Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Core / CSharp / System / Windows / TextDecorationCollectionConverter.cs / 1 / TextDecorationCollectionConverter.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: TextDecorationCollectionConverter class // // History: // 2/23/2004: Garyyang Created the file // //--------------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Globalization; using System.Reflection; using System.Security; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows { ////// TypeConverter for TextDecorationCollection /// public sealed class TextDecorationCollectionConverter : TypeConverter { ////// CanConvertTo method /// /// ITypeDescriptorContext /// Type to convert to ///false will always be returned because TextDecorations cannot be converted to any other type. public override bool CanConvertTo(ITypeDescriptorContext context, Type destinationType) { if (destinationType == typeof(InstanceDescriptor)) { return true; } // return false for any other target type. Don't call base.CanConvertTo() because it would be confusing // in some cases. For example, for destination typeof(String), base convertor just converts the TDC to the // string full name of the type. return false; } ////// CanConvertFrom /// /// ITypeDescriptorContext /// Type to convert to ///true if it can convert from sourceType to TextDecorations, false otherwise public override bool CanConvertFrom(ITypeDescriptorContext context, Type sourceType) { if (sourceType == typeof(string)) { return true; } return false; } ////// ConvertFrom /// /// ITypeDescriptorContext /// CultureInfo /// The input object to be converted to TextDecorations ///the converted value of the input object public override object ConvertFrom(ITypeDescriptorContext context, CultureInfo culture, object input) { if (input == null) { throw GetConvertFromException(input); } string value = input as string; if (null == value) { throw new ArgumentException(SR.Get(SRID.General_BadType, "ConvertFrom"), "input"); } return ConvertFromString(value); } ////// ConvertFromString /// /// The string to be converted into TextDecorationCollection object ///the converted value of the string flag ////// The text parameter can be either string "None" or a combination of the predefined /// TextDecoration names delimited by commas (,). One or more blanks spaces can precede /// or follow each text decoration name or comma. There can't be duplicate TextDecoration names in the /// string. The operation is case-insensitive. /// public static new TextDecorationCollection ConvertFromString(string text) { if (text == null) { return null; } TextDecorationCollection textDecorations = new TextDecorationCollection(); // Flags indicating which Predefined textDecoration has alrady been added. byte MatchedTextDecorationFlags = 0; // Start from the 1st non-whitespace character // Negative index means error is encountered int index = AdvanceToNextNonWhiteSpace(text, 0); while (index >= 0 && index < text.Length) { if (Match(None, text, index)) { // Matched "None" in the input index = AdvanceToNextNonWhiteSpace(text, index + None.Length); if (textDecorations.Count > 0 || index < text.Length) { // Error: "None" can only be specified by its own index = -1; } } else { // Match the input with one of the predefined text decoration names int i; for(i = 0; i < TextDecorationNames.Length && !Match(TextDecorationNames[i], text, index); i++ ); if (i < TextDecorationNames.Length) { // Found a match within the predefined names if ((MatchedTextDecorationFlags & (1 << i)) > 0) { // Error: The matched value is duplicated. index = -1; } else { // Valid match. Add to the collection and remember that this text decoration // has been added textDecorations.Add(PredefinedTextDecorations[i]); MatchedTextDecorationFlags |= (byte)(1 << i); // Advance to the start of next name index = AdvanceToNextNameStart(text, index + TextDecorationNames[i].Length); } } else { // Error: no match found in the predefined names index = -1; } } } if (index < 0) { throw new ArgumentException(SR.Get(SRID.InvalidTextDecorationCollectionString, text)); } return textDecorations; } ////// ConvertTo /// /// ITypeDescriptorContext /// CultureInfo /// the object to be converted to another type /// The destination type of the conversion ///null will always be returned because TextDecorations cannot be converted to any other type. ////// Critical: calls InstanceDescriptor ctor which LinkDemands /// PublicOK: can only make an InstanceDescriptor for TextDecoration, not an arbitrary class /// [SecurityCritical] public override object ConvertTo(ITypeDescriptorContext context, CultureInfo culture, object value, Type destinationType) { if (destinationType == typeof(InstanceDescriptor) && value is IEnumerable) { ConstructorInfo ci = typeof(TextDecorationCollection).GetConstructor( new Type[]{typeof(IEnumerable )} ); return new InstanceDescriptor(ci, new object[]{value}); } // Pass unhandled cases to base class (which will throw exceptions for null value or destinationType.) return base.ConvertTo(context, culture, value, destinationType); } //--------------------------------- // Private methods //--------------------------------- /// /// Match the input against a predefined pattern from certain index onwards /// private static bool Match(string pattern, string input, int index) { int i = 0; for (; i < pattern.Length && index + i < input.Length && pattern[i] == Char.ToUpperInvariant(input[index + i]); i++) ; return (i == pattern.Length); } ////// Advance to the start of next name /// private static int AdvanceToNextNameStart(string input, int index) { // Two names must be seperated by a comma and optionally spaces int separator = AdvanceToNextNonWhiteSpace(input, index); int nextNameStart; if (separator >= input.Length) { // reach the end nextNameStart = input.Length; } else { if (input[separator] == Separator) { nextNameStart = AdvanceToNextNonWhiteSpace(input, separator + 1); if (nextNameStart >= input.Length) { // Error: Separator is at the end of the input nextNameStart = -1; } } else { // Error: There is a non-whitespace, non-separator character following // the matched value nextNameStart = -1; } } return nextNameStart; } ////// Advance to the next non-whitespace character /// private static int AdvanceToNextNonWhiteSpace(string input, int index) { for (; index < input.Length && Char.IsWhiteSpace(input[index]); index++) ; return (index > input.Length) ? input.Length : index; } //--------------------------------- // Private members //--------------------------------- // // Predefined valid names for TextDecorations // Names should be normalized to be upper case // private const string None = "NONE"; private const char Separator = ','; private static readonly string[] TextDecorationNames = new string[] { "OVERLINE", "BASELINE", "UNDERLINE", "STRIKETHROUGH" }; // Predefined TextDecorationCollection values. It should match // the TextDecorationNames array private static readonly TextDecorationCollection[] PredefinedTextDecorations = new TextDecorationCollection[] { TextDecorations.OverLine, TextDecorations.Baseline, TextDecorations.Underline, TextDecorations.Strikethrough }; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: TextDecorationCollectionConverter class // // History: // 2/23/2004: Garyyang Created the file // //--------------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Globalization; using System.Reflection; using System.Security; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows { ////// TypeConverter for TextDecorationCollection /// public sealed class TextDecorationCollectionConverter : TypeConverter { ////// CanConvertTo method /// /// ITypeDescriptorContext /// Type to convert to ///false will always be returned because TextDecorations cannot be converted to any other type. public override bool CanConvertTo(ITypeDescriptorContext context, Type destinationType) { if (destinationType == typeof(InstanceDescriptor)) { return true; } // return false for any other target type. Don't call base.CanConvertTo() because it would be confusing // in some cases. For example, for destination typeof(String), base convertor just converts the TDC to the // string full name of the type. return false; } ////// CanConvertFrom /// /// ITypeDescriptorContext /// Type to convert to ///true if it can convert from sourceType to