Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / CommonUI / System / Drawing / ImageInfo.cs / 1 / ImageInfo.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Drawing { using System.Threading; using System; using System.Diagnostics; using System.Drawing.Imaging; ////// Animates one or more images that have time-based frames. /// This file contains the nested ImageInfo class - See ImageAnimator.cs for the definition of the outer class. /// public sealed partial class ImageAnimator { ////// ImageAnimator nested helper class used to store extra image state info. /// private class ImageInfo { const int PropertyTagFrameDelay = 0x5100; Image image; int frame; int frameCount; bool frameDirty; bool animated; EventHandler onFrameChangedHandler; int[] frameDelay; int frameTimer; ////// public ImageInfo(Image image) { this.image = image; animated = ImageAnimator.CanAnimate(image); if (animated) { frameCount = image.GetFrameCount(FrameDimension.Time); PropertyItem frameDelayItem = image.GetPropertyItem(PropertyTagFrameDelay); // If the image does not have a frame delay, we just return 0. // if (frameDelayItem != null) { // Convert the frame delay from byte[] to int // byte[] values = frameDelayItem.Value; Debug.Assert(values.Length == 4 * FrameCount, "PropertyItem has invalid value byte array"); frameDelay = new int[FrameCount]; for (int i=0; i < FrameCount; ++i) { frameDelay[i] = values[i * 4] + 256 * values[i * 4 + 1] + 256 * 256 * values[i * 4 + 2] + 256 * 256 * 256 * values[i * 4 + 3]; } } } else { frameCount = 1; } if (frameDelay == null) { frameDelay = new int[FrameCount]; } } ////// Whether the image supports animation. /// public bool Animated { get { return animated; } } ////// The current frame. /// public int Frame { get { return frame; } set { if (frame != value) { if (value < 0 || value >= FrameCount) { throw new ArgumentException(SR.GetString(SR.InvalidFrame), "value"); } if (Animated) { frame = value; frameDirty = true; OnFrameChanged(EventArgs.Empty); } } } } ////// The current frame has not been updated. /// public bool FrameDirty { get { return frameDirty; } } ////// public EventHandler FrameChangedHandler { get { return onFrameChangedHandler; } set { onFrameChangedHandler = value; } } ////// The number of frames in the image. /// public int FrameCount { get { return frameCount; } } ////// The delay associated with the frame at the specified index. /// public int FrameDelay(int frame) { return frameDelay[frame]; } ////// internal int FrameTimer { get { return frameTimer; } set { frameTimer = value; } } ////// The image this object wraps. /// internal Image Image { get { return image; } } ////// Selects the current frame as the active frame in the image. /// internal void UpdateFrame() { if (frameDirty) { image.SelectActiveFrame(FrameDimension.Time, Frame); frameDirty = false; } } ////// Raises the FrameChanged event. /// protected void OnFrameChanged(EventArgs e) { if( this.onFrameChangedHandler != null ){ this.onFrameChangedHandler(image, e); } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
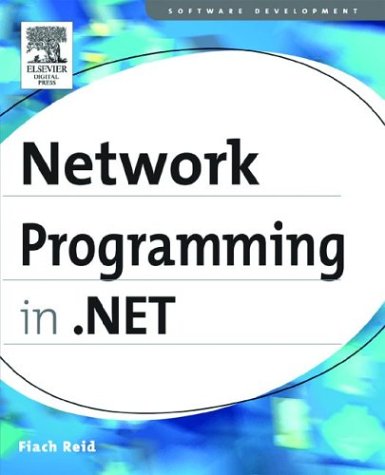
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CodeMemberField.cs
- LocationUpdates.cs
- WinCategoryAttribute.cs
- DataProtectionSecurityStateEncoder.cs
- ViewGenerator.cs
- WebResponse.cs
- ParameterEditorUserControl.cs
- PlatformNotSupportedException.cs
- KerberosTicketHashIdentifierClause.cs
- ChannelManagerHelpers.cs
- ThemeDirectoryCompiler.cs
- HwndHostAutomationPeer.cs
- ResourceContainer.cs
- ControlBindingsCollection.cs
- SoapIgnoreAttribute.cs
- XmlSignificantWhitespace.cs
- PolyBezierSegmentFigureLogic.cs
- NumericUpDownAccelerationCollection.cs
- MatrixAnimationUsingPath.cs
- TreeNodeClickEventArgs.cs
- OleDbSchemaGuid.cs
- ExtenderControl.cs
- ReflectPropertyDescriptor.cs
- PasswordBox.cs
- ClientFormsAuthenticationCredentials.cs
- NumericExpr.cs
- DataGridViewCellFormattingEventArgs.cs
- TemplateApplicationHelper.cs
- PerformanceCounterPermissionEntryCollection.cs
- BatchParser.cs
- OleDbMetaDataFactory.cs
- GridViewItemAutomationPeer.cs
- DataGridViewImageColumn.cs
- ConstrainedDataObject.cs
- ToolStripComboBox.cs
- TemplateNameScope.cs
- WebRequestModuleElement.cs
- HttpCacheVary.cs
- ConnectionInterfaceCollection.cs
- TemplateColumn.cs
- _LoggingObject.cs
- SkipStoryboardToFill.cs
- TemplateBindingExtension.cs
- RotateTransform3D.cs
- safex509handles.cs
- CheckableControlBaseAdapter.cs
- activationcontext.cs
- EventSetter.cs
- Button.cs
- CodeDirectionExpression.cs
- Paragraph.cs
- OdbcConnectionFactory.cs
- ADMembershipProvider.cs
- ListViewCommandEventArgs.cs
- ChannelOptions.cs
- WebPartDisplayMode.cs
- ToolStripArrowRenderEventArgs.cs
- EntityClassGenerator.cs
- ConfigXmlWhitespace.cs
- FixedDocumentPaginator.cs
- MembershipUser.cs
- HtmlLiteralTextAdapter.cs
- ButtonPopupAdapter.cs
- SQLDecimalStorage.cs
- EnvelopedPkcs7.cs
- DataExpression.cs
- DecoratedNameAttribute.cs
- SchemaMapping.cs
- ApplicationManager.cs
- PeerToPeerException.cs
- FlowLayoutSettings.cs
- QilScopedVisitor.cs
- linebase.cs
- PropertyPathConverter.cs
- SuppressIldasmAttribute.cs
- HttpRequestCacheValidator.cs
- SafeTimerHandle.cs
- PropertyPushdownHelper.cs
- PrinterSettings.cs
- Certificate.cs
- TileModeValidation.cs
- VirtualDirectoryMappingCollection.cs
- assemblycache.cs
- KerberosTicketHashIdentifierClause.cs
- UrlMappingsModule.cs
- RemotingService.cs
- DeviceContext.cs
- DesignerWebPartChrome.cs
- MetadataArtifactLoaderComposite.cs
- Cursor.cs
- x509store.cs
- SiteMapSection.cs
- WeakKeyDictionary.cs
- DataServiceQueryOfT.cs
- UIElementHelper.cs
- TransformFinalBlockRequest.cs
- SqlFileStream.cs
- SatelliteContractVersionAttribute.cs
- ParallelActivityDesigner.cs
- OleDbConnectionFactory.cs