Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / BuildTasks / MS / Internal / Localization / LocalizationParserHooks.cs / 1 / LocalizationParserHooks.cs
//---------------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // Description: LocalizationParserHooks. // // History: // 06/16/2005 garyyang - created // //--------------------------------------------------------------------------- using System; using System.Collections; using System.IO; using System.Xml; using System.Text; using MS.Internal.Globalization; using MS.Internal.Markup; using MS.Internal.Tasks; namespace MS.Internal { internal sealed class LocalizationParserHooks : ParserHooks { private MarkupCompiler _compiler; private ArrayList _commentList; private LocalizationComment _currentComment; private LocalizationDirectivesToLocFile _directivesToFile; private bool _isSecondPass; internal LocalizationParserHooks( MarkupCompiler compiler , LocalizationDirectivesToLocFile directivesToFile, bool isSecondPass ) { _compiler = compiler; _directivesToFile = directivesToFile; _isSecondPass = isSecondPass; // The arrray list holds all the comments collected while parsing Xaml. _commentList = new ArrayList(); // It is the comments current being processed. _currentComment = new LocalizationComment(); } internal override ParserAction LoadNode(XamlNode tokenNode) { switch (tokenNode.TokenType) { case XamlNodeType.DocumentStart : { // A single ParserHooks might be used to parse multiple bamls. // We need to clear the comments list at the begining of each baml. _commentList.Clear(); _currentComment = new LocalizationComment(); return ParserAction.Normal; } case XamlNodeType.DefAttribute : { XamlDefAttributeNode node = (XamlDefAttributeNode) tokenNode; if (node.Name == XamlReaderHelper.DefinitionUid) { _currentComment.Uid = node.Value; } return ParserAction.Normal; } case XamlNodeType.Property : { XamlPropertyNode node = (XamlPropertyNode) tokenNode; // When this parer hook is invoked, comments is always output to a seperate file. if (LocComments.IsLocCommentsProperty(node.TypeFullName, node.PropName)) { // try parse the value. Exception will be thrown if not valid. LocComments.ParsePropertyComments(node.Value); _currentComment.Comments = node.Value; return ParserAction.Skip; // skips outputing this node to baml } if ( _directivesToFile == LocalizationDirectivesToLocFile.All && LocComments.IsLocLocalizabilityProperty(node.TypeFullName, node.PropName)) { // try parse the value. Exception will be thrown if not valid. LocComments.ParsePropertyLocalizabilityAttributes(node.Value); _currentComment.Attributes = node.Value; return ParserAction.Skip; // skips outputing this node to baml } return ParserAction.Normal; } case XamlNodeType.EndAttributes : { FlushCommentToList(ref _currentComment); return ParserAction.Normal; } case XamlNodeType.DocumentEnd : { // // When reaching document end, we output all the comments we have collected // so far into a localization comment file. If the parsing was aborted in // MarkupCompilePass1, we would not out the incomplete set of comments because // it will not reach document end. // if (_commentList.Count > 0) { string absoluteOutputPath = _compiler.TargetPath + _compiler.SourceFileInfo.RelativeSourceFilePath + SharedStrings.LocExtension; MemoryStream memStream = new MemoryStream(); // TaskFileService.WriteFile adds BOM for UTF8 Encoding, thus don't add here // when creating XmlTextWriter. using (XmlTextWriter writer = new XmlTextWriter(memStream, new UTF8Encoding(false))) { // output XML for each piece of comment writer.Formatting = Formatting.Indented; writer.WriteStartElement(LocComments.LocResourcesElement); writer.WriteAttributeString(LocComments.LocFileNameAttribute, _compiler.SourceFileInfo.RelativeSourceFilePath); foreach (LocalizationComment comment in _commentList) { writer.WriteStartElement(LocComments.LocCommentsElement); writer.WriteAttributeString(LocComments.LocCommentIDAttribute, comment.Uid); if (comment.Attributes != null) { writer.WriteAttributeString(LocComments.LocLocalizabilityAttribute, comment.Attributes); } if (comment.Comments != null) { writer.WriteAttributeString(LocComments.LocCommentsAttribute, comment.Comments); } writer.WriteEndElement(); } writer.WriteEndElement(); writer.Flush(); _compiler.TaskFileService.WriteFile(memStream.ToArray(), absoluteOutputPath); } } return ParserAction.Normal; } default: { return ParserAction.Normal; } } } ////// Add the existing comment into the cached list and clear the state for the /// next incoming comment. Comments are only collected in pass1. The method is no-op /// in pass2. /// private void FlushCommentToList(ref LocalizationComment comment) { if (_isSecondPass) return; if ( _currentComment.Uid != null && ( _currentComment.Attributes != null || _currentComment.Comments != null ) ) { // add the comments into the list and reset _commentList.Add(_currentComment); _currentComment = new LocalizationComment(); } else { // clear all properties _currentComment.Uid = _currentComment.Attributes = _currentComment.Comments = null; } } private class LocalizationComment { internal string Uid; internal string Comments; internal string Attributes; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // Description: LocalizationParserHooks. // // History: // 06/16/2005 garyyang - created // //--------------------------------------------------------------------------- using System; using System.Collections; using System.IO; using System.Xml; using System.Text; using MS.Internal.Globalization; using MS.Internal.Markup; using MS.Internal.Tasks; namespace MS.Internal { internal sealed class LocalizationParserHooks : ParserHooks { private MarkupCompiler _compiler; private ArrayList _commentList; private LocalizationComment _currentComment; private LocalizationDirectivesToLocFile _directivesToFile; private bool _isSecondPass; internal LocalizationParserHooks( MarkupCompiler compiler , LocalizationDirectivesToLocFile directivesToFile, bool isSecondPass ) { _compiler = compiler; _directivesToFile = directivesToFile; _isSecondPass = isSecondPass; // The arrray list holds all the comments collected while parsing Xaml. _commentList = new ArrayList(); // It is the comments current being processed. _currentComment = new LocalizationComment(); } internal override ParserAction LoadNode(XamlNode tokenNode) { switch (tokenNode.TokenType) { case XamlNodeType.DocumentStart : { // A single ParserHooks might be used to parse multiple bamls. // We need to clear the comments list at the begining of each baml. _commentList.Clear(); _currentComment = new LocalizationComment(); return ParserAction.Normal; } case XamlNodeType.DefAttribute : { XamlDefAttributeNode node = (XamlDefAttributeNode) tokenNode; if (node.Name == XamlReaderHelper.DefinitionUid) { _currentComment.Uid = node.Value; } return ParserAction.Normal; } case XamlNodeType.Property : { XamlPropertyNode node = (XamlPropertyNode) tokenNode; // When this parer hook is invoked, comments is always output to a seperate file. if (LocComments.IsLocCommentsProperty(node.TypeFullName, node.PropName)) { // try parse the value. Exception will be thrown if not valid. LocComments.ParsePropertyComments(node.Value); _currentComment.Comments = node.Value; return ParserAction.Skip; // skips outputing this node to baml } if ( _directivesToFile == LocalizationDirectivesToLocFile.All && LocComments.IsLocLocalizabilityProperty(node.TypeFullName, node.PropName)) { // try parse the value. Exception will be thrown if not valid. LocComments.ParsePropertyLocalizabilityAttributes(node.Value); _currentComment.Attributes = node.Value; return ParserAction.Skip; // skips outputing this node to baml } return ParserAction.Normal; } case XamlNodeType.EndAttributes : { FlushCommentToList(ref _currentComment); return ParserAction.Normal; } case XamlNodeType.DocumentEnd : { // // When reaching document end, we output all the comments we have collected // so far into a localization comment file. If the parsing was aborted in // MarkupCompilePass1, we would not out the incomplete set of comments because // it will not reach document end. // if (_commentList.Count > 0) { string absoluteOutputPath = _compiler.TargetPath + _compiler.SourceFileInfo.RelativeSourceFilePath + SharedStrings.LocExtension; MemoryStream memStream = new MemoryStream(); // TaskFileService.WriteFile adds BOM for UTF8 Encoding, thus don't add