Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / WF / Activities / Rules / RuleSetCollection.cs / 1305376 / RuleSetCollection.cs
using System; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Globalization; using System.Text; using System.Workflow.ComponentModel; namespace System.Workflow.Activities.Rules { #region class RuleSetCollection public sealed class RuleSetCollection : KeyedCollection, IWorkflowChangeDiff { #region members and constructors private bool _runtimeInitialized; [NonSerialized] private object syncLock = new object(); public RuleSetCollection() { } #endregion #region keyed collection members protected override string GetKeyForItem(RuleSet item) { return item.Name; } protected override void InsertItem(int index, RuleSet item) { if (this._runtimeInitialized) throw new InvalidOperationException(SR.GetString(SR.Error_CanNotChangeAtRuntime)); if (item.Name != null && item.Name.Length >= 0 && this.Contains(item.Name)) { string message = string.Format(CultureInfo.CurrentCulture, Messages.RuleSetExists, item.Name); throw new ArgumentException(message); } base.InsertItem(index, item); } protected override void RemoveItem(int index) { if (this._runtimeInitialized) throw new InvalidOperationException(SR.GetString(SR.Error_CanNotChangeAtRuntime)); base.RemoveItem(index); } protected override void SetItem(int index, RuleSet item) { if (this._runtimeInitialized) throw new InvalidOperationException(SR.GetString(SR.Error_CanNotChangeAtRuntime)); base.SetItem(index, item); } new public void Add(RuleSet item) { if (this._runtimeInitialized) throw new InvalidOperationException(SR.GetString(SR.Error_CanNotChangeAtRuntime)); if (null == item) { throw new ArgumentNullException("item"); } if (null == item.Name) { string message = string.Format(CultureInfo.CurrentCulture, Messages.InvalidRuleSetName, "item.Name"); throw new ArgumentException(message); } base.Add(item); } #endregion #region runtime initializing internal void OnRuntimeInitialized() { lock (this.syncLock) { if (this._runtimeInitialized) return; foreach (RuleSet ruleSet in this) { ruleSet.OnRuntimeInitialized(); } _runtimeInitialized = true; } } internal bool RuntimeMode { set { this._runtimeInitialized = value; } get { return this._runtimeInitialized; } } internal string GenerateRuleSetName() { string nameBase = Messages.NewRuleSetName; string newName; int i = 1; do { newName = nameBase + i.ToString(CultureInfo.InvariantCulture); i++; } while(this.Contains(newName)); return newName; } #endregion #region IWorkflowChangeDiff Members public IList Diff(object originalDefinition, object changedDefinition) { List listChanges = new List (); RuleSetCollection originalRuleSets = (RuleSetCollection)originalDefinition; RuleSetCollection changedRuleSets = (RuleSetCollection)changedDefinition; if (null != changedRuleSets) { foreach (RuleSet changedRuleSet in changedRuleSets) { if ((originalRuleSets != null) && (originalRuleSets.Contains(changedRuleSet.Name))) { RuleSet originalRuleSet = originalRuleSets[changedRuleSet.Name]; if (!originalRuleSet.Equals(changedRuleSet)) { listChanges.Add(new UpdatedRuleSetAction(originalRuleSet, changedRuleSet)); } } else { listChanges.Add(new AddedRuleSetAction(changedRuleSet)); } } } if (null != originalRuleSets) { foreach (RuleSet originalRuleSet in originalRuleSets) { if ((changedRuleSets == null) || (!changedRuleSets.Contains(originalRuleSet.Name))) { listChanges.Add(new RemovedRuleSetAction(originalRuleSet)); } } } return listChanges; } #endregion } #endregion } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using System; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Globalization; using System.Text; using System.Workflow.ComponentModel; namespace System.Workflow.Activities.Rules { #region class RuleSetCollection public sealed class RuleSetCollection : KeyedCollection , IWorkflowChangeDiff { #region members and constructors private bool _runtimeInitialized; [NonSerialized] private object syncLock = new object(); public RuleSetCollection() { } #endregion #region keyed collection members protected override string GetKeyForItem(RuleSet item) { return item.Name; } protected override void InsertItem(int index, RuleSet item) { if (this._runtimeInitialized) throw new InvalidOperationException(SR.GetString(SR.Error_CanNotChangeAtRuntime)); if (item.Name != null && item.Name.Length >= 0 && this.Contains(item.Name)) { string message = string.Format(CultureInfo.CurrentCulture, Messages.RuleSetExists, item.Name); throw new ArgumentException(message); } base.InsertItem(index, item); } protected override void RemoveItem(int index) { if (this._runtimeInitialized) throw new InvalidOperationException(SR.GetString(SR.Error_CanNotChangeAtRuntime)); base.RemoveItem(index); } protected