Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / WCF / System.ServiceModel.Activation / System / ServiceModel / Activation / ServiceHttpModule.cs / 1305376 / ServiceHttpModule.cs
//------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace System.ServiceModel.Activation { using System.Diagnostics; using System.Runtime; using System.Security; using System.ServiceModel; using System.Web; class ServiceHttpModule : IHttpModule { [Fx.Tag.SecurityNote(Critical = "Holds pointer to BeginProcessRequest which is SecurityCritical." + "This callback is called outside the PermitOnly context.")] [SecurityCritical] static BeginEventHandler beginEventHandler; //no need to invoke callback for non-WCF requests. static CompletedAsyncResult cachedAsyncResult = new CompletedAsyncResult(null, null); [Fx.Tag.SecurityNote(Critical = "This callback is called outside the PermitOnly context.")] [SecurityCritical] static EndEventHandler endEventHandler; static bool disabled; [Fx.Tag.SecurityNote(Miscellaneous = "RequiresReview - called outside PermitOnly context.")] public void Dispose() { } [Fx.Tag.SecurityNote(Critical = "Entry-point from ASP.NET, accesses begin/bndProcessRequest which are SecurityCritical.")] [SecurityCritical] public void Init(HttpApplication context) { if (ServiceHttpModule.beginEventHandler == null) { ServiceHttpModule.beginEventHandler = new BeginEventHandler(BeginProcessRequest); } if (ServiceHttpModule.endEventHandler == null) { ServiceHttpModule.endEventHandler = new EndEventHandler(EndProcessRequest); } context.AddOnPostAuthenticateRequestAsync( ServiceHttpModule.beginEventHandler, ServiceHttpModule.endEventHandler); } [Fx.Tag.SecurityNote(Critical = "Entry-point from asp.net, called outside PermitOnly context. ASP.NET calls are critical." + "HostedHttpRequestAsyncResult..ctor is critical because it captures HostedImpersonationContext (and makes it available later) " + "so caller must ensure that this is called in the right place.")] [SecurityCritical] static public IAsyncResult BeginProcessRequest(object sender, EventArgs e, AsyncCallback cb, object extraData) { if (ServiceHttpModule.disabled) { return cachedAsyncResult; } try { ServiceHostingEnvironment.SafeEnsureInitialized(); } catch (SecurityException exception) { ServiceHttpModule.disabled = true; if (DiagnosticUtility.ShouldTraceWarning) { DiagnosticUtility.ExceptionUtility.TraceHandledException(exception, TraceEventType.Warning); } // If requesting a .svc file, the HttpHandler will try to handle it. It will call // SafeEnsureInitialized() again, which will fail with the same exception (it is // idempotent on failure). This is the correct behavior. return cachedAsyncResult; } HttpApplication application = (HttpApplication) sender; // Check to see whether the extension is supported. string extension = application.Request.CurrentExecutionFilePathExtension; if (string.IsNullOrEmpty(extension)) { return cachedAsyncResult; } ServiceHostingEnvironment.ServiceType serviceType = ServiceHostingEnvironment.GetServiceType(extension); // do extension check first so that we do not need to do it in aspnetrouting/configurationbasedactivation if (serviceType == ServiceHostingEnvironment.ServiceType.Unknown) { return cachedAsyncResult; } // check for AspNetcompat if (ServiceHostingEnvironment.AspNetCompatibilityEnabled) { // remap httphandler for xamlx in CBA, since there is No physical file and // the xamlx httphandlerfactory will do file exist checking if (serviceType == ServiceHostingEnvironment.ServiceType.Workflow && ServiceHostingEnvironment.IsConfigurationBasedService(application)) { IHttpHandler cbaHandler = new ServiceHttpHandlerFactory().GetHandler( application.Context, application.Request.RequestType, application.Request.RawUrl.ToString(), application.Request.PhysicalApplicationPath); application.Context.RemapHandler(cbaHandler); } return