Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / clr / src / BCL / System / Runtime / Serialization / FormatterServices.cs / 1 / FormatterServices.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== /*============================================================ ** ** Class: FormatterServices ** ** ** Purpose: Provides some static methods to aid with the implementation ** of a Formatter for Serialization. ** ** ============================================================*/ namespace System.Runtime.Serialization { using System; using System.Reflection; using System.Reflection.Cache; using System.Collections; using System.Collections.Generic; using System.Security; using System.Security.Permissions; using System.Runtime.Serialization.Formatters; using System.Runtime.Remoting; using System.Runtime.CompilerServices; using System.Threading; using System.IO; using System.Text; using System.Globalization; [System.Runtime.InteropServices.ComVisible(true)] public sealed class FormatterServices { internal static Dictionarym_MemberInfoTable = new Dictionary (32); private static Object s_FormatterServicesSyncObject = null; private static Object formatterServicesSyncObject { get { if (s_FormatterServicesSyncObject == null) { Object o = new Object(); Interlocked.CompareExchange(ref s_FormatterServicesSyncObject, o, null); } return s_FormatterServicesSyncObject; } } private FormatterServices() { throw new NotSupportedException(); } private static MemberInfo[] GetSerializableMembers(RuntimeType type) { // get the list of all fields FieldInfo[] fields = type.GetFields(BindingFlags.Public | BindingFlags.NonPublic | BindingFlags.Instance); int countProper = 0; for (int i = 0; i < fields.Length; i++) { if ((fields[i].Attributes & FieldAttributes.NotSerialized) == FieldAttributes.NotSerialized) continue; countProper++; } if (countProper != fields.Length) { FieldInfo[] properFields = new FieldInfo[countProper]; countProper = 0; for (int i = 0; i < fields.Length; i++) { if ((fields[i].Attributes & FieldAttributes.NotSerialized) == FieldAttributes.NotSerialized) continue; properFields[countProper] = fields[i]; countProper++; } return properFields; } else return fields; } private static bool CheckSerializable(RuntimeType type) { if (type.IsSerializable) { return true; } return false; } private static MemberInfo[] InternalGetSerializableMembers(RuntimeType type) { ArrayList allMembers=null; MemberInfo[] typeMembers; FieldInfo [] typeFields; RuntimeType parentType; BCLDebug.Assert(type!=null, "[GetAllSerializableMembers]type!=null"); //< if (type.IsInterface) { return new MemberInfo[0]; } if (!(CheckSerializable(type))) { throw new SerializationException(String.Format(CultureInfo.CurrentCulture, Environment.GetResourceString("Serialization_NonSerType"), type.FullName, type.Module.Assembly.FullName)); } //Get all of the serializable members in the class to be serialized. typeMembers = GetSerializableMembers(type); //If this class doesn't extend directly from object, walk its hierarchy and //get all of the private and assembly-access fields (e.g. all fields that aren't //virtual) and include them in the list of things to be serialized. parentType = (RuntimeType)(type.BaseType); if (parentType!=null && parentType!=typeof(Object)) { Type[] parentTypes = null; int parentTypeCount = 0; bool classNamesUnique = GetParentTypes(parentType, out parentTypes, out parentTypeCount); if (parentTypeCount > 0){ allMembers = new ArrayList(); for (int i = 0; i < parentTypeCount;i++){ parentType = (RuntimeType)parentTypes[i]; if (!CheckSerializable(parentType)) { throw new SerializationException(String.Format(CultureInfo.CurrentCulture, Environment.GetResourceString("Serialization_NonSerType"), parentType.FullName, parentType.Module.Assembly.FullName)); } typeFields = parentType.GetFields(BindingFlags.NonPublic | BindingFlags.Instance); String typeName = classNamesUnique ? parentType.Name : parentType.FullName; foreach (FieldInfo field in typeFields) { // Family and Assembly fields will be gathered by the type itself. if (!field.IsNotSerialized) { allMembers.Add(new SerializationFieldInfo((RuntimeFieldInfo)field, typeName)); } } } //If we actually found any new MemberInfo's, we need to create a new MemberInfo array and //copy all of the members which we've found so far into that. if (allMembers!