Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / WinForms / Managed / System / WinForms / DataGridViewIntLinkedList.cs / 1 / DataGridViewIntLinkedList.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System; using System.Diagnostics; using System.Collections; using System.Windows.Forms; using System.ComponentModel; ////// /// internal class DataGridViewIntLinkedList : IEnumerable { private DataGridViewIntLinkedListElement lastAccessedElement; private DataGridViewIntLinkedListElement headElement; private int count, lastAccessedIndex; ///Represents a linked list of integers ///IEnumerator IEnumerable.GetEnumerator() { return new DataGridViewIntLinkedListEnumerator(this.headElement); } /// public DataGridViewIntLinkedList() { lastAccessedIndex = -1; } /// public DataGridViewIntLinkedList(DataGridViewIntLinkedList source) { Debug.Assert(source != null); int elements = source.Count; for (int element = 0; element < elements; element++) { Add(source[element]); } } /// public int this[int index] { get { Debug.Assert(index >= 0); Debug.Assert(index < this.count); if (this.lastAccessedIndex == -1 || index < this.lastAccessedIndex) { DataGridViewIntLinkedListElement tmp = this.headElement; int tmpIndex = index; while (tmpIndex > 0) { tmp = tmp.Next; tmpIndex--; } this.lastAccessedElement = tmp; this.lastAccessedIndex = index; return tmp.Int; } else { while (this.lastAccessedIndex < index) { this.lastAccessedElement = this.lastAccessedElement.Next; this.lastAccessedIndex++; } return this.lastAccessedElement.Int; } } set { Debug.Assert(index >= 0); if (index != this.lastAccessedIndex) { int currentInt = this[index]; Debug.Assert(index == this.lastAccessedIndex); } this.lastAccessedElement.Int = value; } } /// public int Count { get { return this.count; } } /// public int HeadInt { get { Debug.Assert(this.headElement != null); return this.headElement.Int; } } /// public void Add(int integer) { DataGridViewIntLinkedListElement newHead = new DataGridViewIntLinkedListElement(integer); if (this.headElement != null) { newHead.Next = this.headElement; } this.headElement = newHead; this.count++; this.lastAccessedElement = null; this.lastAccessedIndex = -1; } /// public void Clear() { this.lastAccessedElement = null; this.lastAccessedIndex = -1; this.headElement = null; this.count = 0; } /// public bool Contains(int integer) { int index = 0; DataGridViewIntLinkedListElement tmp = this.headElement; while (tmp != null) { if (tmp.Int == integer) { this.lastAccessedElement = tmp; this.lastAccessedIndex = index; return true; } tmp = tmp.Next; index++; } return false; } /// public int IndexOf(int integer) { if (Contains(integer)) { return this.lastAccessedIndex; } else { return -1; } } /// public bool Remove(int integer) { DataGridViewIntLinkedListElement tmp1 = null, tmp2 = this.headElement; while (tmp2 != null) { if (tmp2.Int == integer) { break; } tmp1 = tmp2; tmp2 = tmp2.Next; } if (tmp2.Int == integer) { DataGridViewIntLinkedListElement tmp3 = tmp2.Next; if (tmp1 == null) { this.headElement = tmp3; } else { tmp1.Next = tmp3; } this.count--; this.lastAccessedElement = null; this.lastAccessedIndex = -1; return true; } return false; } /// public void RemoveAt(int index) { DataGridViewIntLinkedListElement tmp1 = null, tmp2 = this.headElement; while (index > 0) { tmp1 = tmp2; tmp2 = tmp2.Next; index--; } DataGridViewIntLinkedListElement tmp3 = tmp2.Next; if (tmp1 == null) { this.headElement = tmp3; } else { tmp1.Next = tmp3; } this.count--; this.lastAccessedElement = null; this.lastAccessedIndex = -1; } } /// /// /// internal class DataGridViewIntLinkedListEnumerator : IEnumerator { private DataGridViewIntLinkedListElement headElement; private DataGridViewIntLinkedListElement current; private bool reset; ///Represents an emunerator of elements