here // when creating XmlTextWriter. using (XmlTextWriter writer = new XmlTextWriter(memStream, new UTF8Encoding(false))) { // output XML for each piece of comment writer.Formatting = Formatting.Indented; writer.WriteStartElement(LocComments.LocResourcesElement); writer.WriteAttributeString(LocComments.LocFileNameAttribute, _compiler.SourceFileInfo.RelativeSourceFilePath); foreach (LocalizationComment comment in _commentList) { writer.WriteStartElement(LocComments.LocCommentsElement); writer.WriteAttributeString(LocComments.LocCommentIDAttribute, comment.Uid); if (comment.Attributes != null) { writer.WriteAttributeString(LocComments.LocLocalizabilityAttribute, comment.Attributes); } if (comment.Comments != null) { writer.WriteAttributeString(LocComments.LocCommentsAttribute, comment.Comments); } writer.WriteEndElement(); } writer.WriteEndElement(); writer.Flush(); _compiler.TaskFileService.WriteFile(memStream.ToArray(), absoluteOutputPath); } } return ParserAction.Normal; } default: { return ParserAction.Normal; } } } ////// Add the existing comment into the cached list and clear the state for the /// next incoming comment. Comments are only collected in pass1. The method is no-op /// in pass2. /// private void FlushCommentToList(ref LocalizationComment comment) { if (_isSecondPass) return; if ( _currentComment.Uid != null && ( _currentComment.Attributes != null || _currentComment.Comments != null ) ) { // add the comments into the list and reset _commentList.Add(_currentComment); _currentComment = new LocalizationComment(); } else { // clear all properties _currentComment.Uid = _currentComment.Attributes = _currentComment.Comments = null; } } private class LocalizationComment { internal string Uid; internal string Comments; internal string Attributes; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
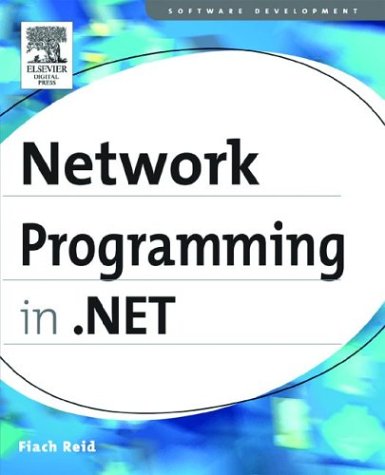
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- GreenMethods.cs
- Int16Animation.cs
- LogRecordSequence.cs
- HttpContextBase.cs
- EntityDataSourceValidationException.cs
- EntityConnectionStringBuilder.cs
- AssemblyCacheEntry.cs
- ExpressionNormalizer.cs
- DataMemberAttribute.cs
- ExcludeFromCodeCoverageAttribute.cs
- X500Name.cs
- ManagementObject.cs
- PagePropertiesChangingEventArgs.cs
- DropDownHolder.cs
- CacheHelper.cs
- NegotiateStream.cs
- GPRECT.cs
- XmlWrappingReader.cs
- TextTrailingCharacterEllipsis.cs
- GridLengthConverter.cs
- MetabaseSettings.cs
- GeometryModel3D.cs
- ProtectedConfigurationSection.cs
- UrlPath.cs
- SimpleHandlerBuildProvider.cs
- EventBuilder.cs
- FileClassifier.cs
- ObjectManager.cs
- DbgCompiler.cs
- PriorityBinding.cs
- ContentPlaceHolder.cs
- TextFormatter.cs
- DependencyPropertyKind.cs
- StateRuntime.cs
- ScrollChrome.cs
- SrgsDocument.cs
- Vector3DAnimationUsingKeyFrames.cs
- UndoUnit.cs
- MobileListItem.cs
- PhysicalAddress.cs
- XhtmlBasicSelectionListAdapter.cs
- DataGridViewLinkCell.cs
- BaseCodePageEncoding.cs
- TargetConverter.cs
- TypefaceMap.cs
- cookiecollection.cs
- QueryConverter.cs
- StringSource.cs
- RoutedEventArgs.cs
- CommonDialog.cs
- Span.cs
- DurableMessageDispatchInspector.cs
- RSACryptoServiceProvider.cs
- FontWeights.cs
- UITypeEditors.cs
- ToolTip.cs
- UriParserTemplates.cs
- XmlUtil.cs
- ReachNamespaceInfo.cs
- RegexCaptureCollection.cs
- xdrvalidator.cs
- CategoryEditor.cs
- RequestCachingSection.cs
- HtmlMeta.cs
- ErrorEventArgs.cs
- EnvelopedPkcs7.cs
- SessionStateUtil.cs
- XmlLanguage.cs
- FixedPageStructure.cs
- AnnotationResourceCollection.cs
- DataGridPagerStyle.cs
- HelloOperationAsyncResult.cs
- JavascriptCallbackBehaviorAttribute.cs
- TextPointer.cs
- SHA1Cng.cs
- MethodExpr.cs
- CodeSubDirectoriesCollection.cs
- IdleTimeoutMonitor.cs
- DescendantBaseQuery.cs
- FilterElement.cs
- sqlser.cs
- FixedSOMPage.cs
- IISUnsafeMethods.cs
- DesignerActionItem.cs
- UIPropertyMetadata.cs
- XmlCDATASection.cs
- Path.cs
- SimpleHandlerBuildProvider.cs
- SymbolPair.cs
- ProcessHostMapPath.cs
- RenderDataDrawingContext.cs
- DataBindEngine.cs
- PointLightBase.cs
- SchemaImporter.cs
- ConfigurationSectionGroup.cs
- StringDictionary.cs
- RemotingConfigParser.cs
- Events.cs
- WebPartDisplayModeCollection.cs
- CallbackException.cs