Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Core / CSharp / System / Windows / Media / Animation / Generated / StringKeyFrameCollection.cs / 1 / StringKeyFrameCollection.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // Please see http://wiki/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- using MS.Internal; using System; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.Diagnostics; using System.Globalization; using System.Windows.Media.Animation; using System.Windows.Media.Media3D; namespace System.Windows.Media.Animation { ////// This collection is used in conjunction with a KeyFrameStringAnimation /// to animate a String property value along a set of key frames. /// public class StringKeyFrameCollection : Freezable, IList { #region Data private List_keyFrames; private static StringKeyFrameCollection s_emptyCollection; #endregion #region Constructors /// /// Creates a new StringKeyFrameCollection. /// public StringKeyFrameCollection() : base() { _keyFrames = new List< StringKeyFrame>(2); } #endregion #region Static Methods ////// An empty StringKeyFrameCollection. /// public static StringKeyFrameCollection Empty { get { if (s_emptyCollection == null) { StringKeyFrameCollection emptyCollection = new StringKeyFrameCollection(); emptyCollection._keyFrames = new List< StringKeyFrame>(0); emptyCollection.Freeze(); s_emptyCollection = emptyCollection; } return s_emptyCollection; } } #endregion #region Freezable ////// Creates a freezable copy of this StringKeyFrameCollection. /// ///The copy public new StringKeyFrameCollection Clone() { return (StringKeyFrameCollection)base.Clone(); } ////// Implementation of ///Freezable.CreateInstanceCore . ///The new Freezable. protected override Freezable CreateInstanceCore() { return new StringKeyFrameCollection(); } ////// Implementation of protected override void CloneCore(Freezable sourceFreezable) { StringKeyFrameCollection sourceCollection = (StringKeyFrameCollection) sourceFreezable; base.CloneCore(sourceFreezable); int count = sourceCollection._keyFrames.Count; _keyFrames = new List< StringKeyFrame>(count); for (int i = 0; i < count; i++) { StringKeyFrame keyFrame = (StringKeyFrame)sourceCollection._keyFrames[i].Clone(); _keyFrames.Add(keyFrame); OnFreezablePropertyChanged(null, keyFrame); } } ///Freezable.CloneCore . ////// Implementation of protected override void CloneCurrentValueCore(Freezable sourceFreezable) { StringKeyFrameCollection sourceCollection = (StringKeyFrameCollection) sourceFreezable; base.CloneCurrentValueCore(sourceFreezable); int count = sourceCollection._keyFrames.Count; _keyFrames = new List< StringKeyFrame>(count); for (int i = 0; i < count; i++) { StringKeyFrame keyFrame = (StringKeyFrame)sourceCollection._keyFrames[i].CloneCurrentValue(); _keyFrames.Add(keyFrame); OnFreezablePropertyChanged(null, keyFrame); } } ///Freezable.CloneCurrentValueCore . ////// Implementation of protected override void GetAsFrozenCore(Freezable sourceFreezable) { StringKeyFrameCollection sourceCollection = (StringKeyFrameCollection) sourceFreezable; base.GetAsFrozenCore(sourceFreezable); int count = sourceCollection._keyFrames.Count; _keyFrames = new List< StringKeyFrame>(count); for (int i = 0; i < count; i++) { StringKeyFrame keyFrame = (StringKeyFrame)sourceCollection._keyFrames[i].GetAsFrozen(); _keyFrames.Add(keyFrame); OnFreezablePropertyChanged(null, keyFrame); } } ///Freezable.GetAsFrozenCore . ////// Implementation of protected override void GetCurrentValueAsFrozenCore(Freezable sourceFreezable) { StringKeyFrameCollection sourceCollection = (StringKeyFrameCollection) sourceFreezable; base.GetCurrentValueAsFrozenCore(sourceFreezable); int count = sourceCollection._keyFrames.Count; _keyFrames = new List< StringKeyFrame>(count); for (int i = 0; i < count; i++) { StringKeyFrame keyFrame = (StringKeyFrame)sourceCollection._keyFrames[i].GetCurrentValueAsFrozen(); _keyFrames.Add(keyFrame); OnFreezablePropertyChanged(null, keyFrame); } } ///Freezable.GetCurrentValueAsFrozenCore . ////// /// protected override bool FreezeCore(bool isChecking) { bool canFreeze = base.FreezeCore(isChecking); for (int i = 0; i < _keyFrames.Count && canFreeze; i++) { canFreeze &= Freezable.Freeze(_keyFrames[i], isChecking); } return canFreeze; } #endregion #region IEnumerable ////// Returns an enumerator of the StringKeyFrames in the collection. /// public IEnumerator GetEnumerator() { ReadPreamble(); return _keyFrames.GetEnumerator(); } #endregion #region ICollection ////// Returns the number of StringKeyFrames in the collection. /// public int Count { get { ReadPreamble(); return _keyFrames.Count; } } ////// See public bool IsSynchronized { get { ReadPreamble(); return (IsFrozen || Dispatcher != null); } } ///ICollection.IsSynchronized . ////// See public object SyncRoot { get { ReadPreamble(); return ((ICollection)_keyFrames).SyncRoot; } } ///ICollection.SyncRoot . ////// Copies all of the StringKeyFrames in the collection to an /// array. /// void ICollection.CopyTo(Array array, int index) { ReadPreamble(); ((ICollection)_keyFrames).CopyTo(array, index); } ////// Copies all of the StringKeyFrames in the collection to an /// array of StringKeyFrames. /// public void CopyTo(StringKeyFrame[] array, int index) { ReadPreamble(); _keyFrames.CopyTo(array, index); } #endregion #region IList ////// Adds a StringKeyFrame to the collection. /// int IList.Add(object keyFrame) { return Add((StringKeyFrame)keyFrame); } ////// Adds a StringKeyFrame to the collection. /// public int Add(StringKeyFrame keyFrame) { if (keyFrame == null) { throw new ArgumentNullException("keyFrame"); } WritePreamble(); OnFreezablePropertyChanged(null, keyFrame); _keyFrames.Add(keyFrame); WritePostscript(); return _keyFrames.Count - 1; } ////// Removes all StringKeyFrames from the collection. /// public void Clear() { WritePreamble(); if (_keyFrames.Count > 0) { for (int i = 0; i < _keyFrames.Count; i++) { OnFreezablePropertyChanged(_keyFrames[i], null); } _keyFrames.Clear(); WritePostscript(); } } ////// Returns true of the collection contains the given StringKeyFrame. /// bool IList.Contains(object keyFrame) { return Contains((StringKeyFrame)keyFrame); } ////// Returns true of the collection contains the given StringKeyFrame. /// public bool Contains(StringKeyFrame keyFrame) { ReadPreamble(); return _keyFrames.Contains(keyFrame); } ////// Returns the index of a given StringKeyFrame in the collection. /// int IList.IndexOf(object keyFrame) { return IndexOf((StringKeyFrame)keyFrame); } ////// Returns the index of a given StringKeyFrame in the collection. /// public int IndexOf(StringKeyFrame keyFrame) { ReadPreamble(); return _keyFrames.IndexOf(keyFrame); } ////// Inserts a StringKeyFrame into a specific location in the collection. /// void IList.Insert(int index, object keyFrame) { Insert(index, (StringKeyFrame)keyFrame); } ////// Inserts a StringKeyFrame into a specific location in the collection. /// public void Insert(int index, StringKeyFrame keyFrame) { if (keyFrame == null) { throw new ArgumentNullException("keyFrame"); } WritePreamble(); OnFreezablePropertyChanged(null, keyFrame); _keyFrames.Insert(index, keyFrame); WritePostscript(); } ////// Returns true if the collection is frozen. /// public bool IsFixedSize { get { ReadPreamble(); return IsFrozen; } } ////// Returns true if the collection is frozen. /// public bool IsReadOnly { get { ReadPreamble(); return IsFrozen; } } ////// Removes a StringKeyFrame from the collection. /// void IList.Remove(object keyFrame) { Remove((StringKeyFrame)keyFrame); } ////// Removes a StringKeyFrame from the collection. /// public void Remove(StringKeyFrame keyFrame) { WritePreamble(); if (_keyFrames.Contains(keyFrame)) { OnFreezablePropertyChanged(keyFrame, null); _keyFrames.Remove(keyFrame); WritePostscript(); } } ////// Removes the StringKeyFrame at the specified