Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / AccessibleTech / longhorn / Automation / UIAutomationClient / System / Windows / Automation / PropertyCondition.cs / 1 / PropertyCondition.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: // // History: // 10/14/2003 : [....] - Created // //--------------------------------------------------------------------------- using System; using System.Globalization; using System.Windows.Automation; using MS.Internal.Automation; namespace System.Windows.Automation { ////// Flags that affect how a property value is compared in a PropertyCondition /// [Flags] #if (INTERNAL_COMPILE) internal enum PropertyConditionFlags #else public enum PropertyConditionFlags #endif { ///Properties are to be compared using default options (eg. case-sensitive comparison for strings) None = 0x00, ///For string comparisons, specifies that a case-insensitive comparison should be used IgnoreCase = 0x01, } ////// Condition that checks whether a property has the specified value /// #if (INTERNAL_COMPILE) internal class PropertyCondition : Condition #else public class PropertyCondition : Condition #endif { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors ////// Constructor to create a condition that checks whether a property has the specified value /// /// The property to check /// The value to check the property for public PropertyCondition( AutomationProperty property, object value ) { Init(property, value, PropertyConditionFlags.None); } ////// Constructor to create a condition that checks whether a property has the specified value /// /// The property to check /// The value to check the property for /// Flags that affect the comparison public PropertyCondition( AutomationProperty property, object value, PropertyConditionFlags flags ) { Init(property, value, flags); } #endregion Constructors //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- #region Public Properties ////// Returns the property that this condition is checking for /// public AutomationProperty Property { get { return _property; } } ////// Returns the value of the property that this condition is checking for /// public object Value { get { return _val; } } ////// Returns the flags used in this property comparison /// public PropertyConditionFlags Flags { get { return _flags; } } #endregion Public Properties //------------------------------------------------------ // // Private Methods // //------------------------------------------------------ #region Private Methods void Init(AutomationProperty property, object val, PropertyConditionFlags flags ) { Misc.ValidateArgumentNonNull(property, "property"); AutomationPropertyInfo info; if (!Schema.GetPropertyInfo(property, out info)) { throw new ArgumentException(SR.Get(SRID.UnsupportedProperty)); } // Check type is appropriate: NotSupported is allowed against any property, // null is allowed for any reference type (ie not for value types), otherwise // type must be assignable from expected type. Type expectedType = info.Type; if (val != AutomationElement.NotSupported && ((val == null && expectedType.IsValueType) || (val != null && !expectedType.IsAssignableFrom(val.GetType())))) { throw new ArgumentException(SR.Get(SRID.PropertyConditionIncorrectType, property.ProgrammaticName, expectedType.Name)); } if ((flags & PropertyConditionFlags.IgnoreCase) != 0) { Misc.ValidateArgument(val is string, SRID.IgnoreCaseRequiresString); } // Some types are handled differently in managed vs