Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Core / CSharp / System / Windows / Media / Animation / Generated / StringAnimationBase.cs / 1 / StringAnimationBase.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // Please see http://wiki/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- // Allow use of presharp: #pragma warning suppress#pragma warning disable 1634, 1691 using MS.Internal; using System; using System.Collections; using System.ComponentModel; using System.Diagnostics; using System.Windows.Media.Animation; using System.Windows.Media.Media3D; using MS.Internal.PresentationCore; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Media.Animation { /// /// /// public abstract class StringAnimationBase : AnimationTimeline { #region Constructors ////// Creates a new StringAnimationBase. /// protected StringAnimationBase() : base() { } #endregion #region Freezable ////// Creates a copy of this StringAnimationBase /// ///The copy public new StringAnimationBase Clone() { return (StringAnimationBase)base.Clone(); } #endregion #region IAnimation ////// Calculates the value this animation believes should be the current value for the property. /// /// /// This value is the suggested origin value provided to the animation /// to be used if the animation does not have its own concept of a /// start value. If this animation is the first in a composition chain /// this value will be the snapshot value if one is available or the /// base property value if it is not; otherise this value will be the /// value returned by the previous animation in the chain with an /// animationClock that is not Stopped. /// /// /// This value is the suggested destination value provided to the animation /// to be used if the animation does not have its own concept of an /// end value. This value will be the base value if the animation is /// in the first composition layer of animations on a property; /// otherwise this value will be the output value from the previous /// composition layer of animations for the property. /// /// /// This is the animationClock which can generate the CurrentTime or /// CurrentProgress value to be used by the animation to generate its /// output value. /// ////// The value this animation believes should be the current value for the property. /// public override sealed object GetCurrentValue(object defaultOriginValue, object defaultDestinationValue, AnimationClock animationClock) { return GetCurrentValue((String)defaultOriginValue, (String)defaultDestinationValue, animationClock); } ////// Returns the type of the target property /// public override sealed Type TargetPropertyType { get { ReadPreamble(); return typeof(String); } } #endregion #region Methods ////// Calculates the value this animation believes should be the current value for the property. /// /// /// This value is the suggested origin value provided to the animation /// to be used if the animation does not have its own concept of a /// start value. If this animation is the first in a composition chain /// this value will be the snapshot value if one is available or the /// base property value if it is not; otherise this value will be the /// value returned by the previous animation in the chain with an /// animationClock that is not Stopped. /// /// /// This value is the suggested destination value provided to the animation /// to be used if the animation does not have its own concept of an /// end value. This value will be the base value if the animation is /// in the first composition layer of animations on a property; /// otherwise this value will be the output value from the previous /// composition layer of animations for the property. /// /// /// This is the animationClock which can generate the CurrentTime or /// CurrentProgress value to be used by the animation to generate its /// output value. /// ////// The value this animation believes should be the current value for the property. /// public String GetCurrentValue(String defaultOriginValue, String defaultDestinationValue, AnimationClock animationClock) { ReadPreamble(); if (animationClock == null) { throw new ArgumentNullException("animationClock"); } // We check for null above but presharp doesn't notice so we suppress the // warning here. #pragma warning suppress 6506 if (animationClock.CurrentState == ClockState.Stopped) { return defaultDestinationValue; } /* if (!AnimatedTypeHelpers.IsValidAnimationValueString(defaultDestinationValue)) { throw new ArgumentException( SR.Get( SRID.Animation_InvalidBaseValue, defaultDestinationValue, defaultDestinationValue.GetType(), GetType()), "defaultDestinationValue"); } */ return GetCurrentValueCore(defaultOriginValue, defaultDestinationValue, animationClock); } ////// Calculates the value this animation believes should be the current value for the property. /// /// /// This value