index from the collection. /// public void RemoveAt(int index) { WritePreamble(); OnFreezablePropertyChanged(_keyFrames[index], null); _keyFrames.RemoveAt(index); WritePostscript(); } ////// Gets or sets the StringKeyFrame at a given index. /// object IList.this[int index] { get { return this[index]; } set { this[index] = (StringKeyFrame)value; } } ////// Gets or sets the StringKeyFrame at a given index. /// public StringKeyFrame this[int index] { get { ReadPreamble(); return _keyFrames[index]; } set { if (value == null) { throw new ArgumentNullException(String.Format(CultureInfo.InvariantCulture, "StringKeyFrameCollection[{0}]", index)); } WritePreamble(); if (value != _keyFrames[index]) { OnFreezablePropertyChanged(_keyFrames[index], value); _keyFrames[index] = value; Debug.Assert(_keyFrames[index] != null); WritePostscript(); } } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // Please see http://wiki/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- using MS.Internal; using System; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.Diagnostics; using System.Globalization; using System.Windows.Media.Animation; using System.Windows.Media.Media3D; namespace System.Windows.Media.Animation { ////// This collection is used in conjunction with a KeyFrameStringAnimation /// to animate a String property value along a set of key frames. /// public class StringKeyFrameCollection : Freezable, IList { #region Data private List_keyFrames; private static StringKeyFrameCollection s_emptyCollection; #endregion #region Constructors /// /// Creates a new StringKeyFrameCollection. /// public StringKeyFrameCollection() : base() { _keyFrames = new List< StringKeyFrame>(2); } #endregion #region Static Methods ////// An empty StringKeyFrameCollection. /// public static StringKeyFrameCollection Empty { get { if (s_emptyCollection == null) { StringKeyFrameCollection emptyCollection = new StringKeyFrameCollection(); emptyCollection._keyFrames = new List< StringKeyFrame>(0); emptyCollection.Freeze(); s_emptyCollection = emptyCollection; } return s_emptyCollection; } } #endregion #region Freezable ////// Creates a freezable copy of this StringKeyFrameCollection. /// ///The copy public new StringKeyFrameCollection Clone() { return (StringKeyFrameCollection)base.Clone(); } ////// Implementation of ///Freezable.CreateInstanceCore . ///The new Freezable. protected override Freezable CreateInstanceCore() { return new StringKeyFrameCollection(); } ////// Implementation of protected override void CloneCore(Freezable sourceFreezable) { StringKeyFrameCollection sourceCollection = (StringKeyFrameCollection) sourceFreezable; base.CloneCore(sourceFreezable); int count = sourceCollection._keyFrames.Count; _keyFrames = new List< StringKeyFrame>(count); for (int i = 0; i < count; i++) { StringKeyFrame keyFrame = (StringKeyFrame)sourceCollection._keyFrames[i].Clone(); _keyFrames.Add(keyFrame); OnFreezablePropertyChanged(null, keyFrame); } } ///Freezable.CloneCore . ////// Implementation of protected override void CloneCurrentValueCore(Freezable sourceFreezable) { StringKeyFrameCollection sourceCollection = (StringKeyFrameCollection) sourceFreezable; base.CloneCurrentValueCore(sourceFreezable); int count = sourceCollection._keyFrames.Count; _keyFrames = new List< StringKeyFrame>(count); for (int i = 0; i < count; i++) { StringKeyFrame keyFrame = (StringKeyFrame)sourceCollection._keyFrames[i].CloneCurrentValue(); _keyFrames.Add(keyFrame); OnFreezablePropertyChanged(null, keyFrame); } } ///Freezable.CloneCurrentValueCore . ////// Implementation of protected override void GetAsFrozenCore(Freezable sourceFreezable) { StringKeyFrameCollection sourceCollection = (StringKeyFrameCollection) sourceFreezable; base.GetAsFrozenCore(sourceFreezable); int count = sourceCollection._keyFrames.Count; _keyFrames = new List< StringKeyFrame>(count); for (int i = 0; i < count; i++) { StringKeyFrame keyFrame = (StringKeyFrame)sourceCollection._keyFrames[i].GetAsFrozen(); _keyFrames.Add(keyFrame); OnFreezablePropertyChanged(null, keyFrame); } } ///Freezable.GetAsFrozenCore . ////// Implementation of protected override void GetCurrentValueAsFrozenCore(Freezable sourceFreezable) { StringKeyFrameCollection sourceCollection = (StringKeyFrameCollection) sourceFreezable; base.GetCurrentValueAsFrozenCore(sourceFreezable); int count = sourceCollection._keyFrames.Count; _keyFrames = new List< StringKeyFrame>(count); for (int i = 0; i < count; i++) { StringKeyFrame keyFrame = (StringKeyFrame)sourceCollection._keyFrames[i].GetCurrentValueAsFrozen(); _keyFrames.Add(keyFrame); OnFreezablePropertyChanged(null, keyFrame); } } ///Freezable.GetCurrentValueAsFrozenCore . ////// /// protected override bool FreezeCore(bool isChecking) { bool canFreeze = base.FreezeCore(isChecking); for (int i = 0; i < _keyFrames.Count && canFreeze; i++) { canFreeze &= Freezable.Freeze(_keyFrames[i], isChecking); } return canFreeze; } #endregion #region IEnumerable ////// Returns an enumerator of the StringKeyFrames in the collection. /// public IEnumerator GetEnumerator() { ReadPreamble(); return _keyFrames.GetEnumerator(); } #endregion #region ICollection ////// Returns the number of StringKeyFrames in the collection. /// public int Count { get { ReadPreamble(); return _keyFrames.Count; } } ////// See public bool IsSynchronized { get { ReadPreamble(); return (IsFrozen || Dispatcher != null); } } ///ICollection.IsSynchronized . ////// See public object SyncRoot { get { ReadPreamble(); return ((ICollection)_keyFrames).SyncRoot; } } ///ICollection.SyncRoot . ////// Copies all of the StringKeyFrames in the collection to an /// array. /// void ICollection.CopyTo(Array array, int index) { ReadPreamble(); ((ICollection)_keyFrames).CopyTo(array, index); } ////// Copies all of the StringKeyFrames in the collection to an /// array of StringKeyFrames. /// public void CopyTo(StringKeyFrame[] array, int index) { ReadPreamble(); _keyFrames.CopyTo(array, index); } #endregion #region IList ////// Adds a StringKeyFrame to the collection. /// int IList.Add(object keyFrame) { return Add((StringKeyFrame)keyFrame); } ////// Adds a StringKeyFrame to the collection. /// public int Add(StringKeyFrame keyFrame) { if (keyFrame == null) { throw new ArgumentNullException("keyFrame"); } WritePreamble(); OnFreezablePropertyChanged(null, keyFrame); _keyFrames.Add(keyFrame); WritePostscript(); return _keyFrames.Count - 1; } ////// Removes all StringKeyFrames from the collection. /// public void Clear() { WritePreamble(); if (_keyFrames.Count > 0) { for (int i = 0; i < _keyFrames.Count; i++) { OnFreezablePropertyChanged(_keyFrames[i], null); } _keyFrames.Clear(); WritePostscript(); } } ////// Returns true of the collection contains the given StringKeyFrame. /// bool IList.Contains(object keyFrame) { return Contains((StringKeyFrame)keyFrame); } ////// Returns true of the collection contains the given StringKeyFrame. /// public bool Contains(StringKeyFrame keyFrame) { ReadPreamble(); return _keyFrames.Contains(keyFrame); } ////// Returns the index of a given StringKeyFrame in the collection. /// int IList.IndexOf(object keyFrame) { return IndexOf((StringKeyFrame)keyFrame); } ////// Returns the index of a given StringKeyFrame in the collection. /// public int IndexOf(StringKeyFrame keyFrame) { ReadPreamble(); return _keyFrames.IndexOf(keyFrame); } ////// Inserts a StringKeyFrame into a specific location in the collection. /// void IList.Insert(int index, object keyFrame) { Insert(index, (StringKeyFrame)keyFrame); } ////// Inserts a StringKeyFrame into a specific location in the collection. /// public void Insert(int index, StringKeyFrame keyFrame) { if (keyFrame == null) { throw new ArgumentNullException("keyFrame"); } WritePreamble(); OnFreezablePropertyChanged(null, keyFrame); _keyFrames.Insert(index, keyFrame); WritePostscript(); } ////// Returns