Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Speech / Src / Internal / ReadOnlyDictionary.cs / 1 / ReadOnlyDictionary.cs
//------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; namespace System.Speech.Internal { internal class ReadOnlyDictionary: IDictionary { public int Count { get { return _dictionary.Count; } } public IEnumerator > GetEnumerator () { return _dictionary.GetEnumerator (); } public V this [K key] { get { return _dictionary [key]; } set { throw new NotSupportedException (SR.Get (SRID.CollectionReadOnly)); } } // Other methods are a pass through to the underlying collection: public bool IsReadOnly { get { return true; } } public bool Contains (KeyValuePair key) { return _dictionary.ContainsKey (key.Key); } public bool ContainsKey (K key) { return _dictionary.ContainsKey (key); } public void CopyTo (KeyValuePair [] array, int index) { ((ICollection >) _dictionary).CopyTo (array, index); } public ICollection Keys { // According to the source of IDictionary.Keys this is a read-only collection. get { return _dictionary.Keys; } } public ICollection Values { // According to the source of IDictionary.Keys this is a read-write collection, // but is a copy of the main dictionary so there's no way to change anything in the main collection. get { return _dictionary.Values; } } // Read-only collection so throw on these methods: public void Add (KeyValuePair key) { throw new NotSupportedException (SR.Get (SRID.CollectionReadOnly)); } public void Add (K key, V value) { throw new NotSupportedException (SR.Get (SRID.CollectionReadOnly)); } public void Clear () { throw new NotSupportedException (SR.Get (SRID.CollectionReadOnly)); } public bool Remove (KeyValuePair key) { throw new NotSupportedException (SR.Get (SRID.CollectionReadOnly)); } public bool Remove (K key) { throw new NotSupportedException (SR.Get (SRID.CollectionReadOnly)); } IEnumerator IEnumerable.GetEnumerator () { return ((IEnumerable >) this).GetEnumerator (); } bool IDictionary .TryGetValue (K key, out V value) { return InternalDictionary.TryGetValue (key, out value); } // Allow internal code to manipulate internal collection internal Dictionary InternalDictionary { get { return _dictionary; } //set { _dictionary = value; } } private Dictionary _dictionary = new Dictionary (); } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
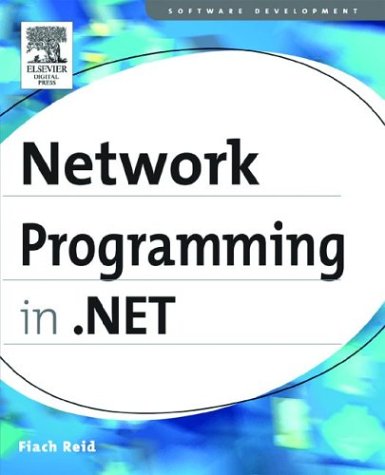
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Graphics.cs
- PropertyGroupDescription.cs
- HealthMonitoringSectionHelper.cs
- SimpleType.cs
- ViewManager.cs
- UnicastIPAddressInformationCollection.cs
- HandledEventArgs.cs
- ConnectorMovedEventArgs.cs
- SoapIncludeAttribute.cs
- GridViewCommandEventArgs.cs
- DateTimeOffset.cs
- MemoryResponseElement.cs
- DrawingCollection.cs
- LinearGradientBrush.cs
- Transform3D.cs
- CodeDelegateCreateExpression.cs
- FormattedTextSymbols.cs
- SqlTransaction.cs
- OuterGlowBitmapEffect.cs
- DataGridTextBoxColumn.cs
- ExpressionDumper.cs
- WindowsAuthenticationModule.cs
- Directory.cs
- TargetInvocationException.cs
- controlskin.cs
- DoubleAnimation.cs
- SqlPersistenceProviderFactory.cs
- TransformConverter.cs
- ISCIIEncoding.cs
- DropShadowBitmapEffect.cs
- CryptoApi.cs
- ErrorFormatter.cs
- ApplicationCommands.cs
- RangeValueProviderWrapper.cs
- AsyncResult.cs
- Literal.cs
- BindingsCollection.cs
- FontSourceCollection.cs
- WebServiceReceiveDesigner.cs
- XmlElementList.cs
- RegexCharClass.cs
- SecurityUtils.cs
- MimeFormatExtensions.cs
- DesignerSerializationVisibilityAttribute.cs
- FileDialog_Vista.cs
- CustomSignedXml.cs
- BuildResultCache.cs
- SynchronizationContext.cs
- AnchoredBlock.cs
- ObjectStateEntryDbUpdatableDataRecord.cs
- WindowsHyperlink.cs
- InProcStateClientManager.cs
- TrackBar.cs
- ExitEventArgs.cs
- DBAsyncResult.cs
- VariableBinder.cs
- TablePatternIdentifiers.cs
- TemplateBindingExpression.cs
- ListViewUpdatedEventArgs.cs
- CompoundFileReference.cs
- EnumerableRowCollectionExtensions.cs
- ThicknessConverter.cs
- OutputCacheSettings.cs
- VirtualizedItemPattern.cs
- TraceHandlerErrorFormatter.cs
- XmlAnyElementAttributes.cs
- BindingNavigator.cs
- SafeArchiveContext.cs
- DataGridViewCellConverter.cs
- TextEffect.cs
- ByteKeyFrameCollection.cs
- EnumerableCollectionView.cs
- initElementDictionary.cs
- AspNetSynchronizationContext.cs
- ManagementEventWatcher.cs
- InvokeFunc.cs
- WebPartManagerInternals.cs
- TemplateControlParser.cs
- ProviderException.cs
- CaseStatementProjectedSlot.cs
- Propagator.cs
- RelatedView.cs
- ItemChangedEventArgs.cs
- ListChangedEventArgs.cs
- CompatibleIComparer.cs
- ValueTypePropertyReference.cs
- BasicBrowserDialog.cs
- ChameleonKey.cs
- Simplifier.cs
- ObjectFactoryCodeDomTreeGenerator.cs
- PreviewPrintController.cs
- SharedPerformanceCounter.cs
- Propagator.JoinPropagator.JoinPredicateVisitor.cs
- PaintValueEventArgs.cs
- TemplateXamlParser.cs
- Point3DCollection.cs
- DockAndAnchorLayout.cs
- StringConcat.cs
- PrintDocument.cs
- ThrowHelper.cs