Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx40 / System.ServiceModel.Activities / System / ServiceModel / Activities / ReceiveReply.cs / 1407647 / ReceiveReply.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.ServiceModel.Activities { using System.Activities; using System.Activities.Statements; using System.Collections.ObjectModel; using System.ComponentModel; using System.ServiceModel.Channels; using System.ServiceModel.Description; using System.ServiceModel.Dispatcher; using System.Windows.Markup; // Used to receive the reply in a request/reply messaging pattern. Must be paired with // a corresponding Send activity. [ContentProperty("Content")] public sealed class ReceiveReply : Activity { CollectioncorrelationInitializers; FromReply responseFormatter; InternalReceiveMessage internalReceive; public ReceiveReply() : base() { base.Implementation = () => { // if CacheMetadata isn't called, bail early if (this.internalReceive == null) { return null; } // requestFormatter is null if we have an untyped message situation if (this.responseFormatter == null) { return this.internalReceive; } else { Variable response = new Variable { Name = "ResponseMessage" }; this.internalReceive.Message = new OutArgument (response); this.responseFormatter.Message = new InArgument (response); return new NoPersistScope { Body = new Sequence { Variables = { response }, Activities = { this.internalReceive, this.responseFormatter, } } }; } }; } // the content to receive (either message or parameters-based) declared by the user [DefaultValue(null)] public ReceiveContent Content { get; set; } // Internally, we should always use InternalContent since this property may have default content that we added internal ReceiveContent InternalContent { get { return this.Content ?? ReceiveContent.DefaultReceiveContent; } } [DefaultValue(null)] public string Action { get; set; } // Reference to the Send activity that is responsible for sending the Request part of the // request/reply pattern. This cannot be null. [DefaultValue(null)] public Send Request { get; set; } // Additional correlations allow situations where a "session" involves multiple // messages between two workflow instances. public Collection CorrelationInitializers { get { if (this.correlationInitializers == null) { this.correlationInitializers = new Collection (); } return this.correlationInitializers; } } protected override void CacheMetadata(ActivityMetadata metadata) { if (this.Request == null) { metadata.AddValidationError(SR.ReceiveReplyRequestCannotBeNull(this.DisplayName)); } else { // Need to validate Send.ServiceContractName and Send.OperationName here so that we can proceed with contract inference if (this.Request.ServiceContractName == null) { string errorOperationName = ContractValidationHelper.GetErrorMessageOperationName(this.Request.OperationName); metadata.AddValidationError(SR.MissingServiceContractName(this.Request.DisplayName, errorOperationName)); } if (string.IsNullOrEmpty(this.Request.OperationName)) { metadata.AddValidationError(SR.MissingOperationName(this.Request.DisplayName)); } } // validate Correlation Initializers MessagingActivityHelper.ValidateCorrelationInitializer(metadata, this.correlationInitializers, true, this.DisplayName, (this.Request != null ? this.Request.OperationName : String.Empty)); // Validate Content string operationName = this.Request != null ? this.Request.OperationName : null; this.InternalContent.CacheMetadata(metadata, this, operationName); if (this.correlationInitializers != null) { for (int i = 0; i < this.correlationInitializers.Count; i++) { CorrelationInitializer initializer = this.correlationInitializers[i]; initializer.ArgumentName = Constants.Parameter + i; RuntimeArgument initializerArgument = new RuntimeArgument(initializer.ArgumentName, Constants.CorrelationHandleType, ArgumentDirection.In); metadata.Bind(initializer.CorrelationHandle, initializerArgument); metadata.AddArgument(initializerArgument); } } if (!metadata.HasViolations) { this.internalReceive = CreateInternalReceive(); InArgument requestReplyHandleFromSend = GetReplyHandleFromSend(); if (requestReplyHandleFromSend != null) { InArgument resultCorrelatesWith = MessagingActivityHelper.CreateReplyCorrelatesWith(requestReplyHandleFromSend); RuntimeArgument resultCorrelatesWithArgument = new RuntimeArgument("ResultCorrelatesWith", Constants.CorrelationHandleType, ArgumentDirection.In); metadata.Bind(resultCorrelatesWith, resultCorrelatesWithArgument); metadata.AddArgument(resultCorrelatesWithArgument); this.internalReceive.CorrelatesWith = (InArgument )InArgument.CreateReference(resultCorrelatesWith, "ResultCorrelatesWith"); } this.InternalContent.ConfigureInternalReceiveReply(this.internalReceive, out this.responseFormatter); if (this.InternalContent is ReceiveMessageContent && MessageBuilder.IsMessageContract(((ReceiveMessageContent)this.InternalContent).InternalDeclaredMessageType)) { this.Request.OperationUsesMessageContract = true; } OperationDescription operation = ContractInferenceHelper.CreateTwoWayOperationDescription(this.Request, this); this.Request.OperationDescription = operation; if (this.responseFormatter != null) { IClientMessageFormatter formatter = ClientOperationFormatterProvider.GetFormatterFromRuntime(operation); this.Request.SetFormatter(formatter); this.responseFormatter.Formatter = formatter; // int index = 0; Type[] faultTypes = new Type[operation.KnownTypes.Count]; foreach (Type type in operation.KnownTypes) { faultTypes[index] = type; index++; } this.responseFormatter.FaultFormatter = new FaultFormatter(faultTypes); } // Add CorrelationQuery to the Send->ReplyCorrelation, we validate that the same query is not added multiple times if (this.correlationInitializers != null && this.correlationInitializers.Count > 0) { Collection internalCorrelationQueryCollection = ContractInferenceHelper.CreateClientCorrelationQueries(null, this.correlationInitializers, this.Action, this.Request.ServiceContractName, this.Request.OperationName, true); foreach (CorrelationQuery query in internalCorrelationQueryCollection) { this.Request.SetReplyCorrelationQuery(query); } } } else { this.internalReceive = null; this.responseFormatter = null; } // We don't have any imported children despite referencing the Request metadata.SetImportedChildrenCollection(new Collection ()); } InternalReceiveMessage CreateInternalReceive() { InternalReceiveMessage result = new InternalReceiveMessage { IsOneWay = false }; if (this.correlationInitializers != null) { foreach (CorrelationInitializer correlation in this.correlationInitializers) { result.CorrelationInitializers.Add(correlation.Clone()); } } // Update the send->IsOneWay if (this.Request != null) { this.Request.SetIsOneWay(false); } return result; } InArgument GetReplyHandleFromSend() { if (this.Request != null) { // If user has set AdditionalCorrelations, then we need to first look for requestReply Handle there foreach (CorrelationInitializer correlation in this.Request.CorrelationInitializers) { RequestReplyCorrelationInitializer requestReplyCorrelation = correlation as RequestReplyCorrelationInitializer; if (requestReplyCorrelation != null && requestReplyCorrelation.CorrelationHandle != null) { return requestReplyCorrelation.CorrelationHandle; } } } return null; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
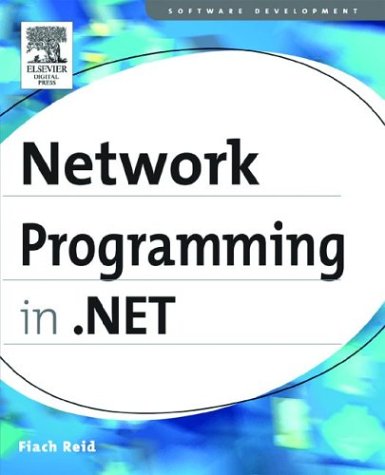
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TextServicesPropertyRanges.cs
- BinaryMessageFormatter.cs
- SerializationHelper.cs
- BuildManagerHost.cs
- QueryableDataSourceEditData.cs
- XmlValueConverter.cs
- PackageDigitalSignature.cs
- ByValueEqualityComparer.cs
- TimerTable.cs
- XmlTextReaderImpl.cs
- RTLAwareMessageBox.cs
- SystemParameters.cs
- PageEventArgs.cs
- EncoderBestFitFallback.cs
- ArcSegment.cs
- SoapEnumAttribute.cs
- ReliabilityContractAttribute.cs
- Attributes.cs
- LassoSelectionBehavior.cs
- TypeSource.cs
- MetafileHeader.cs
- SizeIndependentAnimationStorage.cs
- ZipIOExtraFieldElement.cs
- Style.cs
- XmlLanguageConverter.cs
- KeyValueSerializer.cs
- ChannelTerminatedException.cs
- SortDescriptionCollection.cs
- MSAAEventDispatcher.cs
- MenuItemBindingCollection.cs
- WebHostUnsafeNativeMethods.cs
- RadialGradientBrush.cs
- ConfigDefinitionUpdates.cs
- Vector3D.cs
- DigestComparer.cs
- TdsParameterSetter.cs
- DynamicPropertyHolder.cs
- DisplayNameAttribute.cs
- QueryableDataSourceHelper.cs
- WebPartTransformer.cs
- BaseAppDomainProtocolHandler.cs
- InstanceCompleteException.cs
- XmlSchemaInclude.cs
- WindowsIPAddress.cs
- StartUpEventArgs.cs
- IndentedTextWriter.cs
- RolePrincipal.cs
- SerialPort.cs
- MachineSettingsSection.cs
- TableRowCollection.cs
- HtmlWindowCollection.cs
- RemoteWebConfigurationHostServer.cs
- XmlEnumAttribute.cs
- AttributeData.cs
- WebPartEventArgs.cs
- RegexGroup.cs
- StrokeCollectionConverter.cs
- AssemblyName.cs
- RowVisual.cs
- ComponentManagerBroker.cs
- iisPickupDirectory.cs
- AnnotationObservableCollection.cs
- HandleCollector.cs
- NonNullItemCollection.cs
- ExpressionNormalizer.cs
- complextypematerializer.cs
- UserCancellationException.cs
- WmlLabelAdapter.cs
- ProfileProvider.cs
- CancelEventArgs.cs
- InvalidEnumArgumentException.cs
- XsltQilFactory.cs
- Timer.cs
- FaultContractAttribute.cs
- CaseInsensitiveComparer.cs
- ContainerUIElement3D.cs
- ThicknessKeyFrameCollection.cs
- SqlUdtInfo.cs
- UInt64.cs
- ToolBar.cs
- SimplePropertyEntry.cs
- IdnElement.cs
- SymbolMethod.cs
- ExecutionEngineException.cs
- ColorMap.cs
- LicenseManager.cs
- PeerNameRegistration.cs
- ExpressionVisitorHelpers.cs
- UIPropertyMetadata.cs
- RTLAwareMessageBox.cs
- MimeFormReflector.cs
- HyperLinkColumn.cs
- SqlBulkCopyColumnMapping.cs
- RequestCache.cs
- TextOptions.cs
- IMembershipProvider.cs
- RelationshipConstraintValidator.cs
- SvcMapFile.cs
- Translator.cs
- DataGridViewCellToolTipTextNeededEventArgs.cs