Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataWeb / Server / System / Data / Services / IgnorePropertiesAttribute.cs / 1305376 / IgnorePropertiesAttribute.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// CLR attribute to be annotated on types which indicate the list of properties // to ignore. // // // @owner [....] //--------------------------------------------------------------------- namespace System.Data.Services { using System; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Diagnostics; using System.Reflection; ///Attribute to be annotated on types with ETags. [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Design", "CA1019:DefineAccessorsForAttributeArguments", Justification = "Processed values are available.")] [AttributeUsage(AttributeTargets.Class, AllowMultiple = false, Inherited = true)] public sealed class IgnorePropertiesAttribute : System.Attribute { ///Name of the properties that form the ETag. private readonly ReadOnlyCollectionpropertyNames; // This constructor was added since string[] is not a CLS-compliant type and // compiler gives a warning as error saying this attribute doesn't have any // constructor that takes CLS-compliant type /// /// Initializes a new instance of IgnoreProperties attribute with the property name /// that needs to be ignored /// /// Name of the property that form the ETag for the current type. public IgnorePropertiesAttribute(string propertyName) { WebUtil.CheckArgumentNull(propertyName, "propertyName"); this.propertyNames = new ReadOnlyCollection(new List (new string[1] { propertyName })); } /// /// Initializes a new instance of IgnoreProperties attribute with the list of property names /// that need to be ignored /// /// Name of the properties that form the ETag for the current type. public IgnorePropertiesAttribute(params string[] propertyNames) { WebUtil.CheckArgumentNull(propertyNames, "propertyNames"); if (propertyNames.Length == 0) { throw new ArgumentException(Strings.ETagAttribute_MustSpecifyAtleastOnePropertyName, "propertyNames"); } this.propertyNames = new ReadOnlyCollection(new List (propertyNames)); } /// Name of the properties that needs to be ignored for the current type. public ReadOnlyCollectionPropertyNames { get { return this.propertyNames; } } /// /// Validate and get the list of properties specified by this attribute on the given type. /// /// clr type on which this attribute must have defined. /// whether we need to inherit this attribute or not. /// For context types,we need to, since we can have one context dervied from another, and we want to ignore all the properties on the base ones too. /// For resource types, we don't need to, since we don't want derived types to know about ignore properties of the base type. Also /// from derived type, you cannot change the definition of the base type. /// binding flags to be used for validating property names. ///list of property names specified on IgnoreProperties on the given type. internal static IEnumerableGetProperties(Type type, bool inherit, BindingFlags bindingFlags) { IgnorePropertiesAttribute[] attributes = (IgnorePropertiesAttribute[])type.GetCustomAttributes(typeof(IgnorePropertiesAttribute), inherit); Debug.Assert(attributes.Length == 0 || attributes.Length == 1, "There should be atmost one IgnoreProperties specified"); if (attributes.Length == 1) { foreach (string propertyName in attributes[0].PropertyNames) { if (String.IsNullOrEmpty(propertyName)) { throw new InvalidOperationException(Strings.IgnorePropertiesAttribute_PropertyNameCannotBeNullOrEmpty); } PropertyInfo property = type.GetProperty(propertyName, bindingFlags); if (property == null) { throw new InvalidOperationException(Strings.IgnorePropertiesAttribute_InvalidPropertyName(propertyName, type.FullName)); } } return attributes[0].PropertyNames; } return WebUtil.EmptyStringArray; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // //// CLR attribute to be annotated on types which indicate the list of properties // to ignore. // // // @owner [....] //--------------------------------------------------------------------- namespace System.Data.Services { using System; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Diagnostics; using System.Reflection; ///Attribute to be annotated on types with ETags. [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Design", "CA1019:DefineAccessorsForAttributeArguments", Justification = "Processed values are available.")] [AttributeUsage(AttributeTargets.Class, AllowMultiple = false, Inherited = true)] public sealed class IgnorePropertiesAttribute : System.Attribute { ///Name of the properties that form the ETag. private readonly ReadOnlyCollectionpropertyNames; // This constructor was added since string[] is not a CLS-compliant type and // compiler gives a warning as error saying this attribute doesn't have any // constructor that takes CLS-compliant type /// /// Initializes a new instance of IgnoreProperties attribute with the property name /// that needs to be ignored /// /// Name of the property that form the ETag for the current