Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Framework / System / Windows / Annotations / Storage / AnnotationStore.cs / 1 / AnnotationStore.cs
//------------------------------------------------------------------------------ // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // The AnnotationStore abstract class provides the basic API for // storing and retrieving annotations. // Spec: http://team/sites/ag/Specifications/CAF%20Storage%20Spec.doc // // History: // 10/04/2002: rruiz: Added header comment to AnnotationStore.cs. // 06/01/2003: LGolding: Ported to WCP tree. // 07/17/2003: rruiz: Changed from interface to abstract class; updated API // as per spec. // //----------------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.Xml; using System.Windows.Annotations; using System.Windows.Markup; namespace System.Windows.Annotations.Storage { ////// The AnnotationStore abstract class provides the basic API for /// storing and retrieving annotations. /// public abstract class AnnotationStore : IDisposable { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors ////// Creates an instance of an annotation store. /// protected AnnotationStore() { } ////// Guarantees that Dispose() will eventually be called on this /// instance. /// ~AnnotationStore() { Dispose(false); } #endregion Constructors //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- #region Public Methods ////// Add a new annotation to this store. The new annotation's Id /// is set to a new value. /// /// the annotation to be added to the store ///newAnnotation is null ///newAnnotation already exists in this store, as determined by its Id ///if object has been disposed public abstract void AddAnnotation(Annotation newAnnotation); ////// Delete the specified annotation. /// /// the Id of the annotation to be deleted ///the annotation that was deleted ///if object has been disposed public abstract Annotation DeleteAnnotation(Guid annotationId); ////// Queries the AnnotationStore for annotations that have an anchor /// that contains a locator that begins with the locator parts /// in anchorLocator. /// /// the locator we are looking for ////// A list of annotations that have locators in their anchors /// starting with the same locator parts list as of the input locator /// Can return an empty list, but never null. /// ///if object has been disposed public abstract IListGetAnnotations(ContentLocator anchorLocator); /// /// Returns a list of all annotations in the store /// ///if object has been disposed ///annotations list. Can return an empty list, but never null. public abstract IListGetAnnotations(); /// /// Finds annotation by Id /// /// ///if object has been disposed ///The annotation. Null if the annotation does not exists public abstract Annotation GetAnnotation(Guid annotationId); ////// Causes any buffered data to be written to the underlying storage /// mechanism. Gets called after each operation if /// AutoFlush is set to true. /// ////// Applications that have an explicit save model may choose to call /// this method directly when appropriate. Applications that have an /// implied save model, see AutoFlush. /// ///if object has been disposed ///public abstract void Flush(); /// /// Disposes of any managed and unmanaged resources used by this /// store. /// public void Dispose() { Dispose(true); GC.SuppressFinalize(this); } #endregion Public Methods //------------------------------------------------------ // // Public Operators // //------------------------------------------------------ //----------------------------------------------------- // // Public Properties // //------------------------------------------------------ #region Public Properties ////// When set to true an implementation should call Flush() /// as a side-effect after each operation. /// ////// true if the implementation is set to call Flush() after /// each operation; false otherwise /// public abstract bool AutoFlush { get; set; } #endregion Public Properties //----------------------------------------------------- // // Public Events // //----------------------------------------------------- #region Public Events ////// Event fired when annotations are added or deleted from the /// AnnotationStore. /// public event StoreContentChangedEventHandler StoreContentChanged; ////// Event fired when an author on any Annotation in this /// AnnotationStore changes. This event is useful if you are /// interested in listening to all annotations from this store /// without registering on each individually. /// public event AnnotationAuthorChangedEventHandler AuthorChanged; ////// Event fired when an anchor on any Annotation in this /// AnnotationStore changes. This event is useful if you are /// interested in listening to all annotations from this store /// without registering on each individually. /// public event AnnotationResourceChangedEventHandler AnchorChanged; ////// Event fired when a cargo on any Annotation in this /// AnnotationStore changes. This event is useful if you are /// interested in listening to all annotations from this store /// without registering on each individually. /// public event AnnotationResourceChangedEventHandler CargoChanged; #endregion Public Events //----------------------------------------------------- // // Protected Methods // //------------------------------------------------------ #region Protected Methods ////// Disposes of the resources (other than memory) used by the store. /// /// true to release both managed and unmanaged /// resources; false to release only unmanaged resources protected virtual void Dispose(bool disposing) { lock (SyncRoot) { if (!