=null && allMembers.Count>0) { MemberInfo[] membersTemp = new MemberInfo[allMembers.Count + typeMembers.Length]; Array.Copy(typeMembers, membersTemp, typeMembers.Length); allMembers.CopyTo(membersTemp, typeMembers.Length); typeMembers = membersTemp; } } } return typeMembers; } static bool GetParentTypes(Type parentType, out Type[] parentTypes, out int parentTypeCount){ //Check if there are any dup class names. Then we need to include as part of //typeName to prefix the Field names in SerializationFieldInfo /*out*/ parentTypes = null; /*out*/ parentTypeCount = 0; bool unique = true; for(Type t1 = parentType;t1 != typeof(object); t1 = t1.BaseType){ if (t1.IsInterface) continue; string t1Name = t1.Name; for(int i=0;unique && i m_MemberInfoTable = new Dictionary (32); private static Object s_FormatterServicesSyncObject = null; private static Object formatterServicesSyncObject { get { if (s_FormatterServicesSyncObject == null) { Object o = new Object(); Interlocked.CompareExchange(ref s_FormatterServicesSyncObject, o, null); } return s_FormatterServicesSyncObject; } } private FormatterServices() { throw new NotSupportedException(); } private static MemberInfo[] GetSerializableMembers(RuntimeType type) { // get the list of all fields FieldInfo[] fields = type.GetFields(BindingFlags.Public | BindingFlags.NonPublic | BindingFlags.Instance); int countProper = 0; for (int i = 0; i < fields.Length; i++) { if ((fields[i].Attributes & FieldAttributes.NotSerialized) == FieldAttributes.NotSerialized) continue; countProper++; } if (countProper != fields.Length) { FieldInfo[] properFields = new FieldInfo[countProper]; countProper = 0; for (int i = 0; i < fields.Length; i++) { if ((fields[i].Attributes & FieldAttributes.NotSerialized) == FieldAttributes.NotSerialized) continue; properFields[countProper] = fields[i]; countProper++; } return properFields; } else return fields; } private static bool CheckSerializable(RuntimeType type) { if (type.IsSerializable) { return true; } return false; } private static MemberInfo[] InternalGetSerializableMembers(RuntimeType type) { ArrayList allMembers=null; MemberInfo[] typeMembers; FieldInfo [] typeFields; RuntimeType parentType; BCLDebug.Assert(type!=null, "[GetAllSerializableMembers]type!=null"); //< if (type.IsInterface) { return new MemberInfo[0]; } if (!(CheckSerializable(type))) { throw new SerializationException(String.Format(CultureInfo.CurrentCulture, Environment.GetResourceString("Serialization_NonSerType"), type.FullName, type.Module.Assembly.FullName)); } //Get all of the serializable members in the class to be serialized. typeMembers = GetSerializableMembers(type); //If this class doesn't extend directly from object, walk its hierarchy and //get all of the private and assembly-access fields (e.g. all fields that aren't //virtual) and include them in the list of things to be serialized. parentType = (RuntimeType)(type.BaseType); if (parentType!=null && parentType!