Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / Net / System / Net / Sockets / _OverlappedAsyncResult.cs / 1 / _OverlappedAsyncResult.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Net.Sockets { using System; using System.Net; using System.Runtime.InteropServices; using System.Threading; using Microsoft.Win32; using System.Collections.Generic; // // OverlappedAsyncResult - used to take care of storage for async Socket operation // from the BeginSend, BeginSendTo, BeginReceive, BeginReceiveFrom calls. // internal class OverlappedAsyncResult: BaseOverlappedAsyncResult { // // internal class members // private SocketAddress m_SocketAddress; private SocketAddress m_SocketAddressOriginal; // needed for partial BeginReceiveFrom/EndReceiveFrom completion // These two are used only as alternatives internal WSABuffer m_SingleBuffer; internal WSABuffer[] m_WSABuffers; // // the following two will be used only on WinNT to enable completion ports // // // Constructor. We take in the socket that's creating us, the caller's // state object, and the buffer on which the I/O will be performed. // We save the socket and state, pin the callers's buffer, and allocate // an event for the WaitHandle. // internal OverlappedAsyncResult(Socket socket, Object asyncState, AsyncCallback asyncCallback) : base(socket, asyncState, asyncCallback) { } // internal IntPtr GetSocketAddressPtr() { return Marshal.UnsafeAddrOfPinnedArrayElement(m_SocketAddress.m_Buffer, 0); } // internal IntPtr GetSocketAddressSizePtr() { return Marshal.UnsafeAddrOfPinnedArrayElement(m_SocketAddress.m_Buffer, m_SocketAddress.GetAddressSizeOffset()); } // internal SocketAddress SocketAddress { get { return m_SocketAddress; } } // internal SocketAddress SocketAddressOriginal { get { return m_SocketAddressOriginal; } set { m_SocketAddressOriginal = value; } } // // SetUnmanagedStructures - // Fills in Overlapped Structures used in an Async Overlapped Winsock call // these calls are outside the runtime and are unmanaged code, so we need // to prepare specific structures and ints that lie in unmanaged memory // since the Overlapped calls can be Async // internal void SetUnmanagedStructures(byte[] buffer, int offset, int size, SocketAddress socketAddress, bool pinSocketAddress) { // // Fill in Buffer Array structure that will be used for our send/recv Buffer // m_SocketAddress = socketAddress; if (pinSocketAddress && m_SocketAddress != null) { object[] objectsToPin = null; objectsToPin = new object[2]; objectsToPin[0] = buffer; m_SocketAddress.CopyAddressSizeIntoBuffer(); objectsToPin[1] = m_SocketAddress.m_Buffer; base.SetUnmanagedStructures(objectsToPin); } else { base.SetUnmanagedStructures(buffer); } m_SingleBuffer.Length = size; m_SingleBuffer.Pointer = Marshal.UnsafeAddrOfPinnedArrayElement(buffer, offset); } internal void SetUnmanagedStructures(byte[] buffer, int offset, int size, SocketAddress socketAddress, bool pinSocketAddress, ref OverlappedCache overlappedCache) { SetupCache(ref overlappedCache); SetUnmanagedStructures(buffer, offset, size, socketAddress, pinSocketAddress); } internal void SetUnmanagedStructures(BufferOffsetSize[] buffers) { // // Fill in Buffer Array structure that will be used for our send/recv Buffer // m_WSABuffers = new WSABuffer[buffers.Length]; object[] objectsToPin = new object[buffers.Length]; for (int i = 0; i < buffers.Length; i++) objectsToPin[i] = buffers[i].Buffer; // has to be called now to pin memory base.SetUnmanagedStructures(objectsToPin); for (int i = 0; i < buffers.Length; i++) { m_WSABuffers[i].Length = buffers[i].Size; m_WSABuffers[i].Pointer = Marshal.UnsafeAddrOfPinnedArrayElement(buffers[i].Buffer, buffers[i].Offset); } } internal void SetUnmanagedStructures(BufferOffsetSize[] buffers, ref OverlappedCache overlappedCache) { SetupCache(ref overlappedCache); SetUnmanagedStructures(buffers); } internal void SetUnmanagedStructures(IList> buffers) { // Fill in Buffer Array structure that will be used for our send/recv Buffer // //make sure we don't let the app mess up the buffer array enough to cause //corruption. int count = buffers.Count; ArraySegment [] buffersCopy = new ArraySegment [count]; for (int i=0;i > buffers, ref OverlappedCache overlappedCache) { SetupCache(ref