TextDecorations, false otherwise public override bool CanConvertFrom(ITypeDescriptorContext context, Type sourceType) { if (sourceType == typeof(string)) { return true; } return false; } ////// ConvertFrom /// /// ITypeDescriptorContext /// CultureInfo /// The input object to be converted to TextDecorations ///the converted value of the input object public override object ConvertFrom(ITypeDescriptorContext context, CultureInfo culture, object input) { if (input == null) { throw GetConvertFromException(input); } string value = input as string; if (null == value) { throw new ArgumentException(SR.Get(SRID.General_BadType, "ConvertFrom"), "input"); } return ConvertFromString(value); } ////// ConvertFromString /// /// The string to be converted into TextDecorationCollection object ///the converted value of the string flag ////// The text parameter can be either string "None" or a combination of the predefined /// TextDecoration names delimited by commas (,). One or more blanks spaces can precede /// or follow each text decoration name or comma. There can't be duplicate TextDecoration names in the /// string. The operation is case-insensitive. /// public static new TextDecorationCollection ConvertFromString(string text) { if (text == null) { return null; } TextDecorationCollection textDecorations = new TextDecorationCollection(); // Flags indicating which Predefined textDecoration has alrady been added. byte MatchedTextDecorationFlags = 0; // Start from the 1st non-whitespace character // Negative index means error is encountered int index = AdvanceToNextNonWhiteSpace(text, 0); while (index >= 0 && index < text.Length) { if (Match(None, text, index)) { // Matched "None" in the input index = AdvanceToNextNonWhiteSpace(text, index + None.Length); if (textDecorations.Count > 0 || index < text.Length) { // Error: "None" can only be specified by its own index = -1; } } else { // Match the input with one of the predefined text decoration names int i; for(i = 0; i < TextDecorationNames.Length && !Match(TextDecorationNames[i], text, index); i++ ); if (i < TextDecorationNames.Length) { // Found a match within the predefined names if ((MatchedTextDecorationFlags & (1 << i)) > 0) { // Error: The matched value is duplicated. index = -1; } else { // Valid match. Add to the collection and remember that this text decoration // has been added textDecorations.Add(PredefinedTextDecorations[i]); MatchedTextDecorationFlags |= (byte)(1 << i); // Advance to the start of next name index = AdvanceToNextNameStart(text, index + TextDecorationNames[i].Length); } } else { // Error: no match found in the predefined names index = -1; } } } if (index < 0) { throw new ArgumentException(SR.Get(SRID.InvalidTextDecorationCollectionString, text)); } return textDecorations; } ////// ConvertTo /// /// ITypeDescriptorContext /// CultureInfo /// the object to be converted to another type /// The destination type of the conversion ///null will always be returned because TextDecorations cannot be converted to any other type. ////// Critical: calls InstanceDescriptor ctor which LinkDemands /// PublicOK: can only make an InstanceDescriptor for TextDecoration, not an arbitrary class /// [SecurityCritical] public override object ConvertTo(ITypeDescriptorContext context, CultureInfo culture, object value, Type destinationType) { if (destinationType == typeof(InstanceDescriptor) && value is IEnumerable) { ConstructorInfo ci = typeof(TextDecorationCollection).GetConstructor( new Type[]{typeof(IEnumerable )} ); return new InstanceDescriptor(ci, new object[]{value}); } // Pass unhandled cases to base class (which will throw exceptions for null value or destinationType.) return base.ConvertTo(context, culture, value, destinationType); } //--------------------------------- // Private methods //--------------------------------- /// /// Match the input against a predefined pattern from certain index onwards /// private static bool Match(string pattern, string input, int index) { int i = 0; for (; i < pattern.Length && index + i < input.Length && pattern[i] == Char.ToUpperInvariant(input[index + i]); i++) ; return (i == pattern.Length); } ////// Advance to the start of next