Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / WinForms / Managed / System / WinForms / ComponentModel / COM2Interop / COM2PropertyPageUITypeConverter.cs / 1305376 / COM2PropertyPageUITypeConverter.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms.ComponentModel.Com2Interop { using Microsoft.Win32; using System; using System.ComponentModel; using System.ComponentModel.Design; using System.Diagnostics; using System.Drawing; using System.Drawing.Design; using System.Runtime.InteropServices; using System.Windows.Forms.Design; ////// /// internal class Com2PropertyPageUITypeEditor : Com2ExtendedUITypeEditor, ICom2PropertyPageDisplayService { private Com2PropertyDescriptor propDesc; private Guid guid; public Com2PropertyPageUITypeEditor(Com2PropertyDescriptor pd, Guid guid, UITypeEditor baseEditor) : base(baseEditor){ propDesc = pd; this.guid = guid; } ////// /// Takes the value returned from valueAccess.getValue() and modifies or replaces /// the value, passing the result into valueAccess.setValue(). This is where /// an editor can launch a modal dialog or create a drop down editor to allow /// the user to modify the value. Host assistance in presenting UI to the user /// can be found through the valueAccess.getService function. /// public override object EditValue(ITypeDescriptorContext context, IServiceProvider provider, object value) { IntPtr hWndParent = UnsafeNativeMethods.GetFocus(); // Windows.GetForegroundWindow try { ICom2PropertyPageDisplayService propPageSvc = (ICom2PropertyPageDisplayService)provider.GetService(typeof(ICom2PropertyPageDisplayService)); if (propPageSvc == null) { propPageSvc = this; } object instance = context.Instance; if (!instance.GetType().IsArray) { instance = propDesc.TargetObject; if (instance is ICustomTypeDescriptor) { instance = ((ICustomTypeDescriptor)instance).GetPropertyOwner(propDesc); } } propPageSvc.ShowPropertyPage(propDesc.Name, instance, propDesc.DISPID, this.guid, hWndParent); } catch (Exception ex1) { if (provider != null) { IUIService uiSvc = (IUIService)provider.GetService(typeof(IUIService)); if (uiSvc != null){ uiSvc.ShowError(ex1, SR.GetString(SR.ErrorTypeConverterFailed)); } } } return value; } ////// /// Retrieves the editing style of the Edit method. If the method /// is not supported, this will return None. /// public override UITypeEditorEditStyle GetEditStyle(ITypeDescriptorContext context) { return UITypeEditorEditStyle.Modal; } public unsafe void ShowPropertyPage(string title, object component, int dispid, Guid pageGuid, IntPtr parentHandle){ Guid[] guids = new Guid[]{pageGuid}; IntPtr guidsAddr = Marshal.UnsafeAddrOfPinnedArrayElement(guids, 0); object[] objs = component.GetType().IsArray ? (object[])component : new object[]{component}; int nObjs = objs.Length; IntPtr[] objAddrs = new IntPtr[nObjs]; try { for (int i=0; i < nObjs; i++) { objAddrs[i] = Marshal.GetIUnknownForObject(objs[i]); } fixed (IntPtr* pAddrs = objAddrs) { SafeNativeMethods.OleCreatePropertyFrame(new HandleRef(null, parentHandle), 0, 0, title, nObjs, new HandleRef(null, (IntPtr)(long)pAddrs), 1, new HandleRef(null, guidsAddr), SafeNativeMethods.GetThreadLCID(), 0, IntPtr.Zero ); } } finally { for (int i=0; i < nObjs; i++) { if (objAddrs[i] != IntPtr.Zero) { Marshal.Release(objAddrs[i]); } } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms.ComponentModel.Com2Interop { using Microsoft.Win32; using System; using System.ComponentModel; using System.ComponentModel.Design; using System.Diagnostics; using System.Drawing; using System.Drawing.Design; using System.Runtime.InteropServices; using System.Windows.Forms.Design; ////// /// internal class Com2PropertyPageUITypeEditor : Com2ExtendedUITypeEditor, ICom2PropertyPageDisplayService { private Com2PropertyDescriptor propDesc; private Guid guid; public Com2PropertyPageUITypeEditor(Com2PropertyDescriptor pd, Guid guid, UITypeEditor baseEditor) : base(baseEditor){ propDesc = pd; this.guid = guid; } ////// /// Takes the value returned from valueAccess.getValue() and modifies or replaces /// the value, passing the result into valueAccess.setValue(). This is where /// an editor can launch a modal dialog or create a drop down editor to allow /// the user to modify the value. Host assistance in presenting UI to the user /// can be found through the valueAccess.getService function. /// public override object EditValue(ITypeDescriptorContext context, IServiceProvider