Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Sys / System / IO / compression / GZipStream.cs / 1305376 / GZipStream.cs
namespace System.IO.Compression { using System.IO; using System.Diagnostics; using System.Security.Permissions; public class GZipStream : Stream { private DeflateStream deflateStream; public GZipStream(Stream stream, CompressionMode mode) : this( stream, mode, false) { } public GZipStream(Stream stream, CompressionMode mode, bool leaveOpen) { deflateStream = new DeflateStream(stream, mode, leaveOpen); if (mode == CompressionMode.Compress) { IFileFormatWriter writeCommand = new GZipFormatter(); deflateStream.SetFileFormatWriter(writeCommand); } else { IFileFormatReader readCommand = new GZipDecoder(); deflateStream.SetFileFormatReader(readCommand); } } public override bool CanRead { get { if( deflateStream == null) { return false; } return deflateStream.CanRead; } } public override bool CanWrite { get { if( deflateStream == null) { return false; } return deflateStream.CanWrite; } } public override bool CanSeek { get { if( deflateStream == null) { return false; } return deflateStream.CanSeek; } } public override long Length { get { throw new NotSupportedException(SR.GetString(SR.NotSupported)); } } public override long Position { get { throw new NotSupportedException(SR.GetString(SR.NotSupported)); } set { throw new NotSupportedException(SR.GetString(SR.NotSupported)); } } public override void Flush() { if( deflateStream == null) { throw new ObjectDisposedException(null, SR.GetString(SR.ObjectDisposed_StreamClosed)); } deflateStream.Flush(); return; } public override long Seek(long offset, SeekOrigin origin) { throw new NotSupportedException(SR.GetString(SR.NotSupported)); } public override void SetLength(long value) { throw new NotSupportedException(SR.GetString(SR.NotSupported)); } [HostProtection(ExternalThreading=true)] public override IAsyncResult BeginRead(byte[] array, int offset, int count, AsyncCallback asyncCallback, object asyncState) { if( deflateStream == null) { throw new InvalidOperationException(SR.GetString(SR.ObjectDisposed_StreamClosed)); } return deflateStream.BeginRead(array, offset, count, asyncCallback, asyncState); } public override int EndRead(IAsyncResult asyncResult) { if( deflateStream == null) { throw new InvalidOperationException(SR.GetString(SR.ObjectDisposed_StreamClosed)); } return deflateStream.EndRead(asyncResult); } [HostProtection(ExternalThreading=true)] public override IAsyncResult BeginWrite(byte[] array, int offset, int count, AsyncCallback asyncCallback, object asyncState) { if( deflateStream == null) { throw new InvalidOperationException(SR.GetString(SR.ObjectDisposed_StreamClosed)); } return deflateStream.BeginWrite(array, offset, count, asyncCallback, asyncState); } public override void EndWrite(IAsyncResult asyncResult) { if( deflateStream == null) { throw new InvalidOperationException(SR.GetString(SR.ObjectDisposed_StreamClosed)); } deflateStream.EndWrite(asyncResult); } public override int Read(byte[] array, int offset, int count) { if( deflateStream == null) { throw new ObjectDisposedException(null, SR.GetString(SR.ObjectDisposed_StreamClosed)); } return deflateStream.Read(array, offset, count); } public override void Write(byte[] array, int offset, int count) { if( deflateStream == null) { throw new ObjectDisposedException(null, SR.GetString(SR.ObjectDisposed_StreamClosed)); } deflateStream.Write(array, offset, count); } protected override void Dispose(bool disposing) { try { if (disposing && deflateStream != null) { deflateStream.Close(); } deflateStream = null; } finally { base.Dispose(disposing); } } public Stream BaseStream { get { if( deflateStream != null) { return deflateStream.BaseStream; } else { return null; } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. namespace System.IO.Compression { using System.IO; using System.Diagnostics; using System.Security.Permissions; public class GZipStream : Stream { private DeflateStream deflateStream; public GZipStream(Stream stream, CompressionMode mode) : this( stream, mode, false) { } public GZipStream(Stream stream, CompressionMode mode, bool leaveOpen) { deflateStream = new DeflateStream(stream, mode, leaveOpen); if (mode == CompressionMode.Compress) { IFileFormatWriter writeCommand = new GZipFormatter(); deflateStream.SetFileFormatWriter(writeCommand); } else { IFileFormatReader readCommand = new GZipDecoder(); deflateStream.SetFileFormatReader(readCommand); } } public override bool CanRead { get { if( deflateStream == null) { return false; } return deflateStream.CanRead; } } public override bool CanWrite { get { if( deflateStream == null) { return false; } return deflateStream.CanWrite; } } public override bool CanSeek { get { if( deflateStream == null) { return false; } return deflateStream.CanSeek; } } public override long Length { get { throw new NotSupportedException(SR.GetString(SR.NotSupported)); } } public override long Position { get { throw new NotSupportedException(SR.GetString(SR.NotSupported)); } set { throw new NotSupportedException(SR.GetString(SR.NotSupported)); } } public override void Flush() { if( deflateStream == null) { throw new ObjectDisposedException(null, SR.GetString(SR.ObjectDisposed_StreamClosed)); } deflateStream.Flush(); return; } public override long Seek(long offset, SeekOrigin origin) { throw new NotSupportedException(SR.GetString(SR.NotSupported)); } public override void SetLength(long value) { throw new NotSupportedException(SR.GetString(SR.NotSupported)); } [HostProtection(ExternalThreading=true)] public override IAsyncResult BeginRead(byte[] array, int offset, int count, AsyncCallback asyncCallback, object asyncState) { if( deflateStream == null) { throw new InvalidOperationException(SR.GetString(SR.ObjectDisposed_StreamClosed)); } return deflateStream.BeginRead(array, offset, count, asyncCallback, asyncState); } public override int EndRead(IAsyncResult asyncResult) { if( deflateStream == null) { throw new InvalidOperationException(SR.GetString(SR.ObjectDisposed_StreamClosed)); } return deflateStream.EndRead(asyncResult); } [HostProtection(ExternalThreading=true)] public override IAsyncResult BeginWrite(byte[] array, int offset, int count, AsyncCallback asyncCallback, object asyncState) { if( deflateStream == null) { throw new InvalidOperationException(SR.GetString(SR.ObjectDisposed_StreamClosed)); } return deflateStream.BeginWrite(array, offset, count, asyncCallback, asyncState); } public override void EndWrite(IAsyncResult asyncResult) { if( deflateStream == null) { throw new InvalidOperationException(SR.GetString(SR.ObjectDisposed_StreamClosed)); } deflateStream.EndWrite(asyncResult); } public override int Read(byte[] array, int offset, int count) { if( deflateStream == null) { throw new ObjectDisposedException(null, SR.GetString(SR.ObjectDisposed_StreamClosed)); } return deflateStream.Read(array, offset, count); } public override void Write(byte[] array, int offset, int count) { if( deflateStream == null) { throw new ObjectDisposedException(null, SR.GetString(SR.ObjectDisposed_StreamClosed)); } deflateStream.Write(array, offset, count); } protected override void Dispose(bool disposing) { try { if (disposing && deflateStream != null) { deflateStream.Close(); } deflateStream = null; } finally { base.Dispose(disposing); } } public Stream BaseStream { get { if( deflateStream != null) { return deflateStream.BaseStream; } else { return null; } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
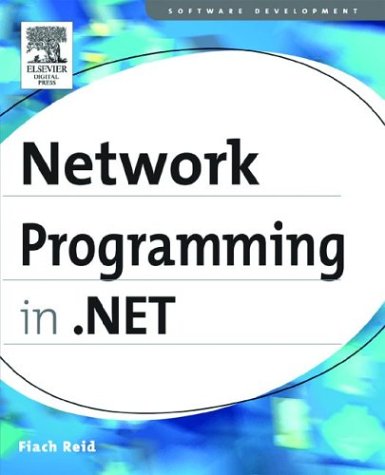
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Wow64ConfigurationLoader.cs
- PropertyMapper.cs
- CodeDOMProvider.cs
- HttpWriter.cs
- Identifier.cs
- SchemaLookupTable.cs
- InfoCardRSAPKCS1KeyExchangeFormatter.cs
- CatalogPartCollection.cs
- LinkDesigner.cs
- WindowsScrollBar.cs
- UriParserTemplates.cs
- UserControl.cs
- VirtualPathUtility.cs
- SafeNativeMethods.cs
- WindowsListViewSubItem.cs
- DocumentGridPage.cs
- RowParagraph.cs
- TreeNodeMouseHoverEvent.cs
- UIElement.cs
- CommandBindingCollection.cs
- _BasicClient.cs
- COM2PictureConverter.cs
- RelationshipEnd.cs
- BoundConstants.cs
- XsltOutput.cs
- ValueConversionAttribute.cs
- EraserBehavior.cs
- ManagementObject.cs
- DataGridViewLinkColumn.cs
- ActiveXHost.cs
- TrackingRecord.cs
- ObjectIDGenerator.cs
- TableRowCollection.cs
- ColumnTypeConverter.cs
- JsonWriter.cs
- AssemblyResourceLoader.cs
- ApplicationCommands.cs
- DiscreteKeyFrames.cs
- WebDescriptionAttribute.cs
- OdbcConnectionFactory.cs
- RunClient.cs
- ListViewItem.cs
- DocumentViewerBase.cs
- ShapeTypeface.cs
- UnsafePeerToPeerMethods.cs
- NetworkCredential.cs
- Crypto.cs
- DetailsViewRow.cs
- ClientScriptManagerWrapper.cs
- DataRowView.cs
- Label.cs
- WindowsFont.cs
- ServiceSecurityAuditBehavior.cs
- TraceContextEventArgs.cs
- ExpressionHelper.cs
- XmlEventCache.cs
- SystemWebCachingSectionGroup.cs
- BitmapEffectGroup.cs
- ProcessDesigner.cs
- XmlTextReaderImpl.cs
- ModuleConfigurationInfo.cs
- DSASignatureFormatter.cs
- AnimationTimeline.cs
- XmlSchemaImport.cs
- QuadraticBezierSegment.cs
- XPathDescendantIterator.cs
- TextWriter.cs
- RangeBase.cs
- SqlFileStream.cs
- ConfigXmlElement.cs
- ProvideValueServiceProvider.cs
- DataReceivedEventArgs.cs
- ForceCopyBuildProvider.cs
- DoubleStorage.cs
- _SingleItemRequestCache.cs
- DataGridViewTextBoxColumn.cs
- SetStateEventArgs.cs
- XsdDateTime.cs
- OleDbWrapper.cs
- DateTimeStorage.cs
- TypeTypeConverter.cs
- WSSecureConversationFeb2005.cs
- SplineKeyFrames.cs
- DropShadowEffect.cs
- TemplateControlCodeDomTreeGenerator.cs
- DesignerActionUI.cs
- WorkflowNamespace.cs
- ListViewDeleteEventArgs.cs
- EntityContainerAssociationSet.cs
- SectionRecord.cs
- SpStreamWrapper.cs
- DataKeyArray.cs
- WebServiceParameterData.cs
- SecurityKeyEntropyMode.cs
- EntityCollection.cs
- ApplicationSecurityManager.cs
- Membership.cs
- RoleManagerSection.cs
- BmpBitmapDecoder.cs
- PermissionSetTriple.cs