overlappedCache); SetUnmanagedStructures(buffers); } // // This method is called after an asynchronous call is made for the user, // it checks and acts accordingly if the IO: // 1) completed synchronously. // 2) was pended. // 3) failed. // internal override object PostCompletion(int numBytes) { if (ErrorCode == 0) { if(Logging.On)LogBuffer(numBytes); } return (int)numBytes; } void LogBuffer(int size) { GlobalLog.Assert(Logging.On, "OverlappedAsyncResult#{0}::LogBuffer()|Logging is off!", ValidationHelper.HashString(this)); if (size > -1) { if (m_WSABuffers != null) { foreach (WSABuffer wsaBuffer in m_WSABuffers) { Logging.Dump(Logging.Sockets, AsyncObject, "PostCompletion", wsaBuffer.Pointer, Math.Min(wsaBuffer.Length, size)); if ((size -= wsaBuffer.Length) <=0) break; } } else { Logging.Dump(Logging.Sockets, AsyncObject, "PostCompletion", m_SingleBuffer.Pointer, Math.Min(m_SingleBuffer.Length, size)); } } } }; // class OverlappedAsyncResult } // namespace System.Net.Sockets // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Net.Sockets { using System; using System.Net; using System.Runtime.InteropServices; using System.Threading; using Microsoft.Win32; using System.Collections.Generic; // // OverlappedAsyncResult - used to take care of storage for async Socket operation // from the BeginSend, BeginSendTo, BeginReceive, BeginReceiveFrom calls. // internal class OverlappedAsyncResult: BaseOverlappedAsyncResult { // // internal class members // private SocketAddress m_SocketAddress; private SocketAddress m_SocketAddressOriginal; // needed for partial BeginReceiveFrom/EndReceiveFrom completion // These two are used only as alternatives internal WSABuffer m_SingleBuffer; internal WSABuffer[] m_WSABuffers; // // the following two will be used only on WinNT to enable completion ports // // // Constructor. We take in the socket that's creating us, the caller's // state object, and the buffer on which the I/O will be performed. // We save the socket and state, pin the callers's buffer, and allocate // an event for the WaitHandle. // internal OverlappedAsyncResult(Socket socket, Object asyncState, AsyncCallback asyncCallback) : base(socket, asyncState, asyncCallback) { } // internal IntPtr GetSocketAddressPtr() { return Marshal.UnsafeAddrOfPinnedArrayElement(m_SocketAddress.m_Buffer, 0); } // internal IntPtr GetSocketAddressSizePtr() { return Marshal.UnsafeAddrOfPinnedArrayElement(m_SocketAddress.m_Buffer, m_SocketAddress.GetAddressSizeOffset()); } // internal SocketAddress SocketAddress { get { return m_SocketAddress; } } // internal SocketAddress SocketAddressOriginal { get { return m_SocketAddressOriginal; } set { m_SocketAddressOriginal = value; } } // // SetUnmanagedStructures - // Fills in Overlapped Structures used in an Async Overlapped Winsock call // these calls are outside the runtime and are unmanaged code, so we need // to prepare specific structures and ints that lie in unmanaged memory // since the Overlapped calls can be Async // internal void SetUnmanagedStructures(byte[] buffer, int offset, int size, SocketAddress socketAddress, bool pinSocketAddress) { // // Fill in Buffer Array structure that will be used for our send/recv Buffer // m_SocketAddress = socketAddress; if (pinSocketAddress && m_SocketAddress != null) { object[] objectsToPin = null; objectsToPin = new object[2]; objectsToPin[0] = buffer; m_SocketAddress.CopyAddressSizeIntoBuffer(); objectsToPin[1] = m_SocketAddress.m_Buffer; base.SetUnmanagedStructures(objectsToPin); } else { base.SetUnmanagedStructures(buffer); } m_SingleBuffer.Length = size; m_SingleBuffer.Pointer = Marshal.UnsafeAddrOfPinnedArrayElement(buffer, offset); } internal void SetUnmanagedStructures(byte[] buffer, int offset, int size, SocketAddress socketAddress, bool pinSocketAddress, ref OverlappedCache overlappedCache) { SetupCache(ref overlappedCache); SetUnmanagedStructures(buffer, offset, size, socketAddress, pinSocketAddress); } internal void SetUnmanagedStructures(BufferOffsetSize[] buffers) { // // Fill in Buffer Array structure that will be used for our send/recv Buffer // m_WSABuffers = new WSABuffer[buffers.Length]; object[] objectsToPin = new object[buffers.Length]; for (int i = 0; i < buffers.Length; i++) objectsToPin[i] = buffers[i].Buffer; // has to be called now to pin