unmanaged - handle those here... if (val is AutomationElement) { // If this is a comparison against a Raw/LogicalElement, // save the runtime ID instead of the element so that we // can take it cross-proc if needed. val = ((AutomationElement)val).GetRuntimeId(); } else if (val is ControlType) { // If this is a control type, use the ID, not the CLR object val = ((ControlType)val).Id; } else if (val is Rect) { Rect rc = (Rect)val; val = new double[] { rc.Left, rc.Top, rc.Width, rc.Height }; } else if (val is Point) { Point pt = (Point)val; val = new double[] { pt.X, pt.Y }; } else if (val is CultureInfo) { val = ((CultureInfo)val).LCID; } _property = property; _val = val; _flags = flags; SetMarshalData(new UiaCoreApi.UiaPropertyCondition(_property.Id, _val, _flags)); } #endregion Private Methods //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ #region Private Fields private AutomationProperty _property; private object _val; private PropertyConditionFlags _flags; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
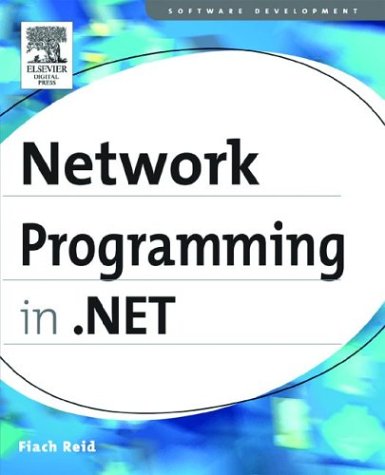
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- RuleInfoComparer.cs
- NativeRecognizer.cs
- HuffmanTree.cs
- ModuleConfigurationInfo.cs
- DesignerObjectListAdapter.cs
- EdmType.cs
- Imaging.cs
- Command.cs
- XmlMapping.cs
- IxmlLineInfo.cs
- SwitchElementsCollection.cs
- WebPartCloseVerb.cs
- DataPagerCommandEventArgs.cs
- COMException.cs
- XamlSerializerUtil.cs
- SiteMembershipCondition.cs
- EntityDataSourceViewSchema.cs
- ConversionValidationRule.cs
- OutputCacheEntry.cs
- LingerOption.cs
- InfoCardAsymmetricCrypto.cs
- ConfigXmlText.cs
- PropertyTab.cs
- recordstatefactory.cs
- Unit.cs
- QueryInterceptorAttribute.cs
- InfoCardTrace.cs
- NativeMethodsOther.cs
- ExtensionSimplifierMarkupObject.cs
- CodeExpressionRuleDeclaration.cs
- ComponentCollection.cs
- ControlAdapter.cs
- SiteMapNodeItemEventArgs.cs
- InputScopeAttribute.cs
- UnauthorizedAccessException.cs
- EventLogEntry.cs
- SystemThemeKey.cs
- TimeSpanStorage.cs
- assertwrapper.cs
- DurableErrorHandler.cs
- ControlAdapter.cs
- TypeDelegator.cs
- AttributeCollection.cs
- MessageRpc.cs
- BindingList.cs
- RoutedEvent.cs
- SqlCrossApplyToCrossJoin.cs
- Separator.cs
- XmlSchemaSequence.cs
- XappLauncher.cs
- BamlRecordHelper.cs
- SettingsPropertyCollection.cs
- PasswordBox.cs
- PrivilegedConfigurationManager.cs
- ProgressBarAutomationPeer.cs
- XNodeSchemaApplier.cs
- _DomainName.cs
- MembershipValidatePasswordEventArgs.cs
- Bezier.cs
- HandlerBase.cs
- CqlLexer.cs
- ApplicationSettingsBase.cs
- WebBrowserHelper.cs
- NavigationFailedEventArgs.cs
- StringKeyFrameCollection.cs
- SqlDataSourceCustomCommandEditor.cs
- PolyQuadraticBezierSegment.cs
- WsdlBuildProvider.cs
- QilXmlReader.cs
- DataListItem.cs
- FontStyleConverter.cs
- WindowsSpinner.cs
- UriExt.cs
- DSASignatureFormatter.cs
- Stacktrace.cs
- CatalogPartCollection.cs
- HMACRIPEMD160.cs
- PropertyEmitterBase.cs
- MultiPropertyDescriptorGridEntry.cs
- XmlParserContext.cs
- WebPartChrome.cs
- SqlMethods.cs
- HeaderCollection.cs
- RawStylusInputReport.cs
- DocumentReference.cs
- GeneralTransform2DTo3D.cs
- NominalTypeEliminator.cs
- ParserHooks.cs
- ExtenderProvidedPropertyAttribute.cs
- _ListenerAsyncResult.cs
- MultiPropertyDescriptorGridEntry.cs
- SchemaTableOptionalColumn.cs
- EventBuilder.cs
- ClientBuildManager.cs
- Activator.cs
- ToolStripRendererSwitcher.cs
- IdentityNotMappedException.cs
- infer.cs
- linebase.cs
- ResourceDisplayNameAttribute.cs