Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / xsp / System / Web / UI / MasterPageParser.cs / 1 / MasterPageParser.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* * Implements the ASP.NET master page parser * * Copyright (c) 1998 Microsoft Corporation */ namespace System.Web.UI { using System; using System.Collections; using System.IO; using System.Security; using System.Security.Permissions; using System.Web.Compilation; using System.Globalization; using System.Web; using System.Web.Util; /* * Parser for MasterPage */ internal sealed class MasterPageParser : UserControlParser { internal override Type DefaultBaseType { get { return typeof(System.Web.UI.MasterPage); } } internal new const string defaultDirectiveName = "master"; internal override string DefaultDirectiveName { get { return defaultDirectiveName; } } internal override Type DefaultFileLevelBuilderType { get { return typeof(FileLevelMasterPageControlBuilder); } } private Type _masterPageType; internal Type MasterPageType { get { return _masterPageType; } } private CaseInsensitiveStringSet _placeHolderList; internal CaseInsensitiveStringSet PlaceHolderList { get { if (_placeHolderList == null) _placeHolderList = new CaseInsensitiveStringSet(); return _placeHolderList; } } // Do not apply the basetype. Override this method // so the userControlbasetype do not affect masterpages. internal override void ApplyBaseType() { } internal override RootBuilder CreateDefaultFileLevelBuilder() { return new FileLevelMasterPageControlBuilder(); } internal override void ProcessDirective(string directiveName, IDictionary directive) { if (StringUtil.EqualsIgnoreCase(directiveName, "masterType")) { if (_masterPageType != null) { ProcessError(SR.GetString(SR.Only_one_directive_allowed, directiveName)); return; } _masterPageType = GetDirectiveType(directive, directiveName); Util.CheckAssignableType(typeof(MasterPage), _masterPageType); } // outputcaching is not allowed on masterpages. else if (StringUtil.EqualsIgnoreCase(directiveName, "outputcache")) { ProcessError(SR.GetString(SR.Directive_not_allowed, directiveName)); return; } else { base.ProcessDirective(directiveName, directive); } } internal override bool ProcessMainDirectiveAttribute(string deviceName, string name, string value, IDictionary parseData) { switch (name) { case "masterpagefile": // Skip validity check for expression builder (e.g. <%$ ... %>) if (IsExpressionBuilderValue(value)) return false; if (value.Length > 0) { // Add dependency on the Type by calling this method Type type = GetReferencedType(value); Util.CheckAssignableType(typeof(MasterPage), type); } // Return false to let the generic attribute processing continue return false; // outputcaching is not allowed on masterpages. case "outputcaching" : ProcessError(SR.GetString(SR.Attr_not_supported_in_directive, name, DefaultDirectiveName)); // Return false to let the generic attribute processing continue return false; default: // We didn't handle the attribute. Try the base class return base.ProcessMainDirectiveAttribute(deviceName, name, value, parseData); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* * Implements the ASP.NET master page parser * * Copyright (c) 1998 Microsoft Corporation */ namespace System.Web.UI { using System; using System.Collections; using System.IO; using System.Security; using System.Security.Permissions; using System.Web.Compilation; using System.Globalization; using System.Web; using System.Web.Util; /* * Parser for MasterPage */ internal sealed class MasterPageParser : UserControlParser { internal override Type DefaultBaseType { get { return typeof(System.Web.UI.MasterPage); } } internal new const string defaultDirectiveName = "master"; internal override string DefaultDirectiveName { get { return defaultDirectiveName; } } internal override Type DefaultFileLevelBuilderType { get { return typeof(FileLevelMasterPageControlBuilder); } } private Type _masterPageType; internal Type MasterPageType { get { return _masterPageType; } } private CaseInsensitiveStringSet _placeHolderList; internal CaseInsensitiveStringSet PlaceHolderList { get { if (_placeHolderList == null) _placeHolderList = new CaseInsensitiveStringSet(); return _placeHolderList; } } // Do not apply the basetype. Override this method // so the userControlbasetype