Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Core / CSharp / System / Windows / Media3D / Generated / Rect3DConverter.cs / 1 / Rect3DConverter.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // Please see http://wiki/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- using MS.Internal; using MS.Internal.Collections; using MS.Internal.PresentationCore; using MS.Utility; using System; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Runtime.InteropServices; using System.Text; using System.Windows.Markup; using System.Windows.Media.Media3D.Converters; using System.Windows.Media; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using System.Security; using System.Security.Permissions; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; using System.Windows.Media.Imaging; #pragma warning disable 1634, 1691 // suppressing PreSharp warnings namespace System.Windows.Media.Media3D { ////// Rect3DConverter - Converter class for converting instances of other types to and from Rect3D instances /// public sealed class Rect3DConverter : TypeConverter { ////// Returns true if this type converter can convert from a given type. /// ////// bool - True if this converter can convert from the provided type, false if not. /// /// The ITypeDescriptorContext for this call. /// The Type being queried for support. public override bool CanConvertFrom(ITypeDescriptorContext context, Type sourceType) { if (sourceType == typeof(string)) { return true; } return base.CanConvertFrom(context, sourceType); } ////// Returns true if this type converter can convert to the given type. /// ////// bool - True if this converter can convert to the provided type, false if not. /// /// The ITypeDescriptorContext for this call. /// The Type being queried for support. public override bool CanConvertTo(ITypeDescriptorContext context, Type destinationType) { if (destinationType == typeof(string)) { return true; } return base.CanConvertTo(context, destinationType); } ////// Attempts to convert to a Rect3D from the given object. /// ////// The Rect3D which was constructed. /// ////// A NotSupportedException is thrown if the example object is null or is not a valid type /// which can be converted to a Rect3D. /// /// The ITypeDescriptorContext for this call. /// The requested CultureInfo. Note that conversion uses "en-US" rather than this parameter. /// The object to convert to an instance of Rect3D. public override object ConvertFrom(ITypeDescriptorContext context, CultureInfo culture, object value) { if (value == null) { throw GetConvertFromException(value); } String source = value as string; if (source != null) { return Rect3D.Parse(source); } return base.ConvertFrom(context, culture, value); } ////// ConvertTo - Attempt to convert an instance of Rect3D to the given type /// ////// The object which was constructoed. /// ////// A NotSupportedException is thrown if "value" is null or not an instance of Rect3D, /// or if the destinationType isn't one of the valid destination types. /// /// The ITypeDescriptorContext for this call. /// The CultureInfo which is respected when converting. /// The object to convert to an instance of "destinationType". /// The type to which this will convert the Rect3D instance. public override object ConvertTo(ITypeDescriptorContext context, CultureInfo culture, object value, Type destinationType) { if (destinationType != null && value is Rect3D) { Rect3D instance = (Rect3D)value; if (destinationType == typeof(string)) { // Delegate to the formatting/culture-aware ConvertToString method. #pragma warning suppress 6506 // instance is obviously not null return instance.ConvertToString(null, culture); } } // Pass unhandled cases to base class (which will throw exceptions for null value or destinationType.) return base.ConvertTo(context, culture, value, destinationType); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // Please see http://wiki/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- using MS.Internal; using MS.Internal.Collections; using MS.Internal.PresentationCore; using MS.Utility; using System; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Runtime.InteropServices; using System.Text; using System.Windows.Markup; using System.Windows.Media.Media3D.Converters; using System.Windows.Media; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using System.Security; using System.Security.Permissions; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; using System.Windows.Media.Imaging; #pragma warning disable 1634, 1691 // suppressing PreSharp warnings namespace System.Windows.Media.Media3D { ////// Rect3DConverter - Converter class for converting instances of other types to and from Rect3D instances /// public sealed class Rect3DConverter : TypeConverter { ////// Returns true if this type converter can convert from a given type. /// ////// bool - True if this converter can convert from the provided type, false if not. /// /// The ITypeDescriptorContext for this