Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Data / System / Data / Filter / DataExpression.cs / 1305376 / DataExpression.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //[....] //[....] //----------------------------------------------------------------------------- namespace System.Data { using System; using System.Diagnostics; using System.Collections.Generic; using System.ComponentModel; using System.Data.SqlTypes; using System.Data.Common; internal sealed class DataExpression : IFilter { internal string originalExpression = null; // original, unoptimized string private bool parsed = false; private bool bound = false; private ExpressionNode expr = null; private DataTable table = null; private readonly StorageType _storageType; private readonly Type _dataType; // This set if the expression is part of ExpressionCoulmn private DataColumn[] dependency = DataTable.zeroColumns; internal DataExpression(DataTable table, string expression) : this(table, expression, null) { } internal DataExpression(DataTable table, string expression, Type type) { ExpressionParser parser = new ExpressionParser(table); parser.LoadExpression(expression); originalExpression = expression; expr = null; if (expression != null) { _storageType = DataStorage.GetStorageType(type); if (_storageType == StorageType.BigInteger) { throw ExprException.UnsupportedDataType(type); } _dataType = type; expr = parser.Parse(); parsed = true; if (expr != null && table != null) { this.Bind(table); } else { bound = false; } } } internal string Expression { get { return (originalExpression != null ? originalExpression : ""); // } } internal ExpressionNode ExpressionNode { get { return expr; } } internal bool HasValue { get { return (null != expr); } } internal void Bind(DataTable table) { this.table = table; if (table == null) return; if (expr != null) { Debug.Assert(parsed, "Invalid calling order: Bind() before Parse()"); Listlist = new List (); expr.Bind(table, list); expr = expr.Optimize(); this.table = table; bound = true; dependency = list.ToArray(); } } internal bool DependsOn(DataColumn column) { if (expr != null) { return expr.DependsOn(column); } else { return false; } } internal object Evaluate() { return Evaluate((DataRow)null, DataRowVersion.Default); } internal object Evaluate(DataRow row, DataRowVersion version) { object result; if (!bound) { this.Bind(this.table); } if (expr != null) { result = expr.Eval(row, version); // if the type is a SqlType (StorageType.Uri < _storageType), convert DBNull values. if (result != DBNull.Value || StorageType.Uri < _storageType) { // we need to convert the return value to the column.Type; try { if (StorageType.Object != _storageType) { result = SqlConvert.ChangeType2(result, _storageType, _dataType, table.FormatProvider); } } catch (Exception e) { // if (!ADP.IsCatchableExceptionType(e)) { throw; } ExceptionBuilder.TraceExceptionForCapture(e); // throw ExprException.DatavalueConvertion(result, _dataType, e); } } } else { result = null; } return result; } internal object Evaluate(DataRow[] rows) { return Evaluate(rows, DataRowVersion.Default); } internal object Evaluate(DataRow[] rows, DataRowVersion version) { if (!bound) { this.Bind(this.table); } if (expr != null) { List recordList = new List (); foreach(DataRow row in rows) { if (row.RowState == DataRowState.Deleted) continue; if (version == DataRowVersion.Original && row.oldRecord == -1) continue; recordList.Add(row.GetRecordFromVersion(version)); } int[] records = recordList.ToArray(); return expr.Eval(records); } else { return DBNull.Value; } } public bool Invoke(DataRow row, DataRowVersion version) { if (expr == null) return true; if (row == null) { throw ExprException.InvokeArgument(); } object val = expr.Eval(row, version); bool result; try { result = ToBoolean(val); } catch (EvaluateException) { throw