Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataEntity / System / Data / Map / ViewGeneration / Structures / MemberProjectedSlot.cs / 1305376 / MemberProjectedSlot.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.Collections.Generic; using System.Text; using System.Diagnostics; using System.Data.Common.Utils; using System.Data.Metadata.Edm; using System.Data.Mapping.ViewGeneration.CqlGeneration; using System.Data.Mapping.ViewGeneration.Utils; namespace System.Data.Mapping.ViewGeneration.Structures { // A wrapper around MemberPath that allows members to be marked as ProjectedSlots internal class MemberProjectedSlot : ProjectedSlot { #region Constructor // effects: Creates a projected slot that references the relevant // jointree node (i.e., internal MemberProjectedSlot(MemberPath node) { m_node = node; } #endregion #region Fields // A comparer object for comparing jointree slots by MemberPaths internal static readonly IEqualityComparerSpecificEqualityComparer = new MemberProjectedSlotEqualityComparer(); private MemberPath m_node; // The node that the var corresponds to #endregion #region Properties // effects: Returns the full metadata path from the root extent to // this node, e.g., Person.Adrs.zip internal MemberPath MemberPath { get { return m_node; } } #endregion #region Methods internal override StringBuilder AsCql(StringBuilder builder, MemberPath outputMember, string blockAlias, int indentLevel) { // If memberpath's type and the outputmember type do not match, // we have to cast. We assume that the mapping loader has already // checked that the casts are ok and emitted warnings TypeUsage memberPathStoreTypeUsage = Helper.GetModelTypeUsage(MemberPath.LeafEdmMember); TypeUsage outputMemberStoreTypeUsage = Helper.GetModelTypeUsage(outputMember.LeafEdmMember); if (false == memberPathStoreTypeUsage.EdmType.Equals(outputMemberStoreTypeUsage.EdmType)) { builder.Append("CAST("); MemberPath.AsCql(builder, blockAlias); builder.Append(" AS "); CqlWriter.AppendEscapedTypeName(builder, outputMemberStoreTypeUsage.EdmType); builder.Append(')'); } else { MemberPath.AsCql(builder, blockAlias); } return builder; } internal override void ToCompactString(StringBuilder builder) { m_node.ToCompactString(builder); } internal string ToUserString() { return m_node.PathToString(false); } protected override bool IsEqualTo(ProjectedSlot right) { MemberProjectedSlot rightSlot = right as MemberProjectedSlot; if (rightSlot == null) { return false; } // We want equality of the paths return MemberPath.EqualityComparer.Equals(m_node, rightSlot.m_node); } protected override int GetHash() { return MemberPath.EqualityComparer.GetHashCode(m_node); } // effects: Given a slot and the new mapping, returns the // corresponding new slot internal override ProjectedSlot RemapSlot(Dictionary remap) { MemberPath remappedNode = null; if (remap.TryGetValue(MemberPath, out remappedNode)) { return new MemberProjectedSlot(remappedNode); } else { return new MemberProjectedSlot(MemberPath); } } #endregion #region Helper methods // requires: prefix corresponds to an entity set or an association end // effects: Given the prefix, determines the slots in "slots" // that correspond to the entity key for the entity set or the // association set end. Returns the list of slots. Returns null if // even one of the key slots is not present in slots internal static List GetKeySlots(IEnumerable slots, MemberPath prefix) { // Get the entity type of the hosted end or entity set EntitySet entitySet = prefix.EntitySet; List keys = ExtentKey.GetKeysForEntityType(prefix, entitySet.ElementType); Debug.Assert(keys.Count > 0, "No keys for entity?"); Debug.Assert(keys.Count == 1, "Currently, we only support primary keys"); // Get the slots for the key List keySlots = GetSlots(slots, keys[0].KeyFields); return keySlots; } // effects: Searches for members in