Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / NetFx35 / System.ServiceModel.Web / System / ServiceModel / Channels / WebMessageEncoderFactory.cs / 1 / WebMessageEncoderFactory.cs
//---------------------------------------------------------------- // Copyright (c) Microsoft Corporation. All rights reserved. //--------------------------------------------------------------- namespace System.ServiceModel.Channels { using System; using System.Collections.Generic; using System.Globalization; using System.IO; using System.Net.Mime; using System.ServiceModel.Diagnostics; using System.Text; using System.Xml; using System.Net; using System.ServiceModel.Web; class WebMessageEncoderFactory : MessageEncoderFactory { WebMessageEncoder messageEncoder; public WebMessageEncoderFactory(Encoding writeEncoding, int maxReadPoolSize, int maxWritePoolSize, XmlDictionaryReaderQuotas quotas, WebContentTypeMapper contentTypeMapper) { messageEncoder = new WebMessageEncoder(writeEncoding, maxReadPoolSize, maxWritePoolSize, quotas, contentTypeMapper); } public override MessageEncoder Encoder { get { return messageEncoder; } } public override MessageVersion MessageVersion { get { return messageEncoder.MessageVersion; } } class WebMessageEncoder : MessageEncoder { const string defaultMediaType = "application/xml"; WebContentTypeMapper contentTypeMapper; string defaultContentType; MessageEncoder jsonMessageEncoder; int maxReadPoolSize; int maxWritePoolSize; MessageEncoder rawMessageEncoder; XmlDictionaryReaderQuotas readerQuotas; MessageEncoder textMessageEncoder; object thisLock; Encoding writeEncoding; public WebMessageEncoder(Encoding writeEncoding, int maxReadPoolSize, int maxWritePoolSize, XmlDictionaryReaderQuotas quotas, WebContentTypeMapper contentTypeMapper) { if (writeEncoding == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("writeEncoding"); } this.thisLock = new object(); TextEncoderDefaults.ValidateEncoding(writeEncoding); this.writeEncoding = writeEncoding; this.maxReadPoolSize = maxReadPoolSize; this.maxWritePoolSize = maxWritePoolSize; this.contentTypeMapper = contentTypeMapper; this.readerQuotas = new XmlDictionaryReaderQuotas(); quotas.CopyTo(this.readerQuotas); this.defaultContentType = String.Format(CultureInfo.InvariantCulture, "{0}; charset={1}", defaultMediaType, TextEncoderDefaults.EncodingToCharSet(writeEncoding)); } public override string ContentType { get { return this.defaultContentType; } } public override string MediaType { get { return defaultMediaType; } } public override MessageVersion MessageVersion { get { return MessageVersion.None; } } MessageEncoder JsonMessageEncoder { get { if (jsonMessageEncoder == null) { lock (ThisLock) { if (jsonMessageEncoder == null) { jsonMessageEncoder = new JsonMessageEncoderFactory(writeEncoding, maxReadPoolSize, maxWritePoolSize, readerQuotas).Encoder; } } } return jsonMessageEncoder; } } MessageEncoder RawMessageEncoder { get { if (rawMessageEncoder == null) { lock (ThisLock) { if (rawMessageEncoder == null) { rawMessageEncoder = new HttpStreamMessageEncoderFactory(readerQuotas).Encoder; } } } return rawMessageEncoder; } } MessageEncoder TextMessageEncoder { get { if (textMessageEncoder == null) { lock (ThisLock) { if (textMessageEncoder == null) { textMessageEncoder = new TextMessageEncoderFactory(MessageVersion.None, writeEncoding, maxReadPoolSize, maxWritePoolSize, readerQuotas).Encoder; } } } return textMessageEncoder; } } object ThisLock { get { return thisLock; } } public override bool IsContentTypeSupported(string contentType) { if (contentType == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("contentType"); } WebContentFormat messageFormat; if (TryGetContentTypeMapping(contentType, out messageFormat) && (messageFormat != WebContentFormat.Default)) { return true; } return RawMessageEncoder.IsContentTypeSupported(contentType) || JsonMessageEncoder.IsContentTypeSupported(contentType) || TextMessageEncoder.IsContentTypeSupported(contentType); } public override Message ReadMessage(ArraySegmentbuffer, BufferManager bufferManager, string contentType) { if (bufferManager == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentNullException("bufferManager")); } WebContentFormat format = GetFormatForContentType(contentType); Message message; switch (format) { case WebContentFormat.Json: message = JsonMessageEncoder.ReadMessage(buffer, bufferManager, contentType); message.Properties.Add(WebBodyFormatMessageProperty.Name, WebBodyFormatMessageProperty.JsonProperty); break; case WebContentFormat.Xml: message = TextMessageEncoder.ReadMessage(buffer, bufferManager, contentType); message.Properties.Add(WebBodyFormatMessageProperty.Name, WebBodyFormatMessageProperty.XmlProperty); break; case WebContentFormat.Raw: message = RawMessageEncoder.ReadMessage(buffer, bufferManager, contentType); message.Properties.Add(WebBodyFormatMessageProperty.Name, WebBodyFormatMessageProperty.RawProperty); break; default: Fx.Assert("This should never get hit because GetFormatForContentType shouldn't return a WebContentFormat other than Json, Xml, and Raw"); throw DiagnosticUtility.ExceptionUtility.ThrowHelperInternal(false); } return message; } public override Message ReadMessage(Stream stream, int maxSizeOfHeaders, string contentType) { if (stream == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentNullException("stream")); } WebContentFormat format = GetFormatForContentType(contentType); Message message; switch (format) { case WebContentFormat.Json: message = JsonMessageEncoder.ReadMessage(stream, maxSizeOfHeaders, contentType); message.Properties.Add(WebBodyFormatMessageProperty.Name, WebBodyFormatMessageProperty.JsonProperty); break; case WebContentFormat.Xml: message = TextMessageEncoder.ReadMessage(stream, maxSizeOfHeaders, contentType); message.Properties.Add(WebBodyFormatMessageProperty.Name, WebBodyFormatMessageProperty.XmlProperty); break; case WebContentFormat.Raw: message = RawMessageEncoder.ReadMessage(stream, maxSizeOfHeaders, contentType); message.Properties.Add(WebBodyFormatMessageProperty.Name, WebBodyFormatMessageProperty.RawProperty); break; default: Fx.Assert("This should never get hit because GetFormatForContentType shouldn't return a WebContentFormat other than Json, Xml, and Raw"); throw DiagnosticUtility.ExceptionUtility.ThrowHelperInternal(false); } return message; } public override ArraySegment WriteMessage(Message message, int maxMessageSize, BufferManager bufferManager, int messageOffset) { if (message == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentNullException("message")); } if (bufferManager == null) { throw TraceUtility.ThrowHelperError(new ArgumentNullException("bufferManager"), message); } if (maxMessageSize < 0) { throw TraceUtility.ThrowHelperError(new ArgumentOutOfRangeException("maxMessageSize", maxMessageSize, SR2.GetString(SR2.ValueMustBeNonNegative)), message); } if (messageOffset < 0 || messageOffset > maxMessageSize) { throw TraceUtility.ThrowHelperError(new ArgumentOutOfRangeException("messageOffset", messageOffset, SR2.GetString(SR2.JsonValueMustBeInRange, 0, maxMessageSize)), message); } ThrowIfMismatchedMessageVersion(message); WebContentFormat messageFormat = ExtractFormatFromMessage(message); switch (messageFormat) { case WebContentFormat.Json: return JsonMessageEncoder.WriteMessage(message, maxMessageSize, bufferManager, messageOffset); case WebContentFormat.Xml: return TextMessageEncoder.WriteMessage(message, maxMessageSize, bufferManager, messageOffset); case WebContentFormat.Raw: return RawMessageEncoder.WriteMessage(message, maxMessageSize, bufferManager, messageOffset); default: Fx.Assert("This should never get hit because GetFormatForContentType shouldn't return a WebContentFormat other than Json, Xml, and Raw"); throw DiagnosticUtility.ExceptionUtility.ThrowHelperInternal(false); } } public override void WriteMessage(Message message, Stream stream) { if (message == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentNullException("message")); } if (stream == null) { throw TraceUtility.ThrowHelperError(new ArgumentNullException("stream"), message); } ThrowIfMismatchedMessageVersion(message); WebContentFormat messageFormat = ExtractFormatFromMessage(message); switch (messageFormat) { case WebContentFormat.Json: JsonMessageEncoder.WriteMessage(message, stream); break; case WebContentFormat.Xml: TextMessageEncoder.WriteMessage(message, stream); break; case WebContentFormat.Raw: RawMessageEncoder.WriteMessage(message, stream); break; default: Fx.Assert("This should never get hit because GetFormatForContentType shouldn't return a WebContentFormat other than Json, Xml, and Raw"); throw DiagnosticUtility.ExceptionUtility.ThrowHelperInternal(false); } } internal override bool IsCharSetSupported(string charSet) { Encoding tmp; return