Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Core / CSharp / System / Windows / Input / Command / SecureUICommand.cs / 1 / SecureUICommand.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: The Command class is used by the developer to define the intent of the User Action // This also serves the purpose of identifying commands or to compare identities of // InputBindings and CommandBindings // // See New spec at : (need link) // // History: // 01/26/2005 : [....] - Created //--------------------------------------------------------------------------- using System; using System.Security; using System.Security.Permissions; using System.Windows; using System.ComponentModel; using System.Collections; using System.Windows.Input; namespace System.Windows.Input { ////// Command /// [TypeConverter("System.Windows.Input.CommandConverter, PresentationFramework, Version=" + Microsoft.Internal.BuildInfo.WCP_VERSION + ", Culture=neutral, PublicKeyToken=" + Microsoft.Internal.BuildInfo.WCP_PUBLIC_KEY_TOKEN + ", Custom=null")] internal class SecureUICommand : RoutedUICommand, ISecureCommand { ////// Critical - should only be write-once in the constructor /// [SecurityCritical] readonly PermissionSet _userInitiated; ////// Creates a new secure command, requiring the specified permissions. Used to delay initialization of Text and InputGestureCollection to time of first use. /// /// PermissionSet to associate with this command /// Name of the Command Property/Field for Serialization /// Type that is registering the property /// Idenfier assigned by the owning type. ////// Critical - assigns to the permission set, a protected resource /// TreatAsSafe - KeyBinding (through InputBinding) will demand this permission before /// binding this command to any key. /// [SecurityCritical, SecurityTreatAsSafe] internal SecureUICommand(PermissionSet userInitiated, string name, Type ownerType, byte commandId) : base(name, ownerType, commandId) { _userInitiated = userInitiated; } ////// Permission required to modify bindings for this /// command, and the permission to assert when /// the command is invoked in a user interactive /// (trusted) fashion. /// ////// Critical - access the permission set, a protected resource /// TreatAsSafe - get only access is safe /// public PermissionSet UserInitiatedPermission { [SecurityCritical, SecurityTreatAsSafe] get { return _userInitiated; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: The Command class is used by the developer to define the intent of the User Action // This also serves the purpose of identifying commands or to compare identities of // InputBindings and CommandBindings // // See New spec at : (need link) // // History: // 01/26/2005 : [....] - Created //--------------------------------------------------------------------------- using System; using System.Security; using System.Security.Permissions; using System.Windows; using System.ComponentModel; using System.Collections; using System.Windows.Input; namespace System.Windows.Input { ////// Command /// [TypeConverter("System.Windows.Input.CommandConverter, PresentationFramework, Version=" + Microsoft.Internal.BuildInfo.WCP_VERSION + ", Culture=neutral, PublicKeyToken=" + Microsoft.Internal.BuildInfo.WCP_PUBLIC_KEY_TOKEN + ", Custom=null")] internal class SecureUICommand : RoutedUICommand, ISecureCommand { ////// Critical - should only be write-once in the constructor /// [SecurityCritical] readonly PermissionSet _userInitiated; ////// Creates a new secure command, requiring the specified permissions. Used to delay initialization of Text and InputGestureCollection to time of first