Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / ndp / fx / src / DataSet / System / Data / EnumerableRowCollectionExtensions.cs / 1 / EnumerableRowCollectionExtensions.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //[....] //----------------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Linq; using System.Linq.Expressions; using System.Globalization; using System.Diagnostics; namespace System.Data { ////// This static class defines the extension methods that add LINQ operator functionality /// within IEnumerableDT and IOrderedEnumerableDT. /// public static class EnumerableRowCollectionExtensions { ////// LINQ's Where operator for generic EnumerableRowCollection. /// public static EnumerableRowCollectionWhere ( this EnumerableRowCollection source, Func predicate) { EnumerableRowCollection edt = new EnumerableRowCollection (source, Enumerable.Where (source, predicate), null); //copy constructor edt.AddPredicate(predicate); return edt; } /// /// LINQ's OrderBy operator for generic EnumerableRowCollection. /// public static OrderedEnumerableRowCollectionOrderBy ( this EnumerableRowCollection source, Func keySelector) { IEnumerable ie = Enumerable.OrderBy (source, keySelector); OrderedEnumerableRowCollection edt = new OrderedEnumerableRowCollection (source, ie); edt.AddSortExpression(keySelector, false, true); return edt; } /// /// LINQ's OrderBy operator for generic EnumerableRowCollection. /// public static OrderedEnumerableRowCollectionOrderBy ( this EnumerableRowCollection source, Func keySelector, IComparer comparer) { IEnumerable ie = Enumerable.OrderBy (source, keySelector, comparer); OrderedEnumerableRowCollection edt = new OrderedEnumerableRowCollection (source, ie); edt.AddSortExpression(keySelector, comparer, false, true); return edt; } /// /// LINQ's OrderByDescending operator for generic EnumerableRowCollection. /// public static OrderedEnumerableRowCollectionOrderByDescending ( this EnumerableRowCollection source, Func keySelector) { IEnumerable ie = Enumerable.OrderByDescending (source, keySelector); OrderedEnumerableRowCollection edt = new OrderedEnumerableRowCollection (source, ie); edt.AddSortExpression(keySelector, true, true); return edt; } /// /// LINQ's OrderByDescending operator for generic EnumerableRowCollection. /// public static OrderedEnumerableRowCollectionOrderByDescending ( this EnumerableRowCollection source, Func keySelector, IComparer comparer) { IEnumerable ie = Enumerable.OrderByDescending (source, keySelector, comparer); OrderedEnumerableRowCollection edt = new OrderedEnumerableRowCollection (source, ie); edt.AddSortExpression(keySelector, comparer, true, true); return edt; } /// /// LINQ's ThenBy operator for generic EnumerableRowCollection. /// public static OrderedEnumerableRowCollectionThenBy ( this OrderedEnumerableRowCollection source, Func keySelector) { IEnumerable ie = Enumerable.ThenBy ((IOrderedEnumerable )source.EnumerableRows, keySelector); OrderedEnumerableRowCollection edt = new OrderedEnumerableRowCollection ((EnumerableRowCollection )source, ie); edt.AddSortExpression(keySelector, /*isDesc*/ false, /*isOrderBy*/ false); return edt; } /// /// LINQ's ThenBy operator for generic EnumerableRowCollection. /// public static OrderedEnumerableRowCollectionThenBy ( this OrderedEnumerableRowCollection source, Func keySelector, IComparer comparer) { IEnumerable ie = Enumerable.ThenBy ((IOrderedEnumerable )source.EnumerableRows, keySelector, comparer); OrderedEnumerableRowCollection edt = new OrderedEnumerableRowCollection ((EnumerableRowCollection )source, ie); edt.AddSortExpression(keySelector, comparer, false, false); return edt; } /// /// LINQ's ThenByDescending operator for generic EnumerableRowCollection. /// public