_disposed) { if (disposing) { // Do nothing here - subclasses will override this // method and dispose of things as appropriate } _disposed = true; } } } ////// Should be called when any annotation's author changes. /// This will fire the AuthorChanged event and cause a flush /// if AutoFlush is true. /// /// the args for the event protected virtual void OnAuthorChanged(AnnotationAuthorChangedEventArgs args) { AnnotationAuthorChangedEventHandler authorChanged = null; // Ignore null authors added to an annotation if (args.Author == null) return; lock (SyncRoot) { authorChanged = AuthorChanged; } if (AutoFlush) { Flush(); } if (authorChanged != null) { authorChanged(this, args); } } ////// Should be called when any annotation's anchor changes. /// This will fire the AnchorChanged event and cause a flush /// if AutoFlush is true. /// /// the args for the event protected virtual void OnAnchorChanged(AnnotationResourceChangedEventArgs args) { AnnotationResourceChangedEventHandler anchorChanged = null; // Ignore null resources added to an annotation if (args.Resource == null) return; lock (SyncRoot) { anchorChanged = AnchorChanged; } if (AutoFlush) { Flush(); } if (anchorChanged != null) { anchorChanged(this, args); } } ////// Should be called when any annotation's cargo changes. /// This will fire the CargoChanged event and cause a flush /// if AutoFlush is true. /// /// the args for the event protected virtual void OnCargoChanged(AnnotationResourceChangedEventArgs args) { AnnotationResourceChangedEventHandler cargoChanged = null; // Ignore null resources added to an annotation if (args.Resource == null) return; lock (SyncRoot) { cargoChanged = CargoChanged; } if (AutoFlush) { Flush(); } if (cargoChanged != null) { cargoChanged(this, args); } } ////// Should be called after every operation in order to trigger /// events and to perform an automatic flush if AutoFlush is true. /// /// arguments for the event to fire protected virtual void OnStoreContentChanged(StoreContentChangedEventArgs e) { StoreContentChangedEventHandler storeContentChanged = null; lock (SyncRoot) { storeContentChanged = StoreContentChanged; } if (AutoFlush) { Flush(); } if (storeContentChanged != null) { storeContentChanged(this, e); } } #endregion Protected Methods //----------------------------------------------------- // // Protected Properties // //------------------------------------------------------ #region Protected Properties ////// This object should be used for synchronization /// by all AnnotationStore implementations /// ///the lock object protected object SyncRoot { get { return lockObject; } } ////// Is this store disposed /// ///protected bool IsDisposed { get { return _disposed; } } #endregion Protected Properties //------------------------------------------------------ // // Private Fields // //----------------------------------------------------- #region Private Fields // Tracks the state of this store - closed or not private bool _disposed = false; /// Private object used for synchronization private Object lockObject = new Object(); #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ // // // Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // The AnnotationStore abstract class provides the basic API for // storing and retrieving annotations. // Spec: http://team/sites/ag/Specifications/CAF%20Storage%20Spec.doc // // History: // 10/04/2002: rruiz: Added header comment to AnnotationStore.cs. // 06/01/2003: LGolding: Ported to WCP tree. // 07/17/2003: rruiz: Changed from interface to abstract class; updated API // as per spec. // //----------------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.Xml; using System.Windows.Annotations; using System.Windows.Markup; namespace System.Windows.Annotations.Storage { ////// The AnnotationStore abstract class provides the basic API for /// storing and retrieving annotations. /// public abstract class AnnotationStore : IDisposable { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors ////// Creates an instance of an annotation store. /// protected AnnotationStore() { } ////// Guarantees that Dispose() will eventually be called on this /// instance. /// ~AnnotationStore() { Dispose(false); } #endregion Constructors //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- #region