TextEncoderDefaults.TryGetEncoding(charSet, out tmp); } WebContentFormat ExtractFormatFromMessage(Message message) { object messageFormatProperty; message.Properties.TryGetValue(WebBodyFormatMessageProperty.Name, out messageFormatProperty); if (messageFormatProperty == null) { return WebContentFormat.Xml; } WebBodyFormatMessageProperty typedMessageFormatProperty = messageFormatProperty as WebBodyFormatMessageProperty; if ((typedMessageFormatProperty == null) || (typedMessageFormatProperty.Format == WebContentFormat.Default)) { return WebContentFormat.Xml; } return typedMessageFormatProperty.Format; } WebContentFormat GetFormatForContentType(string contentType) { WebContentFormat messageFormat; if (TryGetContentTypeMapping(contentType, out messageFormat) && (messageFormat != WebContentFormat.Default)) { return messageFormat; } // Don't pass on null content types to IsContentTypeSupported methods -- they might throw. // If null content type isn't already mapped, return the default format of Raw. if (contentType == null) { return WebContentFormat.Raw; } if (JsonMessageEncoder.IsContentTypeSupported(contentType)) { return WebContentFormat.Json; } if (TextMessageEncoder.IsContentTypeSupported(contentType)) { return WebContentFormat.Xml; } return WebContentFormat.Raw; } bool TryGetContentTypeMapping(string contentType, out WebContentFormat format) { if (contentTypeMapper == null) { format = WebContentFormat.Default; return false; } try { format = contentTypeMapper.GetMessageFormatForContentType(contentType); if (!WebContentFormatHelper.IsDefined(format)) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentException(SR2.GetString(SR2.UnknownWebEncodingFormat, contentType, format))); } return true; } catch (Exception e) { if (DiagnosticUtility.IsFatal(e)) { throw; } throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new CommunicationException( SR2.GetString(SR2.ErrorEncounteredInContentTypeMapper), e)); } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
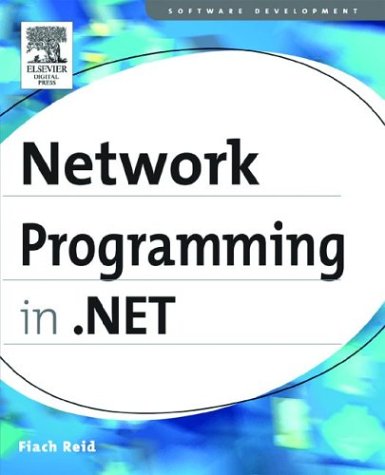
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SafeSystemMetrics.cs
- StylusPointDescription.cs
- SessionStateModule.cs
- x509utils.cs
- ConstraintStruct.cs
- ReturnType.cs
- SmiMetaDataProperty.cs
- __Filters.cs
- RectangleConverter.cs
- ListViewSortEventArgs.cs
- GridItemPatternIdentifiers.cs
- SqlConnectionManager.cs
- XmlSchemaFacet.cs
- FrugalList.cs
- ProvidersHelper.cs
- DockAndAnchorLayout.cs
- InvokePattern.cs
- BeginStoryboard.cs
- FontDifferentiator.cs
- PropertyManager.cs
- SecurityState.cs
- LastQueryOperator.cs
- NativeMethods.cs
- URL.cs
- WebBrowserUriTypeConverter.cs
- PropertyItemInternal.cs
- UInt64.cs
- TemplatePropertyEntry.cs
- MarkupWriter.cs
- DetailsViewPagerRow.cs
- TokenBasedSet.cs
- AvTrace.cs
- RegionIterator.cs
- CodeParameterDeclarationExpression.cs
- ParseChildrenAsPropertiesAttribute.cs
- HostingEnvironment.cs
- NumericUpDownAccelerationCollection.cs
- DataListItem.cs
- GridViewColumn.cs
- ActionFrame.cs
- ObjectConverter.cs
- DocumentPageViewAutomationPeer.cs
- recordstatescratchpad.cs
- PixelFormat.cs
- CaseInsensitiveHashCodeProvider.cs
- UiaCoreApi.cs
- FlowLayout.cs
- Vector3DValueSerializer.cs
- GridPattern.cs
- DependentList.cs
- UICuesEvent.cs
- CodeExpressionCollection.cs
- DataGridViewCellEventArgs.cs
- TimeSpanValidator.cs
- IfElseDesigner.xaml.cs
- SymbolType.cs
- MemberRelationshipService.cs
- SqlPersonalizationProvider.cs
- ClientScriptItemCollection.cs
- ListBox.cs
- FormatException.cs
- SelectedDatesCollection.cs
- DictionaryEntry.cs
- TextPattern.cs
- KerberosTicketHashIdentifierClause.cs
- DependencyPropertyKind.cs
- Stopwatch.cs
- Unit.cs
- ModuleBuilderData.cs
- BitmapEffectDrawing.cs
- MultiViewDesigner.cs
- DataGridColumnCollection.cs
- SafeJobHandle.cs
- SoapElementAttribute.cs
- ThaiBuddhistCalendar.cs
- SqlCachedBuffer.cs
- ModuleBuilderData.cs
- CacheMemory.cs
- UnsafeNativeMethods.cs
- ClrPerspective.cs
- ToolStripItemClickedEventArgs.cs
- Ports.cs
- HttpDictionary.cs
- WSMessageEncoding.cs
- ProfileGroupSettingsCollection.cs
- SmiGettersStream.cs
- DataControlButton.cs
- FunctionParameter.cs
- MarkerProperties.cs
- DaylightTime.cs
- DataMisalignedException.cs
- DecoderFallback.cs
- ISAPIWorkerRequest.cs
- XXXOnTypeBuilderInstantiation.cs
- SemanticBasicElement.cs
- ActiveXHost.cs
- Animatable.cs
- ButtonFlatAdapter.cs
- RequiredFieldValidator.cs
- ZipPackage.cs