static OrderedEnumerableRowCollectionThenByDescending ( this OrderedEnumerableRowCollection source, Func keySelector) { IEnumerable ie = Enumerable.ThenByDescending ((IOrderedEnumerable )source.EnumerableRows, keySelector); OrderedEnumerableRowCollection edt = new OrderedEnumerableRowCollection ((EnumerableRowCollection )source, ie); edt.AddSortExpression(keySelector, /*desc*/ true, false); return edt; } /// /// LINQ's ThenByDescending operator for generic EnumerableRowCollection. /// public static OrderedEnumerableRowCollectionThenByDescending ( this OrderedEnumerableRowCollection source, Func keySelector, IComparer comparer) { IEnumerable ie = Enumerable.ThenByDescending ((IOrderedEnumerable )source.EnumerableRows, keySelector, comparer); OrderedEnumerableRowCollection edt = new OrderedEnumerableRowCollection ((EnumerableRowCollection )source, ie); edt.AddSortExpression(keySelector, comparer, true, false); return edt; } /// /// Executes a Select (Projection) on EnumerableDataTable. If the selector returns a different /// type than the type of rows, then AsLinqDataView is disabled, and the returning EnumerableDataTable /// represents an enumerable over the LINQ Query. /// public static EnumerableRowCollectionSelect( this EnumerableRowCollection source, Func selector) { //Anonymous type or some other type //The only thing that matters from this point on is _enumerableRows IEnumerable typedEnumerable = Enumerable.Select(source, selector); // Dont need predicates or sort expression from this point on since we know // AsLinqDataView is disabled. return new EnumerableRowCollection (((object)source) as EnumerableRowCollection, typedEnumerable, ((object)selector) as Func); } ////// Casts an EnumerableDataTable_TSource into EnumerableDataTable_TResult /// public static EnumerableRowCollectionCast (this EnumerableRowCollection source) { // Since Cast does not have the signature Cast_T_R(..) this call is routed // through the non-generic base class EnumerableDataTable if ((null != source) && source.ElementType.Equals(typeof(TResult))) { return (EnumerableRowCollection )(object)source; } else { //Anonymous type or some other type //The only thing that matters from this point on is _enumerableRows IEnumerable typedEnumerable = Enumerable.Cast (source); EnumerableRowCollection newEdt = new EnumerableRowCollection ( typedEnumerable, typeof(TResult).IsAssignableFrom(source.ElementType) && typeof(DataRow).IsAssignableFrom(typeof(TResult)), source.Table); return newEdt; } } } //end class } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //[....] //----------------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Linq; using System.Linq.Expressions; using System.Globalization; using System.Diagnostics; namespace System.Data { ////// This static class defines the extension methods that add LINQ operator functionality /// within IEnumerableDT and IOrderedEnumerableDT. /// public static class EnumerableRowCollectionExtensions { ////// LINQ's Where operator for generic EnumerableRowCollection. /// public static EnumerableRowCollectionWhere ( this EnumerableRowCollection source, Func predicate) { EnumerableRowCollection edt = new EnumerableRowCollection (source, Enumerable.Where (source, predicate), null); //copy constructor edt.AddPredicate(predicate); return edt; } /// /// LINQ's OrderBy operator for generic EnumerableRowCollection. /// public static OrderedEnumerableRowCollectionOrderBy ( this EnumerableRowCollection source, Func keySelector) { IEnumerable ie = Enumerable.OrderBy (source, keySelector); OrderedEnumerableRowCollection edt = new OrderedEnumerableRowCollection (source, ie); edt.AddSortExpression(keySelector, false, true); return edt; } /// /// LINQ's