Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / infocard / Service / managed / Microsoft / InfoCards / InfocardClientCredentials.cs / 1 / InfocardClientCredentials.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace Microsoft.InfoCards { using System; using System.IdentityModel.Tokens; using System.IdentityModel.Selectors; using System.ServiceModel; using System.ServiceModel.Description; using System.ServiceModel.Security; using System.ServiceModel.Security.Tokens; using System.ServiceModel.Dispatcher; using IDT = Microsoft.InfoCards.Diagnostics.InfoCardTrace; // // Summary // This class provides the credentials for authentication using self issued cards // internal class InfoCardServiceClientCredentials : ClientCredentials { InfoCard m_card; TokenFactoryCredential m_credentials; bool m_isSelfIssued; ProtocolProfile m_protocolProfile; RSATokenProvider m_endorsingSigTokenProvider; // // CTOR // public InfoCardServiceClientCredentials( TokenFactoryCredential creds, ProtocolProfile profile ) { m_credentials = creds; m_protocolProfile = profile; } public InfoCardServiceClientCredentials(InfoCardServiceClientCredentials other, ProtocolProfile profile ) : base(other) { m_credentials = other.m_credentials; m_endorsingSigTokenProvider = other.m_endorsingSigTokenProvider; m_protocolProfile = profile; } public InfoCard SelectedCard { get{ return m_card; } set{ m_card = value; } } public bool IsSelfIssuedCred { get { return m_isSelfIssued; } set { m_isSelfIssued = value; } } public RSATokenProvider EndorsingSignatureTokenProvider { get { return m_endorsingSigTokenProvider; } set { m_endorsingSigTokenProvider = value; } } public ProtocolProfile ProtocolVersionProfile { get { return m_protocolProfile; } } protected override ClientCredentials CloneCore() { return new InfoCardServiceClientCredentials( this, m_protocolProfile ); } public override void ApplyClientBehavior(ServiceEndpoint serviceEndpoint, ClientRuntime behavior) { } public override SecurityTokenManager CreateSecurityTokenManager() { return new InfoCardServiceClientCredentialsSecurityTokenManager(this); } class InfoCardServiceClientCredentialsSecurityTokenManager : ClientCredentialsSecurityTokenManager { InfoCard m_card; TokenFactoryCredential m_credentials; ProtocolProfile m_protocolProfile; public InfoCardServiceClientCredentialsSecurityTokenManager(InfoCardServiceClientCredentials creds) : base(creds) { m_card = creds.SelectedCard; m_credentials = creds.m_credentials; m_protocolProfile = creds.ProtocolVersionProfile; } public override SecurityTokenProvider CreateSecurityTokenProvider(SecurityTokenRequirement tokenRequirement) { if (tokenRequirement == null) { throw IDT.ThrowHelperArgumentNull("tokenRequirement"); } string tokenType = tokenRequirement.TokenType; bool needUseKey = null != ((InfoCardServiceClientCredentials)ClientCredentials).EndorsingSignatureTokenProvider; // // Check if the credential type that is requested matches the one in the selcted card. // If sucessful, return the appropriate tokenprovider. // if (IsIssuedSecurityTokenRequirement(tokenRequirement)) { if (m_credentials.CredentialType != TokenFactoryCredentialType.SelfIssuedCredential) { throw IDT.ThrowHelperError(new TokenCreationException(SR.GetString(SR.CardDoesNotMatchRequiredAuthType))); } IssuedSecurityTokenParameters itp = tokenRequirement.GetProperty(ServiceModelSecurityTokenRequirement.IssuedSecurityTokenParametersProperty); EndpointAddress target = tokenRequirement.GetProperty (ServiceModelSecurityTokenRequirement.TargetAddressProperty); if( itp.IssuerAddress != null && Utility.CompareUri( itp.IssuerAddress.Uri, XmlNames.WSIdentity.SelfIssuerUriValue ) ) { return new CustomTokenProvider( itp, m_card, target, ((InfoCardServiceClientCredentials)base.ClientCredentials).IsSelfIssuedCred, m_protocolProfile ); } else { throw IDT.ThrowHelperError(new TokenCreationException(SR.GetString(SR.InvalidIssuerForIssuedToken))); } } else if (tokenType == SecurityTokenTypes.X509Certificate) { if (tokenRequirement.KeyUsage == SecurityKeyUsage.Signature) { if (m_credentials.CredentialType != TokenFactoryCredentialType.X509CertificateCredential) { throw IDT.ThrowHelperError(new TokenCreationException(SR.GetString(SR.CardDoesNotMatchRequiredAuthType))); } return new RemoteCryptoTokenProvider(this.ClientCredentials.ClientCertificate.Certificate); } else { return base.CreateSecurityTokenProvider(tokenRequirement); } } else if (tokenType == ServiceModelSecurityTokenTypes.MutualSslnego) { if (m_credentials.CredentialType != TokenFactoryCredentialType.X509CertificateCredential) { throw IDT.ThrowHelperError(new TokenCreationException(SR.GetString(SR.CardDoesNotMatchRequiredAuthType))); } return base.CreateSecurityTokenProvider(tokenRequirement); } else if (tokenType == ServiceModelSecurityTokenTypes.AnonymousSslnego) { return base.CreateSecurityTokenProvider(tokenRequirement); } else if (tokenType == ServiceModelSecurityTokenTypes.SecureConversation) { return base.CreateSecurityTokenProvider(tokenRequirement); } else if (tokenType == SecurityTokenTypes.Kerberos || tokenType == ServiceModelSecurityTokenTypes.Spnego) { if (m_credentials.CredentialType != TokenFactoryCredentialType.KerberosCredential) { throw IDT.ThrowHelperError(new TokenCreationException(SR.GetString(SR.CardDoesNotMatchRequiredAuthType))); } return base.CreateSecurityTokenProvider(tokenRequirement); } else if (tokenType == SecurityTokenTypes.UserName) { if (m_credentials.CredentialType != TokenFactoryCredentialType.UserNamePasswordCredential) { throw IDT.ThrowHelperError(new TokenCreationException(SR.GetString(SR.CardDoesNotMatchRequiredAuthType))); } return base.CreateSecurityTokenProvider(tokenRequirement); } else if (tokenType == ServiceModelSecurityTokenTypes.SspiCredential) { if (m_credentials.CredentialType != TokenFactoryCredentialType.KerberosCredential && m_credentials.CredentialType != TokenFactoryCredentialType.UserNamePasswordCredential) { throw IDT.ThrowHelperError(new TokenCreationException(SR.GetString(SR.CardDoesNotMatchRequiredAuthType))); } return base.CreateSecurityTokenProvider(tokenRequirement); } else if( tokenType == SecurityTokenTypes.Rsa && needUseKey ) { // // If this is being asked for it is to prove posession of a private key associated with a public // key passed in the UseKey field of an RST. // InfoCardServiceClientCredentials icClientCreds = (InfoCardServiceClientCredentials)ClientCredentials; return icClientCreds.EndorsingSignatureTokenProvider; } else { return base.CreateSecurityTokenProvider( tokenRequirement ); } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
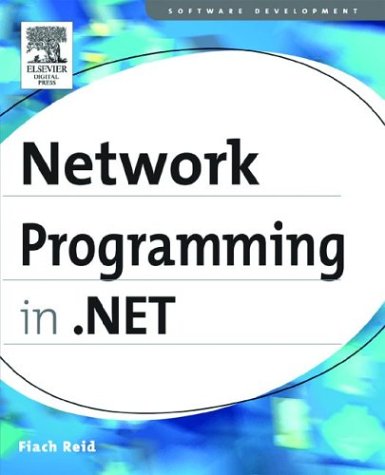
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Point.cs
- BinaryKeyIdentifierClause.cs
- DataControlFieldHeaderCell.cs
- DbExpressionVisitor.cs
- PackageDigitalSignature.cs
- TextHidden.cs
- X509RecipientCertificateClientElement.cs
- PrintPageEvent.cs
- InvalidDataContractException.cs
- LazyTextWriterCreator.cs
- PathGradientBrush.cs
- TaskDesigner.cs
- LowerCaseStringConverter.cs
- Vars.cs
- OAVariantLib.cs
- ErrorLog.cs
- CircleHotSpot.cs
- Matrix.cs
- mactripleDES.cs
- ExtensionSimplifierMarkupObject.cs
- RefreshPropertiesAttribute.cs
- FrameworkElement.cs
- TimeoutTimer.cs
- OutputCacheSection.cs
- WindowsMenu.cs
- CodeArrayCreateExpression.cs
- StretchValidation.cs
- TabControlEvent.cs
- StyleCollection.cs
- FormsAuthentication.cs
- ZipIORawDataFileBlock.cs
- HttpCacheVaryByContentEncodings.cs
- SubMenuStyleCollection.cs
- FormsAuthenticationUserCollection.cs
- GetKeyedHashRequest.cs
- PolicyLevel.cs
- Quaternion.cs
- CompilerHelpers.cs
- HotSpot.cs
- KnowledgeBase.cs
- XmlObjectSerializerWriteContextComplex.cs
- ObjectListItem.cs
- SystemResourceKey.cs
- SqlRetyper.cs
- TextDecoration.cs
- DataConnectionHelper.cs
- figurelengthconverter.cs
- coordinatorfactory.cs
- MultilineStringConverter.cs
- UntypedNullExpression.cs
- WeakReference.cs
- WebServiceParameterData.cs
- PrivateFontCollection.cs
- ResolvedKeyFrameEntry.cs
- XhtmlTextWriter.cs
- TreeViewImageGenerator.cs
- ParagraphVisual.cs
- OdbcDataAdapter.cs
- XPathParser.cs
- IsolatedStoragePermission.cs
- TreeView.cs
- PictureBoxDesigner.cs
- QilStrConcat.cs
- RuntimeHandles.cs
- PolyQuadraticBezierSegmentFigureLogic.cs
- CultureInfoConverter.cs
- RectangleGeometry.cs
- BatchParser.cs
- FunctionCommandText.cs
- ControlCollection.cs
- PathData.cs
- SessionEndedEventArgs.cs
- DataGridRelationshipRow.cs
- COM2PropertyPageUITypeConverter.cs
- Win32PrintDialog.cs
- DesignerRegion.cs
- Point4D.cs
- CryptographicAttribute.cs
- Color.cs
- Certificate.cs
- RotateTransform.cs
- ScriptManagerProxy.cs
- TaskFileService.cs
- DefaultValueTypeConverter.cs
- PKCS1MaskGenerationMethod.cs
- StringValidatorAttribute.cs
- TypeElement.cs
- SystemNetHelpers.cs
- PropertySourceInfo.cs
- NameGenerator.cs
- SqlStream.cs
- ClockGroup.cs
- IChannel.cs
- DiscriminatorMap.cs
- EmptyReadOnlyDictionaryInternal.cs
- LinkedResourceCollection.cs
- HwndProxyElementProvider.cs
- ToolStripSplitButton.cs
- WebPartEditVerb.cs
- SqlNamer.cs