cachedAsyncResult; } if (serviceType == ServiceHostingEnvironment.ServiceType.WCF) { return new HostedHttpRequestAsyncResult(application, false, false, cb, extraData); } if (serviceType == ServiceHostingEnvironment.ServiceType.Workflow) { return new HostedHttpRequestAsyncResult(application, false, true, cb, extraData); } return cachedAsyncResult; } [Fx.Tag.SecurityNote(Miscellaneous = "RequiresReview - called outside PermitOnly context.")] static public void EndProcessRequest(IAsyncResult ar) { //No need to call CompletedAsyncResult.End as the asyncResult has already completed. if (ar is HostedHttpRequestAsyncResult) { HostedHttpRequestAsyncResult.End(ar); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace System.ServiceModel.Activation { using System.Diagnostics; using System.Runtime; using System.Security; using System.ServiceModel; using System.Web; class ServiceHttpModule : IHttpModule { [Fx.Tag.SecurityNote(Critical = "Holds pointer to BeginProcessRequest which is SecurityCritical." + "This callback is called outside the PermitOnly context.")] [SecurityCritical] static BeginEventHandler beginEventHandler; //no need to invoke callback for non-WCF requests. static CompletedAsyncResult cachedAsyncResult = new CompletedAsyncResult(null, null); [Fx.Tag.SecurityNote(Critical = "This callback is called outside the PermitOnly context.")] [SecurityCritical] static EndEventHandler endEventHandler; static bool disabled; [Fx.Tag.SecurityNote(Miscellaneous = "RequiresReview - called outside PermitOnly context.")] public void Dispose() { } [Fx.Tag.SecurityNote(Critical = "Entry-point from ASP.NET, accesses begin/bndProcessRequest which are SecurityCritical.")] [SecurityCritical] public void Init(HttpApplication context) { if (ServiceHttpModule.beginEventHandler == null) { ServiceHttpModule.beginEventHandler = new BeginEventHandler(BeginProcessRequest); } if (ServiceHttpModule.endEventHandler == null) { ServiceHttpModule.endEventHandler = new EndEventHandler(EndProcessRequest); } context.AddOnPostAuthenticateRequestAsync( ServiceHttpModule.beginEventHandler, ServiceHttpModule.endEventHandler); } [Fx.Tag.SecurityNote(Critical = "Entry-point from asp.net, called outside PermitOnly context. ASP.NET calls are critical." + "HostedHttpRequestAsyncResult..ctor is critical because it captures HostedImpersonationContext (and makes it available later) " + "so caller must ensure that this is called in the right place.")] [SecurityCritical] static public IAsyncResult BeginProcessRequest(object sender, EventArgs e, AsyncCallback cb, object extraData) { if (ServiceHttpModule.disabled) { return cachedAsyncResult; } try { ServiceHostingEnvironment.SafeEnsureInitialized(); } catch (SecurityException exception) { ServiceHttpModule.disabled = true; if (DiagnosticUtility.ShouldTraceWarning) { DiagnosticUtility.ExceptionUtility.TraceHandledException(exception, TraceEventType.Warning); } // If requesting a .svc file, the HttpHandler will try to handle it. It will call // SafeEnsureInitialized() again, which will fail with the same exception (it is // idempotent on failure). This is the correct behavior. return cachedAsyncResult; } HttpApplication application = (HttpApplication) sender; // Check to see whether the extension is supported. string extension = application.Request.CurrentExecutionFilePathExtension; if (string.IsNullOrEmpty(extension)) { return cachedAsyncResult; } ServiceHostingEnvironment.ServiceType serviceType = ServiceHostingEnvironment.GetServiceType(extension); // do extension check first so that we do not need to do it in aspnetrouting/configurationbasedactivation if (serviceType == ServiceHostingEnvironment.ServiceType.Unknown) { return cachedAsyncResult; } // check for AspNetcompat if (ServiceHostingEnvironment.AspNetCompatibilityEnabled) { // remap httphandler for xamlx in CBA, since there is No physical file and // the xamlx httphandlerfactory will do file exist checking if (serviceType == ServiceHostingEnvironment.ServiceType.Workflow && ServiceHostingEnvironment.IsConfigurationBasedService(application)) { IHttpHandler cbaHandler = new ServiceHttpHandlerFactory().GetHandler( application.Context, application.Request.RequestType, application.Request.RawUrl.ToString(), application.Request.PhysicalApplicationPath); application.Context.RemapHandler(cbaHandler); } return cachedAsyncResult; } if (serviceType == ServiceHostingEnvironment.ServiceType.WCF) { return new HostedHttpRequestAsyncResult(application, false, false, cb, extraData); } if (serviceType == ServiceHostingEnvironment.ServiceType.Workflow) { return new HostedHttpRequestAsyncResult(application, false, true, cb, extraData); } return cachedAsyncResult; } [Fx.Tag.SecurityNote(Miscellaneous = "RequiresReview - called outside PermitOnly context.")] static public void EndProcessRequest(IAsyncResult ar) { //No need to call CompletedAsyncResult.End as the asyncResult has already completed. if (ar is HostedHttpRequestAsyncResult) { HostedHttpRequestAsyncResult.End(ar); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
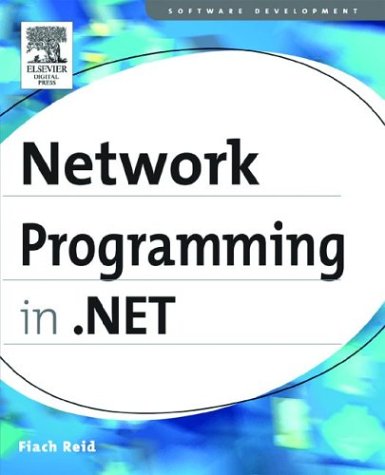
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TypeConverter.cs
- BamlTreeNode.cs
- DispatcherProcessingDisabled.cs
- BindingsCollection.cs
- CreateUserWizardDesigner.cs
- MarginsConverter.cs
- hresults.cs
- ScrollBarRenderer.cs
- DropDownButton.cs
- FrameDimension.cs
- NamespaceMapping.cs
- ConsoleKeyInfo.cs
- Vector3DAnimationBase.cs
- TextControlDesigner.cs
- KeyInfo.cs
- UITypeEditor.cs
- DataSetFieldSchema.cs
- ExcludeFromCodeCoverageAttribute.cs
- ParsedAttributeCollection.cs
- querybuilder.cs
- PasswordRecoveryAutoFormat.cs
- RelationshipDetailsRow.cs
- FrameworkElementFactory.cs
- GridView.cs
- FormClosingEvent.cs
- login.cs
- ProjectionCamera.cs
- SocketConnection.cs
- FilePrompt.cs
- DesignerAdapterAttribute.cs
- WebPartMenu.cs
- DomNameTable.cs
- _ListenerResponseStream.cs
- TextMarkerSource.cs
- ICspAsymmetricAlgorithm.cs
- PrimarySelectionAdorner.cs
- BuildManagerHost.cs
- FixedSOMGroup.cs
- DropShadowBitmapEffect.cs
- SystemFonts.cs
- BamlBinaryReader.cs
- EntityObject.cs
- SystemTcpConnection.cs
- MachineSettingsSection.cs
- TriggerActionCollection.cs
- TextBoxBase.cs
- ValueUnavailableException.cs
- SecurityContext.cs
- ContainerUtilities.cs
- InvalidProgramException.cs
- MatrixIndependentAnimationStorage.cs
- SplitContainerDesigner.cs
- HttpConfigurationSystem.cs
- ListSurrogate.cs
- CodeAssignStatement.cs
- UriScheme.cs
- XmlSchemaRedefine.cs
- ListBoxItemWrapperAutomationPeer.cs
- InputManager.cs
- DelegateHelpers.cs
- View.cs
- MasterPageCodeDomTreeGenerator.cs
- IdentifierCollection.cs
- ApplicationFileCodeDomTreeGenerator.cs
- EmptyStringExpandableObjectConverter.cs
- ExtensionDataReader.cs
- IisTraceListener.cs
- StreamWithDictionary.cs
- ActivityXRefPropertyEditor.cs
- ScrollBarRenderer.cs
- SqlXmlStorage.cs
- AuthorizationSection.cs
- HexParser.cs
- GridViewCommandEventArgs.cs
- ListViewItemSelectionChangedEvent.cs
- NullReferenceException.cs
- TargetException.cs
- ProvidePropertyAttribute.cs
- ComboBoxRenderer.cs
- DeferredElementTreeState.cs
- _HTTPDateParse.cs
- httpserverutility.cs
- CreateParams.cs
- __ComObject.cs
- SignatureHelper.cs
- ToolboxItemImageConverter.cs
- SapiAttributeParser.cs
- ExpressionVisitorHelpers.cs
- Padding.cs
- MemoryRecordBuffer.cs
- XMLDiffLoader.cs
- XmlSchemaType.cs
- GridViewSortEventArgs.cs
- ResolveDuplexCD1AsyncResult.cs
- SamlAttributeStatement.cs
- CompositeActivityMarkupSerializer.cs
- DataGridViewRowsRemovedEventArgs.cs
- PathGradientBrush.cs
- wgx_commands.cs
- GroupStyle.cs