Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / System / Windows / Media / Animation / TimelineClockCollection.cs / 1305600 / TimelineClockCollection.cs
// ClockCollection.cs using System; using System.Collections.Generic; using System.Diagnostics; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; #pragma warning disable 1634, 1691 // suppressing PreSharp warnings namespace System.Windows.Media.Animation { ////// A collection of Clock objects. /// public class ClockCollection : ICollection{ #region External interface #region ICollection #region Properties /// /// Gets the number of elements contained in the collection. /// ////// The number of elements contained in the collection. /// public int Count { get { // _owner.VerifyAccess(); ClockGroup clockGroup = _owner as ClockGroup; if (clockGroup != null) { ListchildList = clockGroup.InternalChildren; if (childList != null) { return childList.Count; } } return 0; } } /// /// /// ///public bool IsReadOnly { get { return true; } } #endregion // Properties #region Methods /// /// /// public void Clear() { throw new NotSupportedException(); } ////// /// /// ///public void Add(Clock item) { throw new NotSupportedException(); } /// /// /// /// ///public bool Remove(Clock item) { throw new NotSupportedException(); } /// /// /// /// ///public bool Contains(Clock item) { if (item == null) { throw new ArgumentNullException("item"); } foreach (Clock t in this) { #pragma warning suppress 6506 // the enumerator will not contain nulls if (t.Equals(item)) return true; } return false; } /// /// Copies the elements of the collection to an array, starting at a /// particular array index. /// /// /// The one-dimensional array that is the destination of the elements /// copied from the collection. The Array must have zero-based indexing. /// /// /// The zero-based index in array at which copying begins. /// public void CopyTo(Clock[] array, int index) { // _owner.VerifyAccess(); ClockGroup clockGroup = _owner as ClockGroup; if (clockGroup != null) { Listlist = clockGroup.InternalChildren; if (list != null) { // Get free parameter validation from Array.Copy list.CopyTo(array, index); } } // } #endregion // Methods #endregion // ICollection #region IEnumerable #region Methods /// /// Returns an enumerator that can iterate through a collection. /// ////// An enumerator that can iterate through a collection. /// IEnumeratorIEnumerable .GetEnumerator() { // _owner.VerifyAccess(); List list = null; ClockGroup clockGroup = _owner as ClockGroup; if (clockGroup != null) { list = clockGroup.InternalChildren; } if (list != null) { return list.GetEnumerator(); } else { return new ClockEnumerator(_owner); } } System.Collections.IEnumerator System.Collections.IEnumerable.GetEnumerator() { return new ClockEnumerator(_owner); } /// /// Checks for equality of two ClockCollections /// /// /// Other object against which to check for equality /// public override bool Equals(object obj) { if (obj is ClockCollection) { return (this == (ClockCollection)obj); } else { return false; } } ////// Checks for equality of two ClockCollections /// /// /// First ClockCollection to check for equality /// /// /// Second ClockCollection to check for equality /// public static bool Equals(ClockCollection objA, ClockCollection objB) { return (objA == objB); } ////// Shallow comparison for equality: A and B have the same owner /// /// /// First ClockCollection to check for equality /// /// /// Second ClockCollection to check for equality /// public static bool operator ==(ClockCollection objA, ClockCollection objB) { if (Object.ReferenceEquals(objA, objB)) { // Exact same object. return true; } else if ( Object.ReferenceEquals(objA, null) || Object.ReferenceEquals(objB, null)) { // One is null, the other isn't. return false; } else { // Both are non-null. #pragma warning disable 56506 // Suppress presharp warning: Parameter 'objA' to this public method must be validated: A null-dereference can occur here. return objA._owner == objB._owner; #pragma warning restore 56506 } } ////// Shallow comparison for inequality: A and B have different owner /// /// /// First ClockCollection to check for inequality /// /// /// Second ClockCollection to check for inequality /// public static bool operator !=(ClockCollection objA, ClockCollection objB) { return !