in a ///linked list. public DataGridViewIntLinkedListEnumerator(DataGridViewIntLinkedListElement headElement) { this.headElement = headElement; this.reset = true; } /// object IEnumerator.Current { get { Debug.Assert(this.current != null); // Since this is for internal use only. return this.current.Int; } } /// bool IEnumerator.MoveNext() { if (this.reset) { Debug.Assert(this.current == null); this.current = this.headElement; this.reset = false; } else { Debug.Assert(this.current != null); // Since this is for internal use only. this.current = this.current.Next; } return (this.current != null); } /// void IEnumerator.Reset() { this.reset = true; this.current = null; } } /// /// /// internal class DataGridViewIntLinkedListElement { private int integer; private DataGridViewIntLinkedListElement next; ///Represents an element in a ///linked list. public DataGridViewIntLinkedListElement(int integer) { this.integer = integer; } /// public int Int { get { return this.integer; } set { this.integer = value; } } /// public DataGridViewIntLinkedListElement Next { get { return this.next; } set { this.next = value; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
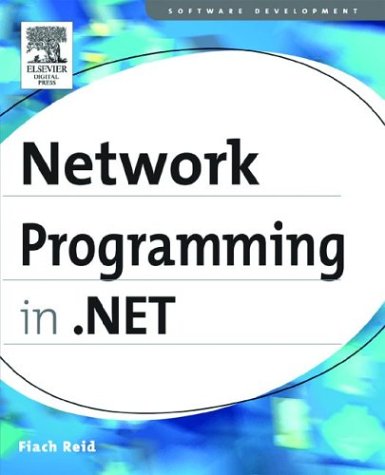
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ResourceSetExpression.cs
- sqlcontext.cs
- EntityDataSourceWrapperPropertyDescriptor.cs
- CodeIterationStatement.cs
- DesignerSerializerAttribute.cs
- AudienceUriMode.cs
- TaskFormBase.cs
- VSDExceptions.cs
- ProfileGroupSettings.cs
- ImpersonationContext.cs
- XPathNodeInfoAtom.cs
- Parser.cs
- MenuItemBindingCollection.cs
- ScriptBehaviorDescriptor.cs
- XamlStream.cs
- InputDevice.cs
- GroupItemAutomationPeer.cs
- BulletedList.cs
- XmlSchemaSimpleContent.cs
- DependsOnAttribute.cs
- PageCache.cs
- Converter.cs
- SoapFault.cs
- SafeFindHandle.cs
- ImageClickEventArgs.cs
- XsltConvert.cs
- CustomValidator.cs
- RequiredFieldValidator.cs
- KnownColorTable.cs
- DesignerTextWriter.cs
- ConstraintManager.cs
- ToolStripActionList.cs
- CngAlgorithmGroup.cs
- Attributes.cs
- Content.cs
- MimeAnyImporter.cs
- BulletChrome.cs
- ResourceProviderFactory.cs
- LinqDataSourceContextEventArgs.cs
- WorkflowApplicationEventArgs.cs
- EmptyEnumerable.cs
- SelectionPattern.cs
- Point3DCollection.cs
- ProcessHostFactoryHelper.cs
- NullableIntAverageAggregationOperator.cs
- UrlRoutingHandler.cs
- BodyGlyph.cs
- ProviderUtil.cs
- SafeHGlobalHandleCritical.cs
- XmlSignatureProperties.cs
- InvalidPrinterException.cs
- _NestedMultipleAsyncResult.cs
- __Filters.cs
- KeyPressEvent.cs
- DataServiceQueryProvider.cs
- DbConnectionHelper.cs
- ReliableSessionBindingElementImporter.cs
- AppDomainCompilerProxy.cs
- DragAssistanceManager.cs
- MetadataPropertyCollection.cs
- DecimalKeyFrameCollection.cs
- DetailsViewDeletedEventArgs.cs
- QuaternionAnimationBase.cs
- Privilege.cs
- VisualCollection.cs
- MdiWindowListItemConverter.cs
- BitmapCache.cs
- TextParagraphCache.cs
- CrossAppDomainChannel.cs
- StyleModeStack.cs
- DependencyProperty.cs
- Win32SafeHandles.cs
- DefaultEventAttribute.cs
- MethodCallConverter.cs
- InfoCardRSAPKCS1SignatureDeformatter.cs
- FtpRequestCacheValidator.cs
- XmlSerializerFaultFormatter.cs
- SaveFileDialog.cs
- DataGridViewRowsRemovedEventArgs.cs
- ScrollItemPatternIdentifiers.cs
- ManipulationDeltaEventArgs.cs
- AsymmetricKeyExchangeDeformatter.cs
- EntityStoreSchemaGenerator.cs
- BitSet.cs
- IPGlobalProperties.cs
- GridViewHeaderRowPresenter.cs
- DynamicRendererThreadManager.cs
- TextDecorationCollectionConverter.cs
- AssociationSet.cs
- IDataContractSurrogate.cs
- ObjectContextServiceProvider.cs
- FileSystemInfo.cs
- CodeAccessPermission.cs
- ObjectSet.cs
- XmlSchemaExporter.cs
- InkPresenter.cs
- RunClient.cs
- XmlSchemaProviderAttribute.cs
- URL.cs
- DbProviderSpecificTypePropertyAttribute.cs