Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / ndp / fx / src / DataEntity / System / Data / Map / ViewGeneration / SignatureGenerator.cs / 2 / SignatureGenerator.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.Data.Common; using System.Data.Common.Utils; using System.Data.Mapping.ViewGeneration.Structures; using System.Collections.Generic; using System.Text; using System.Diagnostics; using System.Data.Metadata.Edm; namespace System.Data.Mapping.ViewGeneration { using AttributeSet = Set; // This class is responsible for managing various multi-constants that // are used in the cell normalization process. It handles their // generation and usage along with the generating of the attributes that // are needed with each multiconstant, i.e., it generates a signature for // each multiconstant internal class SignatureGenerator : InternalBase { #region Constructor // effects: Given an extent and the different condition members // (e.g., isHighpriority, discriminator) that are used in the cells // for this extent, creates a SignatureGenerator object that is // capable of giving the signatures for the cells internal SignatureGenerator(EntitySetBase extent, MetadataWorkspace workspace) { m_workspace = workspace; // We generate the indices for the projected slots as we traverse the metadata m_projectedSlotMap = new MemberPathMap(); // Determine the different values that various types inside // Extent can take can take and compute their cross product to // obtain the set of multiconstants for this extent, // e.g. Person-Address, Customer-Address-Phone (if only customers // have phones) MemberPath extentMember = new MemberPath(extent, workspace); GatherPartialSignature(extentMember, false, // not nullable false); // need not only keys } #endregion #region Fields // Tracks mapping of members of the left side to their corresponding // projected slots (for the aligned right query in a cell wrapper, // the fields are stored in the order specified by this map) private MemberPathMap m_projectedSlotMap; private MetadataWorkspace m_workspace; #endregion #region Properties // effects: Returns a map that maps member paths of the extent (for // which a view is being generated) to a dense space of numbers from // 0 such that each member's number is larger than it's parent's // number, e.g., if Person.Address.Zip has 5 then Person.Address is // less than 5. Say if it is 3 then Person is less than 3. internal MemberPathMapBase ProjectedSlotMap { get { return m_projectedSlotMap; } } #endregion #region Methods // requires: member is an Entity or Complex or Relation type // effects: Starting at "member", generates the multiconstant signatures for // all the fields embedded in it. isNullable indicates if member can // be nulled or not. needKeysOnly indicates whether we need to only // collect members that are keys. private void GatherPartialSignature(MemberPath member, bool isNullable, bool needKeysOnly) { EdmType memberType = member.EdmType; ComplexType complexTypemember = memberType as ComplexType; Debug.Assert(complexTypemember !=null || memberType is EntityType || // for entity sets memberType is AssociationType || // For relation sets memberType is RefType, // for relation ends "GatherPartialSignature only for complex types, entity sets, relationship ends"); if (memberType is ComplexType && needKeysOnly) { // CHANGE_[....]_FEATURE_COMPLEX_TYPE_KEYS // Check if the complex type needs to be traversed or not. If not, just return // from here. Else we need to continue to the code below. Right now, we do not // allow keys inside complex types return; } // Make sure that this member is in the slot map before any of // its embedded objects m_projectedSlotMap.CreateIndex(member); // Add the types can member have, i.e., its type and its subtypes List possibleTypes = new List (); possibleTypes.AddRange(MetadataHelper.GetTypeAndSubtypesOf(memberType, m_workspace, false /*includeAbstractTypes*/)); // Consider each possible type value -- each type value // conributes to a tuple in the result. For that possible type, // add all the type members into the signature foreach (EdmType possibleType in possibleTypes) { // first, collect all scalar-typed members of this type in a signature GatherSignatureFromScalars(member, possibleType, needKeysOnly); // Now drill down into complex-valued members and get a cross // product of the existing signatures // CHANGE_[....]