memory base.SetUnmanagedStructures(objectsToPin); for (int i = 0; i < buffers.Length; i++) { m_WSABuffers[i].Length = buffers[i].Size; m_WSABuffers[i].Pointer = Marshal.UnsafeAddrOfPinnedArrayElement(buffers[i].Buffer, buffers[i].Offset); } } internal void SetUnmanagedStructures(BufferOffsetSize[] buffers, ref OverlappedCache overlappedCache) { SetupCache(ref overlappedCache); SetUnmanagedStructures(buffers); } internal void SetUnmanagedStructures(IList> buffers) { // Fill in Buffer Array structure that will be used for our send/recv Buffer // //make sure we don't let the app mess up the buffer array enough to cause //corruption. int count = buffers.Count; ArraySegment [] buffersCopy = new ArraySegment [count]; for (int i=0;i > buffers, ref OverlappedCache overlappedCache) { SetupCache(ref overlappedCache); SetUnmanagedStructures(buffers); } // // This method is called after an asynchronous call is made for the user, // it checks and acts accordingly if the IO: // 1) completed synchronously. // 2) was pended. // 3) failed. // internal override object PostCompletion(int numBytes) { if (ErrorCode == 0) { if(Logging.On)LogBuffer(numBytes); } return (int)numBytes; } void LogBuffer(int size) { GlobalLog.Assert(Logging.On, "OverlappedAsyncResult#{0}::LogBuffer()|Logging is off!", ValidationHelper.HashString(this)); if (size > -1) { if (m_WSABuffers != null) { foreach (WSABuffer wsaBuffer in m_WSABuffers) { Logging.Dump(Logging.Sockets, AsyncObject, "PostCompletion", wsaBuffer.Pointer, Math.Min(wsaBuffer.Length, size)); if ((size -= wsaBuffer.Length) <=0) break; } } else { Logging.Dump(Logging.Sockets, AsyncObject, "PostCompletion", m_SingleBuffer.Pointer, Math.Min(m_SingleBuffer.Length, size)); } } } }; // class OverlappedAsyncResult } // namespace System.Net.Sockets // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
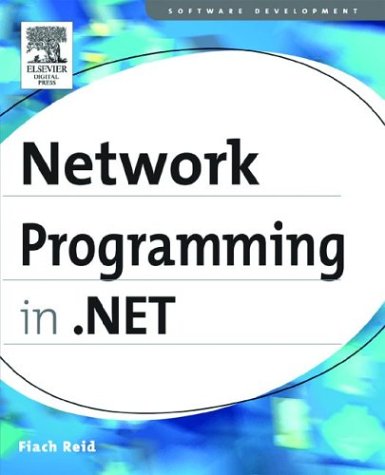
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataServiceProcessingPipeline.cs
- DataSourceControlBuilder.cs
- UnmanagedMarshal.cs
- XmlAnyElementAttributes.cs
- PasswordRecovery.cs
- TripleDESCryptoServiceProvider.cs
- Parser.cs
- SqlFacetAttribute.cs
- LabelInfo.cs
- HttpClientCertificate.cs
- EntityDataSourceQueryBuilder.cs
- DataGrid.cs
- DataMisalignedException.cs
- Models.cs
- SafeNativeMethods.cs
- DragDrop.cs
- XmlArrayAttribute.cs
- DetailsViewRowCollection.cs
- GPRECTF.cs
- SubpageParagraph.cs
- SamlSubjectStatement.cs
- DBPropSet.cs
- PersonalizationProvider.cs
- DataGridViewCheckBoxCell.cs
- Win32PrintDialog.cs
- Triplet.cs
- SqlProviderManifest.cs
- DbConnectionStringBuilder.cs
- StylusPointPropertyInfo.cs
- ContractCodeDomInfo.cs
- HttpListenerElement.cs
- DeviceSpecificDialogCachedState.cs
- HttpDictionary.cs
- CngKeyCreationParameters.cs
- AnnotationResource.cs
- CDSsyncETWBCLProvider.cs
- ConnectionStringEditor.cs
- CodePageEncoding.cs
- StringStorage.cs
- XmlDocumentFragment.cs
- SimpleApplicationHost.cs
- FrameworkElementAutomationPeer.cs
- DataServiceResponse.cs
- FontDifferentiator.cs
- WriteableOnDemandPackagePart.cs
- Rfc4050KeyFormatter.cs
- SqlEnums.cs
- RuleProcessor.cs
- EntryWrittenEventArgs.cs
- KeyGestureConverter.cs
- CngKeyBlobFormat.cs
- ElementUtil.cs
- CorrelationService.cs
- SpStreamWrapper.cs
- XPathAxisIterator.cs
- ArrayWithOffset.cs
- CodeTypeReferenceCollection.cs
- ObjectView.cs
- ComponentSerializationService.cs
- CfgParser.cs
- Inflater.cs
- StateMachineHistory.cs
- PortCache.cs
- ToolboxItemImageConverter.cs
- Model3D.cs
- GiveFeedbackEvent.cs
- MetadataCache.cs
- SimpleLine.cs
- PropertyValue.cs
- Freezable.cs
- SpeechRecognizer.cs
- AppliesToBehaviorDecisionTable.cs
- SrgsGrammarCompiler.cs
- FacetEnabledSchemaElement.cs
- Selection.cs
- DateTimeHelper.cs
- NullableBoolConverter.cs
- Int32Converter.cs
- ListControl.cs
- EpmSourcePathSegment.cs
- TextSelection.cs
- WebEncodingValidatorAttribute.cs
- AssertFilter.cs
- ServiceDocument.cs
- OracleInternalConnection.cs
- NumericUpDownAcceleration.cs
- SerializationFieldInfo.cs
- LicenseContext.cs
- MetadataCacheItem.cs
- MultiByteCodec.cs
- Camera.cs
- SqlNotificationEventArgs.cs
- HwndSourceParameters.cs
- AuthenticatingEventArgs.cs
- SurrogateChar.cs
- Message.cs
- IPHostEntry.cs
- TransformedBitmap.cs
- XmlSchemaSimpleTypeRestriction.cs
- ColorContext.cs