Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / WinForms / Managed / System / WinForms / ThreadExceptionDialog.cs / 1 / ThreadExceptionDialog.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.Windows.Forms { using System.Threading; using System.Runtime.Remoting; using System.Diagnostics; using System.Diagnostics.CodeAnalysis; using System; using System.Text; using System.ComponentModel; using System.Drawing; using System.Windows.Forms; using System.Reflection; using System.Security; using System.Security.Permissions; using System.Runtime.InteropServices; using System.Globalization; ////// /// /// [ ComVisible(true), ClassInterface(ClassInterfaceType.AutoDispatch), SecurityPermission(SecurityAction.InheritanceDemand, Flags = SecurityPermissionFlag.UnmanagedCode), SecurityPermission(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.UnmanagedCode), UIPermission(SecurityAction.Assert, Window=UIPermissionWindow.AllWindows)] public class ThreadExceptionDialog : Form { private const int MAXWIDTH = 440; private const int MAXHEIGHT = 325; // private const int IDI_ERROR = 32513; private PictureBox pictureBox = new PictureBox(); private Label message = new Label(); private Button continueButton = new Button(); private Button quitButton = new Button(); private Button detailsButton = new Button(); private Button helpButton = new Button(); private TextBox details = new TextBox(); private Bitmap expandImage = null; private Bitmap collapseImage = null; private bool detailsVisible = false; ////// Implements a dialog box that is displayed when an unhandled exception occurs in /// a thread. /// ////// /// public ThreadExceptionDialog(Exception t) { string messageRes; string messageText; Button[] buttons; bool detailAnchor = false; WarningException w = t as WarningException; if (w != null) { messageRes = SR.ExDlgWarningText; messageText = w.Message; if (w.HelpUrl == null) { buttons = new Button[] {continueButton}; } else { buttons = new Button[] {continueButton, helpButton}; } } else { messageText = t.Message; detailAnchor = true; if (Application.AllowQuit) { if (t is SecurityException) { messageRes = "ExDlgSecurityErrorText"; } else { messageRes = "ExDlgErrorText"; } buttons = new Button[] {detailsButton, continueButton, quitButton}; } else { if (t is SecurityException) { messageRes = "ExDlgSecurityContinueErrorText"; } else { messageRes = "ExDlgContinueErrorText"; } buttons = new Button[] {detailsButton, continueButton}; } } if (messageText.Length == 0) { messageText = t.GetType().Name; } if (t is SecurityException) { messageText = SR.GetString(messageRes, t.GetType().Name, Trim(messageText)); } else { messageText = SR.GetString(messageRes, Trim(messageText)); } StringBuilder detailsTextBuilder = new StringBuilder(); string newline = "\r\n"; string separator = SR.GetString(SR.ExDlgMsgSeperator); string sectionseparator = SR.GetString(SR.ExDlgMsgSectionSeperator); if (Application.CustomThreadExceptionHandlerAttached) { detailsTextBuilder.Append(SR.GetString(SR.ExDlgMsgHeaderNonSwitchable)); } else { detailsTextBuilder.Append(SR.GetString(SR.ExDlgMsgHeaderSwitchable)); } detailsTextBuilder.Append(string.Format(CultureInfo.CurrentCulture, sectionseparator, SR.GetString(SR.ExDlgMsgExceptionSection))); detailsTextBuilder.Append(t.ToString()); detailsTextBuilder.Append(newline); detailsTextBuilder.Append(newline); detailsTextBuilder.Append(string.Format(CultureInfo.CurrentCulture, sectionseparator, SR.GetString(SR.ExDlgMsgLoadedAssembliesSection))); new FileIOPermission(PermissionState.Unrestricted).Assert(); try { foreach (Assembly asm in AppDomain.CurrentDomain.GetAssemblies()) { AssemblyName name = asm.GetName(); string fileVer = SR.GetString(SR.NotAvailable); try { // bug 113573 -- if there's a path with an escaped value in it // like c:\temp\foo%2fbar, the AssemblyName call will unescape it to // c:\temp\foo\bar, which is wrong, and