_FEATURE_COMPLEX_KEYS: Need to handle getting key fields from complex types GatherSignaturesFromNonScalars(member, possibleType, needKeysOnly); } } // requires: possibleType is the member's type or one of its subtypes // possibleType is a StructuralType // effects: Given a member and the corresponding possible type // that it can take, determine the attributes that are relevant // for this possibleType and returns a multiconstant signature // corresponding to this possibleType and the attributes. If only // keys are needed, collect the key fields only. memberSlotId // indicates the slot number allocated for member in the multiconstant private void GatherSignatureFromScalars(MemberPath member, EdmType possibleType, bool needKeysOnly) { // For each child member of this type, collect all the relevant scalar fields StructuralType structuralType = (StructuralType) possibleType;; foreach (EdmMember childMetadata in structuralType.Members) { if (!Helper.IsEdmProperty(childMetadata)) { // We only care about properties. Associations Ends are // captured in NonScalars really continue; } EdmProperty childProperty = (EdmProperty)childMetadata; // Collect all scalar-typed members of this type // if needKeysOnly, collect only keys if (MetadataHelper.IsNonRefSimpleMember(childProperty) && (!needKeysOnly || MetadataHelper.IsPartOfEntityTypeKey(childProperty))) { MemberPath scalarMember = new MemberPath(member, childMetadata); // Note: scalarMember's parent has already been added to the projectedSlotMap m_projectedSlotMap.CreateIndex(scalarMember); } } } // requires: possibleType is the member's type or one of its subtypes // effects: Given a member and the corresponding possible type that // it can be, computes a cross-product of initial signature with the // different types that can occur inside possibleType private void GatherSignaturesFromNonScalars(MemberPath member, EdmType possibleType, bool needKeysOnly) { int childNumber = 0; // Go through all the members for the complex type while ignoring scalars foreach (EdmMember childMember in Helper.GetAllStructuralMembers(possibleType)) { childNumber++; if (MetadataHelper.IsNonRefSimpleMember(childMember)) { continue; } // CHANGE_[....]_FEATURE_COMPOSITION Fix as composition changes in metadata Debug.Assert(childMember.TypeUsage.EdmType is ComplexType || childMember.TypeUsage.EdmType is RefType, // for relation ends "Only non-scalars currently handled -- complex types, relationship ends"); // RefTypes are not nullable bool isNullable = MetadataHelper.IsMemberNullable(childMember); // Only keys are required for entities hanging off relation ends of an association // or for the first end of composition needKeysOnly = needKeysOnly || (childMember is AssociationEndMember); // CHANGE_[....]_FEATURE_COMPOSITION // (childNumber == 0 || false == ((RelationType)possibleType).IsComposition); MemberPath nonScalarMember = new MemberPath(member, childMember); GatherPartialSignature(nonScalarMember, isNullable, needKeysOnly); } } #endregion #region String methods internal override void ToCompactString(StringBuilder builder) { builder.Append("Projected Slot Map: "); m_projectedSlotMap.ToCompactString(builder); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.Data.Common; using System.Data.Common.Utils; using System.Data.Mapping.ViewGeneration.Structures; using System.Collections.Generic; using System.Text; using System.Diagnostics; using System.Data.Metadata.Edm; namespace System.Data.Mapping.ViewGeneration { using AttributeSet = Set; // This class is responsible for managing various multi-constants that // are used in the cell normalization process. It handles their // generation and usage along with the generating of the attributes that // are needed with each multiconstant, i.e., it generates a signature for // each multiconstant internal class SignatureGenerator : InternalBase { #region Constructor // effects: Given an extent and the different condition members // (e.g., isHighpriority, discriminator) that are used in the cells // for this extent, creates a SignatureGenerator object that is // capable of giving the signatures for the cells internal SignatureGenerator(EntitySetBase extent, MetadataWorkspace workspace) { m_workspace = workspace; // We generate the indices for the projected slots as we traverse the metadata