionDigitsFacet() { FacetType = FacetType.FractionDigits; } } /// public class XmlSchemaWhiteSpaceFacet : XmlSchemaFacet { public XmlSchemaWhiteSpaceFacet() { FacetType = FacetType.Whitespace; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
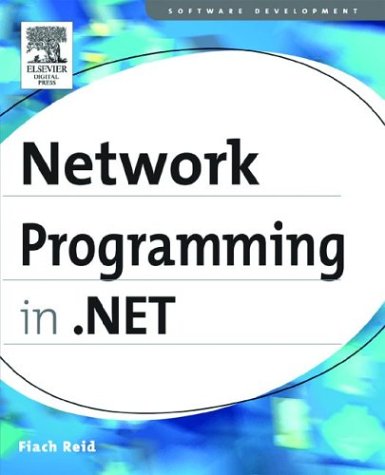
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TimelineGroup.cs
- Tracking.cs
- TemplateManager.cs
- ControlPager.cs
- Margins.cs
- ProtocolInformationReader.cs
- SchemaNotation.cs
- TypeForwardedToAttribute.cs
- PlacementWorkspace.cs
- DataGridViewColumnEventArgs.cs
- EmptyReadOnlyDictionaryInternal.cs
- DataRelationCollection.cs
- SkipQueryOptionExpression.cs
- ClientScriptManagerWrapper.cs
- ObjectMemberMapping.cs
- TTSVoice.cs
- PreProcessInputEventArgs.cs
- CqlParserHelpers.cs
- TraceData.cs
- TypographyProperties.cs
- ResolveMatchesApril2005.cs
- AtomParser.cs
- RelationshipConverter.cs
- Clause.cs
- NetworkCredential.cs
- DataContractSerializerFaultFormatter.cs
- ActiveXHelper.cs
- SqlParameterCollection.cs
- _SslStream.cs
- Html32TextWriter.cs
- ListViewAutomationPeer.cs
- ToolBarButtonClickEvent.cs
- ISAPIWorkerRequest.cs
- ReadOnlyDataSourceView.cs
- SqlUserDefinedTypeAttribute.cs
- DrawingVisualDrawingContext.cs
- XmlAutoDetectWriter.cs
- IsolatedStorageFile.cs
- FieldAccessException.cs
- WaitHandle.cs
- WebPartRestoreVerb.cs
- XMLSyntaxException.cs
- TimeoutConverter.cs
- RowToParametersTransformer.cs
- GridPattern.cs
- GroupBox.cs
- InProcStateClientManager.cs
- XmlDictionary.cs
- MimeObjectFactory.cs
- MouseEventArgs.cs
- XPathPatternBuilder.cs
- IdentifierCollection.cs
- AutomationEvent.cs
- FixUpCollection.cs
- TreeNodeStyleCollection.cs
- PropertyCondition.cs
- WebPartVerbsEventArgs.cs
- ResourceReader.cs
- VSWCFServiceContractGenerator.cs
- WebServiceReceiveDesigner.cs
- HierarchicalDataTemplate.cs
- ColorContext.cs
- DataTableCollection.cs
- SubclassTypeValidatorAttribute.cs
- SoapObjectWriter.cs
- DesignParameter.cs
- CompleteWizardStep.cs
- OleCmdHelper.cs
- XmlTextAttribute.cs
- MergeLocalizationDirectives.cs
- ErrorFormatterPage.cs
- SettingsPropertyValue.cs
- PropertyContainer.cs
- EncoderBestFitFallback.cs
- RichTextBoxAutomationPeer.cs
- UIElement3D.cs
- TableLayoutStyleCollection.cs
- DesignerDataStoredProcedure.cs
- TcpChannelHelper.cs
- InstanceKeyView.cs
- TextSearch.cs
- TraceListeners.cs
- DbConnectionPoolIdentity.cs
- DbProviderFactories.cs
- WebPartChrome.cs
- XPathNodeIterator.cs
- BinHexEncoding.cs
- GregorianCalendar.cs
- HtmlTextArea.cs
- StackOverflowException.cs
- GlyphShapingProperties.cs
- SpotLight.cs
- AxHostDesigner.cs
- SafeReadContext.cs
- SettingsPropertyCollection.cs
- PageWrapper.cs
- sqlinternaltransaction.cs
- InstanceKeyCollisionException.cs
- AttributeUsageAttribute.cs
- DataGridTable.cs