Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx40 / Tools / System.Activities.Presentation / System / Activities / Presentation / Hosting / WindowHelperService.cs / 1482484 / WindowHelperService.cs
//---------------------------------------------------------------- // Copyright (c) Microsoft Corporation. All rights reserved. //--------------------------------------------------------------- namespace System.Activities.Presentation.Hosting { using System; using System.Windows; using System.Windows.Interop; using System.Runtime; using System.Diagnostics.CodeAnalysis; using System.Activities.Presentation.View; public delegate void WindowMessage(int msgId, IntPtr parameter1, IntPtr parameter2 ); [Fx.Tag.XamlVisible(false)] public class WindowHelperService { HwndSource hwndSource; WindowMessage listeners; [SuppressMessage("Microsoft.Naming", "CA1704:IdentifiersShouldBeSpelledCorrectly", Justification = "hwnd is a well known name.")] public WindowHelperService(IntPtr hwnd) { this.ParentWindowHwnd = hwnd; } [SuppressMessage("Microsoft.Naming", "CA1704:IdentifiersShouldBeSpelledCorrectly", Justification = "hwnd is a well known name.")] public IntPtr ParentWindowHwnd { get; private set; } internal FrameworkElement View { get; set; } public bool TrySetWindowOwner(DependencyObject source, Window target) { bool result = false; Fx.Assert(source != null, "Source element cannot be null"); Fx.Assert(target != null, "Target window cannot be null"); if (null != source && null != target) { //try the easy way first Window owner = Window.GetWindow(source); if (null != owner) { target.Owner = owner; result = true; } //no - it didn't work else { IntPtr ownerHwnd = Win32Interop.GetActiveWindow(); if (ownerHwnd == IntPtr.Zero) { ownerHwnd = this.ParentWindowHwnd; } WindowInteropHelper interopHelper = new WindowInteropHelper(target); interopHelper.Owner = ownerHwnd; result = true; } } return result; } public bool RegisterWindowMessageHandler(WindowMessage callback) { bool result = true; if (null == callback) { throw FxTrace.Exception.AsError(new ArgumentNullException("callback")); } //if there are no other callbacks registered - create initial multicast delegate //and hookup message processing if (null == this.listeners) { this.listeners = (WindowMessage)Delegate.Combine(callback); this.HookupMessageProcessing(); } //otherwise, check if callback is not in the list already else { Delegate[] initial = this.listeners.GetInvocationList(); //if it isn't - add it to callback list if (-1 == Array.IndexOf(initial, callback)) { Delegate[] combined = new Delegate[initial.Length + 1]; combined[initial.Length] = callback; Array.Copy(initial, combined, initial.Length); this.listeners = (WindowMessage)Delegate.Combine(combined); } else { result = false; } } return result; } public bool UnregisterWindowMessageHandler(WindowMessage callback) { bool result = false; if (null == callback) { throw FxTrace.Exception.AsError(new ArgumentNullException("callback")); } //if there are any callbacks if (null != this.listeners) { Delegate[] list = this.listeners.GetInvocationList(); //check if given delegate belongs to the list if (-1 != Array.IndexOf (list, callback)) { //if list contained 1 element - there is nobody listening for events - remove hook if (list.Length == 1) { this.hwndSource.RemoveHook(new HwndSourceHook(OnMessage)); } //yes - remove it this.listeners = (WindowMessage)Delegate.Remove(this.listeners, callback); result = true; } } if (!result) { System.Diagnostics.Debug.WriteLine("UnregisterWindowMessageHandler - callback not in list"); } return result; } void HookupMessageProcessing() { //try to create hwnd source object if (null == this.hwndSource) { //first - try to create it using ParentWindow handle if (IntPtr.Zero != this.ParentWindowHwnd) { this.hwndSource = HwndSource.FromHwnd(this.ParentWindowHwnd); } //if didn't succeed - (either handle is null or we are hosted in [....] app) //try to create hwnd source out of designer's view if (null == this.hwndSource) { this.hwndSource = HwndSource.FromVisual(this.View) as HwndSource; } } Fx.Assert(null != this.hwndSource, "HwndSource should not be null!"); if (null != this.hwndSource) { //register for event notifications this.hwndSource.AddHook(new HwndSourceHook(OnMessage)); } } IntPtr OnMessage(IntPtr hwnd, int msgId, IntPtr wParam, IntPtr lParam, ref bool handled) { //notify all listeners about window message this.listeners(msgId, wParam, lParam); return IntPtr.Zero; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
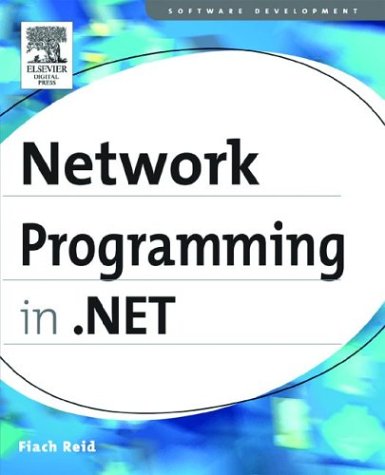
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- srgsitem.cs
- CheckBox.cs
- wmiprovider.cs
- BuildProvidersCompiler.cs
- MouseActionValueSerializer.cs
- LicenseContext.cs
- MarshalDirectiveException.cs
- HighlightComponent.cs
- RankException.cs
- TextBoxLine.cs
- HwndTarget.cs
- CodeAttachEventStatement.cs
- PointKeyFrameCollection.cs
- PageTheme.cs
- CacheRequest.cs
- Deserializer.cs
- DataGridViewRow.cs
- XDRSchema.cs
- SecurityRuntime.cs
- SqlPersonalizationProvider.cs
- CommandExpr.cs
- Image.cs
- basevalidator.cs
- RightsManagementInformation.cs
- TagMapCollection.cs
- controlskin.cs
- TargetParameterCountException.cs
- SubstitutionDesigner.cs
- TypeForwardedToAttribute.cs
- SelectorItemAutomationPeer.cs
- WebSysDescriptionAttribute.cs
- MenuItemBindingCollection.cs
- _NegotiateClient.cs
- TableSectionStyle.cs
- ConnectionPointCookie.cs
- XmlIgnoreAttribute.cs
- SynthesizerStateChangedEventArgs.cs
- SecurityProtocol.cs
- RenderDataDrawingContext.cs
- DefaultClaimSet.cs
- Descriptor.cs
- MSG.cs
- BinaryNode.cs
- GZipDecoder.cs
- Query.cs
- PersistenceException.cs
- QuestionEventArgs.cs
- BamlLocalizabilityResolver.cs
- AuthenticationModuleElement.cs
- GridViewUpdateEventArgs.cs
- StateChangeEvent.cs
- DataSysAttribute.cs
- UIInitializationException.cs
- PermissionSet.cs
- XsltInput.cs
- ArgIterator.cs
- AutomationPattern.cs
- CodeGenerator.cs
- ProtocolsConfigurationHandler.cs
- TabletDeviceInfo.cs
- NativeActivityTransactionContext.cs
- ZipIOExtraFieldZip64Element.cs
- ComNativeDescriptor.cs
- ParseNumbers.cs
- InArgumentConverter.cs
- PageHandlerFactory.cs
- ChtmlFormAdapter.cs
- ConstraintConverter.cs
- DescriptionCreator.cs
- Hash.cs
- DelegatingStream.cs
- SecurityManager.cs
- SR.cs
- HwndMouseInputProvider.cs
- DelegatedStream.cs
- XsltArgumentList.cs
- ControlPropertyNameConverter.cs
- BooleanAnimationBase.cs
- SecurityMessageProperty.cs
- SqlUserDefinedAggregateAttribute.cs
- WindowsComboBox.cs
- CodeLabeledStatement.cs
- LinkLabel.cs
- DataGridViewColumnTypeEditor.cs
- ContainerControlDesigner.cs
- CqlParser.cs
- ConfigXmlSignificantWhitespace.cs
- RawStylusInput.cs
- PropertyGridEditorPart.cs
- DocumentOrderComparer.cs
- CultureTable.cs
- InvalidateEvent.cs
- ResourceExpressionEditorSheet.cs
- TokenCreationParameter.cs
- AuthStoreRoleProvider.cs
- HtmlTableRow.cs
- SourceFilter.cs
- OdbcStatementHandle.cs
- JapaneseCalendar.cs
- XPathItem.cs