(objA == objB); } ////// GetHashCode /// public override int GetHashCode() { return _owner.GetHashCode(); } #endregion // Methods #endregion // IEnumerable #region Properties ////// Gets or sets the element at the specified index. /// ////// The element at the specified index. /// public Clock this[int index] { get { // _owner.VerifyAccess(); Listlist = null; ClockGroup clockGroup = _owner as ClockGroup; if (clockGroup != null) { list = clockGroup.InternalChildren; } if (list == null) { throw new ArgumentOutOfRangeException("index"); } return list[index]; } } #endregion // Properties #endregion // External interface #region Internal implementation #region Types /// /// An enumerator for a ClockCollection object. /// internal struct ClockEnumerator : IEnumerator{ #region Construction /// /// Creates an enumerator for the specified clock. /// /// /// The clock whose children to enumerate. /// internal ClockEnumerator(Clock owner) { _owner = owner; } #endregion // Construction #region IDisposable interface ////// Disposes the enumerator. /// public void Dispose() { // The enumerator doesn't do much, so we don't have to do // anything to dispose it. } #endregion // IDisposable interface #region IEnumerator interface ////// Gets the current element in the collection. /// ////// The current element in the collection. /// Clock IEnumerator.Current { get { throw new InvalidOperationException(SR.Get(SRID.Timing_EnumeratorOutOfRange)); } } #region IEnumerator Members object System.Collections.IEnumerator.Current { get { return ((IEnumerator )this).Current; } } void System.Collections.IEnumerator.Reset() { throw new NotImplementedException(); } #endregion /// /// Advances the enumerator to the next element of the collection. /// ////// true if the enumerator was successfully advanced to the next /// element; false if the enumerator has passed the end of the /// collection. /// public bool MoveNext() { // If the collection is no longer empty, it means it was // modified and we should thrown an exception. Otherwise, we // are still valid, but the collection is empty so we should // just return false. // _owner.VerifyAccess(); ClockGroup clockGroup = _owner as ClockGroup; if (clockGroup != null && clockGroup.InternalChildren != null) { throw new InvalidOperationException(SR.Get(SRID.Timing_EnumeratorInvalidated)); } return false; } #endregion // IEnumerator interface #region Internal implementation #region Data private Clock _owner; #endregion // Data #endregion // Internal implementation } #endregion // Types #region Constructors ////// Creates an initially empty collection of Clock objects. /// /// /// The Clock that owns this collection. /// internal ClockCollection(Clock owner) { Debug.Assert(owner != null, "ClockCollection must have a non-null owner."); _owner = owner; } ////// Disallow parameterless constructors /// private ClockCollection() { Debug.Assert(false, "Parameterless constructor is illegal for ClockCollection."); } #endregion #region Data private Clock _owner; #endregion // Data #endregion // Internal implementation } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. // ClockCollection.cs using System; using System.Collections.Generic; using System.Diagnostics; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; #pragma warning disable 1634, 1691 // suppressing PreSharp warnings namespace System.Windows.Media.Animation { ////// A collection of Clock objects. /// public class ClockCollection : ICollection{ #region External interface #region ICollection #region Properties /// /// Gets the number of elements contained in the collection. /// ////// The number of elements contained in the collection. /// public int Count { get { // _owner.VerifyAccess(); ClockGroup clockGroup = _owner as ClockGroup; if (clockGroup != null) { ListchildList = clockGroup.InternalChildren; if (childList != null) { return childList.Count; } } return 0; } } /// /// /// ///public bool IsReadOnly { get { return true; } } #endregion // Properties #region Methods /// /// /// public void Clear() { throw new NotSupportedException(); } ////// /// /// ///public void Add(Clock item) { throw new NotSupportedException(); } /// /// /// /// ///public bool Remove(Clock item) { throw new NotSupportedException(); } /// /// /// /// ///public