override void SetItem(int index, RuleSet item) { if (this._runtimeInitialized) throw new InvalidOperationException(SR.GetString(SR.Error_CanNotChangeAtRuntime)); base.SetItem(index, item); } new public void Add(RuleSet item) { if (this._runtimeInitialized) throw new InvalidOperationException(SR.GetString(SR.Error_CanNotChangeAtRuntime)); if (null == item) { throw new ArgumentNullException("item"); } if (null == item.Name) { string message = string.Format(CultureInfo.CurrentCulture, Messages.InvalidRuleSetName, "item.Name"); throw new ArgumentException(message); } base.Add(item); } #endregion #region runtime initializing internal void OnRuntimeInitialized() { lock (this.syncLock) { if (this._runtimeInitialized) return; foreach (RuleSet ruleSet in this) { ruleSet.OnRuntimeInitialized(); } _runtimeInitialized = true; } } internal bool RuntimeMode { set { this._runtimeInitialized = value; } get { return this._runtimeInitialized; } } internal string GenerateRuleSetName() { string nameBase = Messages.NewRuleSetName; string newName; int i = 1; do { newName = nameBase + i.ToString(CultureInfo.InvariantCulture); i++; } while(this.Contains(newName)); return newName; } #endregion #region IWorkflowChangeDiff Members public IList Diff(object originalDefinition, object changedDefinition) { List listChanges = new List (); RuleSetCollection originalRuleSets = (RuleSetCollection)originalDefinition; RuleSetCollection changedRuleSets = (RuleSetCollection)changedDefinition; if (null != changedRuleSets) { foreach (RuleSet changedRuleSet in changedRuleSets) { if ((originalRuleSets != null) && (originalRuleSets.Contains(changedRuleSet.Name))) { RuleSet originalRuleSet = originalRuleSets[changedRuleSet.Name]; if (!originalRuleSet.Equals(changedRuleSet)) { listChanges.Add(new UpdatedRuleSetAction(originalRuleSet, changedRuleSet)); } } else { listChanges.Add(new AddedRuleSetAction(changedRuleSet)); } } } if (null != originalRuleSets) { foreach (RuleSet originalRuleSet in originalRuleSets) { if ((changedRuleSets == null) || (!changedRuleSets.Contains(originalRuleSet.Name))) { listChanges.Add(new RemovedRuleSetAction(originalRuleSet)); } } } return listChanges; } #endregion } #endregion } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
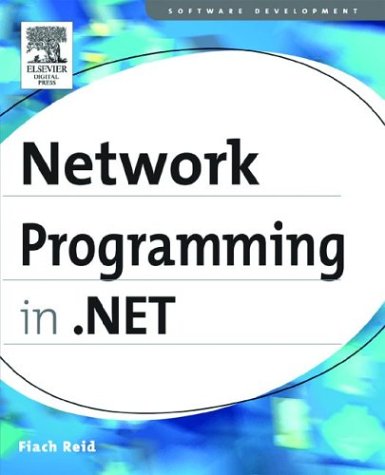
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DBSchemaTable.cs
- Int16AnimationBase.cs
- HiddenField.cs
- UncommonField.cs
- GroupDescription.cs
- ImmutablePropertyDescriptorGridEntry.cs
- ListItemParagraph.cs
- sqlser.cs
- PropertyPathWorker.cs
- Model3DGroup.cs
- StatusBarPanelClickEvent.cs
- ServiceNameCollection.cs
- RowCache.cs
- SynchronizedInputPattern.cs
- MethodCallConverter.cs
- PropertySegmentSerializationProvider.cs
- unsafeIndexingFilterStream.cs
- ActivityCodeGenerator.cs
- RootBrowserWindow.cs
- UInt64Storage.cs
- BufferedGraphics.cs
- RemoteWebConfigurationHostStream.cs
- EmissiveMaterial.cs
- DataControlFieldCollection.cs
- EncoderBestFitFallback.cs
- BamlMapTable.cs
- OutputScopeManager.cs
- Debug.cs
- DataControlHelper.cs
- ArraySegment.cs
- CRYPTPROTECT_PROMPTSTRUCT.cs
- fixedPageContentExtractor.cs
- MetabaseReader.cs
- Currency.cs
- NamespaceDecl.cs
- HelloOperationCD1AsyncResult.cs
- _SafeNetHandles.cs
- TextLineResult.cs
- SelectionRangeConverter.cs
- PageContentAsyncResult.cs
- PointKeyFrameCollection.cs
- PrintPageEvent.cs
- SignedPkcs7.cs
- ToolboxItemCollection.cs
- StringFormat.cs
- StringArrayConverter.cs
- WindowsAuthenticationEventArgs.cs
- ToolTip.cs
- NamedPipeDuplicateContext.cs
- SelfIssuedSamlTokenFactory.cs
- LinkDescriptor.cs
- XmlElementAttributes.cs
- SortedDictionary.cs
- DashStyles.cs
- CalculatedColumn.cs
- DataControlField.cs
- FacetDescription.cs
- DataGridColumnReorderingEventArgs.cs
- MetadataUtilsSmi.cs
- ControlEvent.cs
- HttpDebugHandler.cs
- RemoteWebConfigurationHostServer.cs
- ToolStripPanelCell.cs
- TreeNodeStyleCollection.cs
- IPAddress.cs
- FilterElement.cs
- IProvider.cs
- TreeBuilderXamlTranslator.cs
- TaskHelper.cs
- InternalResources.cs
- RowBinding.cs
- FileEnumerator.cs
- InstanceDataCollection.cs
- BuilderPropertyEntry.cs
- TableSectionStyle.cs
- VScrollBar.cs
- DetailsViewModeEventArgs.cs
- FileSystemEventArgs.cs
- CaseStatement.cs
- Size3DConverter.cs
- TimeSpanStorage.cs
- EventMappingSettingsCollection.cs
- HttpRuntime.cs
- SchemaSetCompiler.cs
- DirectoryRedirect.cs
- ObjectStateManager.cs
- BitmapMetadataEnumerator.cs
- WindowsRegion.cs
- GridViewCommandEventArgs.cs
- EntityDataSourceQueryBuilder.cs
- Update.cs
- Int32CAMarshaler.cs
- PingReply.cs
- Pair.cs
- ZoomComboBox.cs
- HybridDictionary.cs
- LazyTextWriterCreator.cs
- CustomWebEventKey.cs
- ImageBrush.cs
- DataListItemCollection.cs