Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / ndp / fx / src / DataEntity / System / Data / Metadata / MetadataArtifactLoaderComposite.cs / 3 / MetadataArtifactLoaderComposite.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] //--------------------------------------------------------------------- using System.Collections.Generic; using System.Collections; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Text; using System.Xml; using System.Data.Mapping; using System.IO; using System.Security; using System.Security.Permissions; using System.Collections.ObjectModel; namespace System.Data.Metadata.Edm { ////// This class represents a super-collection (a collection of collections) /// of artifact resources. Typically, this "meta-collection" would contain /// artifacts represented as individual files, directories (which are in /// turn collections of files), and embedded resources. /// ///This is the root class for access to all loader objects. internal class MetadataArtifactLoaderComposite : MetadataArtifactLoader, IEnumerable{ /// /// The list of loaders aggregated by the composite. /// private readonly ReadOnlyCollection_children; /// /// Constructor - loads all resources into the _children collection /// /// A list of collections to aggregate public MetadataArtifactLoaderComposite(Listchildren) { Debug.Assert(children != null); _children = new List (children).AsReadOnly(); } public override string Path { get { return string.Empty; } } public override void CollectFilePermissionPaths(List paths, DataSpace spaceToGet) { foreach (MetadataArtifactLoader loader in _children) { loader.CollectFilePermissionPaths(paths, spaceToGet); } } public override bool IsComposite { get { return true; } } /// /// Get the list of paths to all artifacts in the original, unexpanded form /// ///A List of strings identifying paths to all resources public override ListGetOriginalPaths() { List list = new List (); foreach (MetadataArtifactLoader loader in _children) { list.AddRange(loader.GetOriginalPaths()); } return list; } /// /// Get paths to artifacts for a specific DataSpace, in the original, unexpanded /// form /// /// The DataSpace for the artifacts of interest ///A List of strings identifying paths to all artifacts for a specific DataSpace public override ListGetOriginalPaths(DataSpace spaceToGet) { List list = new List (); foreach (MetadataArtifactLoader loader in _children) { list.AddRange(loader.GetOriginalPaths(spaceToGet)); } return list; } /// /// Get paths to artifacts for a specific DataSpace. /// /// The DataSpace for the artifacts of interest ///A List of strings identifying paths to all artifacts for a specific DataSpace public override ListGetPaths(DataSpace spaceToGet) { List list = new List (); foreach (MetadataArtifactLoader loader in _children) { list.AddRange(loader.GetPaths(spaceToGet)); } return list; } /// /// Get paths to all artifacts /// ///A List of strings identifying paths to all resources public override ListGetPaths() { List list = new List (); foreach (MetadataArtifactLoader resource in _children) { list.AddRange(resource.GetPaths()); } return list; } /// /// Aggregates all resource streams from the _children collection /// ///A List of XmlReader objects; cannot be null public override ListGetReaders(Dictionary sourceDictionary) { List list = new List (); foreach (MetadataArtifactLoader resource in _children) { list.AddRange(resource.GetReaders(sourceDictionary)); } return list; } /// /// Get XmlReaders for a specific DataSpace. /// /// The DataSpace corresponding to the requested artifacts ///A List of XmlReader objects public override ListCreateReaders(DataSpace spaceToGet) { List list = new List (); foreach (MetadataArtifactLoader resource in _children) { list.AddRange(resource.CreateReaders(spaceToGet)); } return list; } #region IEnumerable Members public IEnumerator GetEnumerator() { return this._children.GetEnumerator(); } #endregion #region IEnumerable Members IEnumerator IEnumerable.GetEnumerator() { return this._children.GetEnumerator(); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] //--------------------------------------------------------------------- using System.Collections.Generic; using System.Collections; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Text; using System.Xml; using System.Data.Mapping; using System.IO; using System.Security; using System.Security.Permissions; using System.Collections.ObjectModel; namespace System.Data.Metadata.Edm { ////// This class represents a super-collection (a collection of collections) /// of artifact resources. Typically, this "meta-collection" would contain /// artifacts represented as individual files, directories (which are in /// turn collections of files), and embedded resources. /// ///This is the root class for access to all loader objects. internal class MetadataArtifactLoaderComposite : MetadataArtifactLoader, IEnumerable{ /// /// The list of loaders aggregated by the composite. /// private readonly ReadOnlyCollection_children; /// /// Constructor - loads all resources into the _children collection /// /// A list of collections to aggregate public