bool Contains(Clock item) { if (item == null) { throw new ArgumentNullException("item"); } foreach (Clock t in this) { #pragma warning suppress 6506 // the enumerator will not contain nulls if (t.Equals(item)) return true; } return false; } /// /// Copies the elements of the collection to an array, starting at a /// particular array index. /// /// /// The one-dimensional array that is the destination of the elements /// copied from the collection. The Array must have zero-based indexing. /// /// /// The zero-based index in array at which copying begins. /// public void CopyTo(Clock[] array, int index) { // _owner.VerifyAccess(); ClockGroup clockGroup = _owner as ClockGroup; if (clockGroup != null) { Listlist = clockGroup.InternalChildren; if (list != null) { // Get free parameter validation from Array.Copy list.CopyTo(array, index); } } // } #endregion // Methods #endregion // ICollection #region IEnumerable #region Methods /// /// Returns an enumerator that can iterate through a collection. /// ////// An enumerator that can iterate through a collection. /// IEnumeratorIEnumerable .GetEnumerator() { // _owner.VerifyAccess(); List list = null; ClockGroup clockGroup = _owner as ClockGroup; if (clockGroup != null) { list = clockGroup.InternalChildren; } if (list != null) { return list.GetEnumerator(); } else { return new ClockEnumerator(_owner); } } System.Collections.IEnumerator System.Collections.IEnumerable.GetEnumerator() { return new ClockEnumerator(_owner); } /// /// Checks for equality of two ClockCollections /// /// /// Other object against which to check for equality /// public override bool Equals(object obj) { if (obj is ClockCollection) { return (this == (ClockCollection)obj); } else { return false; } } ////// Checks for equality of two ClockCollections /// /// /// First ClockCollection to check for equality /// /// /// Second ClockCollection to check for equality /// public static bool Equals(ClockCollection objA, ClockCollection objB) { return (objA == objB); } ////// Shallow comparison for equality: A and B have the same owner /// /// /// First ClockCollection to check for equality /// /// /// Second ClockCollection to check for equality /// public static bool operator ==(ClockCollection objA, ClockCollection objB) { if (Object.ReferenceEquals(objA, objB)) { // Exact same object. return true; } else if ( Object.ReferenceEquals(objA, null) || Object.ReferenceEquals(objB, null)) { // One is null, the other isn't. return false; } else { // Both are non-null. #pragma warning disable 56506 // Suppress presharp warning: Parameter 'objA' to this public method must be validated: A null-dereference can occur here. return objA._owner == objB._owner; #pragma warning restore 56506 } } ////// Shallow comparison for inequality: A and B have different owner /// /// /// First ClockCollection to check for inequality /// /// /// Second ClockCollection to check for inequality /// public static bool operator !=(ClockCollection objA, ClockCollection objB) { return !(objA == objB); } ////// GetHashCode /// public override int GetHashCode() { return _owner.GetHashCode(); } #endregion // Methods #endregion // IEnumerable #region Properties ////// Gets or sets the element at the specified index. /// ////// The element at the specified index. /// public Clock this[int index] { get { // _owner.VerifyAccess(); Listlist = null; ClockGroup clockGroup = _owner as ClockGroup; if (clockGroup != null) { list = clockGroup.InternalChildren; } if (list == null) { throw new ArgumentOutOfRangeException("index"); } return list[index]; } } #endregion // Properties #endregion // External interface #region Internal implementation #region Types /// /// An enumerator for a ClockCollection object. /// internal struct ClockEnumerator : IEnumerator{ #region Construction /// /// Creates an enumerator for the specified clock. /// /// /// The clock whose children to enumerate. /// internal ClockEnumerator(Clock owner) { _owner = owner; } #endregion // Construction #region IDisposable interface ////// Disposes the enumerator. /// public void Dispose() { // The enumerator doesn't do much, so we don't have to do // anything to dispose it. } #endregion // IDisposable interface #region IEnumerator interface ////// Gets the current element in the collection. /// ////// The