Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / WCF / IdentityModel / System / IdentityModel / Tokens / SamlAttributeStatement.cs / 1305376 / SamlAttributeStatement.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.IdentityModel.Tokens { using System.Collections.Generic; using System.Collections.ObjectModel; using System.Globalization; using System.IdentityModel; using System.IdentityModel.Claims; using System.IdentityModel.Selectors; using System.Runtime.Serialization; using System.Xml.Serialization; using System.Xml; public class SamlAttributeStatement : SamlSubjectStatement { readonly ImmutableCollectionattributes = new ImmutableCollection (); bool isReadOnly = false; public SamlAttributeStatement() { } public SamlAttributeStatement(SamlSubject samlSubject, IEnumerable attributes) : base(samlSubject) { if (attributes == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentNullException("attributes")); foreach (SamlAttribute attribute in attributes) { if (attribute == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgument(SR.GetString(SR.SAMLEntityCannotBeNullOrEmpty, XD.SamlDictionary.Attribute.Value)); this.attributes.Add(attribute); } CheckObjectValidity(); } public IList Attributes { get { return this.attributes; } } public override bool IsReadOnly { get { return this.isReadOnly; } } public override void MakeReadOnly() { if (!this.isReadOnly) { foreach (SamlAttribute attribute in attributes) { attribute.MakeReadOnly(); } this.attributes.MakeReadOnly(); this.isReadOnly = true; } } void CheckObjectValidity() { if (this.SamlSubject == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new SecurityTokenException(SR.GetString(SR.SAMLSubjectStatementRequiresSubject))); if (this.attributes.Count == 0) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new SecurityTokenException(SR.GetString(SR.SAMLAttributeShouldHaveOneValue))); } public override void ReadXml(XmlDictionaryReader reader, SamlSerializer samlSerializer, SecurityTokenSerializer keyInfoSerializer, SecurityTokenResolver outOfBandTokenResolver) { if (reader == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentNullException("reader")); if (samlSerializer == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentNullException("samlSerializer")); #pragma warning suppress 56506 // samlSerializer.DictionaryManager is never null. SamlDictionary dictionary = samlSerializer.DictionaryManager.SamlDictionary; reader.MoveToContent(); reader.Read(); if (reader.IsStartElement(dictionary.Subject, dictionary.Namespace)) { SamlSubject subject = new SamlSubject(); subject.ReadXml(reader, samlSerializer, keyInfoSerializer, outOfBandTokenResolver); base.SamlSubject = subject; } else { // SAML Subject is a required Attribute Statement clause. throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new SecurityTokenException(SR.GetString(SR.SAMLAttributeStatementMissingSubjectOnRead))); } while (reader.IsStartElement()) { if (reader.IsStartElement(dictionary.Attribute, dictionary.Namespace)) { // SAML Attribute is a extensibility point. So ask the SAML serializer // to load this part. SamlAttribute attribute = samlSerializer.LoadAttribute(reader, keyInfoSerializer, outOfBandTokenResolver); if (attribute == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new SecurityTokenException(SR.GetString(SR.SAMLUnableToLoadAttribute))); this.attributes.Add(attribute); } else { break; } } if (this.attributes.Count == 0) { // Each Attribute statement should have at least one attribute. throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new SecurityTokenException(SR.GetString(SR.SAMLAttributeStatementMissingAttributeOnRead))); } reader.MoveToContent(); reader.ReadEndElement(); } public override void WriteXml(XmlDictionaryWriter writer, SamlSerializer samlSerializer, SecurityTokenSerializer keyInfoSerializer) { CheckObjectValidity(); if (writer == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentNullException("writer")); if (samlSerializer == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentNullException("samlSerializer")); #pragma warning suppress 56506 // samlSerializer.DictionaryManager is never null. SamlDictionary dictionary = samlSerializer.DictionaryManager.SamlDictionary; writer.WriteStartElement(dictionary.PreferredPrefix.Value, dictionary.AttributeStatement, dictionary.Namespace); this.SamlSubject.WriteXml(writer, samlSerializer, keyInfoSerializer); for (int i = 0; i < this.attributes.Count; i++) { this.attributes[i].WriteXml(writer, samlSerializer, keyInfoSerializer); } writer.WriteEndElement(); } protected override void AddClaimsToList(IList claims) { if (claims == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("claims"); for (int i = 0; i < attributes.Count; i++) { if (attributes[i] != null) { ReadOnlyCollection attributeClaims = attributes[i].ExtractClaims(); if (attributeClaims != null) { for (int j = 0; j < attributeClaims.Count; ++j) if (attributeClaims[j] != null) claims.Add(attributeClaims[j]); } } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.IdentityModel.Tokens { using System.Collections.Generic; using System.Collections.ObjectModel; using System.Globalization; using System.IdentityModel; using System.IdentityModel.Claims; using System.IdentityModel.Selectors; using System.Runtime.Serialization; using System.Xml.Serialization; using System.Xml; public class SamlAttributeStatement : SamlSubjectStatement { readonly ImmutableCollection attributes = new ImmutableCollection (); bool isReadOnly = false; public SamlAttributeStatement() { } public SamlAttributeStatement(SamlSubject samlSubject, IEnumerable attributes) : base(samlSubject) { if (attributes == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentNullException("attributes")); foreach (SamlAttribute attribute in attributes) { if (attribute == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgument(SR.GetString(SR.SAMLEntityCannotBeNullOrEmpty, XD.SamlDictionary.Attribute.Value)); this.attributes.Add(attribute); } CheckObjectValidity(); } public IList Attributes { get { return this.attributes; } } public override bool IsReadOnly { get { return this.isReadOnly; } } public override void MakeReadOnly() { if (!this.isReadOnly) { foreach (SamlAttribute attribute in attributes) { attribute.MakeReadOnly(); } this.attributes.MakeReadOnly(); this.isReadOnly = true; } } void CheckObjectValidity() { if (this.SamlSubject == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new SecurityTokenException(SR.GetString(SR.SAMLSubjectStatementRequiresSubject))); if (this.attributes.Count == 0) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new SecurityTokenException(SR.GetString(SR.SAMLAttributeShouldHaveOneValue))); } public override void ReadXml(XmlDictionaryReader reader, SamlSerializer samlSerializer, SecurityTokenSerializer keyInfoSerializer, SecurityTokenResolver outOfBandTokenResolver) { if (reader == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentNullException("reader")); if (samlSerializer == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentNullException("samlSerializer")); #pragma warning suppress 56506 // samlSerializer.DictionaryManager is never null. SamlDictionary dictionary = samlSerializer.DictionaryManager.SamlDictionary; reader.MoveToContent(); reader.Read(); if (reader.IsStartElement(dictionary.Subject, dictionary.Namespace)) { SamlSubject subject = new SamlSubject(); subject.ReadXml(reader, samlSerializer, keyInfoSerializer, outOfBandTokenResolver); base.SamlSubject = subject; } else { // SAML Subject is a required Attribute Statement clause. throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new SecurityTokenException(SR.GetString(SR.SAMLAttributeStatementMissingSubjectOnRead))); } while (reader.IsStartElement()) { if (reader.IsStartElement(dictionary.Attribute, dictionary.Namespace)) { // SAML Attribute is a extensibility point. So ask the SAML serializer // to load this part. SamlAttribute attribute = samlSerializer.LoadAttribute(reader, keyInfoSerializer, outOfBandTokenResolver); if (attribute == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new SecurityTokenException(SR.GetString(SR.SAMLUnableToLoadAttribute))); this.attributes.Add(attribute); } else { break; } } if (this.attributes.Count == 0) { // Each Attribute statement should have at least one attribute. throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new SecurityTokenException(SR.GetString(SR.SAMLAttributeStatementMissingAttributeOnRead))); } reader.MoveToContent(); reader.ReadEndElement(); } public override void WriteXml(XmlDictionaryWriter writer, SamlSerializer samlSerializer, SecurityTokenSerializer keyInfoSerializer) { CheckObjectValidity(); if (writer == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentNullException("writer")); if (samlSerializer == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentNullException("samlSerializer")); #pragma warning suppress 56506 // samlSerializer.DictionaryManager is never null. SamlDictionary dictionary = samlSerializer.DictionaryManager.SamlDictionary; writer.WriteStartElement(dictionary.PreferredPrefix.Value, dictionary.AttributeStatement, dictionary.Namespace); this.SamlSubject.WriteXml(writer, samlSerializer, keyInfoSerializer); for (int i = 0; i < this.attributes.Count; i++) { this.attributes[i].WriteXml(writer, samlSerializer, keyInfoSerializer); } writer.WriteEndElement(); } protected override void AddClaimsToList(IList claims) { if (claims == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("claims"); for (int i = 0; i < attributes.Count; i++) { if (attributes[i] != null) { ReadOnlyCollection attributeClaims = attributes[i].ExtractClaims(); if (attributeClaims != null) { for (int j = 0; j < attributeClaims.Count; ++j) if (attributeClaims[j] != null) claims.Add(attributeClaims[j]); } } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
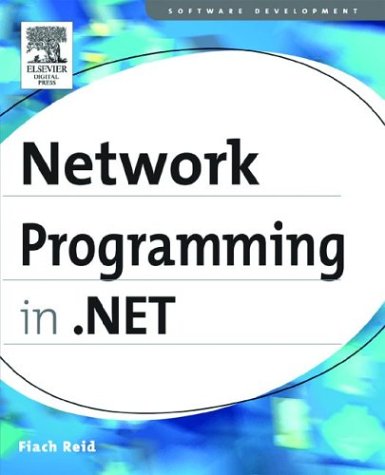
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SaveFileDialog.cs
- XpsSerializationException.cs
- DefaultPrintController.cs
- SqlVisitor.cs
- RefType.cs
- MessagePartProtectionMode.cs
- Int32CollectionValueSerializer.cs
- UInt16Storage.cs
- HtmlInputHidden.cs
- ListControlConvertEventArgs.cs
- ThreadStaticAttribute.cs
- ModelItemCollectionImpl.cs
- LabelInfo.cs
- HighContrastHelper.cs
- NativeMethods.cs
- MetadataUtilsSmi.cs
- InputScope.cs
- BufferModeSettings.cs
- EncryptedData.cs
- WebPartZoneDesigner.cs
- HyperlinkAutomationPeer.cs
- HighContrastHelper.cs
- SharedPersonalizationStateInfo.cs
- QueryExtender.cs
- NetNamedPipeSecurityElement.cs
- PrimarySelectionGlyph.cs
- Stream.cs
- QueryableDataSourceEditData.cs
- PointAnimationBase.cs
- XmlReaderSettings.cs
- SqlClientWrapperSmiStream.cs
- SqlMethodCallConverter.cs
- ParallelTimeline.cs
- CqlBlock.cs
- ImageDrawing.cs
- OdbcHandle.cs
- DBSchemaRow.cs
- BoundField.cs
- HandledMouseEvent.cs
- ListViewItem.cs
- ParserStreamGeometryContext.cs
- ObjectDesignerDataSourceView.cs
- BitmapCodecInfo.cs
- BindingContext.cs
- SqlInternalConnectionSmi.cs
- SignHashRequest.cs
- DeclaredTypeValidatorAttribute.cs
- Root.cs
- PriorityQueue.cs
- FileSystemEventArgs.cs
- SemanticTag.cs
- Matrix.cs
- Control.cs
- AttributeUsageAttribute.cs
- CodeTypeParameter.cs
- OutputCacheModule.cs
- GeometryCombineModeValidation.cs
- DesignerTextWriter.cs
- RequiredFieldValidator.cs
- OLEDB_Util.cs
- DispatcherObject.cs
- XmlUtil.cs
- VisualStyleElement.cs
- WebRequestModuleElement.cs
- InputQueue.cs
- LoginName.cs
- MetadataHelper.cs
- VisualStyleTypesAndProperties.cs
- SetUserLanguageRequest.cs
- SafeNativeMethods.cs
- PageOrientation.cs
- ChildrenQuery.cs
- DragEvent.cs
- EtwTrackingParticipant.cs
- Pair.cs
- AddingNewEventArgs.cs
- DesignerVerb.cs
- SoapExtensionTypeElementCollection.cs
- GregorianCalendar.cs
- ChannelFactoryRefCache.cs
- IdentityValidationException.cs
- serverconfig.cs
- EncryptedReference.cs
- MaskedTextBoxTextEditorDropDown.cs
- MemberInfoSerializationHolder.cs
- IsolatedStorage.cs
- NullRuntimeConfig.cs
- FormCollection.cs
- WebPartConnectionsConfigureVerb.cs
- XmlWriter.cs
- ExpressionConverter.cs
- XmlRawWriter.cs
- Rotation3DAnimation.cs
- DataGridViewCellParsingEventArgs.cs
- OperationSelectorBehavior.cs
- SetterBaseCollection.cs
- AttributeQuery.cs
- SafeProcessHandle.cs
- BmpBitmapDecoder.cs
- WebPartConnectionsCancelEventArgs.cs