Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / clr / src / BCL / System / Runtime / InteropServices / TCEAdapterGen / TCEAdapterGenerator.cs / 1 / TCEAdapterGenerator.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== namespace System.Runtime.InteropServices.TCEAdapterGen { using System.Runtime.InteropServices; using System; using System.Reflection; using System.Reflection.Emit; using System.Collections; using System.Threading; internal class TCEAdapterGenerator { public void Process(ModuleBuilder ModBldr, ArrayList EventItfList) { // Store the input/output module. m_Module = ModBldr; // Generate the TCE adapters for all the event sources. int NumEvItfs = EventItfList.Count; for ( int cEventItfs = 0; cEventItfs < NumEvItfs; cEventItfs++ ) { // Retrieve the event interface info. EventItfInfo CurrEventItf = (EventItfInfo)EventItfList[cEventItfs]; // Retrieve the information from the event interface info. Type EventItfType = CurrEventItf.GetEventItfType(); Type SrcItfType = CurrEventItf.GetSrcItfType(); String EventProviderName = CurrEventItf.GetEventProviderName(); // Generate the sink interface helper. Type SinkHelperType = new EventSinkHelperWriter( m_Module, SrcItfType, EventItfType ).Perform(); // Generate the event provider. new EventProviderWriter( m_Module, EventProviderName, EventItfType, SrcItfType, SinkHelperType ).Perform(); } } internal static void SetClassInterfaceTypeToNone(TypeBuilder tb) { // Create the ClassInterface(ClassInterfaceType.None) CA builder if we haven't created it yet. if (s_NoClassItfCABuilder == null) { Type []aConsParams = new Type[1]; aConsParams[0] = typeof(ClassInterfaceType); ConstructorInfo Cons = typeof(ClassInterfaceAttribute).GetConstructor(aConsParams); Object[] aArgs = new Object[1]; aArgs[0] = ClassInterfaceType.None; s_NoClassItfCABuilder = new CustomAttributeBuilder(Cons, aArgs); } // Set the class interface type to none. tb.SetCustomAttribute(s_NoClassItfCABuilder); } internal static TypeBuilder DefineUniqueType(String strInitFullName, TypeAttributes attrs, Type BaseType, Type[] aInterfaceTypes, ModuleBuilder mb) { String strFullName = strInitFullName; int PostFix = 2; // Find the first unique name for the type. for (; mb.GetType(strFullName) != null; strFullName = strInitFullName + "_" + PostFix, PostFix++); // Define a type with the determined unique name. return mb.DefineType(strFullName, attrs, BaseType, aInterfaceTypes); } internal static void SetHiddenAttribute(TypeBuilder tb) { if (s_HiddenCABuilder == null) { // Hide the type from Object Browsers Type []aConsParams = new Type[1]; aConsParams[0] = typeof(TypeLibTypeFlags); ConstructorInfo Cons = typeof(TypeLibTypeAttribute).GetConstructor(aConsParams); Object []aArgs = new Object[1]; aArgs[0] = TypeLibTypeFlags.FHidden; s_HiddenCABuilder = new CustomAttributeBuilder(Cons, aArgs); } tb.SetCustomAttribute(s_HiddenCABuilder); } internal static MethodInfo[] GetNonPropertyMethods(Type type) { MethodInfo[] aMethods = type.GetMethods(); ArrayList methods = new ArrayList(aMethods); PropertyInfo[] props = type.GetProperties(); foreach(PropertyInfo prop in props) { MethodInfo[] accessors = prop.GetAccessors(); foreach (MethodInfo accessor in accessors) { for (int i=0; i < methods.Count; i++) { if ((MethodInfo)methods[i] == accessor) methods.RemoveAt(i); } } } MethodInfo[] retMethods = new MethodInfo[methods.Count]; methods.CopyTo(retMethods); return retMethods; } internal static MethodInfo[] GetPropertyMethods(Type type) { MethodInfo[] aMethods = type.GetMethods(); ArrayList