slots and returns the // corresponding slots in the same order as present in // members. Returns null if even one member is not present in slots internal static List GetSlots(IEnumerable slots, IEnumerable members) { List result = new List (); foreach (MemberPath member in members) { MemberProjectedSlot slot = GetSlotForMember(Helpers.AsSuperTypeList (slots), member); if (slot == null) { return null; } result.Add(slot); } return result; } // effects: Searches for member in slots and returns the corresponding slot // If none is found, returns null internal static MemberProjectedSlot GetSlotForMember(IEnumerable slots, MemberPath member) { foreach (MemberProjectedSlot slot in slots) { if (MemberPath.EqualityComparer.Equals(slot.MemberPath, member)) { return slot; } } return null; } #endregion #region JoinTreeSlot Comparer classes // summary: Two JoinTreeSlots are equal when their MemberPaths are equal (JoinTreeSlot.IsEqualTo returns true) internal class MemberProjectedSlotEqualityComparer : IEqualityComparer { // effects: Returns true if left and right are semantically equivalent public bool Equals(MemberProjectedSlot left, MemberProjectedSlot right) { return ProjectedSlot.EqualityComparer.Equals(left, right); } public int GetHashCode(MemberProjectedSlot key) { return ProjectedSlot.EqualityComparer.GetHashCode(key); } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
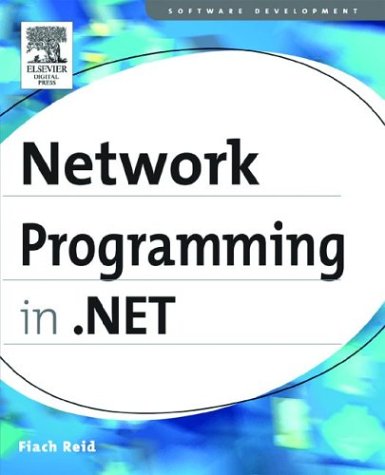
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ComplexLine.cs
- GeneralTransform.cs
- TextTreeObjectNode.cs
- WebPartVerbCollection.cs
- UnwrappedTypesXmlSerializerManager.cs
- Compiler.cs
- ResizeGrip.cs
- COM2PropertyDescriptor.cs
- ValidatingPropertiesEventArgs.cs
- MultiTouchSystemGestureLogic.cs
- DialogResultConverter.cs
- MembershipPasswordException.cs
- ArrangedElement.cs
- StreamWithDictionary.cs
- ClockGroup.cs
- HostSecurityManager.cs
- SecUtil.cs
- FontUnitConverter.cs
- LocatorGroup.cs
- PagePropertiesChangingEventArgs.cs
- MsdtcClusterUtils.cs
- ExternalCalls.cs
- BaseConfigurationRecord.cs
- BaseResourcesBuildProvider.cs
- TextRenderer.cs
- SimpleWorkerRequest.cs
- ApplicationFileCodeDomTreeGenerator.cs
- RegexTree.cs
- WizardPanel.cs
- HostedBindingBehavior.cs
- XmlNamespaceManager.cs
- NativeMethods.cs
- PnrpPeerResolverBindingElement.cs
- AttributeTableBuilder.cs
- ExcCanonicalXml.cs
- TickBar.cs
- Viewport3DVisual.cs
- BindingRestrictions.cs
- WebExceptionStatus.cs
- Soap12ServerProtocol.cs
- CommandPlan.cs
- ProvidersHelper.cs
- FormsAuthenticationModule.cs
- TextBox.cs
- TiffBitmapEncoder.cs
- TrustLevelCollection.cs
- Model3DGroup.cs
- ProfilePropertySettings.cs
- UnionExpr.cs
- ColumnCollection.cs
- ModelItemImpl.cs
- BamlBinaryReader.cs
- ApplicationHost.cs
- GraphicsState.cs
- Globals.cs
- CacheAxisQuery.cs
- LowerCaseStringConverter.cs
- ReadOnlyActivityGlyph.cs
- XmlValidatingReader.cs
- WebPartUtil.cs
- GestureRecognitionResult.cs
- ListView.cs
- MenuEventArgs.cs
- EditingCommands.cs
- Transform.cs
- SelectionChangedEventArgs.cs
- MeasureItemEvent.cs
- DbConnectionInternal.cs
- ScriptControlDescriptor.cs
- ReflectionUtil.cs
- DataKey.cs
- HttpApplication.cs
- WsatTransactionInfo.cs
- DataGridViewColumnCollection.cs
- ConsoleEntryPoint.cs
- DrawListViewSubItemEventArgs.cs
- TextEncodedRawTextWriter.cs
- AssertUtility.cs
- NameValuePermission.cs
- MobileControlBuilder.cs
- ToolStripPanelSelectionBehavior.cs
- XDRSchema.cs
- GroupItemAutomationPeer.cs
- SubstitutionDesigner.cs
- _AutoWebProxyScriptEngine.cs
- EncoderExceptionFallback.cs
- HybridDictionary.cs
- PrintPreviewGraphics.cs
- TimelineClockCollection.cs
- PeerNameRecord.cs
- UserMapPath.cs
- XmlCharCheckingReader.cs
- AspNetHostingPermission.cs
- Token.cs
- OTFRasterizer.cs
- CultureSpecificCharacterBufferRange.cs
- TextComposition.cs
- DbConnectionInternal.cs
- TypedTableBaseExtensions.cs
- NavigationExpr.cs