Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / fx / src / xsp / System / Web / Configuration / CustomErrorsSection.cs / 1 / CustomErrorsSection.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Configuration { using System; using System.Xml; using System.Configuration; using System.Collections.Specialized; using System.Collections; using System.IO; using System.Text; using System.Globalization; using System.Web.Util; using System.Web.Configuration; using System.Security.Permissions; /* From Machine.Config*/ [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] public sealed class CustomErrorsSection : ConfigurationSection { private static ConfigurationPropertyCollection _properties; private static readonly ConfigurationProperty _propDefaultRedirect = new ConfigurationProperty("defaultRedirect", typeof(string), null, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propRedirectMode = new ConfigurationProperty("redirectMode", typeof(CustomErrorsRedirectMode), CustomErrorsRedirectMode.ResponseRedirect, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propMode = new ConfigurationProperty("mode", typeof(CustomErrorsMode), CustomErrorsMode.RemoteOnly, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propErrors = new ConfigurationProperty(null, typeof(CustomErrorCollection), null, ConfigurationPropertyOptions.IsDefaultCollection); private string basepath = null; private string _DefaultAbsolutePath = null; private static CustomErrorsSection _default = null; static CustomErrorsSection() { // Property initialization _properties = new ConfigurationPropertyCollection(); _properties.Add(_propDefaultRedirect); _properties.Add(_propRedirectMode); _properties.Add(_propMode); _properties.Add(_propErrors); } public CustomErrorsSection() { } protected override ConfigurationPropertyCollection Properties { get { return _properties; } } [ConfigurationProperty("defaultRedirect")] public string DefaultRedirect { get { return (string)base[_propDefaultRedirect]; } set { base[_propDefaultRedirect] = value; } } [ConfigurationProperty("redirectMode", DefaultValue = CustomErrorsRedirectMode.ResponseRedirect)] public CustomErrorsRedirectMode RedirectMode { get { return (CustomErrorsRedirectMode)base[_propRedirectMode]; } set { base[_propRedirectMode] = value; } } [ConfigurationProperty("mode", DefaultValue = CustomErrorsMode.RemoteOnly)] public CustomErrorsMode Mode { get { return (CustomErrorsMode)base[_propMode]; } set { base[_propMode] = value; } } [ConfigurationProperty("", IsDefaultCollection = true)] public CustomErrorCollection Errors { get { return (CustomErrorCollection)base[_propErrors]; } } internal String DefaultAbsolutePath { get { if (_DefaultAbsolutePath == null) { _DefaultAbsolutePath = GetAbsoluteRedirect(DefaultRedirect, basepath); } return _DefaultAbsolutePath; } } internal String GetRedirectString(int code) { String r = null; if (Errors != null) { CustomError ce = Errors[(string)code.ToString(CultureInfo.InvariantCulture)]; if (ce != null) r = GetAbsoluteRedirect(ce.Redirect, basepath); } if (r == null) { r = DefaultAbsolutePath; } return r; } protected override void Reset(ConfigurationElement parentElement) { base.Reset(parentElement); CustomErrorsSection parent = parentElement as CustomErrorsSection; if (parent != null) { basepath = parent.basepath; } } protected override void DeserializeSection(XmlReader reader) { WebContext context; base.DeserializeSection(reader); // Determine Web Context context = EvaluationContext.HostingContext as WebContext; if (context != null) { basepath = UrlPath.AppendSlashToPathIfNeeded(context.Path); } } // // helper to create absolute redirect // internal static String GetAbsoluteRedirect(String path, String basePath) { if (path != null && UrlPath.IsRelativeUrl(path)) { if (String.IsNullOrEmpty(basePath)) basePath = "/"; path = UrlPath.Combine(basePath, path); } return path; } internal static CustomErrorsSection GetSettings(HttpContext context) { return GetSettings(context, false); } internal static CustomErrorsSection GetSettings(HttpContext context, bool canThrow) { CustomErrorsSection ce = null; RuntimeConfig runtimeConfig = null; if (canThrow) { runtimeConfig = RuntimeConfig.GetConfig(context); if (runtimeConfig != null) { ce = runtimeConfig.CustomErrors; } } else { runtimeConfig = RuntimeConfig.GetLKGConfig(context); if (runtimeConfig != null) { ce = runtimeConfig.CustomErrors; } if (ce == null) { if (_default == null) { _default = new CustomErrorsSection(); } ce = _default; } } return ce; } internal bool CustomErrorsEnabled(HttpRequest request) { // This could throw if the config file is malformed, but we don't want // to throw from here, as it would mess up error handling try { // Always turn of custom errors in retail deployment mode (DevDiv 36396) if (DeploymentSection.RetailInternal) return true; } catch { } switch (Mode) { case CustomErrorsMode.Off: return false; case CustomErrorsMode.On: return true; case CustomErrorsMode.RemoteOnly: return (!request.IsLocal); default: return false; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Configuration { using System; using System.Xml; using System.Configuration; using System.Collections.Specialized; using System.Collections; using System.IO; using System.Text; using System.Globalization; using System.Web.Util; using System.Web.Configuration; using System.Security.Permissions; /* From Machine.Config*/ [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] public sealed class CustomErrorsSection : ConfigurationSection { private static ConfigurationPropertyCollection _properties; private static readonly ConfigurationProperty _propDefaultRedirect = new ConfigurationProperty("defaultRedirect", typeof(string), null, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propRedirectMode = new ConfigurationProperty("redirectMode", typeof(CustomErrorsRedirectMode), CustomErrorsRedirectMode.ResponseRedirect, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propMode = new ConfigurationProperty("mode", typeof(CustomErrorsMode), CustomErrorsMode.RemoteOnly, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propErrors = new ConfigurationProperty(null, typeof(CustomErrorCollection), null, ConfigurationPropertyOptions.IsDefaultCollection); private string basepath = null; private string _DefaultAbsolutePath = null; private static CustomErrorsSection _default = null; static CustomErrorsSection() { // Property initialization _properties = new ConfigurationPropertyCollection(); _properties.Add(_propDefaultRedirect); _properties.Add(_propRedirectMode); _properties.Add(_propMode); _properties.Add(_propErrors); } public CustomErrorsSection() { } protected override ConfigurationPropertyCollection Properties { get { return _properties; } } [ConfigurationProperty("defaultRedirect")] public string DefaultRedirect { get { return (string)base[_propDefaultRedirect]; } set { base[_propDefaultRedirect] = value; } } [ConfigurationProperty("redirectMode", DefaultValue = CustomErrorsRedirectMode.ResponseRedirect)] public CustomErrorsRedirectMode RedirectMode { get { return (CustomErrorsRedirectMode)base[_propRedirectMode]; } set { base[_propRedirectMode] = value; } } [ConfigurationProperty("mode", DefaultValue = CustomErrorsMode.RemoteOnly)] public CustomErrorsMode Mode { get { return (CustomErrorsMode)base[_propMode]; } set { base[_propMode] = value; } } [ConfigurationProperty("", IsDefaultCollection = true)] public CustomErrorCollection Errors { get { return (CustomErrorCollection)base[_propErrors]; } } internal String DefaultAbsolutePath { get { if (_DefaultAbsolutePath == null) { _DefaultAbsolutePath = GetAbsoluteRedirect(DefaultRedirect, basepath); } return _DefaultAbsolutePath; } } internal String GetRedirectString(int code) { String r = null; if (Errors != null) { CustomError ce = Errors[(string)code.ToString(CultureInfo.InvariantCulture)]; if (ce != null) r = GetAbsoluteRedirect(ce.Redirect, basepath); } if (r == null) { r = DefaultAbsolutePath; } return r; } protected override void Reset(ConfigurationElement parentElement) { base.Reset(parentElement); CustomErrorsSection parent = parentElement as CustomErrorsSection; if (parent != null) { basepath = parent.basepath; } } protected override void DeserializeSection(XmlReader reader) { WebContext context; base.DeserializeSection(reader); // Determine Web Context context = EvaluationContext.HostingContext