OrderBy operator for generic EnumerableRowCollection. /// public static OrderedEnumerableRowCollectionOrderBy ( this EnumerableRowCollection source, Func keySelector, IComparer comparer) { IEnumerable ie = Enumerable.OrderBy (source, keySelector, comparer); OrderedEnumerableRowCollection edt = new OrderedEnumerableRowCollection (source, ie); edt.AddSortExpression(keySelector, comparer, false, true); return edt; } /// /// LINQ's OrderByDescending operator for generic EnumerableRowCollection. /// public static OrderedEnumerableRowCollectionOrderByDescending ( this EnumerableRowCollection source, Func keySelector) { IEnumerable ie = Enumerable.OrderByDescending (source, keySelector); OrderedEnumerableRowCollection edt = new OrderedEnumerableRowCollection (source, ie); edt.AddSortExpression(keySelector, true, true); return edt; } /// /// LINQ's OrderByDescending operator for generic EnumerableRowCollection. /// public static OrderedEnumerableRowCollectionOrderByDescending ( this EnumerableRowCollection source, Func keySelector, IComparer comparer) { IEnumerable ie = Enumerable.OrderByDescending (source, keySelector, comparer); OrderedEnumerableRowCollection edt = new OrderedEnumerableRowCollection (source, ie); edt.AddSortExpression(keySelector, comparer, true, true); return edt; } /// /// LINQ's ThenBy operator for generic EnumerableRowCollection. /// public static OrderedEnumerableRowCollectionThenBy ( this OrderedEnumerableRowCollection source, Func keySelector) { IEnumerable ie = Enumerable.ThenBy ((IOrderedEnumerable )source.EnumerableRows, keySelector); OrderedEnumerableRowCollection edt = new OrderedEnumerableRowCollection ((EnumerableRowCollection )source, ie); edt.AddSortExpression(keySelector, /*isDesc*/ false, /*isOrderBy*/ false); return edt; } /// /// LINQ's ThenBy operator for generic EnumerableRowCollection. /// public static OrderedEnumerableRowCollectionThenBy ( this OrderedEnumerableRowCollection source, Func keySelector, IComparer comparer) { IEnumerable ie = Enumerable.ThenBy ((IOrderedEnumerable )source.EnumerableRows, keySelector, comparer); OrderedEnumerableRowCollection edt = new OrderedEnumerableRowCollection ((EnumerableRowCollection )source, ie); edt.AddSortExpression(keySelector, comparer, false, false); return edt; } /// /// LINQ's ThenByDescending operator for generic EnumerableRowCollection. /// public static OrderedEnumerableRowCollectionThenByDescending ( this OrderedEnumerableRowCollection source, Func keySelector) { IEnumerable ie = Enumerable.ThenByDescending ((IOrderedEnumerable )source.EnumerableRows, keySelector); OrderedEnumerableRowCollection edt = new OrderedEnumerableRowCollection ((EnumerableRowCollection )source, ie); edt.AddSortExpression(keySelector, /*desc*/ true, false); return edt; } /// /// LINQ's ThenByDescending operator for generic EnumerableRowCollection. /// public static OrderedEnumerableRowCollectionThenByDescending ( this OrderedEnumerableRowCollection source, Func keySelector, IComparer comparer) { IEnumerable ie = Enumerable.ThenByDescending ((IOrderedEnumerable )source.EnumerableRows, keySelector, comparer); OrderedEnumerableRowCollection edt = new OrderedEnumerableRowCollection ((EnumerableRowCollection )source, ie); edt.AddSortExpression(keySelector, comparer, true, false); return edt; } /// /// Executes a Select (Projection) on EnumerableDataTable. If the selector returns a different /// type than the type of rows, then AsLinqDataView is disabled, and the returning EnumerableDataTable /// represents an enumerable over the LINQ Query. /// public static EnumerableRowCollectionSelect( this EnumerableRowCollection source, Func selector) { //Anonymous type or some other type //The only thing that matters