Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / WinForms / Managed / System / WinForms / SplitterPanel.cs / 1 / SplitterPanel.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.Windows.Forms { using Microsoft.Win32; using System; using System.ComponentModel; using System.Diagnostics; using System.Security; using System.Security.Permissions; using System.Windows.Forms; using System.Drawing; using System.Drawing.Design; using System.Drawing.Imaging; using System.Runtime.InteropServices; using System.ComponentModel.Design; using System.ComponentModel.Design.Serialization; ////// /// TBD. /// [ ComVisible(true), ClassInterface(ClassInterfaceType.AutoDispatch), Docking(DockingBehavior.Never), Designer("System.Windows.Forms.Design.SplitterPanelDesigner, " + AssemblyRef.SystemDesign), ToolboxItem(false) ] public sealed class SplitterPanel : Panel { SplitContainer owner = null; private bool collapsed = false; ///public SplitterPanel(SplitContainer owner) : base() { this.owner = owner; SetStyle(ControlStyles.ResizeRedraw, true); } internal bool Collapsed { get { return collapsed; } set { collapsed = value; } } /// /// /// Override AutoSize to make it hidden from the user in the designer /// [ EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), Browsable(false) ] public new bool AutoSize { get { return base.AutoSize; } set { base.AutoSize = value; } } ///[Browsable(false), EditorBrowsable(EditorBrowsableState.Never)] new public event EventHandler AutoSizeChanged { add { base.AutoSizeChanged += value; } remove { base.AutoSizeChanged -= value; } } /// /// Allows the control to optionally shrink when AutoSize is true. /// [ EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), Browsable(false), Localizable(false) ] public override AutoSizeMode AutoSizeMode { get { return AutoSizeMode.GrowOnly; } set { } } ////// /// Override Anchor to make it hidden from the user in the designer /// [ EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), Browsable(false) ] public new AnchorStyles Anchor { get { return base.Anchor; } set { base.Anchor = value; } } ////// /// Indicates what type of border the Splitter control has. This value /// comes from the System.Windows.Forms.BorderStyle enumeration. /// [ EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), Browsable(false) ] public new BorderStyle BorderStyle { get { return base.BorderStyle; } set { base.BorderStyle = value; } } ////// /// The dock property. The dock property controls to which edge /// of the container this control is docked to. For example, when docked to /// the top of the container, the control will be displayed flush at the /// top of the container, extending the length of the container. /// [ EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), Browsable(false) ] public new DockStyle Dock { get { return base.Dock; } set { base.Dock = value; } } ////// /// Override DockPadding to make it hidden from the user in the designer /// [ EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), Browsable(false) ] new public DockPaddingEdges DockPadding { get { return base.DockPadding; } } ////// /// The height of this SplitterPanel /// [ SRCategory(SR.CatLayout), Browsable(false), EditorBrowsable(EditorBrowsableState.Always), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), SRDescription(SR.ControlHeightDescr) ] public new int Height { get { if (Collapsed) { return 0; } return base.Height; } set { throw new NotSupportedException(SR.GetString(SR.SplitContainerPanelHeight)); } } internal int HeightInternal { get { return ((Panel)this).Height; } set { ((Panel)this).Height = value; } } ////// /// Override Location to make it hidden from the user in the designer /// [ EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), Browsable(false) ] public new Point Location { get { return base.Location; } set { base.Location = value; } } ////// /// Deriving classes can override this to configure a default size for their control. /// This is more efficient than setting the size in the control's constructor. /// protected override Padding DefaultMargin { get { return new Padding(0, 0, 0, 0); } } ////// /// Override AutoSize to make it hidden from the user in the designer /// [ EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), Browsable(false) ] public new Size MinimumSize { get { return base.MinimumSize; } set { base.MinimumSize = value; } } ////// /// Override AutoSize to make it hidden from the user in the designer /// [ EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), Browsable(false) ] public new Size MaximumSize { get { return base.MaximumSize; } set { base.MaximumSize = value; } } ////// /// Name of this control. The designer