Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / clr / src / BCL / System / Reflection / Emit / EventBuilder.cs / 1305376 / EventBuilder.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== /*============================================================ ** ** Class: EventBuilder ** **[....] ** ** ** Eventbuilder is for client to define eevnts for a class ** ** ===========================================================*/ namespace System.Reflection.Emit { using System; using System.Reflection; using System.Security.Permissions; using System.Runtime.InteropServices; using System.Diagnostics.Contracts; // // A EventBuilder is always associated with a TypeBuilder. The TypeBuilder.DefineEvent // method will return a new EventBuilder to a client. // [HostProtection(MayLeakOnAbort = true)] [ClassInterface(ClassInterfaceType.None)] [ComDefaultInterface(typeof(_EventBuilder))] [System.Runtime.InteropServices.ComVisible(true)] public sealed class EventBuilder : _EventBuilder { // Make a private constructor so these cannot be constructed externally. private EventBuilder() {} // Constructs a EventBuilder. // internal EventBuilder( ModuleBuilder mod, // the module containing this EventBuilder String name, // Event name EventAttributes attr, // event attribute such as Public, Private, and Protected defined above //int eventType, // event type TypeBuilder type, // containing type EventToken evToken) { m_name = name; m_module = mod; m_attributes = attr; m_evToken = evToken; m_type = type; } // Return the Token for this event within the TypeBuilder that the // event is defined within. public EventToken GetEventToken() { return m_evToken; } [System.Security.SecurityCritical] // auto-generated private void SetMethodSemantics(MethodBuilder mdBuilder, MethodSemanticsAttributes semantics) { if (mdBuilder == null) { throw new ArgumentNullException("mdBuilder"); } Contract.EndContractBlock(); m_type.ThrowIfCreated(); TypeBuilder.DefineMethodSemantics( m_module.GetNativeHandle(), m_evToken.Token, semantics, mdBuilder.GetToken().Token); } [System.Security.SecuritySafeCritical] // auto-generated public void SetAddOnMethod(MethodBuilder mdBuilder) { SetMethodSemantics(mdBuilder, MethodSemanticsAttributes.AddOn); } [System.Security.SecuritySafeCritical] // auto-generated public void SetRemoveOnMethod(MethodBuilder mdBuilder) { SetMethodSemantics(mdBuilder, MethodSemanticsAttributes.RemoveOn); } [System.Security.SecuritySafeCritical] // auto-generated public void SetRaiseMethod(MethodBuilder mdBuilder) { SetMethodSemantics(mdBuilder, MethodSemanticsAttributes.Fire); } [System.Security.SecuritySafeCritical] // auto-generated public void AddOtherMethod(MethodBuilder mdBuilder) { SetMethodSemantics(mdBuilder, MethodSemanticsAttributes.Other); } // Use this function if client decides to form the custom attribute blob themselves [System.Security.SecuritySafeCritical] // auto-generated [System.Runtime.InteropServices.ComVisible(true)] public void SetCustomAttribute(ConstructorInfo con, byte[] binaryAttribute) { if (con == null) throw new ArgumentNullException("con"); if (binaryAttribute == null) throw new ArgumentNullException("binaryAttribute"); Contract.EndContractBlock(); m_type.ThrowIfCreated(); TypeBuilder.DefineCustomAttribute( m_module, m_evToken.Token, m_module.GetConstructorToken(con).Token, binaryAttribute, false, false); } // Use this function if client wishes to build CustomAttribute using CustomAttributeBuilder [System.Security.SecuritySafeCritical] // auto-generated public void SetCustomAttribute(CustomAttributeBuilder customBuilder) { if (customBuilder == null) { throw new ArgumentNullException("customBuilder"); } Contract.EndContractBlock(); m_type.ThrowIfCreated(); customBuilder.CreateCustomAttribute(m_module, m_evToken.Token); } void _EventBuilder.GetTypeInfoCount(out uint pcTInfo) { throw new NotImplementedException(); } void _EventBuilder.GetTypeInfo(uint iTInfo, uint lcid, IntPtr ppTInfo) { throw new NotImplementedException(); } void _EventBuilder.GetIDsOfNames([In] ref Guid riid, IntPtr rgszNames, uint cNames, uint lcid, IntPtr rgDispId) { throw new NotImplementedException(); } void _EventBuilder.Invoke(uint dispIdMember, [In] ref Guid riid, uint lcid, short wFlags, IntPtr pDispParams, IntPtr pVarResult, IntPtr pExcepInfo, IntPtr puArgErr) { throw new NotImplementedException(); } // These are package private so that TypeBuilder can access them. private String m_name; // The name of the event private EventToken m_evToken; // The token of this event private ModuleBuilder m_module; private EventAttributes m_attributes; private TypeBuilder m_type; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== /*============================================================ ** ** Class: EventBuilder ** **[....] ** ** ** Eventbuilder is for client to define eevnts for a class ** ** ===========================================================*/ namespace System.Reflection.Emit { using System; using System.Reflection; using System.Security.Permissions; using System.Runtime.InteropServices; using System.Diagnostics.Contracts; // // A EventBuilder is always associated with a TypeBuilder. The TypeBuilder.DefineEvent // method will return a new EventBuilder to a client. // [HostProtection(MayLeakOnAbort = true)] [ClassInterface(ClassInterfaceType.None)] [ComDefaultInterface(typeof(_EventBuilder))] [System.Runtime.InteropServices.ComVisible(true)] public sealed class EventBuilder : _EventBuilder { // Make a private constructor so these cannot be constructed externally. private EventBuilder() {} // Constructs a EventBuilder. // internal EventBuilder( ModuleBuilder mod, // the module containing this EventBuilder String name, // Event name EventAttributes attr, // event attribute such as Public, Private, and Protected defined above //int eventType, // event type TypeBuilder type, // containing type EventToken evToken) { m_name = name; m_module = mod; m_attributes = attr; m_evToken = evToken; m_type = type; } // Return the Token for this event within the TypeBuilder that the // event is defined within. public EventToken GetEventToken() { return m_evToken; } [System.Security.SecurityCritical] // auto-generated private void SetMethodSemantics(MethodBuilder mdBuilder, MethodSemanticsAttributes semantics) { if (mdBuilder == null) { throw new ArgumentNullException("mdBuilder"); } Contract.EndContractBlock(); m_type.ThrowIfCreated(); TypeBuilder.DefineMethodSemantics( m_module.GetNativeHandle(), m_evToken.Token, semantics, mdBuilder.GetToken().Token); } [System.Security.SecuritySafeCritical] // auto-generated public void SetAddOnMethod(MethodBuilder mdBuilder) { SetMethodSemantics(mdBuilder, MethodSemanticsAttributes.AddOn); } [System.Security.SecuritySafeCritical] // auto-generated public void SetRemoveOnMethod(MethodBuilder mdBuilder) { SetMethodSemantics(mdBuilder, MethodSemanticsAttributes.RemoveOn); } [System.Security.SecuritySafeCritical] // auto-generated public void SetRaiseMethod(MethodBuilder mdBuilder) { SetMethodSemantics(mdBuilder, MethodSemanticsAttributes.Fire); } [System.Security.SecuritySafeCritical] // auto-generated public void AddOtherMethod(MethodBuilder mdBuilder) { SetMethodSemantics(mdBuilder, MethodSemanticsAttributes.Other); } // Use this function if client decides to form the custom attribute blob themselves [System.Security.SecuritySafeCritical] // auto-generated [System.Runtime.InteropServices.ComVisible(true)] public void SetCustomAttribute(ConstructorInfo con, byte[] binaryAttribute) { if (con == null) throw new ArgumentNullException("con"); if (binaryAttribute == null) throw new ArgumentNullException("binaryAttribute"); Contract.EndContractBlock(); m_type.ThrowIfCreated(); TypeBuilder.DefineCustomAttribute( m_module, m_evToken.Token, m_module.GetConstructorToken(con).Token, binaryAttribute, false, false); } // Use