is the suggested origin value provided to the animation /// to be used if the animation does not have its own concept of a /// start value. If this animation is the first in a composition chain /// this value will be the snapshot value if one is available or the /// base property value if it is not; otherise this value will be the /// value returned by the previous animation in the chain with an /// animationClock that is not Stopped. /// /// /// This value is the suggested destination value provided to the animation /// to be used if the animation does not have its own concept of an /// end value. This value will be the base value if the animation is /// in the first composition layer of animations on a property; /// otherwise this value will be the output value from the previous /// composition layer of animations for the property. /// /// /// This is the animationClock which can generate the CurrentTime or /// CurrentProgress value to be used by the animation to generate its /// output value. /// ////// The value this animation believes should be the current value for the property. /// protected abstract String GetCurrentValueCore(String defaultOriginValue, String defaultDestinationValue, AnimationClock animationClock); #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // Please see http://wiki/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- // Allow use of presharp: #pragma warning suppress#pragma warning disable 1634, 1691 using MS.Internal; using System; using System.Collections; using System.ComponentModel; using System.Diagnostics; using System.Windows.Media.Animation; using System.Windows.Media.Media3D; using MS.Internal.PresentationCore; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Media.Animation { /// /// /// public abstract class StringAnimationBase : AnimationTimeline { #region Constructors ////// Creates a new StringAnimationBase. /// protected StringAnimationBase() : base() { } #endregion #region Freezable ////// Creates a copy of this StringAnimationBase /// ///The copy public new StringAnimationBase Clone() { return (StringAnimationBase)base.Clone(); } #endregion #region IAnimation ////// Calculates the value this animation believes should be the current value for the property. /// /// /// This value is the suggested origin value provided to the animation /// to be used if the animation does not have its own concept of a /// start value. If this animation is the first in a composition chain /// this value will be the snapshot value if one is available or the /// base property value if it is not; otherise this value will be the /// value returned by the previous animation in the chain with an /// animationClock that is not Stopped. /// /// /// This value is the suggested destination value provided to the animation /// to be used if the animation does not have its own concept of an /// end value. This value will be the base value if the animation is /// in the first composition layer of animations on a property; /// otherwise this value will be the output value from the previous /// composition layer of animations for the property. /// /// /// This is the animationClock which can generate the CurrentTime or /// CurrentProgress value to be used by the animation to generate its /// output value. /// ////// The value this animation believes should be the current value for the property. /// public override sealed object GetCurrentValue(object defaultOriginValue, object defaultDestinationValue, AnimationClock animationClock) { return GetCurrentValue((String)defaultOriginValue, (String)defaultDestinationValue, animationClock); } ////// Returns the type of the target property /// public override sealed Type TargetPropertyType { get { ReadPreamble(); return typeof(String); } } #endregion #region Methods ////// Calculates the value this animation believes should be the current value for the property. /// /// /// This value is the suggested origin value provided to the animation /// to be used if the animation does not have its own concept of a /// start value. If this animation is the first in a composition chain /// this value will be the snapshot value if one is available or the /// base property value if it is not; otherise this value will be the /// value returned by the previous animation in the chain with an /// animationClock that is not Stopped. /// /// /// This value is the suggested destination value provided to the animation /// to be used if the animation does not have its own concept of an /// end value. This value will be the base value if the animation is /// in the first composition layer of animations on a property; /// otherwise this value will be the output value from the previous /// composition layer of animations for the property. /// /// /// This is the animationClock which can generate the CurrentTime or /// CurrentProgress