Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / MS / Internal / FontFace / MatchingStyle.cs / 1305600 / MatchingStyle.cs
//------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // // File: MatchingStyle.cs // // Contents: Font face matching style // // Created: 11-4-2003 Worachai Chaoweeraprasit (wchao) // 11-14-2005 mleonov - Removed style simulation logic. // Now simulated faces are created when constructing a font collection. // //----------------------------------------------------------------------- using System; using System.Diagnostics; using System.Security; using System.Runtime.InteropServices; using System.Windows; using System.Windows.Media; namespace MS.Internal.FontFace { ////// Font face style used for style matching of face /// internal struct MatchingStyle { private Vector _vector; // These should be prime numbers. private const double FontWeightScale = 5; private const double FontStyleScale = 7; private const double FontStretchScale = 11; internal MatchingStyle( FontStyle style, FontWeight weight, FontStretch stretch ) { _vector = new Vector( (stretch.ToOpenTypeStretch() - FontStretches.Normal.ToOpenTypeStretch()) * FontStretchScale, style.GetStyleForInternalConstruction() * FontStyleScale, (weight.ToOpenTypeWeight() - FontWeights.Normal.ToOpenTypeWeight()) / 100.0 * FontWeightScale ); } ////// Equality testing between two matching styles /// public static bool operator ==(MatchingStyle l, MatchingStyle r) { return l._vector == r._vector; } ////// Inequality testing between two matching styles /// public static bool operator !=(MatchingStyle l, MatchingStyle r) { return l._vector != r._vector; } ////// Equality testing between two matching styles /// public override bool Equals(Object o) { if(o == null) return false; return o is MatchingStyle && this == (MatchingStyle)o; } ////// Get hash code for this style /// public override int GetHashCode() { return _vector.GetHashCode(); } ////// See whether this is a better match to the specified matching target style /// /// matching target style /// current best match /// matching style internal static bool IsBetterMatch( MatchingStyle target, MatchingStyle best, ref MatchingStyle matching ) { return matching.IsBetterMatch(target, best); } ////// See whether this is a better match to the specified matching target /// internal bool IsBetterMatch( MatchingStyle target, MatchingStyle best ) { double currentDiffSize = (_vector - target._vector).LengthSquared; double bestDiffSize = (best._vector - target._vector).LengthSquared; // better match found when... if(currentDiffSize < bestDiffSize) { // the distance from the current vector to target is shorter return true; } else if(currentDiffSize == bestDiffSize) { double dotCurrent = Vector.DotProduct(_vector, target._vector); double dotBest = Vector.DotProduct(best._vector, target._vector); if(dotCurrent > dotBest) { // when distances are equal, the current vector has a stronger // projection onto target. return true; } else if(dotCurrent == dotBest) { if( _vector.X > best._vector.X || ( _vector.X == best._vector.X && ( _vector.Y > best._vector.Y || ( _vector.Y == best._vector.Y && _vector.Z > best._vector.Z)))) { // when projections onto target are still equally strong, the current // vector has a stronger component. return true; } } } return false; } ////// Small subset of 3D-vector implementation for style matching specific use /// ////// There is a generic Vector class in 3D land, but using it would bring in /// many other 3D types which is unnecessary for our limited use of vector. /// /// Using 3D types here would also mean introducing 3D types to FontCacheService /// code which logically should have nothing to do with it. /// private struct Vector { private double _x, _y, _z; ////// Constructor that sets vector's initial values. /// /// Value of the X coordinate of the new vector. /// Value of the Y coordinate of the new vector. /// Value of the Z coordinate of the new vector. internal Vector(double x, double y, double z) { _x = x; _y = y; _z = z; } ////// Retrieves or sets vector's X value. /// internal double X { get { return _x; } } ////// Retrieves or sets vector's Y value. /// internal double Y { get { return _y; } } ////// Retrieves or sets vector's Z value. /// internal double Z { get { return _z; } } ////// Length of the vector squared. /// internal double LengthSquared { get{ return _x * _x + _y * _y + _z * _z; } } ////// Vector dot product. /// internal static double DotProduct(Vector l, Vector r) { return l._x * r._x + l._y * r._y + l._z * r._z; } ////// Vector subtraction. /// public static Vector operator -(Vector l, Vector r) { return new Vector(l._x - r._x, l._y - r._y, l._z - r._z); } ////// Equality testing between two vectors. /// public static bool operator ==(Vector l, Vector r) { return ((l._x == r._x) && (l._y == r._y) && (l._z == r._z)); } ////// Inequality testing between two vectors. /// public static bool operator !