m_projectedSlotMap = new MemberPathMap(); // Determine the different values that various types inside // Extent can take can take and compute their cross product to // obtain the set of multiconstants for this extent, // e.g. Person-Address, Customer-Address-Phone (if only customers // have phones) MemberPath extentMember = new MemberPath(extent, workspace); GatherPartialSignature(extentMember, false, // not nullable false); // need not only keys } #endregion #region Fields // Tracks mapping of members of the left side to their corresponding // projected slots (for the aligned right query in a cell wrapper, // the fields are stored in the order specified by this map) private MemberPathMap m_projectedSlotMap; private MetadataWorkspace m_workspace; #endregion #region Properties // effects: Returns a map that maps member paths of the extent (for // which a view is being generated) to a dense space of numbers from // 0 such that each member's number is larger than it's parent's // number, e.g., if Person.Address.Zip has 5 then Person.Address is // less than 5. Say if it is 3 then Person is less than 3. internal MemberPathMapBase ProjectedSlotMap { get { return m_projectedSlotMap; } } #endregion #region Methods // requires: member is an Entity or Complex or Relation type // effects: Starting at "member", generates the multiconstant signatures for // all the fields embedded in it. isNullable indicates if member can // be nulled or not. needKeysOnly indicates whether we need to only // collect members that are keys. private void GatherPartialSignature(MemberPath member, bool isNullable, bool needKeysOnly) { EdmType memberType = member.EdmType; ComplexType complexTypemember = memberType as ComplexType; Debug.Assert(complexTypemember !=null || memberType is EntityType || // for entity sets memberType is AssociationType || // For relation sets memberType is RefType, // for relation ends "GatherPartialSignature only for complex types, entity sets, relationship ends"); if (memberType is ComplexType && needKeysOnly) { // CHANGE_[....]_FEATURE_COMPLEX_TYPE_KEYS // Check if the complex type needs to be traversed or not. If not, just return // from here. Else we need to continue to the code below. Right now, we do not // allow keys inside complex types return; } // Make sure that this member is in the slot map before any of // its embedded objects m_projectedSlotMap.CreateIndex(member); // Add the types can member have, i.e., its type and its subtypes List possibleTypes = new List (); possibleTypes.AddRange(MetadataHelper.GetTypeAndSubtypesOf(memberType, m_workspace, false /*includeAbstractTypes*/)); // Consider each possible type value -- each type value // conributes to a tuple in the result. For that possible type, // add all the type members into the signature foreach (EdmType possibleType in possibleTypes) { // first, collect all scalar-typed members of this type in a signature GatherSignatureFromScalars(member, possibleType, needKeysOnly); // Now drill down into complex-valued members and get a cross // product of the existing signatures // CHANGE_[....]_FEATURE_COMPLEX_KEYS: Need to handle getting key fields from complex types GatherSignaturesFromNonScalars(member, possibleType, needKeysOnly); } } // requires: possibleType is the member's type or one of its subtypes // possibleType is a StructuralType // effects: Given a member and the corresponding possible type // that it can take, determine the attributes that are relevant // for this possibleType and returns a multiconstant signature // corresponding to this possibleType and the attributes. If only // keys are needed, collect the key fields only. memberSlotId // indicates the slot number allocated for member in the multiconstant private void GatherSignatureFromScalars(MemberPath member, EdmType possibleType, bool needKeysOnly) { // For each child member of this type, collect all the relevant scalar fields StructuralType structuralType = (StructuralType) possibleType;; foreach (EdmMember childMetadata in structuralType.Members) { if (!Helper.IsEdmProperty(childMetadata)) { // We only care about properties. Associations Ends are // captured in NonScalars really continue; } EdmProperty childProperty = (EdmProperty)childMetadata; // Collect all scalar-typed members of this type // if needKeysOnly, collect only keys if (MetadataHelper.IsNonRefSimpleMember(childProperty) && (!needKeysOnly || MetadataHelper.IsPartOfEntityTypeKey(childProperty))) { MemberPath scalarMember = new MemberPath(member, childMetadata); // Note: scalarMember's parent has already been added to the projectedSlotMap m_projectedSlotMap.CreateIndex(scalarMember); } } } // requires: possibleType is the member's type or one of its subtypes // effects: Given a member and the corresponding possible type that // it can be, computes a cross-product of initial signature with the // different types that can occur inside possibleType private void GatherSignaturesFromNonScalars(MemberPath member, EdmType possibleType, bool needKeysOnly) { int childNumber = 0; // Go through all the members for the complex type while ignoring scalars foreach (EdmMember childMember in Helper.GetAllStructuralMembers(possibleType)) { childNumber++; if (MetadataHelper.IsNonRefSimpleMember(childMember)) { continue; } // CHANGE_[....]_FEATURE_COMPOSITION Fix as composition changes in metadata Debug.Assert(childMember.TypeUsage.EdmType is ComplexType || childMember.TypeUsage.EdmType is RefType, // for relation ends "Only non-scalars currently handled -- complex types, relationship ends"); // RefTypes are not nullable bool isNullable = MetadataHelper.IsMemberNullable(childMember); // Only keys are required for entities hanging off relation ends of an association // or for the first end of composition needKeysOnly = needKeysOnly || (childMember is AssociationEndMember); // CHANGE_[....]_FEATURE_COMPOSITION // (childNumber == 0 || false == ((RelationType)possibleType).IsComposition); MemberPath nonScalarMember = new MemberPath(member, childMember); GatherPartialSignature(nonScalarMember, isNullable, needKeysOnly); } } #endregion #region String methods internal override void ToCompactString(StringBuilder builder) { builder.Append("Projected Slot Map: "); m_projectedSlotMap.ToCompactString(builder); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
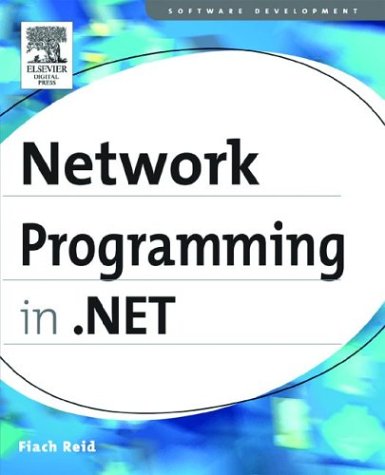
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- StyleCollection.cs
- ArgIterator.cs
- SqlFunctionAttribute.cs
- XhtmlConformanceSection.cs
- TextEndOfParagraph.cs
- AddInController.cs
- SQLDecimalStorage.cs
- Errors.cs
- DurableInstanceProvider.cs
- ClockController.cs
- KeysConverter.cs
- NeutralResourcesLanguageAttribute.cs
- GridLengthConverter.cs
- DockAndAnchorLayout.cs
- TargetConverter.cs
- ACL.cs
- Thumb.cs
- AstTree.cs
- ValueOfAction.cs
- SystemNetworkInterface.cs
- Int16KeyFrameCollection.cs
- BamlReader.cs
- RetrieveVirtualItemEventArgs.cs
- OperandQuery.cs
- SAPIEngineTypes.cs
- hebrewshape.cs
- BaseDataList.cs
- SerializationAttributes.cs
- DataGridAddNewRow.cs
- DataGridTablesFactory.cs
- controlskin.cs
- Choices.cs
- coordinatorscratchpad.cs
- WindowsStartMenu.cs
- ProcessRequestArgs.cs
- BuildProvider.cs
- IsolatedStorageFileStream.cs
- iisPickupDirectory.cs
- AttributeQuery.cs
- DataRelationCollection.cs
- GridProviderWrapper.cs
- Margins.cs
- TakeOrSkipWhileQueryOperator.cs
- DataReceivedEventArgs.cs
- ColumnResizeAdorner.cs
- SqlBulkCopyColumnMappingCollection.cs
- EncryptedPackageFilter.cs
- Metafile.cs
- HMACSHA1.cs
- ProjectionPruner.cs
- WebEventCodes.cs
- AutomationFocusChangedEventArgs.cs
- ListItem.cs
- ISFClipboardData.cs
- XmlElementAttributes.cs
- StringReader.cs
- OracleEncoding.cs
- ConfigurationFileMap.cs
- ExpressionBuilderContext.cs
- XamlBrushSerializer.cs
- TypedTableHandler.cs
- DetailsViewInsertEventArgs.cs
- ListBoxItemAutomationPeer.cs
- SQLChars.cs
- DragEvent.cs
- XmlDomTextWriter.cs
- FlowLayoutSettings.cs
- AuthenticationManager.cs
- SQLInt16Storage.cs
- ReflectionPermission.cs
- XmlSerializerAssemblyAttribute.cs
- BamlLocalizableResource.cs
- HtmlInputButton.cs
- SpnegoTokenProvider.cs
- _DigestClient.cs
- DbReferenceCollection.cs
- RootCodeDomSerializer.cs
- RpcCryptoContext.cs
- SqlSupersetValidator.cs
- XmlEntityReference.cs
- EpmContentDeSerializerBase.cs
- JavaScriptSerializer.cs
- AspCompat.cs
- HtmlLiteralTextAdapter.cs
- ScrollProperties.cs
- ElementsClipboardData.cs
- PrintDialog.cs
- ScalarOps.cs
- AddInContractAttribute.cs
- BindStream.cs
- NetNamedPipeBinding.cs
- DocumentXmlWriter.cs
- CodeMemberEvent.cs
- DesignTimeSiteMapProvider.cs
- _NestedMultipleAsyncResult.cs
- DeferredReference.cs
- BulletedListEventArgs.cs
- CheckBoxField.cs
- WindowsPen.cs
- DropDownList.cs