type. public IgnorePropertiesAttribute(string propertyName) { WebUtil.CheckArgumentNull(propertyName, "propertyName"); this.propertyNames = new ReadOnlyCollection(new List (new string[1] { propertyName })); } /// /// Initializes a new instance of IgnoreProperties attribute with the list of property names /// that need to be ignored /// /// Name of the properties that form the ETag for the current type. public IgnorePropertiesAttribute(params string[] propertyNames) { WebUtil.CheckArgumentNull(propertyNames, "propertyNames"); if (propertyNames.Length == 0) { throw new ArgumentException(Strings.ETagAttribute_MustSpecifyAtleastOnePropertyName, "propertyNames"); } this.propertyNames = new ReadOnlyCollection(new List (propertyNames)); } /// Name of the properties that needs to be ignored for the current type. public ReadOnlyCollectionPropertyNames { get { return this.propertyNames; } } /// /// Validate and get the list of properties specified by this attribute on the given type. /// /// clr type on which this attribute must have defined. /// whether we need to inherit this attribute or not. /// For context types,we need to, since we can have one context dervied from another, and we want to ignore all the properties on the base ones too. /// For resource types, we don't need to, since we don't want derived types to know about ignore properties of the base type. Also /// from derived type, you cannot change the definition of the base type. /// binding flags to be used for validating property names. ///list of property names specified on IgnoreProperties on the given type. internal static IEnumerableGetProperties(Type type, bool inherit, BindingFlags bindingFlags) { IgnorePropertiesAttribute[] attributes = (IgnorePropertiesAttribute[])type.GetCustomAttributes(typeof(IgnorePropertiesAttribute), inherit); Debug.Assert(attributes.Length == 0 || attributes.Length == 1, "There should be atmost one IgnoreProperties specified"); if (attributes.Length == 1) { foreach (string propertyName in attributes[0].PropertyNames) { if (String.IsNullOrEmpty(propertyName)) { throw new InvalidOperationException(Strings.IgnorePropertiesAttribute_PropertyNameCannotBeNullOrEmpty); } PropertyInfo property = type.GetProperty(propertyName, bindingFlags); if (property == null) { throw new InvalidOperationException(Strings.IgnorePropertiesAttribute_InvalidPropertyName(propertyName, type.FullName)); } } return attributes[0].PropertyNames; } return WebUtil.EmptyStringArray; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
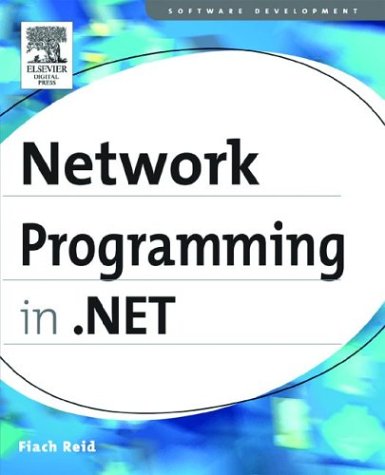
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataGridViewTextBoxCell.cs
- SiteMapDataSource.cs
- ObjectSecurity.cs
- ComponentCollection.cs
- RuntimeUtils.cs
- XPathNode.cs
- KeyEventArgs.cs
- DateTimeValueSerializerContext.cs
- ObjectStateEntryBaseUpdatableDataRecord.cs
- ContentElement.cs
- WindowsGraphicsWrapper.cs
- SkinBuilder.cs
- LayeredChannelListener.cs
- TimeManager.cs
- AssemblyBuilder.cs
- WebRequestModulesSection.cs
- SafePointer.cs
- PtsContext.cs
- XpsResourcePolicy.cs
- TableLayoutPanel.cs
- AssemblyNameProxy.cs
- HybridDictionary.cs
- WebUtil.cs
- ActivityLocationReferenceEnvironment.cs
- RuntimeResourceSet.cs
- HttpConfigurationContext.cs
- CompensationHandlingFilter.cs
- XPathDocument.cs
- EntitySqlException.cs
- XPathEmptyIterator.cs
- XamlSerializerUtil.cs
- COM2FontConverter.cs
- ContextMenu.cs
- ContainerVisual.cs
- PassportAuthenticationEventArgs.cs
- TypographyProperties.cs
- SoundPlayer.cs
- DataGridViewHitTestInfo.cs
- MonthCalendarDesigner.cs
- ColumnWidthChangingEvent.cs
- BCLDebug.cs
- Attributes.cs
- Int16Animation.cs
- RadioButton.cs
- ListenerAdaptersInstallComponent.cs
- SymbolEqualComparer.cs
- BindingGraph.cs
- SqlCacheDependency.cs
- ToolBarDesigner.cs
- OneOfScalarConst.cs
- HelloMessageApril2005.cs
- ChildChangedEventArgs.cs
- HttpContextServiceHost.cs
- DataListItemEventArgs.cs
- StrokeNodeOperations2.cs
- ServiceContractAttribute.cs
- WebPartsSection.cs
- RestHandlerFactory.cs
- ControlBuilderAttribute.cs
- ValidationError.cs
- __Error.cs
- SimplePropertyEntry.cs
- SqlClientMetaDataCollectionNames.cs
- TextEditorTyping.cs
- UnauthorizedAccessException.cs
- NativeMethods.cs
- RequestQueue.cs
- EditorPartChrome.cs
- _emptywebproxy.cs
- DynamicUpdateCommand.cs
- RemotingConfiguration.cs
- RegexEditorDialog.cs
- PrintController.cs
- SplayTreeNode.cs
- XDeferredAxisSource.cs
- Positioning.cs
- ClientSideProviderDescription.cs
- DBProviderConfigurationHandler.cs
- BitmapCache.cs
- ContextBase.cs
- AttachedPropertyBrowsableAttribute.cs
- RtfNavigator.cs
- ContentElement.cs
- WebPartEditorOkVerb.cs
- StateDesigner.CommentLayoutGlyph.cs
- ToolStripOverflowButton.cs
- SyndicationSerializer.cs
- ToolStripRendererSwitcher.cs
- BufferAllocator.cs
- ValidatorCollection.cs
- TreeNodeBinding.cs
- PropertyInformationCollection.cs
- LinkLabelLinkClickedEvent.cs
- DataGridPagerStyle.cs
- LogRecordSequence.cs
- BevelBitmapEffect.cs
- BitmapEffectOutputConnector.cs
- EntityProxyTypeInfo.cs
- HtmlControlAdapter.cs
- ServiceHost.cs