=(Vector l, Vector r) { return !(l == r); } ////// Equality testing between the vector and a given object. /// public override bool Equals(Object o) { if(null == o) { return false; } if(o is Vector) { Vector vector = (Vector)o; return (this == vector); } else { return false; } } ////// Compute a hash code for this vector. /// public override int GetHashCode() { return _x.GetHashCode() ^ _y.GetHashCode() ^ _z.GetHashCode(); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // // File: MatchingStyle.cs // // Contents: Font face matching style // // Created: 11-4-2003 Worachai Chaoweeraprasit (wchao) // 11-14-2005 mleonov - Removed style simulation logic. // Now simulated faces are created when constructing a font collection. // //----------------------------------------------------------------------- using System; using System.Diagnostics; using System.Security; using System.Runtime.InteropServices; using System.Windows; using System.Windows.Media; namespace MS.Internal.FontFace { ////// Font face style used for style matching of face /// internal struct MatchingStyle { private Vector _vector; // These should be prime numbers. private const double FontWeightScale = 5; private const double FontStyleScale = 7; private const double FontStretchScale = 11; internal MatchingStyle( FontStyle style, FontWeight weight, FontStretch stretch ) { _vector = new Vector( (stretch.ToOpenTypeStretch() - FontStretches.Normal.ToOpenTypeStretch()) * FontStretchScale, style.GetStyleForInternalConstruction() * FontStyleScale, (weight.ToOpenTypeWeight() - FontWeights.Normal.ToOpenTypeWeight()) / 100.0 * FontWeightScale ); } ////// Equality testing between two matching styles /// public static bool operator ==(MatchingStyle l, MatchingStyle r) { return l._vector == r._vector; } ////// Inequality testing between two matching styles /// public static bool operator !=(MatchingStyle l, MatchingStyle r) { return l._vector != r._vector; } ////// Equality testing between two matching styles /// public override bool Equals(Object o) { if(o == null) return false; return o is MatchingStyle && this == (MatchingStyle)o; } ////// Get hash code for this style /// public override int GetHashCode() { return _vector.GetHashCode(); } ////// See whether this is a better match to the specified matching target style /// /// matching target style /// current best match /// matching style internal static bool IsBetterMatch( MatchingStyle target, MatchingStyle best, ref MatchingStyle matching ) { return matching.IsBetterMatch(target, best); } ////// See whether this is a better match to the specified matching target /// internal bool IsBetterMatch( MatchingStyle target, MatchingStyle best ) { double currentDiffSize = (_vector - target._vector).LengthSquared; double bestDiffSize = (best._vector - target._vector).LengthSquared; // better match found when... if(currentDiffSize < bestDiffSize) { // the distance from the current vector to target is shorter return true; } else if(currentDiffSize == bestDiffSize) { double dotCurrent = Vector.DotProduct(_vector, target._vector); double dotBest = Vector.DotProduct(best._vector, target._vector); if(dotCurrent > dotBest) { // when distances are equal, the current vector has a stronger // projection onto target. return true; } else if(dotCurrent == dotBest) { if( _vector.X > best._vector.X || ( _vector.X == best._vector.X && ( _vector.Y > best._vector.Y || ( _vector.Y == best._vector.Y && _vector.Z > best._vector.Z)))) { // when projections onto target are still equally strong, the current // vector has a stronger component. return true; } } } return false; } ////// Small subset of 3D-vector implementation for style matching specific use /// ////// There is a generic Vector class in 3D land, but using