Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Core / System / Windows / Media3D / Point4D.cs / 1 / Point4D.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: 4D point implementation. // // See spec at [....]/medialayer/Specifications/Avalon3D%20API%20Spec.mht // // History: // 06/04/2003 : [....] - Created // //--------------------------------------------------------------------------- using System.Windows; using System.Windows.Media.Media3D; using System; namespace System.Windows.Media.Media3D { ////// Point4D - 4D point representation. /// Defaults to (0,0,0,0). /// public partial struct Point4D { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors ////// Constructor that sets point's initial values. /// /// Value of the X coordinate of the new point. /// Value of the Y coordinate of the new point. /// Value of the Z coordinate of the new point. /// Value of the W coordinate of the new point. public Point4D(double x, double y, double z, double w) { _x = x; _y = y; _z = z; _w = w; } #endregion Constructors //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- #region Public Methods ////// Offset - update point position by adding deltaX to X, deltaY to Y, deltaZ to Z, and deltaW to W. /// /// Offset in the X direction. /// Offset in the Y direction. /// Offset in the Z direction. /// Offset in the W direction. public void Offset(double deltaX, double deltaY, double deltaZ, double deltaW) { _x += deltaX; _y += deltaY; _z += deltaZ; _w += deltaW; } ////// Addition. /// /// First point being added. /// Second point being added. ///Result of addition. public static Point4D operator +(Point4D point1, Point4D point2) { return new Point4D(point1._x + point2._x, point1._y + point2._y, point1._z + point2._z, point1._w + point2._w); } ////// Addition. /// /// First point being added. /// Second point being added. ///Result of addition. public static Point4D Add(Point4D point1, Point4D point2) { return new Point4D(point1._x + point2._x, point1._y + point2._y, point1._z + point2._z, point1._w + point2._w); } ////// Subtraction. /// /// Point from which we are subtracting the second point. /// Point being subtracted. ///Vector between the two points. public static Point4D operator -(Point4D point1, Point4D point2) { return new Point4D(point1._x - point2._x, point1._y - point2._y, point1._z - point2._z, point1._w - point2._w); } ////// Subtraction. /// /// Point from which we are subtracting the second point. /// Point being subtracted. ///Vector between the two points. public static Point4D Subtract(Point4D point1, Point4D point2) { return new Point4D(point1._x - point2._x, point1._y - point2._y, point1._z - point2._z, point1._w - point2._w); } ////// Point4D * Matrix3D multiplication. /// /// Point being transformed. /// Transformation matrix applied to the point. ///Result of the transformation matrix applied to the point. public static Point4D operator *(Point4D point, Matrix3D matrix) { return matrix.Transform(point); } ////// Point4D * Matrix3D multiplication. /// /// Point being transformed. /// Transformation matrix applied to the point. ///Result of the transformation matrix applied to the point. public static Point4D Multiply(Point4D point, Matrix3D matrix) { return matrix.Transform(point); } #endregion Public Methods } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
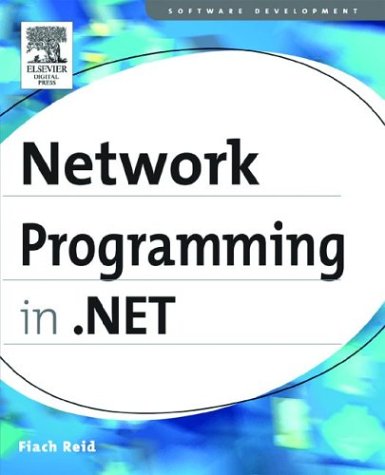
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- HttpHandlersSection.cs
- QuaternionValueSerializer.cs
- SplayTreeNode.cs
- DbConvert.cs
- CreateUserWizardStep.cs
- XmlNamespaceMapping.cs
- ThreadExceptionEvent.cs
- PersianCalendar.cs
- WorkerRequest.cs
- CheckBoxBaseAdapter.cs
- DataGridTemplateColumn.cs
- DataGridViewComboBoxCell.cs
- LongSumAggregationOperator.cs
- Font.cs
- EnumBuilder.cs
- SystemException.cs
- FieldBuilder.cs
- TemplatedWizardStep.cs
- BuildDependencySet.cs
- DEREncoding.cs
- LinearGradientBrush.cs
- StringBuilder.cs
- KernelTypeValidation.cs
- BuilderPropertyEntry.cs
- SliderAutomationPeer.cs
- RectangleHotSpot.cs
- WebAdminConfigurationHelper.cs
- Highlights.cs
- XmlHierarchyData.cs
- ResourcesBuildProvider.cs
- AssemblyResolver.cs
- TextInfo.cs
- DataList.cs
- MasterPage.cs
- TextChangedEventArgs.cs
- DataGridViewTopRowAccessibleObject.cs
- FullTextState.cs
- InheritedPropertyDescriptor.cs
- dataSvcMapFileLoader.cs
- Ticks.cs
- MulticastOption.cs
- ReadContentAsBinaryHelper.cs
- XmlRootAttribute.cs
- ComponentManagerBroker.cs
- RsaSecurityTokenAuthenticator.cs
- PixelFormatConverter.cs
- ProtocolsConfigurationEntry.cs
- tooltip.cs
- DnsEndpointIdentity.cs
- SmiTypedGetterSetter.cs
- WebPartZone.cs
- SectionUpdates.cs
- ToolStripDropDownItemDesigner.cs
- PerCallInstanceContextProvider.cs
- StringPropertyBuilder.cs
- DbModificationClause.cs
- XmlCollation.cs
- BamlBinaryReader.cs
- AnnotationAuthorChangedEventArgs.cs
- WsatTransactionHeader.cs
- DeclarativeExpressionConditionDeclaration.cs
- IsolatedStoragePermission.cs
- CustomErrorCollection.cs
- ApplicationTrust.cs
- ApplicationTrust.cs
- XmlSchemaCollection.cs
- XPathNodeInfoAtom.cs
- HorizontalAlignConverter.cs
- ErrorInfoXmlDocument.cs
- EdmValidator.cs
- PathFigureCollectionConverter.cs
- ValuePatternIdentifiers.cs
- ClickablePoint.cs
- DeviceContexts.cs
- Documentation.cs
- GridSplitter.cs
- ServerIdentity.cs
- OracleDataAdapter.cs
- UrlAuthFailedErrorFormatter.cs
- EnumDataContract.cs
- RemotingSurrogateSelector.cs
- CodeAttributeArgumentCollection.cs
- ResourceManagerWrapper.cs
- DictionaryGlobals.cs
- Timeline.cs
- UserControl.cs
- DataExpression.cs
- DataGridViewSelectedCellCollection.cs
- _ScatterGatherBuffers.cs
- IISUnsafeMethods.cs
- ScriptReference.cs
- ThreadSafeList.cs
- HuffmanTree.cs
- IdnElement.cs
- HtmlInputControl.cs
- HtmlMeta.cs
- AdRotator.cs
- CheckBoxList.cs
- Margins.cs
- OverflowException.cs