methods = new ArrayList(); PropertyInfo[] props = type.GetProperties(); foreach(PropertyInfo prop in props) { MethodInfo[] accessors = prop.GetAccessors(); foreach (MethodInfo accessor in accessors) { methods.Add(accessor); } } MethodInfo[] retMethods = new MethodInfo[methods.Count]; methods.CopyTo(retMethods); return retMethods; } private ModuleBuilder m_Module = null; private Hashtable m_SrcItfToSrcItfInfoMap = new Hashtable(); private static CustomAttributeBuilder s_NoClassItfCABuilder = null; private static CustomAttributeBuilder s_HiddenCABuilder = null; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== namespace System.Runtime.InteropServices.TCEAdapterGen { using System.Runtime.InteropServices; using System; using System.Reflection; using System.Reflection.Emit; using System.Collections; using System.Threading; internal class TCEAdapterGenerator { public void Process(ModuleBuilder ModBldr, ArrayList EventItfList) { // Store the input/output module. m_Module = ModBldr; // Generate the TCE adapters for all the event sources. int NumEvItfs = EventItfList.Count; for ( int cEventItfs = 0; cEventItfs < NumEvItfs; cEventItfs++ ) { // Retrieve the event interface info. EventItfInfo CurrEventItf = (EventItfInfo)EventItfList[cEventItfs]; // Retrieve the information from the event interface info. Type EventItfType = CurrEventItf.GetEventItfType(); Type SrcItfType = CurrEventItf.GetSrcItfType(); String EventProviderName = CurrEventItf.GetEventProviderName(); // Generate the sink interface helper. Type SinkHelperType = new EventSinkHelperWriter( m_Module, SrcItfType, EventItfType ).Perform(); // Generate the event provider. new EventProviderWriter( m_Module, EventProviderName, EventItfType, SrcItfType, SinkHelperType ).Perform(); } } internal static void SetClassInterfaceTypeToNone(TypeBuilder tb) { // Create the ClassInterface(ClassInterfaceType.None) CA builder if we haven't created it yet. if (s_NoClassItfCABuilder == null) { Type []aConsParams = new Type[1]; aConsParams[0] = typeof(ClassInterfaceType); ConstructorInfo Cons = typeof(ClassInterfaceAttribute).GetConstructor(aConsParams); Object[] aArgs = new Object[1]; aArgs[0] = ClassInterfaceType.None; s_NoClassItfCABuilder = new CustomAttributeBuilder(Cons, aArgs); } // Set the class interface type to none. tb.SetCustomAttribute(s_NoClassItfCABuilder); } internal static TypeBuilder DefineUniqueType(String strInitFullName, TypeAttributes attrs, Type BaseType, Type[] aInterfaceTypes, ModuleBuilder mb) { String strFullName = strInitFullName; int PostFix = 2; // Find the first unique name for the type. for (; mb.GetType(strFullName) != null; strFullName = strInitFullName + "_" + PostFix, PostFix++); // Define a type with the determined unique name. return mb.DefineType(strFullName, attrs, BaseType, aInterfaceTypes); } internal static void SetHiddenAttribute(TypeBuilder tb) { if (s_HiddenCABuilder == null) { // Hide the type from Object Browsers Type []aConsParams = new Type[1]; aConsParams[0] = typeof(TypeLibTypeFlags); ConstructorInfo Cons = typeof(TypeLibTypeAttribute).GetConstructor(aConsParams); Object []aArgs = new Object[1]; aArgs[0] = TypeLibTypeFlags.FHidden; s_HiddenCABuilder = new CustomAttributeBuilder(Cons, aArgs); } tb.SetCustomAttribute(s_HiddenCABuilder); } internal static MethodInfo[] GetNonPropertyMethods(Type type) { MethodInfo[] aMethods = type.GetMethods(); ArrayList methods = new ArrayList(aMethods); PropertyInfo[] props = type.GetProperties(); foreach(PropertyInfo prop in props) { MethodInfo[] accessors = prop.GetAccessors(); foreach (MethodInfo accessor in accessors) { for (int i=0; i < methods.Count; i++) { if ((MethodInfo)methods[i] == accessor) methods.RemoveAt(i); } } } MethodInfo[] retMethods = new MethodInfo[methods.Count]; methods.CopyTo(retMethods); return retMethods; } internal static MethodInfo[] GetPropertyMethods(Type type) { MethodInfo[] aMethods = type.GetMethods(); ArrayList methods = new ArrayList(); PropertyInfo[] props = type.GetProperties(); foreach(PropertyInfo prop in props) { MethodInfo[] accessors = prop.GetAccessors(); foreach (MethodInfo accessor in accessors) { methods.Add(accessor); } } MethodInfo[] retMethods = new MethodInfo[methods.Count]; methods.CopyTo(retMethods); return retMethods; } private ModuleBuilder m_Module = null; private Hashtable m_SrcItfToSrcItfInfoMap = new Hashtable(); private static CustomAttributeBuilder s_NoClassItfCABuilder = null; private static CustomAttributeBuilder s_HiddenCABuilder = null; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
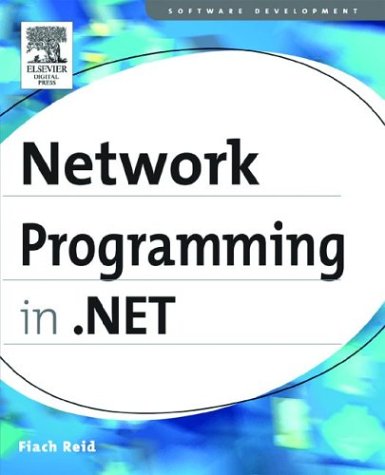
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TdsValueSetter.cs
- RelatedPropertyManager.cs
- SemanticResultKey.cs
- PackageRelationshipSelector.cs
- BooleanKeyFrameCollection.cs
- SortableBindingList.cs
- ObjectContext.cs
- Utilities.cs
- WmiPutTraceRecord.cs
- GradientBrush.cs
- Bidi.cs
- HierarchicalDataTemplate.cs
- IdentityValidationException.cs
- CacheHelper.cs
- PathSegment.cs
- DocumentViewerAutomationPeer.cs
- ColorConvertedBitmap.cs
- ISO2022Encoding.cs
- NotificationContext.cs
- FieldToken.cs
- oledbmetadatacollectionnames.cs
- EntityViewGenerationAttribute.cs
- PageCatalogPart.cs
- DescendantOverDescendantQuery.cs
- AnnotationAuthorChangedEventArgs.cs
- QilReplaceVisitor.cs
- CommandManager.cs
- ModelProperty.cs
- PersianCalendar.cs
- EngineSiteSapi.cs
- Literal.cs
- HashFinalRequest.cs
- SettingsAttributes.cs
- CheckBoxPopupAdapter.cs
- ProfileEventArgs.cs
- ExcCanonicalXml.cs
- ReflectionPermission.cs
- RenderingEventArgs.cs
- DesignerActionPropertyItem.cs
- SecurityKeyUsage.cs
- SparseMemoryStream.cs
- CodeVariableReferenceExpression.cs
- ResourceExpressionBuilder.cs
- _IPv4Address.cs
- UnaryOperationBinder.cs
- SerializableAttribute.cs
- Connection.cs
- SendMailErrorEventArgs.cs
- SimpleLine.cs
- DataSvcMapFileSerializer.cs
- HandlerFactoryCache.cs
- SkipStoryboardToFill.cs
- WmlLabelAdapter.cs
- Geometry.cs
- DataProtection.cs
- TextServicesHost.cs
- HMACSHA512.cs
- MetadataExporter.cs
- FormViewModeEventArgs.cs
- Pair.cs
- VisualProxy.cs
- CompilationRelaxations.cs
- DesignerTextBoxAdapter.cs
- StorageRoot.cs
- TypedTableBase.cs
- ZipFileInfo.cs
- WindowsEditBox.cs
- ColumnMapProcessor.cs
- ScriptingJsonSerializationSection.cs
- BitmapEffectGroup.cs
- PageParserFilter.cs
- WebPartHelpVerb.cs
- Vector3DAnimationBase.cs
- SerializationAttributes.cs
- LocalizationParserHooks.cs
- IIS7WorkerRequest.cs
- OdbcCommandBuilder.cs
- HttpHandlersInstallComponent.cs
- RawStylusInputCustomData.cs
- ComponentResourceKey.cs
- DataGridViewTextBoxCell.cs
- PointAnimationClockResource.cs
- AnyReturnReader.cs
- HierarchicalDataBoundControlAdapter.cs
- SyndicationDeserializer.cs
- WizardPanel.cs
- AutomationPeer.cs
- SHA1Managed.cs
- FastPropertyAccessor.cs
- TextSearch.cs
- ColorConverter.cs
- PasswordTextNavigator.cs
- StorageMappingFragment.cs
- LinqDataSourceContextEventArgs.cs
- ProxyHwnd.cs
- TriggerCollection.cs
- OpenFileDialog.cs
- DataGridViewRowCancelEventArgs.cs
- ParallelEnumerableWrapper.cs
- FlowDocumentPage.cs