Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / WinForms / Managed / System / WinForms / TextBoxRenderer.cs / 1 / TextBoxRenderer.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System; using System.Drawing; using System.Diagnostics.CodeAnalysis; using System.Windows.Forms.Internal; using System.Windows.Forms.VisualStyles; using Microsoft.Win32; ////// /// public sealed class TextBoxRenderer { //Make this per-thread, so that different threads can safely use these methods. [ThreadStatic] private static VisualStyleRenderer visualStyleRenderer = null; private static readonly VisualStyleElement TextBoxElement = VisualStyleElement.TextBox.TextEdit.Normal; //cannot instantiate private TextBoxRenderer() { } ////// This is a rendering class for the TextBox control. /// ////// /// public static bool IsSupported { get { return VisualStyleRenderer.IsSupported; // no downlevel support } } private static void DrawBackground(Graphics g, Rectangle bounds, TextBoxState state) { visualStyleRenderer.DrawBackground(g, bounds); if (state != TextBoxState.Disabled) { Color windowColor = visualStyleRenderer.GetColor(ColorProperty.FillColor); if (windowColor != SystemColors.Window) { Rectangle fillRect = visualStyleRenderer.GetBackgroundContentRectangle(g, bounds); //then we need to re-fill the background. g.FillRectangle(new SolidBrush(SystemColors.Window), fillRect); } } } ////// Returns true if this class is supported for the current OS and user/application settings, /// otherwise returns false. /// ////// /// [ SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters") // Using Graphics instead of IDeviceContext intentionally ] public static void DrawTextBox(Graphics g, Rectangle bounds, TextBoxState state) { InitializeRenderer((int)state); DrawBackground(g, bounds, state); } ////// Renders a TextBox control. /// ////// /// public static void DrawTextBox(Graphics g, Rectangle bounds, string textBoxText, Font font, TextBoxState state) { DrawTextBox(g, bounds, textBoxText, font, TextFormatFlags.TextBoxControl, state); } ////// Renders a TextBox control. /// ////// /// public static void DrawTextBox(Graphics g, Rectangle bounds, string textBoxText, Font font, Rectangle textBounds, TextBoxState state) { DrawTextBox(g, bounds, textBoxText, font, textBounds, TextFormatFlags.TextBoxControl, state); } ////// Renders a TextBox control. /// ////// /// public static void DrawTextBox(Graphics g, Rectangle bounds, string textBoxText, Font font, TextFormatFlags flags, TextBoxState state) { InitializeRenderer((int)state); Rectangle textBounds = visualStyleRenderer.GetBackgroundContentRectangle(g, bounds); textBounds.Inflate(-2, -2); DrawTextBox(g, bounds, textBoxText, font, textBounds, flags, state); } ////// Renders a TextBox control. /// ////// /// [ SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters") // Using Graphics instead of IDeviceContext intentionally ] public static void DrawTextBox(Graphics g, Rectangle bounds, string textBoxText, Font font, Rectangle textBounds, TextFormatFlags flags, TextBoxState state) { InitializeRenderer((int)state); DrawBackground(g, bounds, state); Color textColor = visualStyleRenderer.GetColor(ColorProperty.TextColor); TextRenderer.DrawText(g, textBoxText, font, textBounds, textColor, flags); } private static void InitializeRenderer(int state) { if (visualStyleRenderer == null) { visualStyleRenderer = new VisualStyleRenderer(TextBoxElement.ClassName, TextBoxElement.Part, state); } else { visualStyleRenderer.SetParameters(TextBoxElement.ClassName, TextBoxElement.Part, state); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ ///// Renders a TextBox control. /// ///// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System; using System.Drawing; using System.Diagnostics.CodeAnalysis; using System.Windows.Forms.Internal; using System.Windows.Forms.VisualStyles; using Microsoft.Win32; ////// /// public sealed class TextBoxRenderer { //Make this per-thread, so that different threads can safely use these methods. [ThreadStatic] private static VisualStyleRenderer visualStyleRenderer = null; private static readonly VisualStyleElement TextBoxElement = VisualStyleElement.TextBox.TextEdit.Normal; //cannot instantiate private TextBoxRenderer() { } ////// This is a rendering class for the TextBox control. /// ////// /// public static bool IsSupported { get { return VisualStyleRenderer.IsSupported; // no downlevel support } } private static void DrawBackground(Graphics g, Rectangle bounds, TextBoxState state) { visualStyleRenderer.DrawBackground(g, bounds); if (state != TextBoxState.Disabled) { Color windowColor = visualStyleRenderer.GetColor(ColorProperty.FillColor); if (windowColor != SystemColors.Window) { Rectangle fillRect = visualStyleRenderer.GetBackgroundContentRectangle(g, bounds); //then we need to re-fill the background. g.FillRectangle(new