this will fail. It doesn't look like the // assembly name class handles this properly -- even the "CodeBase" property is un-escaped // so we can't circumvent this. // if (name.EscapedCodeBase != null && name.EscapedCodeBase.Length > 0) { Uri codeBase = new Uri(name.EscapedCodeBase); if (codeBase.Scheme == "file") { fileVer = FileVersionInfo.GetVersionInfo(NativeMethods.GetLocalPath(name.EscapedCodeBase)).FileVersion; } } } catch(System.IO.FileNotFoundException){ } detailsTextBuilder.Append(SR.GetString(SR.ExDlgMsgLoadedAssembliesEntry, name.Name, name.Version, fileVer, name.EscapedCodeBase)); detailsTextBuilder.Append(separator); } } finally { CodeAccessPermission.RevertAssert(); } detailsTextBuilder.Append(string.Format(CultureInfo.CurrentCulture, sectionseparator, SR.GetString(SR.ExDlgMsgJITDebuggingSection))); if (Application.CustomThreadExceptionHandlerAttached) { detailsTextBuilder.Append(SR.GetString(SR.ExDlgMsgFooterNonSwitchable)); } else { detailsTextBuilder.Append(SR.GetString(SR.ExDlgMsgFooterSwitchable)); } detailsTextBuilder.Append(newline); detailsTextBuilder.Append(newline); string detailsText = detailsTextBuilder.ToString(); Graphics g = message.CreateGraphicsInternal(); Size textSize = Size.Ceiling(g.MeasureString(messageText, Font, MAXWIDTH - 84)); textSize.Height += 4; g.Dispose(); if (textSize.Width < 180) textSize.Width = 180; if (textSize.Height > MAXHEIGHT) textSize.Height = MAXHEIGHT; int width = textSize.Width + 84; int buttonTop = Math.Max(textSize.Height, 40) + 26; // SECREVIEW : We must get a hold of the parent to get at it's text // : to make this dialog look like the parent. // IntSecurity.GetParent.Assert(); try { Form activeForm = Form.ActiveForm; if (activeForm == null || activeForm.Text.Length == 0) { Text = SR.GetString(SR.ExDlgCaption); } else { Text = SR.GetString(SR.ExDlgCaption2, activeForm.Text); } } finally { CodeAccessPermission.RevertAssert(); } AcceptButton = continueButton; CancelButton = continueButton; FormBorderStyle = FormBorderStyle.FixedDialog; MaximizeBox = false; MinimizeBox = false; StartPosition = FormStartPosition.CenterScreen; Icon = null; ClientSize = new Size(width, buttonTop + 31); TopMost = true; pictureBox.Location = new Point(0, 0); pictureBox.Size = new Size(64, 64); pictureBox.SizeMode = PictureBoxSizeMode.CenterImage; if (t is SecurityException) { pictureBox.Image = SystemIcons.Information.ToBitmap(); } else { pictureBox.Image = SystemIcons.Error.ToBitmap(); } Controls.Add(pictureBox); message.SetBounds(64, 8 + (40 - Math.Min(textSize.Height, 40)) / 2, textSize.Width, textSize.Height); message.Text = messageText; Controls.Add(message); continueButton.Text = SR.GetString(SR.ExDlgContinue); continueButton.FlatStyle = FlatStyle.Standard; continueButton.DialogResult = DialogResult.Cancel; quitButton.Text = SR.GetString(SR.ExDlgQuit); quitButton.FlatStyle = FlatStyle.Standard; quitButton.DialogResult = DialogResult.Abort; helpButton.Text = SR.GetString(SR.ExDlgHelp); helpButton.FlatStyle = FlatStyle.Standard; helpButton.DialogResult = DialogResult.Yes; detailsButton.Text = SR.GetString(SR.ExDlgShowDetails); detailsButton.FlatStyle = FlatStyle.Standard; detailsButton.Click += new EventHandler(DetailsClick); Button b = null; int startIndex = 0; if (detailAnchor) { b = detailsButton; expandImage = new Bitmap(this.GetType(), "down.bmp"); expandImage.MakeTransparent(); collapseImage = new Bitmap(this.GetType(), "up.bmp"); collapseImage.MakeTransparent(); b.SetBounds( 8, buttonTop, 100, 23 ); b.Image = expandImage; b.ImageAlign = ContentAlignment.MiddleLeft; Controls.Add(b); startIndex = 1; } int buttonLeft = (width - 8 - ((buttons.Length - startIndex) * 105 - 5)); for (int i = startIndex; i < buttons.Length; i++) { b = buttons[i]; b.SetBounds(buttonLeft, buttonTop, 100, 23); Controls.Add(b); buttonLeft += 105; } details.Text = detailsText; details.ScrollBars = ScrollBars.Both; details.Multiline = true; details.ReadOnly = true; details.WordWrap = false; details.TabStop = false; details.AcceptsReturn = false; details.SetBounds(8, buttonTop + 31, width - 16,154); Controls.Add(details); } ////// Initializes a new instance of the ///class. /// /// /// /// [Browsable(false), EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden)] public override bool AutoSize { [SuppressMessage("Microsoft.Security", "CA2123:OverrideLinkDemandsShouldBeIdenticalToBase")] get { return base.AutoSize; } [SuppressMessage("Microsoft.Security", "CA2123:OverrideLinkDemandsShouldBeIdenticalToBase")] set { base.AutoSize = value; } } ////// Hide the property /// ///[Browsable(false), EditorBrowsable(EditorBrowsableState.Never)] new public event EventHandler AutoSizeChanged { add { base.AutoSizeChanged += value; } remove { base.AutoSizeChanged -= value; } } /// /// /// Called when the details button is clicked. /// private void DetailsClick(object sender, EventArgs eventargs) { int delta = details.Height + 8; if (detailsVisible) delta = -delta; Height = Height + delta; detailsVisible = !detailsVisible; detailsButton.Image = detailsVisible ? collapseImage : expandImage; } private static string Trim(string s) { if (s == null) return s; int i = s.Length; while (i > 0 && s[i - 1] == '.') i--; return s.Substring(0, i); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
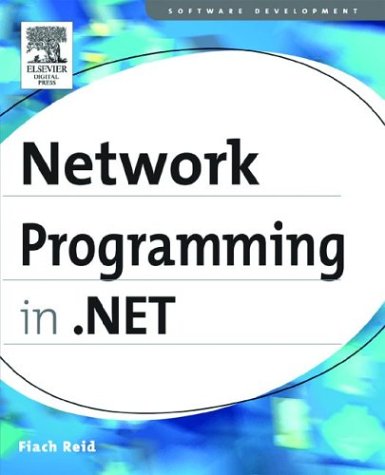
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- RankException.cs
- WebPartZoneCollection.cs
- Stackframe.cs
- ControlEvent.cs
- DropDownButton.cs
- Activity.cs
- ZoneLinkButton.cs
- LogExtent.cs
- RootNamespaceAttribute.cs
- RegexNode.cs
- WebPartConnectVerb.cs
- SqlProcedureAttribute.cs
- PeekCompletedEventArgs.cs
- ComponentRenameEvent.cs
- GroupStyle.cs
- CustomErrorsSectionWrapper.cs
- HtmlElementErrorEventArgs.cs
- SqlUnionizer.cs
- DynamicILGenerator.cs
- HandledMouseEvent.cs
- FreezableCollection.cs
- SaveFileDialog.cs
- DbProviderSpecificTypePropertyAttribute.cs
- TemplateBindingExpressionConverter.cs
- DataColumnChangeEvent.cs
- Cursors.cs
- BigIntegerStorage.cs
- NativeActivityFaultContext.cs
- WinFormsSecurity.cs
- MenuItemStyle.cs
- CodeEventReferenceExpression.cs
- ApplicationServiceManager.cs
- GridEntryCollection.cs
- DataGridSortCommandEventArgs.cs
- DocumentXPathNavigator.cs
- OracleBinary.cs
- ListViewInsertEventArgs.cs
- AuthenticationService.cs
- ZipPackagePart.cs
- ProfileParameter.cs
- TrustLevel.cs
- Comparer.cs
- HttpRawResponse.cs
- FixedDocumentPaginator.cs
- EntityTypeEmitter.cs
- TableLayoutRowStyleCollection.cs
- ItemList.cs
- AutomationProperties.cs
- SqlProvider.cs
- Hyperlink.cs
- StandardCommands.cs
- SaveFileDialog.cs
- AuthenticationService.cs
- HtmlTitle.cs
- TableCellAutomationPeer.cs
- SecuritySessionClientSettings.cs
- TimersDescriptionAttribute.cs
- ParseElement.cs
- InitializationEventAttribute.cs
- ManagementClass.cs
- safex509handles.cs
- StructuralCache.cs
- JavascriptCallbackBehaviorAttribute.cs
- XPathExpr.cs
- Quad.cs
- XmlNavigatorFilter.cs
- SafeRightsManagementHandle.cs
- UnmanagedBitmapWrapper.cs
- PrintDialog.cs
- DataSourceControl.cs
- SqlCacheDependencyDatabaseCollection.cs
- DebugHandleTracker.cs
- IRCollection.cs
- PasswordRecoveryDesigner.cs
- BulletedListDesigner.cs
- DataComponentNameHandler.cs
- messageonlyhwndwrapper.cs
- ServiceNotStartedException.cs
- JsonFormatGeneratorStatics.cs
- CommentAction.cs
- RoleServiceManager.cs
- DtrList.cs
- ChooseAction.cs
- XmlSchemaObjectTable.cs
- WebPartEditorCancelVerb.cs
- EditorPartCollection.cs
- WebDisplayNameAttribute.cs
- ProjectionPlan.cs
- DataGrid.cs
- basenumberconverter.cs
- ContentPlaceHolder.cs
- ResourcesBuildProvider.cs
- TrustLevelCollection.cs
- Constants.cs
- BindingContext.cs
- RegionIterator.cs
- contentDescriptor.cs
- ToolStripMenuItemDesigner.cs
- X509CertificateCollection.cs
- RawUIStateInputReport.cs