true if the collection is frozen. /// public bool IsFixedSize { get { ReadPreamble(); return IsFrozen; } } ////// Returns true if the collection is frozen. /// public bool IsReadOnly { get { ReadPreamble(); return IsFrozen; } } ////// Removes a StringKeyFrame from the collection. /// void IList.Remove(object keyFrame) { Remove((StringKeyFrame)keyFrame); } ////// Removes a StringKeyFrame from the collection. /// public void Remove(StringKeyFrame keyFrame) { WritePreamble(); if (_keyFrames.Contains(keyFrame)) { OnFreezablePropertyChanged(keyFrame, null); _keyFrames.Remove(keyFrame); WritePostscript(); } } ////// Removes the StringKeyFrame at the specified index from the collection. /// public void RemoveAt(int index) { WritePreamble(); OnFreezablePropertyChanged(_keyFrames[index], null); _keyFrames.RemoveAt(index); WritePostscript(); } ////// Gets or sets the StringKeyFrame at a given index. /// object IList.this[int index] { get { return this[index]; } set { this[index] = (StringKeyFrame)value; } } ////// Gets or sets the StringKeyFrame at a given index. /// public StringKeyFrame this[int index] { get { ReadPreamble(); return _keyFrames[index]; } set { if (value == null) { throw new ArgumentNullException(String.Format(CultureInfo.InvariantCulture, "StringKeyFrameCollection[{0}]", index)); } WritePreamble(); if (value != _keyFrames[index]) { OnFreezablePropertyChanged(_keyFrames[index], value); _keyFrames[index] = value; Debug.Assert(_keyFrames[index] != null); WritePostscript(); } } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
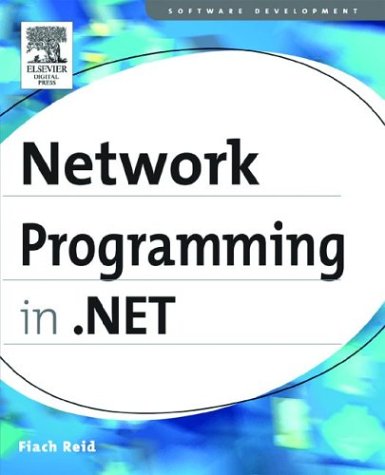
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- FillBehavior.cs
- SkewTransform.cs
- TextSpan.cs
- StylusDevice.cs
- Options.cs
- StrokeRenderer.cs
- DragCompletedEventArgs.cs
- XmlKeywords.cs
- ContextProperty.cs
- ScriptReferenceBase.cs
- EnumDataContract.cs
- SafeNativeMethodsMilCoreApi.cs
- StateDesigner.CommentLayoutGlyph.cs
- LoaderAllocator.cs
- Button.cs
- CalendarModeChangedEventArgs.cs
- BaseProcessor.cs
- CompositeFontInfo.cs
- PieceNameHelper.cs
- HttpConfigurationContext.cs
- SerialStream.cs
- DateTimeSerializationSection.cs
- BlockUIContainer.cs
- UIElement3D.cs
- x509utils.cs
- SQLMembershipProvider.cs
- Rfc2898DeriveBytes.cs
- ServerType.cs
- DataGridViewElement.cs
- RelationshipConverter.cs
- WmfPlaceableFileHeader.cs
- Vars.cs
- ConfigsHelper.cs
- UnlockInstanceCommand.cs
- CmsInterop.cs
- OdbcTransaction.cs
- ScriptReference.cs
- CompoundFileStreamReference.cs
- MaterialGroup.cs
- DistributedTransactionPermission.cs
- CompletedAsyncResult.cs
- TextContainerChangeEventArgs.cs
- DataGridToolTip.cs
- ActiveXMessageFormatter.cs
- WebRequestModuleElement.cs
- BuildManager.cs
- FieldMetadata.cs
- DataGridViewAutoSizeModeEventArgs.cs
- Separator.cs
- RegularExpressionValidator.cs
- XmlNamespaceManager.cs
- WebFormsRootDesigner.cs
- TextProperties.cs
- TimeSpanValidator.cs
- SafeHandles.cs
- BindingEditor.xaml.cs
- KeySplineConverter.cs
- DateTimeOffset.cs
- ConfigDefinitionUpdates.cs
- Helpers.cs
- ServiceOperationUIEditor.cs
- CodeTypeOfExpression.cs
- WindowsPen.cs
- SelectedGridItemChangedEvent.cs
- BaseComponentEditor.cs
- PathData.cs
- BaseParser.cs
- DataGridLinkButton.cs
- MemberInfoSerializationHolder.cs
- EmptyCollection.cs
- GridEntry.cs
- WebBrowserDesigner.cs
- WebBrowserContainer.cs
- PublishLicense.cs
- BackgroundWorker.cs
- Misc.cs
- EventLogEntry.cs
- SplayTreeNode.cs
- JournalEntry.cs
- LateBoundBitmapDecoder.cs
- TripleDESCryptoServiceProvider.cs
- HelpInfo.cs
- BitmapScalingModeValidation.cs
- ChainOfDependencies.cs
- EpmSyndicationContentSerializer.cs
- EpmSourceTree.cs
- MatcherBuilder.cs
- ImageCodecInfo.cs
- StrokeCollection2.cs
- GridViewActionList.cs
- RenderCapability.cs
- WebPartDescription.cs
- OdbcFactory.cs
- XmlText.cs
- Span.cs
- ConfigXmlWhitespace.cs
- MissingMethodException.cs
- DockPanel.cs
- OleServicesContext.cs
- RealizationContext.cs