do not affect masterpages. internal override void ApplyBaseType() { } internal override RootBuilder CreateDefaultFileLevelBuilder() { return new FileLevelMasterPageControlBuilder(); } internal override void ProcessDirective(string directiveName, IDictionary directive) { if (StringUtil.EqualsIgnoreCase(directiveName, "masterType")) { if (_masterPageType != null) { ProcessError(SR.GetString(SR.Only_one_directive_allowed, directiveName)); return; } _masterPageType = GetDirectiveType(directive, directiveName); Util.CheckAssignableType(typeof(MasterPage), _masterPageType); } // outputcaching is not allowed on masterpages. else if (StringUtil.EqualsIgnoreCase(directiveName, "outputcache")) { ProcessError(SR.GetString(SR.Directive_not_allowed, directiveName)); return; } else { base.ProcessDirective(directiveName, directive); } } internal override bool ProcessMainDirectiveAttribute(string deviceName, string name, string value, IDictionary parseData) { switch (name) { case "masterpagefile": // Skip validity check for expression builder (e.g. <%$ ... %>) if (IsExpressionBuilderValue(value)) return false; if (value.Length > 0) { // Add dependency on the Type by calling this method Type type = GetReferencedType(value); Util.CheckAssignableType(typeof(MasterPage), type); } // Return false to let the generic attribute processing continue return false; // outputcaching is not allowed on masterpages. case "outputcaching" : ProcessError(SR.GetString(SR.Attr_not_supported_in_directive, name, DefaultDirectiveName)); // Return false to let the generic attribute processing continue return false; default: // We didn't handle the attribute. Try the base class return base.ProcessMainDirectiveAttribute(deviceName, name, value, parseData); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
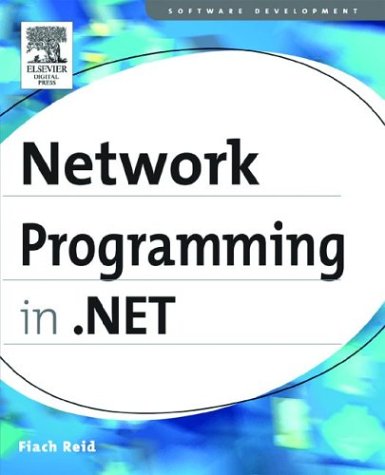
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MessageSmuggler.cs
- SchemaImporterExtensionElement.cs
- HttpVersion.cs
- StateWorkerRequest.cs
- MultiPropertyDescriptorGridEntry.cs
- WebPartEditorApplyVerb.cs
- unsafenativemethodstextservices.cs
- LinkedList.cs
- TCPListener.cs
- TemplateControlBuildProvider.cs
- HttpResponse.cs
- FormViewInsertedEventArgs.cs
- CacheVirtualItemsEvent.cs
- securitycriticaldataClass.cs
- RoleGroupCollection.cs
- WebControl.cs
- StickyNoteHelper.cs
- UserControlCodeDomTreeGenerator.cs
- SyndicationDeserializer.cs
- ConfigurationLockCollection.cs
- SqlDataSourceSelectingEventArgs.cs
- ContentTextAutomationPeer.cs
- ImageInfo.cs
- EncryptedKey.cs
- WebPartUtil.cs
- OperatingSystemVersionCheck.cs
- WriteableOnDemandStream.cs
- ResourcesGenerator.cs
- Pens.cs
- DataGridViewCellStyleBuilderDialog.cs
- RequestCachePolicy.cs
- OdbcConnectionPoolProviderInfo.cs
- AspProxy.cs
- sqlinternaltransaction.cs
- DataGridViewAutoSizeColumnModeEventArgs.cs
- DataGridViewCellStateChangedEventArgs.cs
- XPathAxisIterator.cs
- CollectionContainer.cs
- WebOperationContext.cs
- DragSelectionMessageFilter.cs
- ReadContentAsBinaryHelper.cs
- ValueUnavailableException.cs
- ResXResourceReader.cs
- ConfigXmlSignificantWhitespace.cs
- HttpProfileBase.cs
- XmlSchemaAll.cs
- ObjectManager.cs
- PointAnimationBase.cs
- XNodeValidator.cs
- CachedPathData.cs
- ColorMap.cs
- GorillaCodec.cs
- xdrvalidator.cs
- CmsInterop.cs
- SafeViewOfFileHandle.cs
- SoapConverter.cs
- MediaTimeline.cs
- XpsFilter.cs
- FontFamilyConverter.cs
- LayoutTableCell.cs
- ServicesUtilities.cs
- ButtonField.cs
- Font.cs
- Application.cs
- SrgsRule.cs
- EmptyStringExpandableObjectConverter.cs
- ConsoleKeyInfo.cs
- MetadataItemSerializer.cs
- ListBindableAttribute.cs
- InfocardChannelParameter.cs
- WebPartMovingEventArgs.cs
- SimpleWebHandlerParser.cs
- PagePropertiesChangingEventArgs.cs
- WorkflowInstance.cs
- XPathNode.cs
- BlurBitmapEffect.cs
- SafeProcessHandle.cs
- StructuredType.cs
- ItemDragEvent.cs
- ExtensionsSection.cs
- DataTableNameHandler.cs
- HttpResponseHeader.cs
- Events.cs
- KnownTypesHelper.cs
- DynamicRenderer.cs
- AutomationEvent.cs
- WebBrowser.cs
- CultureInfoConverter.cs
- XmlDataDocument.cs
- cache.cs
- ListViewItemSelectionChangedEvent.cs
- DBCommand.cs
- SvcMapFileSerializer.cs
- ItemList.cs
- MatrixTransform.cs
- CompilerErrorCollection.cs
- PolicyStatement.cs
- EndPoint.cs
- GestureRecognizer.cs
- CodeThrowExceptionStatement.cs