MetadataArtifactLoaderComposite(Listchildren) { Debug.Assert(children != null); _children = new List (children).AsReadOnly(); } public override string Path { get { return string.Empty; } } public override void CollectFilePermissionPaths(List paths, DataSpace spaceToGet) { foreach (MetadataArtifactLoader loader in _children) { loader.CollectFilePermissionPaths(paths, spaceToGet); } } public override bool IsComposite { get { return true; } } /// /// Get the list of paths to all artifacts in the original, unexpanded form /// ///A List of strings identifying paths to all resources public override ListGetOriginalPaths() { List list = new List (); foreach (MetadataArtifactLoader loader in _children) { list.AddRange(loader.GetOriginalPaths()); } return list; } /// /// Get paths to artifacts for a specific DataSpace, in the original, unexpanded /// form /// /// The DataSpace for the artifacts of interest ///A List of strings identifying paths to all artifacts for a specific DataSpace public override ListGetOriginalPaths(DataSpace spaceToGet) { List list = new List (); foreach (MetadataArtifactLoader loader in _children) { list.AddRange(loader.GetOriginalPaths(spaceToGet)); } return list; } /// /// Get paths to artifacts for a specific DataSpace. /// /// The DataSpace for the artifacts of interest ///A List of strings identifying paths to all artifacts for a specific DataSpace public override ListGetPaths(DataSpace spaceToGet) { List list = new List (); foreach (MetadataArtifactLoader loader in _children) { list.AddRange(loader.GetPaths(spaceToGet)); } return list; } /// /// Get paths to all artifacts /// ///A List of strings identifying paths to all resources public override ListGetPaths() { List list = new List (); foreach (MetadataArtifactLoader resource in _children) { list.AddRange(resource.GetPaths()); } return list; } /// /// Aggregates all resource streams from the _children collection /// ///A List of XmlReader objects; cannot be null public override ListGetReaders(Dictionary sourceDictionary) { List list = new List (); foreach (MetadataArtifactLoader resource in _children) { list.AddRange(resource.GetReaders(sourceDictionary)); } return list; } /// /// Get XmlReaders for a specific DataSpace. /// /// The DataSpace corresponding to the requested artifacts ///A List of XmlReader objects public override ListCreateReaders(DataSpace spaceToGet) { List list = new List (); foreach (MetadataArtifactLoader resource in _children) { list.AddRange(resource.CreateReaders(spaceToGet)); } return list; } #region IEnumerable Members public IEnumerator GetEnumerator() { return this._children.GetEnumerator(); } #endregion #region IEnumerable Members IEnumerator IEnumerable.GetEnumerator() { return this._children.GetEnumerator(); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
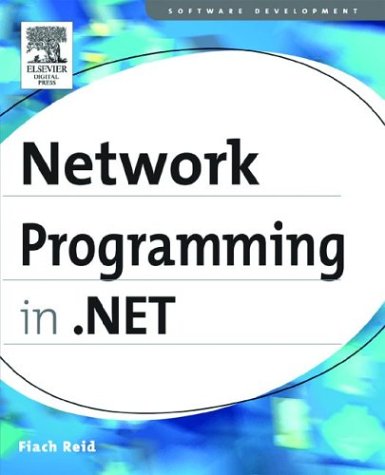
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CodeBinaryOperatorExpression.cs
- EFTableProvider.cs
- WebPermission.cs
- TrackingStringDictionary.cs
- SerializationEventsCache.cs
- ToolStripProfessionalLowResolutionRenderer.cs
- AssemblyCache.cs
- Point3DKeyFrameCollection.cs
- AlgoModule.cs
- ButtonRenderer.cs
- RemoteWebConfigurationHost.cs
- TypeValidationEventArgs.cs
- Stack.cs
- XmlRawWriter.cs
- UDPClient.cs
- WebPartCloseVerb.cs
- FormViewUpdateEventArgs.cs
- DataListItemCollection.cs
- HttpWebRequest.cs
- DataContract.cs
- SoapDocumentServiceAttribute.cs
- ProfileManager.cs
- Control.cs
- AsyncOperationManager.cs
- DES.cs
- AspNetHostingPermission.cs
- IIS7UserPrincipal.cs
- input.cs
- GetBrowserTokenRequest.cs
- SuppressMergeCheckAttribute.cs
- UTF8Encoding.cs
- WebResourceAttribute.cs
- SecurityMode.cs
- RadioButtonDesigner.cs
- FactoryGenerator.cs
- Canvas.cs
- PropertyDescriptorComparer.cs
- counter.cs
- ChtmlTextWriter.cs
- TargetException.cs
- RoleService.cs
- System.Data_BID.cs
- CryptographicAttribute.cs
- EntityDataSource.cs
- ArrangedElement.cs
- Group.cs
- IsolatedStorage.cs
- ObjectStorage.cs
- CanonicalizationDriver.cs
- HijriCalendar.cs
- SafeMILHandle.cs
- CodeDirectionExpression.cs
- Hash.cs
- VirtualDirectoryMapping.cs
- path.cs
- CalendarKeyboardHelper.cs
- AmbientValueAttribute.cs
- KnownBoxes.cs
- Span.cs
- DummyDataSource.cs
- ProfileManager.cs
- GorillaCodec.cs
- PseudoWebRequest.cs
- ToolStripProgressBar.cs
- _UncName.cs
- TraceHandlerErrorFormatter.cs
- CategoryNameCollection.cs
- ConstNode.cs
- Win32Native.cs
- TextTreeInsertUndoUnit.cs
- DoubleAnimation.cs
- XPathBinder.cs
- MarkupObject.cs
- Page.cs
- WebPartDescription.cs
- _ProxyRegBlob.cs
- ReferentialConstraint.cs
- WebHostScriptMappingsInstallComponent.cs
- DependsOnAttribute.cs
- ExtenderControl.cs
- _DisconnectOverlappedAsyncResult.cs
- ObjectFactoryCodeDomTreeGenerator.cs
- HistoryEventArgs.cs
- XmlSignatureManifest.cs
- StylusPlugin.cs
- PolygonHotSpot.cs
- ConstraintStruct.cs
- DesignTimeSiteMapProvider.cs
- NetworkInformationException.cs
- WindowsEditBox.cs
- WCFBuildProvider.cs
- WebPartTransformerAttribute.cs
- TextMarkerSource.cs
- ItemCollection.cs
- CodeRegionDirective.cs
- AssemblyContextControlItem.cs
- ReceiveCompletedEventArgs.cs
- Perspective.cs
- WinFormsSpinner.cs
- UnsafeNativeMethodsCLR.cs