from this point on is _enumerableRows IEnumerable typedEnumerable = Enumerable.Select(source, selector); // Dont need predicates or sort expression from this point on since we know // AsLinqDataView is disabled. return new EnumerableRowCollection (((object)source) as EnumerableRowCollection, typedEnumerable, ((object)selector) as Func); } ////// Casts an EnumerableDataTable_TSource into EnumerableDataTable_TResult /// public static EnumerableRowCollectionCast (this EnumerableRowCollection source) { // Since Cast does not have the signature Cast_T_R(..) this call is routed // through the non-generic base class EnumerableDataTable if ((null != source) && source.ElementType.Equals(typeof(TResult))) { return (EnumerableRowCollection )(object)source; } else { //Anonymous type or some other type //The only thing that matters from this point on is _enumerableRows IEnumerable typedEnumerable = Enumerable.Cast (source); EnumerableRowCollection newEdt = new EnumerableRowCollection ( typedEnumerable, typeof(TResult).IsAssignableFrom(source.ElementType) && typeof(DataRow).IsAssignableFrom(typeof(TResult)), source.Table); return newEdt; } } } //end class } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
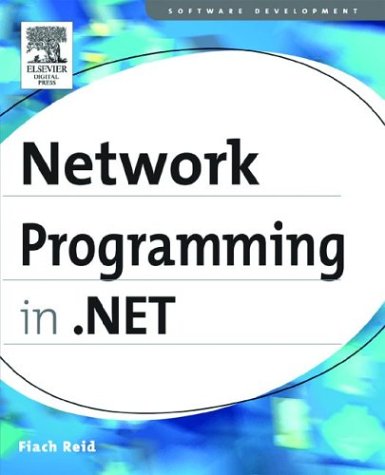
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- RootBuilder.cs
- MetadataAssemblyHelper.cs
- FormattedText.cs
- SemaphoreSlim.cs
- EventLogQuery.cs
- ReadOnlyAttribute.cs
- SecurityDocument.cs
- WebBrowserNavigatingEventHandler.cs
- ItemsControlAutomationPeer.cs
- TextDecorationLocationValidation.cs
- LocationReferenceEnvironment.cs
- HttpDictionary.cs
- _StreamFramer.cs
- Membership.cs
- Geometry.cs
- WSTrustFeb2005.cs
- DynamicQueryableWrapper.cs
- NamedPermissionSet.cs
- AdRotatorDesigner.cs
- MediaCommands.cs
- SqlCacheDependencyDatabaseCollection.cs
- CorrelationTokenInvalidatedHandler.cs
- List.cs
- COSERVERINFO.cs
- Parsers.cs
- XmlAttribute.cs
- CustomWebEventKey.cs
- ParameterModifier.cs
- MenuCommandsChangedEventArgs.cs
- InternalRelationshipCollection.cs
- GridViewRowPresenter.cs
- DataGridViewLinkColumn.cs
- InstrumentationTracker.cs
- PrePrepareMethodAttribute.cs
- TextTreeTextElementNode.cs
- DataBindingValueUIHandler.cs
- DataGridViewColumnHeaderCell.cs
- FormsAuthenticationModule.cs
- DataListDesigner.cs
- safelinkcollection.cs
- DictionarySectionHandler.cs
- ReliableOutputSessionChannel.cs
- ListCardsInFileRequest.cs
- Serializer.cs
- TextProperties.cs
- TokenBasedSet.cs
- SharedPerformanceCounter.cs
- SrgsText.cs
- updatecommandorderer.cs
- WebConfigurationManager.cs
- InkCanvasSelectionAdorner.cs
- TextFindEngine.cs
- Vector3DAnimationUsingKeyFrames.cs
- RepeaterCommandEventArgs.cs
- PhysicalOps.cs
- XmlException.cs
- CharKeyFrameCollection.cs
- WindowsServiceCredential.cs
- InputDevice.cs
- Peer.cs
- ProtocolsConfigurationHandler.cs
- wgx_render.cs
- DecimalConstantAttribute.cs
- SafeArrayRankMismatchException.cs
- ConfigurationProperty.cs
- OleDbPermission.cs
- ImageBrush.cs
- IisTraceWebEventProvider.cs
- WebPartConnectionCollection.cs
- SAPICategories.cs
- MaskInputRejectedEventArgs.cs
- RawMouseInputReport.cs
- NonVisualControlAttribute.cs
- CharacterMetricsDictionary.cs
- KeyGesture.cs
- Padding.cs
- SecurityKeyUsage.cs
- IgnoreFlushAndCloseStream.cs
- RegionIterator.cs
- TimelineCollection.cs
- XmlUTF8TextReader.cs
- Pen.cs
- ManagedIStream.cs
- BCryptNative.cs
- precedingsibling.cs
- Delegate.cs
- SqlClientWrapperSmiStream.cs
- Profiler.cs
- TextHidden.cs
- CompilerError.cs
- ObjectTypeMapping.cs
- DESCryptoServiceProvider.cs
- DataKeyCollection.cs
- DBCommand.cs
- ListBox.cs
- TypeDelegator.cs
- PartialCachingControl.cs
- WebPartDescription.cs
- RawStylusActions.cs
- AuthenticationException.cs