value to be used by the animation to generate its /// output value. /// ////// The value this animation believes should be the current value for the property. /// public String GetCurrentValue(String defaultOriginValue, String defaultDestinationValue, AnimationClock animationClock) { ReadPreamble(); if (animationClock == null) { throw new ArgumentNullException("animationClock"); } // We check for null above but presharp doesn't notice so we suppress the // warning here. #pragma warning suppress 6506 if (animationClock.CurrentState == ClockState.Stopped) { return defaultDestinationValue; } /* if (!AnimatedTypeHelpers.IsValidAnimationValueString(defaultDestinationValue)) { throw new ArgumentException( SR.Get( SRID.Animation_InvalidBaseValue, defaultDestinationValue, defaultDestinationValue.GetType(), GetType()), "defaultDestinationValue"); } */ return GetCurrentValueCore(defaultOriginValue, defaultDestinationValue, animationClock); } ////// Calculates the value this animation believes should be the current value for the property. /// /// /// This value is the suggested origin value provided to the animation /// to be used if the animation does not have its own concept of a /// start value. If this animation is the first in a composition chain /// this value will be the snapshot value if one is available or the /// base property value if it is not; otherise this value will be the /// value returned by the previous animation in the chain with an /// animationClock that is not Stopped. /// /// /// This value is the suggested destination value provided to the animation /// to be used if the animation does not have its own concept of an /// end value. This value will be the base value if the animation is /// in the first composition layer of animations on a property; /// otherwise this value will be the output value from the previous /// composition layer of animations for the property. /// /// /// This is the animationClock which can generate the CurrentTime or /// CurrentProgress value to be used by the animation to generate its /// output value. /// ////// The value this animation believes should be the current value for the property. /// protected abstract String GetCurrentValueCore(String defaultOriginValue, String defaultDestinationValue, AnimationClock animationClock); #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
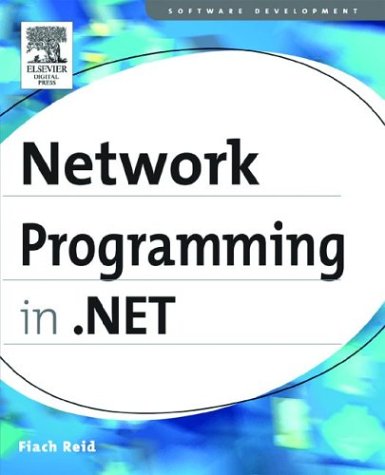
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Simplifier.cs
- DataGridAutoFormat.cs
- DocumentGrid.cs
- CompositeActivityDesigner.cs
- Guid.cs
- DbDataReader.cs
- TransactionFlowAttribute.cs
- Material.cs
- IdnMapping.cs
- ConstantExpression.cs
- DataTableNewRowEvent.cs
- filewebrequest.cs
- input.cs
- WCFBuildProvider.cs
- SqlCacheDependency.cs
- TextHidden.cs
- QilSortKey.cs
- HtmlInputText.cs
- _RequestLifetimeSetter.cs
- CodeSnippetTypeMember.cs
- CommandTreeTypeHelper.cs
- SafeFindHandle.cs
- IncomingWebResponseContext.cs
- EdmItemError.cs
- AsmxEndpointPickerExtension.cs
- DataGridViewAutoSizeModeEventArgs.cs
- PathFigure.cs
- XmlDocumentType.cs
- SqlSupersetValidator.cs
- SettingsProperty.cs
- SamlAttributeStatement.cs
- Image.cs
- AdRotatorDesigner.cs
- CollectionChange.cs
- Run.cs
- Cell.cs
- DefaultTraceListener.cs
- odbcmetadatacollectionnames.cs
- DataGridViewRowStateChangedEventArgs.cs
- CompareValidator.cs
- TypeConverterValueSerializer.cs
- ToolBarButtonClickEvent.cs
- ThicknessConverter.cs
- QueryCreatedEventArgs.cs
- BuildProviderCollection.cs
- DataTableClearEvent.cs
- TypeRefElement.cs
- TypeToken.cs
- CollectionConverter.cs
- OracleTransaction.cs
- CellTreeNode.cs
- SqlExpander.cs
- OletxResourceManager.cs
- CodeDesigner.cs
- SchemaElement.cs
- WebPartVerbsEventArgs.cs
- UdpDuplexChannel.cs
- TerminatorSinks.cs
- StylusOverProperty.cs
- ProgressiveCrcCalculatingStream.cs
- FixedElement.cs
- _KerberosClient.cs
- BidOverLoads.cs
- ClrProviderManifest.cs
- TypeDescriptionProvider.cs
- PositiveTimeSpanValidatorAttribute.cs
- TrustExchangeException.cs
- TypefaceCollection.cs
- XmlnsCompatibleWithAttribute.cs
- ContentElement.cs
- MouseActionConverter.cs
- CodePageUtils.cs
- WeakReadOnlyCollection.cs
- RequestSecurityToken.cs
- COM2PropertyPageUITypeConverter.cs
- WebPartsPersonalization.cs
- FloatUtil.cs
- IdentityHolder.cs
- Parser.cs
- SettingsPropertyValue.cs
- EntityUtil.cs
- BitmapSourceSafeMILHandle.cs
- PreservationFileWriter.cs
- TileModeValidation.cs
- NoPersistProperty.cs
- ReverseInheritProperty.cs
- TypeConverterAttribute.cs
- securitycriticaldataformultiplegetandset.cs
- SQLGuid.cs
- AttachedAnnotationChangedEventArgs.cs
- MappingMetadataHelper.cs
- OdbcEnvironment.cs
- TreeView.cs
- XmlSerializationGeneratedCode.cs
- AutomationPattern.cs
- AutomationPropertyInfo.cs
- WebPartTransformerCollection.cs
- validation.cs
- BufferedGraphicsContext.cs
- WebPartDisplayMode.cs