it would bring in /// many other 3D types which is unnecessary for our limited use of vector. /// /// Using 3D types here would also mean introducing 3D types to FontCacheService /// code which logically should have nothing to do with it. /// private struct Vector { private double _x, _y, _z; ////// Constructor that sets vector's initial values. /// /// Value of the X coordinate of the new vector. /// Value of the Y coordinate of the new vector. /// Value of the Z coordinate of the new vector. internal Vector(double x, double y, double z) { _x = x; _y = y; _z = z; } ////// Retrieves or sets vector's X value. /// internal double X { get { return _x; } } ////// Retrieves or sets vector's Y value. /// internal double Y { get { return _y; } } ////// Retrieves or sets vector's Z value. /// internal double Z { get { return _z; } } ////// Length of the vector squared. /// internal double LengthSquared { get{ return _x * _x + _y * _y + _z * _z; } } ////// Vector dot product. /// internal static double DotProduct(Vector l, Vector r) { return l._x * r._x + l._y * r._y + l._z * r._z; } ////// Vector subtraction. /// public static Vector operator -(Vector l, Vector r) { return new Vector(l._x - r._x, l._y - r._y, l._z - r._z); } ////// Equality testing between two vectors. /// public static bool operator ==(Vector l, Vector r) { return ((l._x == r._x) && (l._y == r._y) && (l._z == r._z)); } ////// Inequality testing between two vectors. /// public static bool operator !=(Vector l, Vector r) { return !(l == r); } ////// Equality testing between the vector and a given object. /// public override bool Equals(Object o) { if(null == o) { return false; } if(o is Vector) { Vector vector = (Vector)o; return (this == vector); } else { return false; } } ////// Compute a hash code for this vector. /// public override int GetHashCode() { return _x.GetHashCode() ^ _y.GetHashCode() ^ _z.GetHashCode(); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
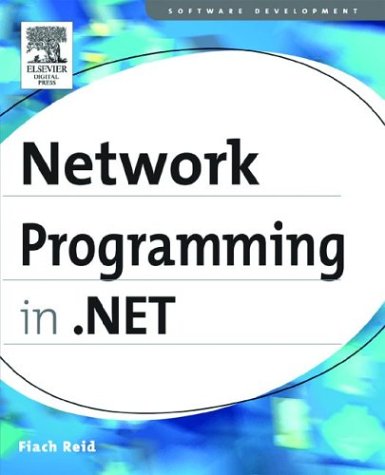
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ElementAction.cs
- SplineQuaternionKeyFrame.cs
- SerializationException.cs
- ExpandoClass.cs
- XmlConvert.cs
- SystemBrushes.cs
- WindowsGraphicsCacheManager.cs
- MDIClient.cs
- WebZoneDesigner.cs
- SnapshotChangeTrackingStrategy.cs
- BitmapMetadata.cs
- BlurBitmapEffect.cs
- EventWaitHandle.cs
- CallbackDebugElement.cs
- XmlWhitespace.cs
- SmiGettersStream.cs
- HostedHttpContext.cs
- DataGridViewAdvancedBorderStyle.cs
- TriggerAction.cs
- BuildProviderCollection.cs
- VarRefManager.cs
- ToolStripKeyboardHandlingService.cs
- EntityDataSourceWrapperCollection.cs
- ListenerChannelContext.cs
- WindowsTitleBar.cs
- SoapMessage.cs
- PropertyItemInternal.cs
- Adorner.cs
- TCEAdapterGenerator.cs
- LabelExpression.cs
- Image.cs
- AssemblyAttributes.cs
- QuinticEase.cs
- Serialization.cs
- _TLSstream.cs
- PassportAuthenticationModule.cs
- TextRange.cs
- WebPartConnectVerb.cs
- TemplateControl.cs
- PackagingUtilities.cs
- XmlSchemaAnnotation.cs
- LogicalExpressionTypeConverter.cs
- NestedContainer.cs
- Repeater.cs
- RegexRunnerFactory.cs
- SelectorAutomationPeer.cs
- SpanIndex.cs
- TemplateBindingExtension.cs
- CompositeFontInfo.cs
- sqlstateclientmanager.cs
- Parallel.cs
- InsufficientMemoryException.cs
- RestHandlerFactory.cs
- ListViewSelectEventArgs.cs
- PropertyItem.cs
- IOException.cs
- CSharpCodeProvider.cs
- SecurityCriticalDataForSet.cs
- ScriptComponentDescriptor.cs
- Int16.cs
- XpsResourcePolicy.cs
- DataListItemEventArgs.cs
- LogSwitch.cs
- SQLDateTimeStorage.cs
- COMException.cs
- UriSection.cs
- OutputBuffer.cs
- RenderDataDrawingContext.cs
- TrackingLocationCollection.cs
- KeyInfo.cs
- WrappedReader.cs
- Parser.cs
- IgnoreDataMemberAttribute.cs
- DoubleLinkListEnumerator.cs
- SystemUdpStatistics.cs
- SynchronizationFilter.cs
- LZCodec.cs
- Int64Storage.cs
- Tool.cs
- MsmqInputMessagePool.cs
- ScrollData.cs
- DeleteIndexBinder.cs
- ErrorInfoXmlDocument.cs
- CodeVariableDeclarationStatement.cs
- GridViewCancelEditEventArgs.cs
- TextTreeRootTextBlock.cs
- Metadata.cs
- FromReply.cs
- SQLInt16.cs
- CommandID.cs
- BamlResourceContent.cs
- SqlDataSourceFilteringEventArgs.cs
- WebControlParameterProxy.cs
- KeyGestureConverter.cs
- FlatButtonAppearance.cs
- RoleManagerSection.cs
- TemplateControl.cs
- RangeBase.cs
- DefaultExpression.cs
- CorePropertiesFilter.cs