SolidBrush(SystemColors.Window), fillRect); } } } ////// Returns true if this class is supported for the current OS and user/application settings, /// otherwise returns false. /// ////// /// [ SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters") // Using Graphics instead of IDeviceContext intentionally ] public static void DrawTextBox(Graphics g, Rectangle bounds, TextBoxState state) { InitializeRenderer((int)state); DrawBackground(g, bounds, state); } ////// Renders a TextBox control. /// ////// /// public static void DrawTextBox(Graphics g, Rectangle bounds, string textBoxText, Font font, TextBoxState state) { DrawTextBox(g, bounds, textBoxText, font, TextFormatFlags.TextBoxControl, state); } ////// Renders a TextBox control. /// ////// /// public static void DrawTextBox(Graphics g, Rectangle bounds, string textBoxText, Font font, Rectangle textBounds, TextBoxState state) { DrawTextBox(g, bounds, textBoxText, font, textBounds, TextFormatFlags.TextBoxControl, state); } ////// Renders a TextBox control. /// ////// /// public static void DrawTextBox(Graphics g, Rectangle bounds, string textBoxText, Font font, TextFormatFlags flags, TextBoxState state) { InitializeRenderer((int)state); Rectangle textBounds = visualStyleRenderer.GetBackgroundContentRectangle(g, bounds); textBounds.Inflate(-2, -2); DrawTextBox(g, bounds, textBoxText, font, textBounds, flags, state); } ////// Renders a TextBox control. /// ////// /// [ SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters") // Using Graphics instead of IDeviceContext intentionally ] public static void DrawTextBox(Graphics g, Rectangle bounds, string textBoxText, Font font, Rectangle textBounds, TextFormatFlags flags, TextBoxState state) { InitializeRenderer((int)state); DrawBackground(g, bounds, state); Color textColor = visualStyleRenderer.GetColor(ColorProperty.TextColor); TextRenderer.DrawText(g, textBoxText, font, textBounds, textColor, flags); } private static void InitializeRenderer(int state) { if (visualStyleRenderer == null) { visualStyleRenderer = new VisualStyleRenderer(TextBoxElement.ClassName, TextBoxElement.Part, state); } else { visualStyleRenderer.SetParameters(TextBoxElement.ClassName, TextBoxElement.Part, state); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007./// Renders a TextBox control. /// ///
Link Menu
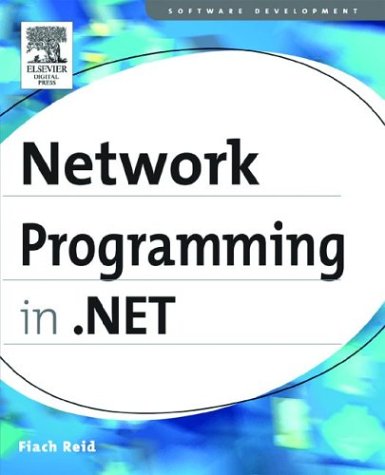
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- PenCursorManager.cs
- SqlDataSourceEnumerator.cs
- ReverseComparer.cs
- DllNotFoundException.cs
- CodeGeneratorOptions.cs
- ErrorStyle.cs
- VersionedStream.cs
- PageCodeDomTreeGenerator.cs
- AutoSizeComboBox.cs
- ThreadStateException.cs
- SuppressIldasmAttribute.cs
- UnsafePeerToPeerMethods.cs
- DataBoundControl.cs
- PopupRoot.cs
- ImplicitInputBrush.cs
- SoapDocumentMethodAttribute.cs
- ListChangedEventArgs.cs
- WebPartEditorOkVerb.cs
- UTF8Encoding.cs
- COM2ExtendedBrowsingHandler.cs
- Relationship.cs
- TemplatePropertyEntry.cs
- ContextMenuStripActionList.cs
- SizeConverter.cs
- DataGridViewLayoutData.cs
- WebEventTraceProvider.cs
- SchemaManager.cs
- WorkflowRuntimeSection.cs
- xsdvalidator.cs
- GeneralTransform3DTo2D.cs
- CreationContext.cs
- StateWorkerRequest.cs
- Point3DAnimationBase.cs
- OleDbDataReader.cs
- ToolStripDropDown.cs
- KeyedQueue.cs
- CryptoProvider.cs
- EditorPart.cs
- XmlWrappingReader.cs
- baseaxisquery.cs
- ConfigUtil.cs
- ListBindingHelper.cs
- CodeEventReferenceExpression.cs
- BStrWrapper.cs
- DataBindingCollection.cs
- EntityTemplateUserControl.cs
- ObjectItemCollectionAssemblyCacheEntry.cs
- XamlSerializerUtil.cs
- NTAccount.cs
- Int32Storage.cs
- COM2IVsPerPropertyBrowsingHandler.cs
- ByteStorage.cs
- CapiHashAlgorithm.cs
- CompositeScriptReference.cs
- SynchronizingStream.cs
- EventSinkHelperWriter.cs
- WindowsAuthenticationModule.cs
- ConnectionPool.cs
- WebPartCatalogCloseVerb.cs
- ActivityInstanceMap.cs
- EntitySqlQueryState.cs
- InvokeSchedule.cs
- XmlSchemaDocumentation.cs
- WebPartHelpVerb.cs
- MsmqOutputChannel.cs
- TitleStyle.cs
- HandlerFactoryCache.cs
- ValidationErrorInfo.cs
- ZipPackagePart.cs
- TypeUtil.cs
- HwndAppCommandInputProvider.cs
- WhitespaceRuleLookup.cs
- ToolStripSeparatorRenderEventArgs.cs
- ListViewPagedDataSource.cs
- MobileContainerDesigner.cs
- CapacityStreamGeometryContext.cs
- RSAOAEPKeyExchangeDeformatter.cs
- XsdCachingReader.cs
- CategoryGridEntry.cs
- UriSectionData.cs
- XslException.cs
- TrimSurroundingWhitespaceAttribute.cs
- AuthenticationManager.cs
- TextTreeRootTextBlock.cs
- URLString.cs
- EffectiveValueEntry.cs
- GeometryGroup.cs
- TryExpression.cs
- BridgeDataReader.cs
- ZipIOLocalFileDataDescriptor.cs
- UniqueConstraint.cs
- ObjectStateEntry.cs
- IPAddress.cs
- ClientSession.cs
- WindowsFormsHost.cs
- ErrorLog.cs
- ItemAutomationPeer.cs
- ComMethodElement.cs
- TextSchema.cs
- SerializerWriterEventHandlers.cs