=typeof(Object)) { Type[] parentTypes = null; int parentTypeCount = 0; bool classNamesUnique = GetParentTypes(parentType, out parentTypes, out parentTypeCount); if (parentTypeCount > 0){ allMembers = new ArrayList(); for (int i = 0; i < parentTypeCount;i++){ parentType = (RuntimeType)parentTypes[i]; if (!CheckSerializable(parentType)) { throw new SerializationException(String.Format(CultureInfo.CurrentCulture, Environment.GetResourceString("Serialization_NonSerType"), parentType.FullName, parentType.Module.Assembly.FullName)); } typeFields = parentType.GetFields(BindingFlags.NonPublic | BindingFlags.Instance); String typeName = classNamesUnique ? parentType.Name : parentType.FullName; foreach (FieldInfo field in typeFields) { // Family and Assembly fields will be gathered by the type itself. if (!field.IsNotSerialized) { allMembers.Add(new SerializationFieldInfo((RuntimeFieldInfo)field, typeName)); } } } //If we actually found any new MemberInfo's, we need to create a new MemberInfo array and //copy all of the members which we've found so far into that. if (allMembers!=null && allMembers.Count>0) { MemberInfo[] membersTemp = new MemberInfo[allMembers.Count + typeMembers.Length]; Array.Copy(typeMembers, membersTemp, typeMembers.Length); allMembers.CopyTo(membersTemp, typeMembers.Length); typeMembers = membersTemp; } } } return typeMembers; } static bool GetParentTypes(Type parentType, out Type[] parentTypes, out int parentTypeCount){ //Check if there are any dup class names. Then we need to include as part of //typeName to prefix the Field names in SerializationFieldInfo /*out*/ parentTypes = null; /*out*/ parentTypeCount = 0; bool unique = true; for(Type t1 = parentType;t1 != typeof(object); t1 = t1.BaseType){ if (t1.IsInterface) continue; string t1Name = t1.Name; for(int i=0;unique && i
Link Menu
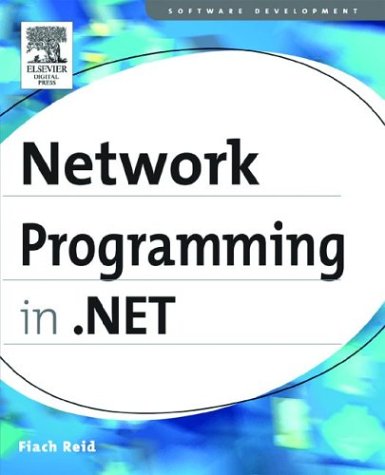
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XamlSerializerUtil.cs
- Padding.cs
- TrustExchangeException.cs
- DataSysAttribute.cs
- fixedPageContentExtractor.cs
- FormParameter.cs
- FormsAuthenticationModule.cs
- UpdateExpressionVisitor.cs
- SqlInternalConnectionSmi.cs
- DataRecord.cs
- DataControlFieldsEditor.cs
- cache.cs
- CompositeScriptReference.cs
- NavigateEvent.cs
- Number.cs
- XmlHierarchicalEnumerable.cs
- FixedTextBuilder.cs
- EngineSiteSapi.cs
- SqlBulkCopy.cs
- ValidationSummaryDesigner.cs
- LicenseManager.cs
- ToolStrip.cs
- DataStreams.cs
- CrossAppDomainChannel.cs
- TextTreeInsertElementUndoUnit.cs
- OleDbDataReader.cs
- SpellerStatusTable.cs
- StringHandle.cs
- TimeStampChecker.cs
- WebPartUserCapability.cs
- ConnectionPoint.cs
- HtmlLink.cs
- ControlBindingsCollection.cs
- GridEntryCollection.cs
- AlignmentXValidation.cs
- DPAPIProtectedConfigurationProvider.cs
- DetailsViewUpdatedEventArgs.cs
- CacheRequest.cs
- TranslateTransform3D.cs
- HTTPNotFoundHandler.cs
- RelationHandler.cs
- log.cs
- ParameterElementCollection.cs
- CmsUtils.cs
- AutomationPatternInfo.cs
- ListDependantCardsRequest.cs
- CodeGeneratorOptions.cs
- HtmlShimManager.cs
- ProtocolsConfiguration.cs
- ConfigurationSection.cs
- OleDbFactory.cs
- ThreadStateException.cs
- RemotingServices.cs
- RegexMatch.cs
- SqlMethodTransformer.cs
- QuadraticBezierSegment.cs
- ClientBuildManager.cs
- CodeTypeMember.cs
- ModifierKeysValueSerializer.cs
- TagPrefixCollection.cs
- Expression.cs
- BufferedGraphics.cs
- ObservableCollection.cs
- LongValidator.cs
- ChannelManager.cs
- SignatureGenerator.cs
- DependsOnAttribute.cs
- BamlLocalizableResource.cs
- ActionItem.cs
- AuthenticationSection.cs
- DesignerDataColumn.cs
- OutOfProcStateClientManager.cs
- PreviewPageInfo.cs
- EntityAdapter.cs
- FunctionCommandText.cs
- CompositeActivityValidator.cs
- WindowsButton.cs
- EventSinkActivityDesigner.cs
- BinHexEncoder.cs
- GridSplitterAutomationPeer.cs
- CopyOfAction.cs
- DataGridState.cs
- WindowProviderWrapper.cs
- WindowsFormsEditorServiceHelper.cs
- AutomationIdentifierGuids.cs
- XmlValidatingReader.cs
- COM2IProvidePropertyBuilderHandler.cs
- XmlAnyElementAttributes.cs
- AttachedPropertyBrowsableForChildrenAttribute.cs
- WorkflowOperationAsyncResult.cs
- DbTransaction.cs
- DataGridViewColumnStateChangedEventArgs.cs
- ViewgenContext.cs
- RawStylusInputCustomData.cs
- PriorityQueue.cs
- HttpWebResponse.cs
- DefaultSerializationProviderAttribute.cs
- CreateRefExpr.cs
- BatchServiceHost.cs
- Wildcard.cs