Public Methods ////// Add a new annotation to this store. The new annotation's Id /// is set to a new value. /// /// the annotation to be added to the store ///newAnnotation is null ///newAnnotation already exists in this store, as determined by its Id ///if object has been disposed public abstract void AddAnnotation(Annotation newAnnotation); ////// Delete the specified annotation. /// /// the Id of the annotation to be deleted ///the annotation that was deleted ///if object has been disposed public abstract Annotation DeleteAnnotation(Guid annotationId); ////// Queries the AnnotationStore for annotations that have an anchor /// that contains a locator that begins with the locator parts /// in anchorLocator. /// /// the locator we are looking for ////// A list of annotations that have locators in their anchors /// starting with the same locator parts list as of the input locator /// Can return an empty list, but never null. /// ///if object has been disposed public abstract IListGetAnnotations(ContentLocator anchorLocator); /// /// Returns a list of all annotations in the store /// ///if object has been disposed ///annotations list. Can return an empty list, but never null. public abstract IListGetAnnotations(); /// /// Finds annotation by Id /// /// ///if object has been disposed ///The annotation. Null if the annotation does not exists public abstract Annotation GetAnnotation(Guid annotationId); ////// Causes any buffered data to be written to the underlying storage /// mechanism. Gets called after each operation if /// AutoFlush is set to true. /// ////// Applications that have an explicit save model may choose to call /// this method directly when appropriate. Applications that have an /// implied save model, see AutoFlush. /// ///if object has been disposed ///public abstract void Flush(); /// /// Disposes of any managed and unmanaged resources used by this /// store. /// public void Dispose() { Dispose(true); GC.SuppressFinalize(this); } #endregion Public Methods //------------------------------------------------------ // // Public Operators // //------------------------------------------------------ //----------------------------------------------------- // // Public Properties // //------------------------------------------------------ #region Public Properties ////// When set to true an implementation should call Flush() /// as a side-effect after each operation. /// ////// true if the implementation is set to call Flush() after /// each operation; false otherwise /// public abstract bool AutoFlush { get; set; } #endregion Public Properties //----------------------------------------------------- // // Public Events // //----------------------------------------------------- #region Public Events ////// Event fired when annotations are added or deleted from the /// AnnotationStore. /// public event StoreContentChangedEventHandler StoreContentChanged; ////// Event fired when an author on any Annotation in this /// AnnotationStore changes. This event is useful if you are /// interested in listening to all annotations from this store /// without registering on each individually. /// public event AnnotationAuthorChangedEventHandler AuthorChanged; ////// Event fired when an anchor on any Annotation in this /// AnnotationStore changes. This event is useful if you are /// interested in listening to all annotations from this store /// without registering on each individually. /// public event AnnotationResourceChangedEventHandler AnchorChanged; ////// Event fired when a cargo on any Annotation in this /// AnnotationStore changes. This event is useful if you are /// interested in listening to all annotations from this store /// without registering on each individually. /// public event AnnotationResourceChangedEventHandler CargoChanged; #endregion Public Events //----------------------------------------------------- // // Protected Methods // //------------------------------------------------------ #region Protected Methods ////// Disposes of the resources (other than memory) used by the store. /// /// true to release both managed and unmanaged /// resources; false to release only unmanaged resources protected virtual void Dispose(bool disposing) { lock (SyncRoot) { if (!