Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Speech / Src / Internal / Synthesis / EngineSiteSapi.cs / 1 / EngineSiteSapi.cs
//------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // // // // History: // 2/1/2005 [....] Created from the Sapi Managed code //----------------------------------------------------------------- using System; using System.IO; using System.Runtime.InteropServices; using System.Runtime.InteropServices.ComTypes; using System.Speech.Synthesis.TtsEngine; using System.Speech.Internal.SapiInterop; #pragma warning disable 1634, 1691 // Allows suppression of certain PreSharp messages. #pragma warning disable 56500 // Remove all the catch all statements warnings used by the interop layer namespace System.Speech.Internal.Synthesis { [ComVisible (true)] internal class EngineSiteSapi : ISpEngineSite { //******************************************************************* // // Constructors // //******************************************************************* #region Constructors internal EngineSiteSapi (EngineSite site, ResourceLoader resourceLoader) { _site = site; } #endregion //******************************************************************** // // Internal Methods // //******************************************************************* #region Internal Methods ////// Adds events directly to an event sink. /// /// /// void ISpEngineSite.AddEvents ([MarshalAs (UnmanagedType.LPArray, SizeParamIndex = 1)] SpeechEventSapi [] eventsSapi, int ulCount) { SpeechEventInfo [] events = new SpeechEventInfo [eventsSapi.Length]; for (int i = 0; i < eventsSapi.Length; i++) { SpeechEventSapi sapiEvt = eventsSapi [i]; events [i].EventId = sapiEvt.EventId; events [i].ParameterType = sapiEvt.ParameterType; events [i].Param1 = (int) sapiEvt.Param1; events [i].Param2 = sapiEvt.Param2; } _site.AddEvents (events, ulCount); } ////// Passes back the event interest for the voice. /// void ISpEngineSite.GetEventInterest (out Int64 eventInterest) { eventInterest = (uint) _site.EventInterest; } ////// Queries the voice object to determine which real-time action(s) to perform /// ///[PreserveSig] int ISpEngineSite.GetActions () { return (int) _site.Actions; } /// /// Queries the voice object to determine which real-time action(s) to perform. /// /// /// /// void ISpEngineSite.Write (IntPtr pBuff, int cb, IntPtr pcbWritten) { pcbWritten = (IntPtr) _site.Write (pBuff, cb); } ////// Retrieves the current TTS rendering rate adjustment that should be used by the engine. /// /// void ISpEngineSite.GetRate (out int pRateAdjust) { pRateAdjust = _site.Rate; } ////// Retrieves the base output volume level the engine should use during synthesis. /// /// void ISpEngineSite.GetVolume (out Int16 pusVolume) { pusVolume = (Int16) _site.Volume; } ////// Retrieves the number and type of items to be skipped in the text stream. /// /// /// void ISpEngineSite.GetSkipInfo (out int peType, out int plNumItems) { SkipInfo si = _site.GetSkipInfo (); if (si != null) { peType = si.Type; plNumItems = si.Count; } else { peType = (int) 1; // BSPVSKIPTYPE.SPVST_SENTENCE; plNumItems = 0; } } ////// Notifies that the last skip request has been completed and to pass it the results. /// /// void ISpEngineSite.CompleteSkip (int ulNumSkipped) { _site.CompleteSkip (ulNumSkipped); } ////// Load a file either from a local network or from the Internet. /// /// /// /// void ISpEngineSite.LoadResource (string uri, ref string mediaType, out IStream stream) { mediaType = null; #pragma warning disable 56518 // BinaryReader can't be disposed because underlying stream still in use. try { // Get the mime type Stream localStream = _site.LoadResource (new Uri (uri, UriKind.RelativeOrAbsolute), mediaType); BinaryReader reader = new BinaryReader (localStream); byte [] waveFormat = System.Speech.Internal.Synthesis.AudioBase.GetWaveFormat (reader); mediaType = null; if (waveFormat != null) { WAVEFORMATEX hdr = WAVEFORMATEX.ToWaveHeader (waveFormat); switch ((WaveFormatId) hdr.wFormatTag) { case WaveFormatId.Alaw: case WaveFormatId.Mulaw: case WaveFormatId.Pcm: mediaType = "audio/x-wav"; break; } } localStream.Position = 0; stream = new SpStreamWrapper (localStream); } catch { stream = null; } #pragma warning restore 56518 } #endregion //******************************************************************** // // Private Fields // //******************************************************************** #region private Fields private EngineSite _site; private enum WaveFormatId { Pcm = 1, Alaw = 0x0006, Mulaw = 0x0007, } #endregion } //******************************************************************* // // Internal Interfaces // //******************************************************************** #region