as WebContext; if (context != null) { basepath = UrlPath.AppendSlashToPathIfNeeded(context.Path); } } // // helper to create absolute redirect // internal static String GetAbsoluteRedirect(String path, String basePath) { if (path != null && UrlPath.IsRelativeUrl(path)) { if (String.IsNullOrEmpty(basePath)) basePath = "/"; path = UrlPath.Combine(basePath, path); } return path; } internal static CustomErrorsSection GetSettings(HttpContext context) { return GetSettings(context, false); } internal static CustomErrorsSection GetSettings(HttpContext context, bool canThrow) { CustomErrorsSection ce = null; RuntimeConfig runtimeConfig = null; if (canThrow) { runtimeConfig = RuntimeConfig.GetConfig(context); if (runtimeConfig != null) { ce = runtimeConfig.CustomErrors; } } else { runtimeConfig = RuntimeConfig.GetLKGConfig(context); if (runtimeConfig != null) { ce = runtimeConfig.CustomErrors; } if (ce == null) { if (_default == null) { _default = new CustomErrorsSection(); } ce = _default; } } return ce; } internal bool CustomErrorsEnabled(HttpRequest request) { // This could throw if the config file is malformed, but we don't want // to throw from here, as it would mess up error handling try { // Always turn of custom errors in retail deployment mode (DevDiv 36396) if (DeploymentSection.RetailInternal) return true; } catch { } switch (Mode) { case CustomErrorsMode.Off: return false; case CustomErrorsMode.On: return true; case CustomErrorsMode.RemoteOnly: return (!request.IsLocal); default: return false; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
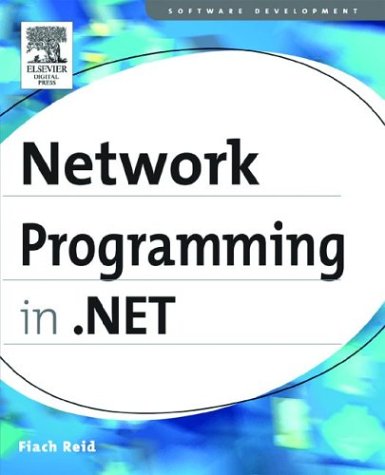
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- UpdateExpressionVisitor.cs
- ICollection.cs
- SchemaRegistration.cs
- SpinWait.cs
- CmsInterop.cs
- SamlAuthenticationStatement.cs
- SafeRightsManagementEnvironmentHandle.cs
- PersistenceProviderDirectory.cs
- XmlAttributeCollection.cs
- ReflectEventDescriptor.cs
- NamedPipeProcessProtocolHandler.cs
- AutomationPropertyInfo.cs
- OleDbRowUpdatedEvent.cs
- CssTextWriter.cs
- CodePrimitiveExpression.cs
- ClockController.cs
- safemediahandle.cs
- ModelEditingScope.cs
- ProtocolsConfigurationEntry.cs
- WebServiceMethodData.cs
- CmsInterop.cs
- ParameterModifier.cs
- ZeroOpNode.cs
- DoubleAverageAggregationOperator.cs
- SmuggledIUnknown.cs
- GatewayIPAddressInformationCollection.cs
- SqlStream.cs
- FacetEnabledSchemaElement.cs
- DateTimeValueSerializerContext.cs
- HiddenFieldPageStatePersister.cs
- URL.cs
- MgmtConfigurationRecord.cs
- WebPartVerbCollection.cs
- DBParameter.cs
- SocketException.cs
- ColorEditor.cs
- ExpressionNode.cs
- RelationalExpressions.cs
- Model3D.cs
- SqlWebEventProvider.cs
- TextTreeUndo.cs
- DefinitionUpdate.cs
- NameValueSectionHandler.cs
- ObjectListComponentEditor.cs
- CodeObject.cs
- ScrollEvent.cs
- WSSecurityPolicy.cs
- CardSpacePolicyElement.cs
- Point4DValueSerializer.cs
- SolidBrush.cs
- ThrowHelper.cs
- XmlJsonReader.cs
- Codec.cs
- ImageList.cs
- PaperSource.cs
- Soap12ServerProtocol.cs
- Cursors.cs
- TransactionContextValidator.cs
- FileSystemEnumerable.cs
- SerializationFieldInfo.cs
- IndexedWhereQueryOperator.cs
- SubMenuStyleCollection.cs
- HyperLinkColumn.cs
- SqlMultiplexer.cs
- SerializationAttributes.cs
- SafeRightsManagementSessionHandle.cs
- InvalidTimeZoneException.cs
- ArrayList.cs
- InstanceNotReadyException.cs
- ImagingCache.cs
- MD5Cng.cs
- ToolStripComboBox.cs
- DataGridViewRowErrorTextNeededEventArgs.cs
- TaskExceptionHolder.cs
- ToolBarButtonClickEvent.cs
- TdsRecordBufferSetter.cs
- SystemNetworkInterface.cs
- ConfigurationSettings.cs
- GregorianCalendar.cs
- SchemaMapping.cs
- MemoryRecordBuffer.cs
- DbProviderFactoriesConfigurationHandler.cs
- FastEncoderWindow.cs
- SystemWebExtensionsSectionGroup.cs
- SqlDataSourceCache.cs
- Item.cs
- SaveFileDialog.cs
- RawStylusSystemGestureInputReport.cs
- AssertSection.cs
- QuadraticBezierSegment.cs
- ExpressionPrefixAttribute.cs
- ModelItemKeyValuePair.cs
- SQlBooleanStorage.cs
- WebServiceErrorEvent.cs
- FontFamilyValueSerializer.cs
- FamilyMap.cs
- ObjectQuery_EntitySqlExtensions.cs
- ThicknessConverter.cs
- TextDecorationCollection.cs
- Point4DValueSerializer.cs