_disposed) { if (disposing) { // Do nothing here - subclasses will override this // method and dispose of things as appropriate } _disposed = true; } } } ////// Should be called when any annotation's author changes. /// This will fire the AuthorChanged event and cause a flush /// if AutoFlush is true. /// /// the args for the event protected virtual void OnAuthorChanged(AnnotationAuthorChangedEventArgs args) { AnnotationAuthorChangedEventHandler authorChanged = null; // Ignore null authors added to an annotation if (args.Author == null) return; lock (SyncRoot) { authorChanged = AuthorChanged; } if (AutoFlush) { Flush(); } if (authorChanged != null) { authorChanged(this, args); } } ////// Should be called when any annotation's anchor changes. /// This will fire the AnchorChanged event and cause a flush /// if AutoFlush is true. /// /// the args for the event protected virtual void OnAnchorChanged(AnnotationResourceChangedEventArgs args) { AnnotationResourceChangedEventHandler anchorChanged = null; // Ignore null resources added to an annotation if (args.Resource == null) return; lock (SyncRoot) { anchorChanged = AnchorChanged; } if (AutoFlush) { Flush(); } if (anchorChanged != null) { anchorChanged(this, args); } } ////// Should be called when any annotation's cargo changes. /// This will fire the CargoChanged event and cause a flush /// if AutoFlush is true. /// /// the args for the event protected virtual void OnCargoChanged(AnnotationResourceChangedEventArgs args) { AnnotationResourceChangedEventHandler cargoChanged = null; // Ignore null resources added to an annotation if (args.Resource == null) return; lock (SyncRoot) { cargoChanged = CargoChanged; } if (AutoFlush) { Flush(); } if (cargoChanged != null) { cargoChanged(this, args); } } ////// Should be called after every operation in order to trigger /// events and to perform an automatic flush if AutoFlush is true. /// /// arguments for the event to fire protected virtual void OnStoreContentChanged(StoreContentChangedEventArgs e) { StoreContentChangedEventHandler storeContentChanged = null; lock (SyncRoot) { storeContentChanged = StoreContentChanged; } if (AutoFlush) { Flush(); } if (storeContentChanged != null) { storeContentChanged(this, e); } } #endregion Protected Methods //----------------------------------------------------- // // Protected Properties // //------------------------------------------------------ #region Protected Properties ////// This object should be used for synchronization /// by all AnnotationStore implementations /// ///the lock object protected object SyncRoot { get { return lockObject; } } ////// Is this store disposed /// ///protected bool IsDisposed { get { return _disposed; } } #endregion Protected Properties //------------------------------------------------------ // // Private Fields // //----------------------------------------------------- #region Private Fields // Tracks the state of this store - closed or not private bool _disposed = false; /// Private object used for synchronization private Object lockObject = new Object(); #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
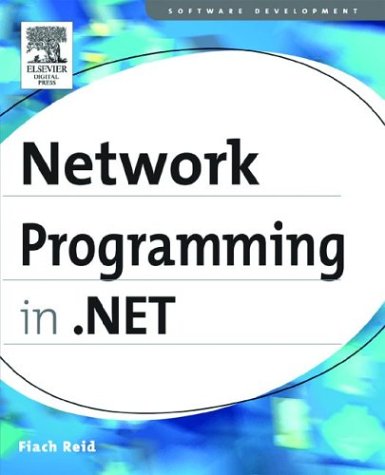
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DbReferenceCollection.cs
- OrderPreservingSpoolingTask.cs
- coordinatorscratchpad.cs
- Vector3dCollection.cs
- SystemColorTracker.cs
- PathSegment.cs
- LinqMaximalSubtreeNominator.cs
- ActiveXContainer.cs
- DiscoveryClientBindingElement.cs
- InvalidDataException.cs
- ErrorHandler.cs
- TextBoxLine.cs
- UnaryExpression.cs
- MenuTracker.cs
- GrammarBuilderWildcard.cs
- AutomationEventArgs.cs
- ICollection.cs
- RawUIStateInputReport.cs
- UInt64Converter.cs
- Floater.cs
- ListBox.cs
- querybuilder.cs
- HyperLinkDataBindingHandler.cs
- DiagnosticTraceSource.cs
- codemethodreferenceexpression.cs
- AdapterDictionary.cs
- GridViewCommandEventArgs.cs
- userdatakeys.cs
- ObjectAnimationUsingKeyFrames.cs
- QfeChecker.cs
- Selection.cs
- CodeExpressionStatement.cs
- DataListCommandEventArgs.cs
- RSAOAEPKeyExchangeDeformatter.cs
- KnownTypesHelper.cs
- ApplicationDirectoryMembershipCondition.cs
- HttpHandlerAction.cs
- PriorityItem.cs
- UnsafeNativeMethods.cs
- CodeNamespaceImportCollection.cs
- WorkflowShape.cs
- PatternMatchRules.cs
- SharedPersonalizationStateInfo.cs
- AccessDataSourceView.cs
- CodeIndexerExpression.cs
- SQLGuidStorage.cs
- ComponentEditorForm.cs
- SoapIncludeAttribute.cs
- PublisherIdentityPermission.cs
- FlowDocumentReaderAutomationPeer.cs
- Operand.cs
- mediaclock.cs
- TransformedBitmap.cs
- ConfigXmlElement.cs
- CommandEventArgs.cs
- InputBuffer.cs
- Vector3DCollectionValueSerializer.cs
- ByteKeyFrameCollection.cs
- StrokeCollection.cs
- OrderByBuilder.cs
- ActionMessageFilterTable.cs
- KeyValueSerializer.cs
- WebEventCodes.cs
- clipboard.cs
- GetWinFXPath.cs
- FeatureSupport.cs
- _CookieModule.cs
- StructureChangedEventArgs.cs
- Transform3DCollection.cs
- CopyCodeAction.cs
- BigInt.cs
- ExpressionParser.cs
- ResourceSet.cs
- QualifiedCellIdBoolean.cs
- PersonalizationAdministration.cs
- XmlnsCompatibleWithAttribute.cs
- CapabilitiesPattern.cs
- InternalControlCollection.cs
- TemplateBuilder.cs
- TabItem.cs
- Environment.cs
- ApplyImportsAction.cs
- DrawingAttributeSerializer.cs
- ArraySortHelper.cs
- Console.cs
- ActiveXMessageFormatter.cs
- _TLSstream.cs
- ProviderConnectionPointCollection.cs
- NativeMethods.cs
- sqlinternaltransaction.cs
- DesignerForm.cs
- OperationInfoBase.cs
- StreamGeometry.cs
- ObjectReferenceStack.cs
- CancellationHandler.cs
- SystemInfo.cs
- KeyEventArgs.cs
- HttpCookieCollection.cs
- BaseCodeDomTreeGenerator.cs
- ThreadExceptionDialog.cs