will set this to the same /// as the programatic Id "(name)" of the control. The name can be /// used as a key into the ControlCollection. /// [ EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), Browsable(false) ] public new string Name { get { return base.Name; } set { base.Name = value; } } ////// /// The parent of this control. /// internal SplitContainer Owner { get { return owner; } } ////// /// The parent of this control. /// [ EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), Browsable(false) ] public new Control Parent { get { return base.Parent; } set { base.Parent = value; } } ////// /// Override Size to make it hidden from the user in the designer /// [ EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), Browsable(false) ] public new Size Size { get { if (Collapsed) { return Size.Empty; } return base.Size; } set { base.Size = value; } } ////// /// Override TabIndex to make it hidden from the user in the designer /// [ EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), Browsable(false) ] public new int TabIndex { get { return base.TabIndex; } set { base.TabIndex = value; } } ////// /// Override TabStop to make it hidden from the user in the designer /// [ EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), Browsable(false) ] public new bool TabStop { get { return base.TabStop; } set { base.TabStop = value; } } ////// /// Override Visible to make it hidden from the user in the designer /// [ EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), Browsable(false) ] public new bool Visible { get { return base.Visible; } set { base.Visible = value; } } ////// /// The width of this control. /// [ SRCategory(SR.CatLayout), Browsable(false), EditorBrowsable(EditorBrowsableState.Always), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), SRDescription(SR.ControlWidthDescr) ] public new int Width { get { if (Collapsed) { return 0; } return base.Width; } set { throw new NotSupportedException(SR.GetString(SR.SplitContainerPanelWidth)); } } internal int WidthInternal { get { return ((Panel)this).Width; } set { ((Panel)this).Width = value; } } ////// /// Override VisibleChanged to make it hidden from the user in the designer /// [ EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), Browsable(false) ] public new event EventHandler VisibleChanged { add { base.VisibleChanged += value; } remove { base.VisibleChanged -= value; } } ////// /// Override DockChanged to make it hidden from the user in the designer /// [ EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), Browsable(false) ] public new event EventHandler DockChanged { add { base.DockChanged += value; } remove { base.DockChanged -= value; } } ////// /// Override LocationChanged to make it hidden from the user in the designer /// [ EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), Browsable(false) ] public new event EventHandler LocationChanged { add { base.LocationChanged += value; } remove { base.LocationChanged -= value; } } ////// /// Override TabIndexChanged to make it hidden from the user in the designer /// [ EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), Browsable(false) ] public new event EventHandler TabIndexChanged { add { base.TabIndexChanged += value; } remove { base.TabIndexChanged -= value; } } ////// /// Override TabStopChanged to make it hidden from the user in the designer /// [ EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), Browsable(false) ] public new event EventHandler TabStopChanged { add { base.TabStopChanged += value; } remove { base.TabStopChanged -= value; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.Windows.Forms { using Microsoft.Win32; using System; using System.ComponentModel; using System.Diagnostics; using System.Security; using System.Security.Permissions; using System.Windows.Forms; using System.Drawing; using System.Drawing.Design; using System.Drawing.Imaging; using System.Runtime.InteropServices; using System.ComponentModel.Design; using System.ComponentModel.Design.Serialization; ////// /// TBD. /// [ ComVisible(true), ClassInterface(ClassInterfaceType.AutoDispatch), Docking(DockingBehavior.Never), Designer("System.Windows.Forms.Design.SplitterPanelDesigner, " + AssemblyRef.SystemDesign), ToolboxItem(false) ] public sealed class SplitterPanel : Panel { SplitContainer owner = null; private bool collapsed = false; ///public SplitterPanel(SplitContainer owner) : base() { this.owner = owner; SetStyle(ControlStyles.ResizeRedraw, true); } internal bool Collapsed { get { return collapsed; } set { collapsed = value; } } /// /// /// Override AutoSize to make it hidden from the user in the designer /// [ EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), Browsable(false) ] public new bool AutoSize { get { return base.AutoSize; } set { base.AutoSize = value; } } ///[Browsable(false), EditorBrowsable(EditorBrowsableState.Never)] new public event EventHandler AutoSizeChanged { add { base.AutoSizeChanged += value; } remove { base.AutoSizeChanged -= value; } } /// /// Allows the control to optionally shrink when AutoSize is true. /// [ EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), Browsable(false), Localizable(false) ] public override AutoSizeMode AutoSizeMode { get { return AutoSizeMode.GrowOnly; } set { } } ////// /// Override Anchor to make it hidden from the user in the designer /// [ EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), Browsable(false) ] public new AnchorStyles Anchor { get { return base.Anchor; } set { base.Anchor = value; } } ////// /// Indicates what type of border the Splitter control has. This value /// comes from the System.Windows.Forms.BorderStyle enumeration. /// [ EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), Browsable(false) ] public new BorderStyle BorderStyle { get { return base.BorderStyle; } set { base.BorderStyle = value; } } ////// /// The dock property. The dock property controls to which edge /// of the container this control is docked to. For example, when docked to /// the top of the container, the control will be displayed flush at the /// top of the container, extending the length of the container. /// [ EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), Browsable(false) ] public new DockStyle Dock { get { return base.Dock; } set { base.Dock = value; } } ////// /// Override DockPadding to make it hidden from the user in the designer /// [ EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), Browsable(false) ] new public DockPaddingEdges DockPadding { get { return base.DockPadding; } } ////// /// The height of this SplitterPanel /// [ SRCategory(SR.CatLayout), Browsable(false), EditorBrowsable(EditorBrowsableState.Always), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), SRDescription(SR.ControlHeightDescr) ] public new int Height { get { if (Collapsed) { return 0; } return base.Height; } set { throw new NotSupportedException(SR.GetString(SR.SplitContainerPanelHeight)); } } internal int HeightInternal { get { return ((Panel)this).Height; } set { ((Panel)this).Height = value; } } ////// /// Override Location to make it hidden from the user in the designer /// [ EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), Browsable(false) ] public new Point Location { get { return base.Location; } set { base.Location = value; } } ////// /// Deriving classes can override this to configure a default size for their control. /// This is more efficient than setting the size in the control's constructor. /// protected override Padding DefaultMargin { get { return new Padding(0, 0, 0, 0); } } ////// /// Override AutoSize to make it hidden from the user in the designer /// [ EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), Browsable(false) ] public new Size MinimumSize { get { return base.MinimumSize; } set { base.MinimumSize = value; } } ////// /// Override AutoSize to make it hidden from the user in the designer /// [ EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), Browsable(false) ] public new Size MaximumSize { get { return base.MaximumSize; } set { base.MaximumSize = value; } } ////// /// Name of this control. The designer will set this to the same /// as the programatic Id "(name)" of the control. The name can be /// used as a key into the ControlCollection. /// [ EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), Browsable(false) ] public new string Name { get { return base.Name; } set { base.Name = value; } } ////// /// The parent of this control. /// internal SplitContainer Owner { get { return owner; } } ////// /// The parent of this control. /// [ EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), Browsable(false) ] public new Control Parent { get { return base.Parent; } set { base.Parent = value; } } ////// /// Override Size to make it hidden from the user in the designer /// [ EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), Browsable(false) ] public new Size Size { get { if (Collapsed) { return Size.Empty; } return base.Size; } set { base.Size = value; } } ////// /// Override TabIndex to make it hidden from the user in the designer /// [ EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), Browsable(false) ] public new int TabIndex { get { return base.TabIndex; } set { base.TabIndex = value; } } ////// /// Override TabStop to make it hidden from the user in the designer /// [ EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), Browsable(false) ] public new bool TabStop { get { return base.TabStop; } set { base.TabStop = value; } } ////// /// Override Visible to make it hidden from the user in the designer /// [ EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), Browsable(false) ] public new bool Visible { get { return base.Visible; } set { base.Visible = value; } } ////// /// The width of this control. /// [ SRCategory(SR.CatLayout), Browsable(false), EditorBrowsable(EditorBrowsableState.Always), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), SRDescription(SR.ControlWidthDescr) ] public new int Width { get { if (Collapsed) { return 0; } return base.Width; } set { throw new NotSupportedException(SR.GetString(SR.SplitContainerPanelWidth)); } } internal int WidthInternal { get { return ((Panel)this).Width; } set { ((Panel)this).Width = value; } } ////// /// Override VisibleChanged to make it hidden from the user in the designer /// [ EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), Browsable(false) ] public new event EventHandler VisibleChanged { add { base.VisibleChanged += value; } remove { base.VisibleChanged -= value; } } ////// /// Override DockChanged to make it hidden from the user in the designer /// [ EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), Browsable(false) ] public new event EventHandler DockChanged { add { base.DockChanged += value; } remove { base.DockChanged -= value; } } ////// /// Override LocationChanged to make it hidden from the user in the designer /// [ EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), Browsable(false) ] public new event EventHandler LocationChanged { add { base.LocationChanged += value; } remove { base.LocationChanged -= value; } } ////// /// Override TabIndexChanged to make it hidden from the user in the designer /// [ EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), Browsable(false) ] public new event EventHandler TabIndexChanged { add { base.TabIndexChanged += value; } remove { base.TabIndexChanged -= value; } } ////// /// Override TabStopChanged to make it hidden from the user in the designer /// [ EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), Browsable(false) ] public new event EventHandler TabStopChanged { add { base.TabStopChanged += value; } remove { base.TabStopChanged -= value; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
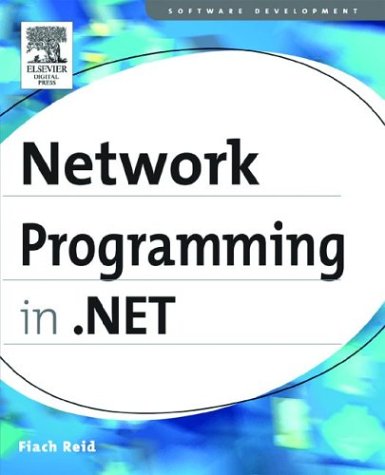
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- BitmapImage.cs
- CodeLinePragma.cs
- Context.cs
- InplaceBitmapMetadataWriter.cs
- Highlights.cs
- TagNameToTypeMapper.cs
- DataDesignUtil.cs
- DataView.cs
- AssemblyBuilderData.cs
- CssStyleCollection.cs
- ExtentCqlBlock.cs
- BindableTemplateBuilder.cs
- Utils.cs
- RestClientProxyHandler.cs
- TagNameToTypeMapper.cs
- DataGridColumnCollection.cs
- TreeViewDataItemAutomationPeer.cs
- TraceData.cs
- smtppermission.cs
- GeometryConverter.cs
- Tuple.cs
- HighlightVisual.cs
- RenderingEventArgs.cs
- EditorZone.cs
- AndCondition.cs
- DecimalConverter.cs
- Activity.cs
- BuildResultCache.cs
- XmlElement.cs
- ConvertersCollection.cs
- HttpListenerElement.cs
- XmlResolver.cs
- ApplicationActivator.cs
- PartitionedStream.cs
- QuotedPrintableStream.cs
- OleDbParameter.cs
- RunWorkerCompletedEventArgs.cs
- DbException.cs
- Image.cs
- ServiceDescriptionSerializer.cs
- LoginUtil.cs
- WpfXamlMember.cs
- GeometryHitTestResult.cs
- NumberAction.cs
- RegisteredArrayDeclaration.cs
- OptimizerPatterns.cs
- ProxyElement.cs
- TextServicesContext.cs
- SR.cs
- AttributeEmitter.cs
- WindowsFormsHostPropertyMap.cs
- WindowsFormsLinkLabel.cs
- OleDbRowUpdatingEvent.cs
- AuthenticationService.cs
- CqlBlock.cs
- ZipFileInfoCollection.cs
- XMLSyntaxException.cs
- TlsnegoTokenAuthenticator.cs
- LayoutUtils.cs
- FlowDocument.cs
- Compiler.cs
- ConnectionPoint.cs
- AuthenticationServiceManager.cs
- DataGridTextBoxColumn.cs
- CardSpaceSelector.cs
- GlyphInfoList.cs
- HMACSHA256.cs
- UpdateEventArgs.cs
- XmlDsigSep2000.cs
- QueryAccessibilityHelpEvent.cs
- RegistrationServices.cs
- DataBindingsDialog.cs
- ListView.cs
- Point4DConverter.cs
- QuaternionKeyFrameCollection.cs
- NullReferenceException.cs
- ComPlusServiceLoader.cs
- XPathAncestorIterator.cs
- ConfigXmlWhitespace.cs
- DecimalConstantAttribute.cs
- _Win32.cs
- Environment.cs
- BuildResult.cs
- Label.cs
- DbException.cs
- CookieProtection.cs
- contentDescriptor.cs
- UrlMappingsSection.cs
- Collection.cs
- Int32Converter.cs
- TableStyle.cs
- WpfPayload.cs
- ButtonField.cs
- CacheForPrimitiveTypes.cs
- TypeBuilderInstantiation.cs
- SectionVisual.cs
- XPathExpr.cs
- TimeSpanSecondsConverter.cs
- OptimisticConcurrencyException.cs
- RichTextBoxAutomationPeer.cs