name /// private static int AdvanceToNextNameStart(string input, int index) { // Two names must be seperated by a comma and optionally spaces int separator = AdvanceToNextNonWhiteSpace(input, index); int nextNameStart; if (separator >= input.Length) { // reach the end nextNameStart = input.Length; } else { if (input[separator] == Separator) { nextNameStart = AdvanceToNextNonWhiteSpace(input, separator + 1); if (nextNameStart >= input.Length) { // Error: Separator is at the end of the input nextNameStart = -1; } } else { // Error: There is a non-whitespace, non-separator character following // the matched value nextNameStart = -1; } } return nextNameStart; } ////// Advance to the next non-whitespace character /// private static int AdvanceToNextNonWhiteSpace(string input, int index) { for (; index < input.Length && Char.IsWhiteSpace(input[index]); index++) ; return (index > input.Length) ? input.Length : index; } //--------------------------------- // Private members //--------------------------------- // // Predefined valid names for TextDecorations // Names should be normalized to be upper case // private const string None = "NONE"; private const char Separator = ','; private static readonly string[] TextDecorationNames = new string[] { "OVERLINE", "BASELINE", "UNDERLINE", "STRIKETHROUGH" }; // Predefined TextDecorationCollection values. It should match // the TextDecorationNames array private static readonly TextDecorationCollection[] PredefinedTextDecorations = new TextDecorationCollection[] { TextDecorations.OverLine, TextDecorations.Baseline, TextDecorations.Underline, TextDecorations.Strikethrough }; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
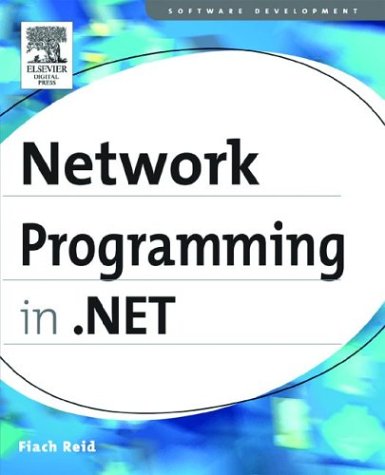
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- StyleConverter.cs
- PKCS1MaskGenerationMethod.cs
- DBConnectionString.cs
- SystemIPAddressInformation.cs
- InfocardExtendedInformationCollection.cs
- DmlSqlGenerator.cs
- iisPickupDirectory.cs
- OverflowException.cs
- _NetworkingPerfCounters.cs
- CustomErrorCollection.cs
- XmlNodeChangedEventArgs.cs
- InheritanceRules.cs
- SettingsProviderCollection.cs
- EarlyBoundInfo.cs
- SourceFilter.cs
- DataBindingExpressionBuilder.cs
- SectionInput.cs
- ThemeDirectoryCompiler.cs
- cookiecontainer.cs
- XPathNodeList.cs
- HttpCookieCollection.cs
- SafeMarshalContext.cs
- TrackingParticipant.cs
- DesignUtil.cs
- XmlMtomWriter.cs
- HttpCapabilitiesSectionHandler.cs
- SchemaTableOptionalColumn.cs
- ProfileService.cs
- MarshalByValueComponent.cs
- LayoutTable.cs
- SqlBulkCopy.cs
- TypeExtensionSerializer.cs
- SecureUICommand.cs
- OdbcUtils.cs
- exports.cs
- ServiceModelStringsVersion1.cs
- XmlSchemaIdentityConstraint.cs
- DesignerInterfaces.cs
- PreProcessor.cs
- ObjectRef.cs
- JpegBitmapDecoder.cs
- EllipseGeometry.cs
- DataGridViewImageCell.cs
- WebPartActionVerb.cs
- WizardSideBarListControlItemEventArgs.cs
- Scheduler.cs
- ListBox.cs
- OperatingSystem.cs
- StringSource.cs
- RowSpanVector.cs
- MSHTMLHost.cs
- X509Extension.cs
- HttpCookieCollection.cs
- Visual3DCollection.cs
- ProcessModelInfo.cs
- MetadataItemEmitter.cs
- CachedBitmap.cs
- WeakRefEnumerator.cs
- BooleanToVisibilityConverter.cs
- PointCollection.cs
- NextPreviousPagerField.cs
- ProfileModule.cs
- AuthenticationConfig.cs
- MetadataItem_Static.cs
- Message.cs
- ReadOnlyHierarchicalDataSource.cs
- PropagatorResult.cs
- ConstrainedDataObject.cs
- CultureSpecificStringDictionary.cs
- ServiceParser.cs
- PageContent.cs
- DataGridViewDataConnection.cs
- VirtualizedCellInfoCollection.cs
- RelationshipManager.cs
- ThicknessAnimationUsingKeyFrames.cs
- DrawingContextWalker.cs
- SqlFormatter.cs
- HttpCachePolicyWrapper.cs
- BuildManager.cs
- ApplicationFileCodeDomTreeGenerator.cs
- CompoundFileIOPermission.cs
- _OverlappedAsyncResult.cs
- HyperLinkDataBindingHandler.cs
- TypeToStringValueConverter.cs
- TreeNodeEventArgs.cs
- BufferBuilder.cs
- SplayTreeNode.cs
- RegexFCD.cs
- ClientUtils.cs
- EntityContainerEmitter.cs
- Condition.cs
- xmlsaver.cs
- WinFormsSpinner.cs
- ScrollPattern.cs
- ModelItemImpl.cs
- ErrorRuntimeConfig.cs
- ProcessHostFactoryHelper.cs
- SrgsNameValueTag.cs
- DataRowExtensions.cs
- WebException.cs