call. /// The Type being queried for support. public override bool CanConvertFrom(ITypeDescriptorContext context, Type sourceType) { if (sourceType == typeof(string)) { return true; } return base.CanConvertFrom(context, sourceType); } ////// Returns true if this type converter can convert to the given type. /// ////// bool - True if this converter can convert to the provided type, false if not. /// /// The ITypeDescriptorContext for this call. /// The Type being queried for support. public override bool CanConvertTo(ITypeDescriptorContext context, Type destinationType) { if (destinationType == typeof(string)) { return true; } return base.CanConvertTo(context, destinationType); } ////// Attempts to convert to a Rect3D from the given object. /// ////// The Rect3D which was constructed. /// ////// A NotSupportedException is thrown if the example object is null or is not a valid type /// which can be converted to a Rect3D. /// /// The ITypeDescriptorContext for this call. /// The requested CultureInfo. Note that conversion uses "en-US" rather than this parameter. /// The object to convert to an instance of Rect3D. public override object ConvertFrom(ITypeDescriptorContext context, CultureInfo culture, object value) { if (value == null) { throw GetConvertFromException(value); } String source = value as string; if (source != null) { return Rect3D.Parse(source); } return base.ConvertFrom(context, culture, value); } ////// ConvertTo - Attempt to convert an instance of Rect3D to the given type /// ////// The object which was constructoed. /// ////// A NotSupportedException is thrown if "value" is null or not an instance of Rect3D, /// or if the destinationType isn't one of the valid destination types. /// /// The ITypeDescriptorContext for this call. /// The CultureInfo which is respected when converting. /// The object to convert to an instance of "destinationType". /// The type to which this will convert the Rect3D instance. public override object ConvertTo(ITypeDescriptorContext context, CultureInfo culture, object value, Type destinationType) { if (destinationType != null && value is Rect3D) { Rect3D instance = (Rect3D)value; if (destinationType == typeof(string)) { // Delegate to the formatting/culture-aware ConvertToString method. #pragma warning suppress 6506 // instance is obviously not null return instance.ConvertToString(null, culture); } } // Pass unhandled cases to base class (which will throw exceptions for null value or destinationType.) return base.ConvertTo(context, culture, value, destinationType); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
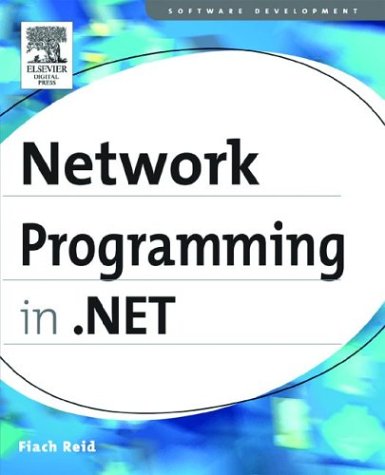
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Material.cs
- TraceContextRecord.cs
- ISAPIApplicationHost.cs
- isolationinterop.cs
- Rotation3DAnimationUsingKeyFrames.cs
- AuthenticationModuleElement.cs
- InfoCardRSAOAEPKeyExchangeDeformatter.cs
- HostVisual.cs
- TextDecoration.cs
- SecurityKeyUsage.cs
- DbCommandDefinition.cs
- PtsContext.cs
- RemoteDebugger.cs
- OperandQuery.cs
- BuildProvider.cs
- PersistenceContextEnlistment.cs
- SapiAttributeParser.cs
- recordstate.cs
- SessionPageStateSection.cs
- TranslateTransform3D.cs
- DrawingContextDrawingContextWalker.cs
- RawStylusInputReport.cs
- XPathDocumentNavigator.cs
- HiddenFieldDesigner.cs
- TypedReference.cs
- UserPreferenceChangedEventArgs.cs
- DateTimeFormatInfo.cs
- SettingsSection.cs
- ChannelManager.cs
- MediaContextNotificationWindow.cs
- BulletedListEventArgs.cs
- DataFormat.cs
- ValueTypeFixupInfo.cs
- ProtocolsConfigurationHandler.cs
- SyndicationPerson.cs
- ServiceOperationUIEditor.cs
- LayoutTable.cs
- DBConnection.cs
- RulePatternOps.cs
- CompositeActivityCodeGenerator.cs
- DesignerActionMethodItem.cs
- EUCJPEncoding.cs
- InvalidateEvent.cs
- SrgsElementFactory.cs
- SoapCommonClasses.cs
- DelegateTypeInfo.cs
- CatalogPartCollection.cs
- OleDbStruct.cs
- RequestCacheValidator.cs
- ModelFactory.cs
- ComponentEditorPage.cs
- Main.cs
- SelectionManager.cs
- ListSortDescriptionCollection.cs
- Grid.cs
- InteropAutomationProvider.cs
- APCustomTypeDescriptor.cs
- ColorConverter.cs
- MonitorWrapper.cs
- CollectionConverter.cs
- TraceFilter.cs
- EnumMember.cs
- QilTernary.cs
- RuntimeEnvironment.cs
- SymmetricAlgorithm.cs
- ScrollBar.cs
- StateBag.cs
- XmlNodeList.cs
- SoapElementAttribute.cs
- InputMethod.cs
- ModifierKeysValueSerializer.cs
- Canvas.cs
- RequiredFieldValidator.cs
- PropertyGrid.cs
- basenumberconverter.cs
- TextParagraphCache.cs
- XPathNodeInfoAtom.cs
- DataGrid.cs
- DirectoryInfo.cs
- TableProviderWrapper.cs
- MetaChildrenColumn.cs
- EntityDataSourceWrapperCollection.cs
- RuleRef.cs
- OleDbError.cs
- BoolExpression.cs
- HashCryptoHandle.cs
- SubtreeProcessor.cs
- HttpValueCollection.cs
- PlacementWorkspace.cs
- Tablet.cs
- NativeMethods.cs
- GlyphRunDrawing.cs
- CodeMemberMethod.cs
- TrackingProfileCache.cs
- IndicFontClient.cs
- ColorKeyFrameCollection.cs
- FtpCachePolicyElement.cs
- Context.cs
- GuidTagList.cs
- input.cs