this function if client wishes to build CustomAttribute using CustomAttributeBuilder [System.Security.SecuritySafeCritical] // auto-generated public void SetCustomAttribute(CustomAttributeBuilder customBuilder) { if (customBuilder == null) { throw new ArgumentNullException("customBuilder"); } Contract.EndContractBlock(); m_type.ThrowIfCreated(); customBuilder.CreateCustomAttribute(m_module, m_evToken.Token); } void _EventBuilder.GetTypeInfoCount(out uint pcTInfo) { throw new NotImplementedException(); } void _EventBuilder.GetTypeInfo(uint iTInfo, uint lcid, IntPtr ppTInfo) { throw new NotImplementedException(); } void _EventBuilder.GetIDsOfNames([In] ref Guid riid, IntPtr rgszNames, uint cNames, uint lcid, IntPtr rgDispId) { throw new NotImplementedException(); } void _EventBuilder.Invoke(uint dispIdMember, [In] ref Guid riid, uint lcid, short wFlags, IntPtr pDispParams, IntPtr pVarResult, IntPtr pExcepInfo, IntPtr puArgErr) { throw new NotImplementedException(); } // These are package private so that TypeBuilder can access them. private String m_name; // The name of the event private EventToken m_evToken; // The token of this event private ModuleBuilder m_module; private EventAttributes m_attributes; private TypeBuilder m_type; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
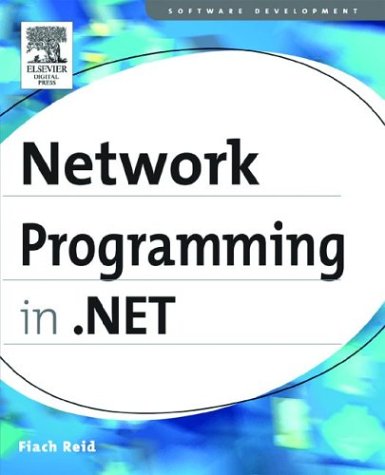
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ArcSegment.cs
- BinaryFormatter.cs
- ExpressionDumper.cs
- SubMenuStyle.cs
- ContractUtils.cs
- ZoneMembershipCondition.cs
- MailDefinition.cs
- HttpClientChannel.cs
- Int32Storage.cs
- webclient.cs
- GatewayIPAddressInformationCollection.cs
- DecimalConstantAttribute.cs
- NameValuePair.cs
- NativeMethods.cs
- Registry.cs
- ResXDataNode.cs
- DynamicILGenerator.cs
- StructuralType.cs
- ManagementException.cs
- WmpBitmapEncoder.cs
- EntityDataSourceView.cs
- BooleanToVisibilityConverter.cs
- PageContentAsyncResult.cs
- DependencyPropertyDescriptor.cs
- Separator.cs
- WinEventWrap.cs
- FixedTextPointer.cs
- DebugInfoExpression.cs
- SafeLibraryHandle.cs
- DiscoveryDocumentReference.cs
- PageSetupDialog.cs
- ProtocolsConfiguration.cs
- BypassElement.cs
- FloatMinMaxAggregationOperator.cs
- ConfigurationSectionCollection.cs
- SerialReceived.cs
- NavigationProperty.cs
- HighContrastHelper.cs
- XmlSchemaSimpleType.cs
- EntityDataSourceView.cs
- HttpStreamMessageEncoderFactory.cs
- TranslateTransform3D.cs
- SspiNegotiationTokenProvider.cs
- Content.cs
- HotSpotCollection.cs
- ProgressBarAutomationPeer.cs
- SystemFonts.cs
- SecurityPolicySection.cs
- Menu.cs
- StaticSiteMapProvider.cs
- DataSourceSelectArguments.cs
- ReadOnlyDataSourceView.cs
- BitmapEffectGeneralTransform.cs
- PeerTransportSecurityElement.cs
- ConfigXmlWhitespace.cs
- _KerberosClient.cs
- CodeObjectCreateExpression.cs
- AdRotator.cs
- DataReceivedEventArgs.cs
- FormViewPageEventArgs.cs
- WebHttpBinding.cs
- SmtpTransport.cs
- ValidationResult.cs
- DataServiceProcessingPipelineEventArgs.cs
- DataBinder.cs
- MetadataException.cs
- PopOutPanel.cs
- ObjectDataSourceMethodEventArgs.cs
- BinaryEditor.cs
- ToolStripScrollButton.cs
- DefaultProxySection.cs
- ContextProperty.cs
- ContentPresenter.cs
- ProgressBarAutomationPeer.cs
- BmpBitmapEncoder.cs
- OverrideMode.cs
- WebResourceAttribute.cs
- EntityDataSourceValidationException.cs
- RegisteredDisposeScript.cs
- Rights.cs
- CoTaskMemSafeHandle.cs
- FormViewUpdatedEventArgs.cs
- WebPartDisplayMode.cs
- HttpModuleCollection.cs
- ProcessModuleCollection.cs
- recordstatefactory.cs
- RowVisual.cs
- DoWorkEventArgs.cs
- InkSerializer.cs
- TrustLevel.cs
- QueryCursorEventArgs.cs
- ClonableStack.cs
- DesignOnlyAttribute.cs
- PrefixQName.cs
- Base64Decoder.cs
- CompensationToken.cs
- LeftCellWrapper.cs
- ValidationPropertyAttribute.cs
- DBCommandBuilder.cs
- PageHandlerFactory.cs