current element in the collection. /// Clock IEnumerator.Current { get { throw new InvalidOperationException(SR.Get(SRID.Timing_EnumeratorOutOfRange)); } } #region IEnumerator Members object System.Collections.IEnumerator.Current { get { return ((IEnumerator )this).Current; } } void System.Collections.IEnumerator.Reset() { throw new NotImplementedException(); } #endregion /// /// Advances the enumerator to the next element of the collection. /// ////// true if the enumerator was successfully advanced to the next /// element; false if the enumerator has passed the end of the /// collection. /// public bool MoveNext() { // If the collection is no longer empty, it means it was // modified and we should thrown an exception. Otherwise, we // are still valid, but the collection is empty so we should // just return false. // _owner.VerifyAccess(); ClockGroup clockGroup = _owner as ClockGroup; if (clockGroup != null && clockGroup.InternalChildren != null) { throw new InvalidOperationException(SR.Get(SRID.Timing_EnumeratorInvalidated)); } return false; } #endregion // IEnumerator interface #region Internal implementation #region Data private Clock _owner; #endregion // Data #endregion // Internal implementation } #endregion // Types #region Constructors ////// Creates an initially empty collection of Clock objects. /// /// /// The Clock that owns this collection. /// internal ClockCollection(Clock owner) { Debug.Assert(owner != null, "ClockCollection must have a non-null owner."); _owner = owner; } ////// Disallow parameterless constructors /// private ClockCollection() { Debug.Assert(false, "Parameterless constructor is illegal for ClockCollection."); } #endregion #region Data private Clock _owner; #endregion // Data #endregion // Internal implementation } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
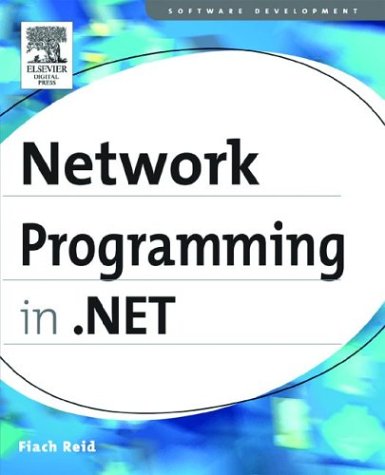
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataServiceRequest.cs
- SelectionEditor.cs
- SetterBase.cs
- EntityDataSourceDataSelection.cs
- HtmlForm.cs
- WindowsRichEdit.cs
- TreeNodeBindingCollection.cs
- GeneralTransform2DTo3DTo2D.cs
- WebHttpBehavior.cs
- XmlSerializer.cs
- AdvancedBindingPropertyDescriptor.cs
- SqlError.cs
- DesignerDataView.cs
- Stack.cs
- DataGridViewRowHeaderCell.cs
- XmlElement.cs
- FixedFlowMap.cs
- Hashtable.cs
- ScriptingAuthenticationServiceSection.cs
- DataBindingList.cs
- Button.cs
- sqlstateclientmanager.cs
- EventTrigger.cs
- TypeConverterHelper.cs
- WebRequest.cs
- DBCommandBuilder.cs
- SafeRightsManagementPubHandle.cs
- DrawingContextWalker.cs
- CardSpaceException.cs
- Screen.cs
- UrlMappingCollection.cs
- SafeArrayTypeMismatchException.cs
- ObjectDataSource.cs
- WindowProviderWrapper.cs
- MatrixValueSerializer.cs
- NodeFunctions.cs
- MergeFailedEvent.cs
- BasicExpressionVisitor.cs
- ParseHttpDate.cs
- TreeNodeStyleCollection.cs
- mediaeventshelper.cs
- TreeNodeBinding.cs
- WebPartDisplayMode.cs
- Assert.cs
- UserPersonalizationStateInfo.cs
- XmlSiteMapProvider.cs
- BufferedWebEventProvider.cs
- DataGridViewComboBoxCell.cs
- ContextStack.cs
- AnnotationAuthorChangedEventArgs.cs
- XPathParser.cs
- WebPartZoneCollection.cs
- IndexedGlyphRun.cs
- FrugalMap.cs
- UnmanagedHandle.cs
- ServiceOperation.cs
- WindowsListViewSubItem.cs
- MethodBody.cs
- SiteMapNodeCollection.cs
- MeasureItemEvent.cs
- CustomUserNameSecurityTokenAuthenticator.cs
- TemplateBamlRecordReader.cs
- ApplicationTrust.cs
- XmlDomTextWriter.cs
- MbpInfo.cs
- StatusBarItemAutomationPeer.cs
- AggregateNode.cs
- ImageConverter.cs
- CompilerGlobalScopeAttribute.cs
- login.cs
- GeometryConverter.cs
- AQNBuilder.cs
- ScriptReferenceEventArgs.cs
- OdbcEnvironmentHandle.cs
- SqlVisitor.cs
- ValidationResult.cs
- AffineTransform3D.cs
- ChtmlTextWriter.cs
- FixedSOMImage.cs
- InternalConfigSettingsFactory.cs
- ReadOnlyDictionary.cs
- FixedSOMTable.cs
- ConfigurationPropertyAttribute.cs
- DataGridViewImageColumn.cs
- DataSourceCache.cs
- WebConfigurationManager.cs
- ParsedRoute.cs
- XmlSignatureManifest.cs
- DataChangedEventManager.cs
- Point3D.cs
- FragmentQueryProcessor.cs
- AuthenticationModulesSection.cs
- DataServiceResponse.cs
- ParsedRoute.cs
- DbDataRecord.cs
- XmlUrlResolver.cs
- RuleRefElement.cs
- XPathNodeInfoAtom.cs
- DLinqTableProvider.cs
- ProfileGroupSettingsCollection.cs