use. /// /// PermissionSet to associate with this command /// Name of the Command Property/Field for Serialization /// Type that is registering the property /// Idenfier assigned by the owning type. ////// Critical - assigns to the permission set, a protected resource /// TreatAsSafe - KeyBinding (through InputBinding) will demand this permission before /// binding this command to any key. /// [SecurityCritical, SecurityTreatAsSafe] internal SecureUICommand(PermissionSet userInitiated, string name, Type ownerType, byte commandId) : base(name, ownerType, commandId) { _userInitiated = userInitiated; } ////// Permission required to modify bindings for this /// command, and the permission to assert when /// the command is invoked in a user interactive /// (trusted) fashion. /// ////// Critical - access the permission set, a protected resource /// TreatAsSafe - get only access is safe /// public PermissionSet UserInitiatedPermission { [SecurityCritical, SecurityTreatAsSafe] get { return _userInitiated; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
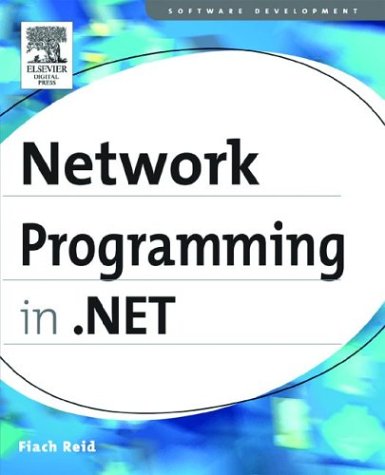
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SqlGenerator.cs
- XmlIncludeAttribute.cs
- TextRunTypographyProperties.cs
- XMLSyntaxException.cs
- DocumentPageView.cs
- NamedPermissionSet.cs
- Pair.cs
- HttpRequestWrapper.cs
- NamespaceCollection.cs
- WorkflowApplicationUnhandledExceptionEventArgs.cs
- DomainUpDown.cs
- StagingAreaInputItem.cs
- UpdateEventArgs.cs
- StringBlob.cs
- CharEnumerator.cs
- SemanticResultKey.cs
- WsdlBuildProvider.cs
- ComponentConverter.cs
- WindowsEditBoxRange.cs
- MetabaseSettingsIis7.cs
- DataRelationPropertyDescriptor.cs
- ToolStripDropTargetManager.cs
- NamespaceInfo.cs
- QueryStringParameter.cs
- MdiWindowListItemConverter.cs
- ElementMarkupObject.cs
- AsymmetricKeyExchangeDeformatter.cs
- DesignerDataSchemaClass.cs
- EdmToObjectNamespaceMap.cs
- JoinGraph.cs
- Model3D.cs
- SharedHttpTransportManager.cs
- SoapTypeAttribute.cs
- VisualState.cs
- LocalizableAttribute.cs
- HTMLTextWriter.cs
- TreeViewCancelEvent.cs
- TableLayoutPanelCellPosition.cs
- ObjectSet.cs
- RuntimeArgumentHandle.cs
- autovalidator.cs
- TextEditorMouse.cs
- CryptoApi.cs
- UniqueIdentifierService.cs
- RouteValueExpressionBuilder.cs
- MultipleViewProviderWrapper.cs
- SqlDataReader.cs
- ObjectViewListener.cs
- EventPrivateKey.cs
- StylesEditorDialog.cs
- IndexedString.cs
- QueueException.cs
- ModelService.cs
- DataGridViewRowPostPaintEventArgs.cs
- ClickablePoint.cs
- Region.cs
- SystemNetworkInterface.cs
- PerformanceCountersBase.cs
- NullableIntMinMaxAggregationOperator.cs
- SiteMapSection.cs
- DocComment.cs
- AutoCompleteStringCollection.cs
- Hashtable.cs
- MatchingStyle.cs
- GroupItemAutomationPeer.cs
- PersistNameAttribute.cs
- SelectionEditingBehavior.cs
- TextEditorTables.cs
- Pair.cs
- CodeTypeParameterCollection.cs
- Span.cs
- _TransmitFileOverlappedAsyncResult.cs
- InheritanceAttribute.cs
- MasterPageParser.cs
- GraphicsState.cs
- ObjectStorage.cs
- ListViewHitTestInfo.cs
- Schedule.cs
- HostingEnvironmentException.cs
- HiddenFieldPageStatePersister.cs
- DiscoveryInnerClientAdhocCD1.cs
- WindowsUpDown.cs
- AuthenticationSection.cs
- AnimationClock.cs
- GeometryHitTestParameters.cs
- DataGridViewTextBoxColumn.cs
- XmlArrayItemAttributes.cs
- DataContract.cs
- CryptographicAttribute.cs
- PenLineJoinValidation.cs
- LinqDataSourceInsertEventArgs.cs
- PropertyInformationCollection.cs
- SizeKeyFrameCollection.cs
- PropertyPathConverter.cs
- Context.cs
- AnchoredBlock.cs
- SecureStringHasher.cs
- GridViewRowCollection.cs
- NameValueFileSectionHandler.cs
- WorkflowCommandExtensionItem.cs