provider, object value) { IntPtr hWndParent = UnsafeNativeMethods.GetFocus(); // Windows.GetForegroundWindow try { ICom2PropertyPageDisplayService propPageSvc = (ICom2PropertyPageDisplayService)provider.GetService(typeof(ICom2PropertyPageDisplayService)); if (propPageSvc == null) { propPageSvc = this; } object instance = context.Instance; if (!instance.GetType().IsArray) { instance = propDesc.TargetObject; if (instance is ICustomTypeDescriptor) { instance = ((ICustomTypeDescriptor)instance).GetPropertyOwner(propDesc); } } propPageSvc.ShowPropertyPage(propDesc.Name, instance, propDesc.DISPID, this.guid, hWndParent); } catch (Exception ex1) { if (provider != null) { IUIService uiSvc = (IUIService)provider.GetService(typeof(IUIService)); if (uiSvc != null){ uiSvc.ShowError(ex1, SR.GetString(SR.ErrorTypeConverterFailed)); } } } return value; } ////// /// Retrieves the editing style of the Edit method. If the method /// is not supported, this will return None. /// public override UITypeEditorEditStyle GetEditStyle(ITypeDescriptorContext context) { return UITypeEditorEditStyle.Modal; } public unsafe void ShowPropertyPage(string title, object component, int dispid, Guid pageGuid, IntPtr parentHandle){ Guid[] guids = new Guid[]{pageGuid}; IntPtr guidsAddr = Marshal.UnsafeAddrOfPinnedArrayElement(guids, 0); object[] objs = component.GetType().IsArray ? (object[])component : new object[]{component}; int nObjs = objs.Length; IntPtr[] objAddrs = new IntPtr[nObjs]; try { for (int i=0; i < nObjs; i++) { objAddrs[i] = Marshal.GetIUnknownForObject(objs[i]); } fixed (IntPtr* pAddrs = objAddrs) { SafeNativeMethods.OleCreatePropertyFrame(new HandleRef(null, parentHandle), 0, 0, title, nObjs, new HandleRef(null, (IntPtr)(long)pAddrs), 1, new HandleRef(null, guidsAddr), SafeNativeMethods.GetThreadLCID(), 0, IntPtr.Zero ); } } finally { for (int i=0; i < nObjs; i++) { if (objAddrs[i] != IntPtr.Zero) { Marshal.Release(objAddrs[i]); } } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
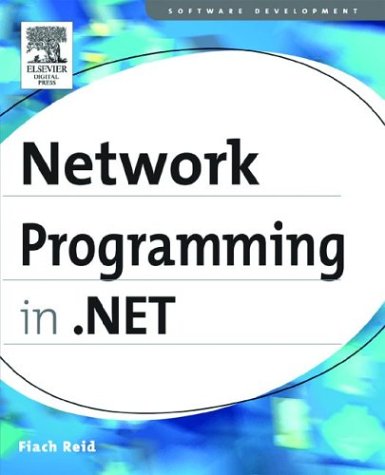
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- EdmEntityTypeAttribute.cs
- GroupBox.cs
- Queue.cs
- StateManagedCollection.cs
- ServiceHttpHandlerFactory.cs
- SchemaNotation.cs
- ExpressionCopier.cs
- DiscoveryEndpointElement.cs
- IntranetCredentialPolicy.cs
- StringUtil.cs
- Package.cs
- SymbolMethod.cs
- DocumentReferenceCollection.cs
- JsonUriDataContract.cs
- ExpressionBinding.cs
- TextParagraphCache.cs
- WebMessageBodyStyleHelper.cs
- WebBrowserHelper.cs
- ConfigXmlText.cs
- XmlException.cs
- OracleParameterCollection.cs
- QilInvokeEarlyBound.cs
- ActivationServices.cs
- SharedStream.cs
- PathFigureCollection.cs
- DataServiceException.cs
- ResourceType.cs
- EventMappingSettings.cs
- TableCell.cs
- DirectoryRedirect.cs
- SafeReversePInvokeHandle.cs
- FixedTextView.cs
- SizeLimitedCache.cs
- HttpModuleAction.cs
- Triplet.cs
- XslAst.cs
- safesecurityhelperavalon.cs
- UrlPath.cs
- WebPartEditVerb.cs
- OSFeature.cs
- UrlRoutingHandler.cs
- MailMessage.cs
- FontStretchConverter.cs
- ObjectDataSourceSelectingEventArgs.cs
- ConsumerConnectionPointCollection.cs
- SqlDataSourceCommandEventArgs.cs
- ObservableCollection.cs
- StrokeNodeOperations2.cs
- SynchronizedInputProviderWrapper.cs
- FamilyMap.cs
- EntityProviderServices.cs
- PropertyEmitter.cs
- Registration.cs
- DispatchWrapper.cs
- SqlNotificationEventArgs.cs
- RunClient.cs
- DesignerWebPartChrome.cs
- SpeechEvent.cs
- basemetadatamappingvisitor.cs
- StringAnimationBase.cs
- FrameworkTemplate.cs
- DrawingCollection.cs
- PersianCalendar.cs
- XPathExpr.cs
- SystemIPGlobalStatistics.cs
- ProfileGroupSettings.cs
- SoapSchemaExporter.cs
- TransformDescriptor.cs
- ScriptHandlerFactory.cs
- StylusTip.cs
- TableStyle.cs
- OracleBFile.cs
- TextEncodedRawTextWriter.cs
- DataKeyCollection.cs
- BuildProvider.cs
- DocumentGridContextMenu.cs
- Enumerable.cs
- MessageSmuggler.cs
- StickyNote.cs
- RegisteredArrayDeclaration.cs
- ImageClickEventArgs.cs
- TraceHandler.cs
- IisTraceWebEventProvider.cs
- XmlCollation.cs
- XamlSerializerUtil.cs
- BuiltInExpr.cs
- AuthenticatingEventArgs.cs
- TimersDescriptionAttribute.cs
- AccessedThroughPropertyAttribute.cs
- Light.cs
- ResourceReferenceKeyNotFoundException.cs
- SendKeys.cs
- ConnectivityStatus.cs
- SymbolEqualComparer.cs
- MetadataArtifactLoaderResource.cs
- TableCell.cs
- KoreanCalendar.cs
- _Win32.cs
- ChtmlPhoneCallAdapter.cs
- XmlPropertyBag.cs