Internal Interfaces ////// TODOC /// [ComImport, Guid ("9880499B-CCE9-11D2-B503-00C04F797396"), System.Runtime.InteropServices.InterfaceTypeAttribute (1)] internal interface ISpEngineSite { ////// TODOC /// void AddEvents ([MarshalAs (UnmanagedType.LPArray, SizeParamIndex = 1)] SpeechEventSapi [] events, int count); ////// TODOC /// void GetEventInterest (out Int64 eventInterest); ////// TODOC /// [PreserveSig] int GetActions (); ////// TODOC /// void Write (IntPtr data, int count, IntPtr bytesWritten); ////// TODOC /// void GetRate (out int rate); ////// TODOC /// void GetVolume (out Int16 volume); ////// TODOC /// void GetSkipInfo (out int type, out int count); ////// TODOC /// void CompleteSkip (int skipped); ////// TODOC /// void LoadResource ([MarshalAs(UnmanagedType.LPWStr)] string resource, ref string mediaType, out IStream stream); } ////// TODOC /// [StructLayout (LayoutKind.Sequential)] internal struct SpeechEventSapi { ////// TODOC /// public Int16 EventId; ////// TODOC /// public Int16 ParameterType; ////// TODOC /// public Int32 StreamNumber; ////// TODOC /// public Int64 AudioStreamOffset; ////// TODOC /// public IntPtr Param1; // Always just a numeric type - contains no unmanaged resources so does not need special clean-up. ////// TODOC /// public IntPtr Param2; // Can be a numeric type, or pointer to string or object. Use SafeSapiLParamHandle to cleanup. /// TODOC public static bool operator == (SpeechEventSapi event1, SpeechEventSapi event2) { return event1.EventId == event2.EventId && event1.ParameterType == event2.ParameterType && event1.StreamNumber == event2.StreamNumber && event1.AudioStreamOffset == event2.AudioStreamOffset && event1.Param1 == event2.Param1 && event1.Param2 == event2.Param2; } /// TODOC public static bool operator != (SpeechEventSapi event1, SpeechEventSapi event2) { return !(event1 == event2); } /// TODOC public override bool Equals (object obj) { if (!(obj is SpeechEventSapi)) { return false; } return this == (SpeechEventSapi) obj; } /// TODOC public override int GetHashCode () { return base.GetHashCode (); } } #endregion } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
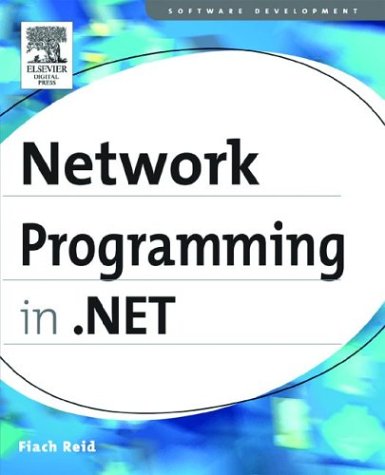
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- NonDualMessageSecurityOverHttpElement.cs
- OleDbPropertySetGuid.cs
- PhysicalOps.cs
- Executor.cs
- AnimationClock.cs
- StringTraceRecord.cs
- Events.cs
- GZipStream.cs
- PartialTrustVisibleAssembliesSection.cs
- Span.cs
- ASCIIEncoding.cs
- Win32SafeHandles.cs
- PageCatalogPart.cs
- FormClosedEvent.cs
- Repeater.cs
- ModifierKeysValueSerializer.cs
- SqlTriggerAttribute.cs
- StrokeNodeOperations2.cs
- ApplicationHost.cs
- HttpRuntime.cs
- IDReferencePropertyAttribute.cs
- GeometryDrawing.cs
- DoubleAnimationBase.cs
- TableMethodGenerator.cs
- DelayedRegex.cs
- DBBindings.cs
- PaintValueEventArgs.cs
- TextRangeEditTables.cs
- TypeExtension.cs
- SystemFonts.cs
- SqlTriggerContext.cs
- WebPartVerbCollection.cs
- ListViewInsertEventArgs.cs
- Vector3D.cs
- ClickablePoint.cs
- CipherData.cs
- Model3DCollection.cs
- COM2FontConverter.cs
- ScriptingScriptResourceHandlerSection.cs
- XmlSerializationGeneratedCode.cs
- DateTimeConstantAttribute.cs
- HiddenFieldPageStatePersister.cs
- ServerType.cs
- DetailsViewUpdateEventArgs.cs
- UserInitiatedRoutedEventPermission.cs
- CodeStatementCollection.cs
- ItemCheckedEvent.cs
- ClientCredentialsElement.cs
- CssStyleCollection.cs
- EventLogPermissionAttribute.cs
- ContextMenu.cs
- StrokeNodeOperations.cs
- WebPartHelpVerb.cs
- WebDescriptionAttribute.cs
- TransformCollection.cs
- JpegBitmapDecoder.cs
- XPathAncestorQuery.cs
- ObjectSet.cs
- ToolboxItemFilterAttribute.cs
- ObjectParameter.cs
- ProcessThreadDesigner.cs
- ExceptionHelpers.cs
- VirtualPathUtility.cs
- XComponentModel.cs
- PasswordBoxAutomationPeer.cs
- EndpointInfoCollection.cs
- TextBoxLine.cs
- OptimisticConcurrencyException.cs
- StatusBarPanel.cs
- ParseElementCollection.cs
- ProfessionalColorTable.cs
- BindableTemplateBuilder.cs
- VirtualPath.cs
- LayoutEditorPart.cs
- ObjectHandle.cs
- BorderGapMaskConverter.cs
- MethodBuilderInstantiation.cs
- ColumnPropertiesGroup.cs
- UserPreferenceChangedEventArgs.cs
- SeekableReadStream.cs
- URI.cs
- TerminateDesigner.cs
- BamlRecords.cs
- KeyboardDevice.cs
- XmlTextReader.cs
- GcHandle.cs
- ScriptingScriptResourceHandlerSection.cs
- FrameworkPropertyMetadata.cs
- UserControlBuildProvider.cs
- DecodeHelper.cs
- ChangeDirector.cs
- WebConfigurationHostFileChange.cs
- StorageRoot.cs
- RefreshPropertiesAttribute.cs
- TlsnegoTokenAuthenticator.cs
- SkewTransform.cs
- BehaviorEditorPart.cs
- ManipulationCompletedEventArgs.cs
- MSAANativeProvider.cs
- BuilderPropertyEntry.cs