ExprException.FilterConvertion(Expression); } return result; } internal DataColumn[] GetDependency() { Debug.Assert(dependency != null, "GetDependencies: null, we should have created an empty list"); return dependency; } internal bool IsTableAggregate() { if (expr != null) return expr.IsTableConstant(); else return false; } internal static bool IsUnknown(object value) { return DataStorage.IsObjectNull(value); } internal bool HasLocalAggregate() { if (expr != null) return expr.HasLocalAggregate(); else return false; } internal bool HasRemoteAggregate() { if (expr != null) return expr.HasRemoteAggregate(); else return false; } internal static bool ToBoolean(object value) { if (IsUnknown(value)) return false; if (value is bool) return(bool)value; if (value is SqlBoolean){ return (((SqlBoolean)value).IsTrue); } //check for SqlString is not added, value for true and false should be given with String, not with SqlString if (value is string) { try { return Boolean.Parse((string)value); } catch (Exception e) { // if (!ADP.IsCatchableExceptionType(e)) { throw; } ExceptionBuilder.TraceExceptionForCapture(e); // throw ExprException.DatavalueConvertion(value, typeof(bool), e); } } throw ExprException.DatavalueConvertion(value, typeof(bool), null); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
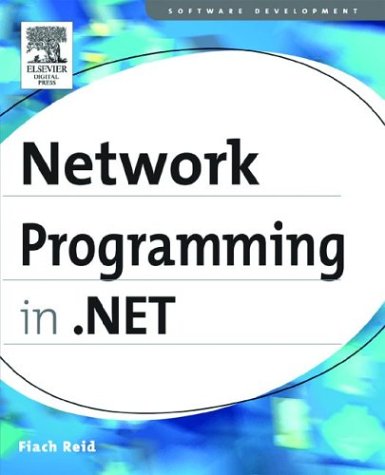
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TemplateInstanceAttribute.cs
- SetterBaseCollection.cs
- TreeNodeConverter.cs
- AttributeSetAction.cs
- PropertySourceInfo.cs
- ConnectionManagementSection.cs
- Input.cs
- SQLInt32Storage.cs
- XmlTextAttribute.cs
- X509ScopedServiceCertificateElement.cs
- SourceInterpreter.cs
- XmlSchemaAnyAttribute.cs
- MergeExecutor.cs
- PropertyDescriptorCollection.cs
- TaskHelper.cs
- FilterQueryOptionExpression.cs
- HelpInfo.cs
- AuthorizationRuleCollection.cs
- AutomationAttributeInfo.cs
- TableLayoutColumnStyleCollection.cs
- WebServiceHost.cs
- XPathNodePointer.cs
- BaseAutoFormat.cs
- JsonStringDataContract.cs
- SafeThreadHandle.cs
- DataGridViewControlCollection.cs
- Rights.cs
- WorkflowInstance.cs
- ContentTextAutomationPeer.cs
- AxHost.cs
- RuntimeConfigLKG.cs
- ProcessModuleCollection.cs
- WrappedKeySecurityTokenParameters.cs
- UniqueConstraint.cs
- TextEndOfParagraph.cs
- DateTimeFormat.cs
- SqlEnums.cs
- RenderingBiasValidation.cs
- DependencyObject.cs
- OletxEnlistment.cs
- Binding.cs
- StreamAsIStream.cs
- PasswordTextContainer.cs
- AsyncCodeActivityContext.cs
- LifetimeServices.cs
- RawStylusSystemGestureInputReport.cs
- WinFormsComponentEditor.cs
- FixedElement.cs
- TextEndOfSegment.cs
- DynamicPropertyHolder.cs
- HtmlShimManager.cs
- DemultiplexingDispatchMessageFormatter.cs
- CryptoApi.cs
- RightsManagementManager.cs
- ContextMenuAutomationPeer.cs
- RotateTransform3D.cs
- DataBindingCollectionEditor.cs
- WebPartConnectionsConfigureVerb.cs
- PriorityBinding.cs
- SqlUDTStorage.cs
- CodeNamespaceCollection.cs
- OutputCacheSettings.cs
- XamlBuildProvider.cs
- COM2IManagedPerPropertyBrowsingHandler.cs
- CompletionProxy.cs
- BigInt.cs
- TempFiles.cs
- FixedSOMGroup.cs
- MobileControlBuilder.cs
- Point.cs
- BorderGapMaskConverter.cs
- FilterElement.cs
- SafeRegistryHandle.cs
- BitHelper.cs
- ComboBoxItem.cs
- GridErrorDlg.cs
- DoubleMinMaxAggregationOperator.cs
- UInt16Converter.cs
- BitmapEffectInputData.cs
- OleDbRowUpdatingEvent.cs
- FormatterServices.cs
- CriticalHandle.cs
- TaiwanCalendar.cs
- ListViewGroup.cs
- ConfigurationErrorsException.cs
- HealthMonitoringSection.cs
- StringAnimationUsingKeyFrames.cs
- ShaperBuffers.cs
- CodeCatchClause.cs
- ViewManager.cs
- ZipIOCentralDirectoryDigitalSignature.cs
- AsyncPostBackTrigger.cs
- SetUserLanguageRequest.cs
- ModifierKeysValueSerializer.cs
- ShadowGlyph.cs
- ToolbarAUtomationPeer.cs
- UnaryQueryOperator.cs
- ObjectContextServiceProvider.cs
- SizeAnimationClockResource.cs
- CheckedPointers.cs