Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Core / CSharp / MS / Internal / Ink / InkSerializedFormat / GuidTagList.cs / 1 / GuidTagList.cs
using System; using System.Collections; using System.IO; using System.Windows.Ink; using MS.Internal.IO.Packaging; namespace MS.Internal.Ink.InkSerializedFormat { ////// Summary description for GuidTagList. /// internal class GuidList { private System.Collections.Generic.List_CustomGuids = new System.Collections.Generic.List (); public GuidList() { } /// /// Adds a guid to the list of Custom Guids if it is not a known guid and is already not /// in the list of Custom Guids /// /// ///public bool Add(Guid guid) { // If the guid is not found in the known guids list nor in the custom guid list, // add that to the custom guid list if (0 == FindTag(guid, true)) { _CustomGuids.Add(guid); return true; } else return false; } /// /// Finds the tag for a Guid based on the list of Known Guids /// /// ///public static KnownTagCache.KnownTagIndex FindKnownTag(Guid guid) { // Find out if the guid is in the known guid table for (byte iIndex = 0; iIndex < KnownIdCache.OriginalISFIdTable.Length; ++iIndex) if (guid == KnownIdCache.OriginalISFIdTable[iIndex]) return KnownIdCache.KnownGuidBaseIndex + iIndex; // Couldnt find in the known list return 0; } /// /// Finds the tag for a guid based on the list of Custom Guids /// /// ///KnownTagCache.KnownTagIndex FindCustomTag(Guid guid) { int i; for (i = 0; i < _CustomGuids.Count; i++) { if (guid.Equals(_CustomGuids[i])) return (KnownTagCache.KnownTagIndex)(KnownIdCache.CustomGuidBaseIndex + i); } return KnownTagCache.KnownTagIndex.Unknown; } /// /// Finds a tag corresponding to a guids /// /// /// ///public KnownTagCache.KnownTagIndex FindTag(Guid guid, bool bFindInKnownListFirst) { KnownTagCache.KnownTagIndex tag = KnownTagCache.KnownTagIndex.Unknown; if (bFindInKnownListFirst) { tag = FindKnownTag(guid); if (KnownTagCache.KnownTagIndex.Unknown == tag) tag = FindCustomTag(guid); } else { tag = FindCustomTag(guid); if (KnownTagCache.KnownTagIndex.Unknown == tag) tag = FindKnownTag(guid); } return tag; } /// /// Finds a known guid based on a Tag /// /// ///static Guid FindKnownGuid(KnownTagCache.KnownTagIndex tag) { if (tag < KnownIdCache.KnownGuidBaseIndex) { throw new ArgumentException(StrokeCollectionSerializer.ISFDebugMessage("Tag is outside of the known guid tag range")); } // Get the index in the OriginalISFIdTable array first uint nIndex = (uint)(tag - KnownIdCache.KnownGuidBaseIndex); // If invalid, return Guid.Empty if (KnownIdCache.OriginalISFIdTable.Length <= nIndex) return Guid.Empty; // Otherwise, return the guid return KnownIdCache.OriginalISFIdTable[nIndex]; } /// /// Finds a Custom Guid based on a Tag /// /// ///Guid FindCustomGuid(KnownTagCache.KnownTagIndex tag) { if ((int)tag < (int)KnownIdCache.CustomGuidBaseIndex) { throw new ArgumentException(StrokeCollectionSerializer.ISFDebugMessage("Tag is outside of the known guid tag range")); } // Get the index in the OriginalISFIdTable array first int nIndex = (int)(tag - KnownIdCache.CustomGuidBaseIndex); // If invalid, return Guid.Empty if ((0 > nIndex) || (_CustomGuids.Count <= nIndex)) return Guid.Empty; // Otherwise, return the guid return (Guid)_CustomGuids[(int)nIndex]; } /// /// Finds a guid based on Tag /// /// ///public Guid FindGuid(KnownTagCache.KnownTagIndex tag) { if (tag < (KnownTagCache.KnownTagIndex)KnownIdCache.CustomGuidBaseIndex) { Guid guid = FindKnownGuid(tag); if (Guid.Empty != guid) return guid; return FindCustomGuid(tag); } else { Guid guid = FindCustomGuid(tag); if (Guid.Empty != guid) return guid; return FindKnownGuid(tag); } } /// /// Returns the expected size of data if it is a known guid /// /// ///public static uint GetDataSizeIfKnownGuid(Guid guid) { for (uint i = 0; i < KnownIdCache.OriginalISFIdTable.Length; ++i) { if (guid == KnownIdCache.OriginalISFIdTable[i]) { return KnownIdCache.OriginalISFIdPersistenceSize[i]; } } return 0; } /// /// Serializes the GuidList in the memory stream and returns the size /// /// If null, calculates the size only ///public uint Save(Stream stream) { // calculate the number of custom guids to persist // custom guids are those which are not reserved in ISF via 'tags' uint ul = (uint)(_CustomGuids.Count * Native.SizeOfGuid); // if there are no custom guids, then the guid list can be persisted // without any cost ('tags' are freely storeable) if (ul == 0) { return 0; } // if only the size was requested, return it if (null == stream) { return (uint)(ul + SerializationHelper.VarSize(ul) + SerializationHelper.VarSize((uint)KnownTagCache.KnownTagIndex.GuidTable)); } // encode the guid table tag in the output stream uint cbWrote = SerializationHelper.Encode(stream, (uint)KnownTagCache.KnownTagIndex.GuidTable); // encode the size of the guid table cbWrote += SerializationHelper.Encode(stream, ul); // encode each guid in the table for (int i = 0; i < _CustomGuids.Count; i++) { Guid guid = (Guid)_CustomGuids[i]; stream.Write(guid.ToByteArray(), 0, (int)Native.SizeOfGuid); } cbWrote += ul; return cbWrote; } /// /// Deserializes the GuidList from the memory stream /// /// /// ///public uint Load(Stream strm, uint size) { uint cbsize = 0; _CustomGuids.Clear(); uint count = size / Native.SizeOfGuid; byte[] guids = new byte[Native.SizeOfGuid]; for (uint i = 0; i < count; i++) { // NTRAID:WINDOWSOS#1622775-2006/04/26-WAYNEZEN, // Stream.Read could read less number of bytes than the request. We call ReliableRead that // reads the bytes in a loop until all requested bytes are received or reach the end of the stream. uint bytesRead = StrokeCollectionSerializer.ReliableRead(strm, guids, Native.SizeOfGuid); cbsize += bytesRead; if ( bytesRead == Native.SizeOfGuid ) { _CustomGuids.Add(new Guid(guids)); } else { // If Stream.Read cannot return the expected number of bytes, we should break here. // The caller - StrokeCollectionSerializer.DecodeRawISF will check our return value. // An exception might be thrown if reading is failed. break; } } return cbsize; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using System; using System.Collections; using System.IO; using System.Windows.Ink; using MS.Internal.IO.Packaging; namespace MS.Internal.Ink.InkSerializedFormat { /// /// Summary description for GuidTagList. /// internal class GuidList { private System.Collections.Generic.List_CustomGuids = new System.Collections.Generic.List (); public GuidList() { } /// /// Adds a guid to the list of Custom Guids if it is not a known guid and is already not /// in the list of Custom Guids /// /// ///public bool Add(Guid guid) { // If the guid is not found in the known guids list nor in the custom guid list, // add that to the custom guid list if (0 == FindTag(guid, true)) { _CustomGuids.Add(guid); return true; } else return false; } /// /// Finds the tag for a Guid based on the list of Known Guids /// /// ///public static KnownTagCache.KnownTagIndex FindKnownTag(Guid guid) { // Find out if the guid is in the known guid table for (byte iIndex = 0; iIndex < KnownIdCache.OriginalISFIdTable.Length; ++iIndex) if (guid == KnownIdCache.OriginalISFIdTable[iIndex]) return KnownIdCache.KnownGuidBaseIndex + iIndex; // Couldnt find in the known list return 0; } /// /// Finds the tag for a guid based on the list of Custom Guids /// /// ///KnownTagCache.KnownTagIndex FindCustomTag(Guid guid) { int i; for (i = 0; i < _CustomGuids.Count; i++) { if (guid.Equals(_CustomGuids[i])) return (KnownTagCache.KnownTagIndex)(KnownIdCache.CustomGuidBaseIndex + i); } return KnownTagCache.KnownTagIndex.Unknown; } /// /// Finds a tag corresponding to a guids /// /// /// ///public KnownTagCache.KnownTagIndex FindTag(Guid guid, bool bFindInKnownListFirst) { KnownTagCache.KnownTagIndex tag = KnownTagCache.KnownTagIndex.Unknown; if (bFindInKnownListFirst) { tag = FindKnownTag(guid); if (KnownTagCache.KnownTagIndex.Unknown == tag) tag = FindCustomTag(guid); } else { tag = FindCustomTag(guid); if (KnownTagCache.KnownTagIndex.Unknown == tag) tag = FindKnownTag(guid); } return tag; } /// /// Finds a known guid based on a Tag /// /// ///static Guid FindKnownGuid(KnownTagCache.KnownTagIndex tag) { if (tag < KnownIdCache.KnownGuidBaseIndex) { throw new ArgumentException(StrokeCollectionSerializer.ISFDebugMessage("Tag is outside of the known guid tag range")); } // Get the index in the OriginalISFIdTable array first uint nIndex = (uint)(tag - KnownIdCache.KnownGuidBaseIndex); // If invalid, return Guid.Empty if (KnownIdCache.OriginalISFIdTable.Length <= nIndex) return Guid.Empty; // Otherwise, return the guid return KnownIdCache.OriginalISFIdTable[nIndex]; } /// /// Finds a Custom Guid based on a Tag /// /// ///Guid FindCustomGuid(KnownTagCache.KnownTagIndex tag) { if ((int)tag < (int)KnownIdCache.CustomGuidBaseIndex) { throw new ArgumentException(StrokeCollectionSerializer.ISFDebugMessage("Tag is outside of the known guid tag range")); } // Get the index in the OriginalISFIdTable array first int nIndex = (int)(tag - KnownIdCache.CustomGuidBaseIndex); // If invalid, return Guid.Empty if ((0 > nIndex) || (_CustomGuids.Count <= nIndex)) return Guid.Empty; // Otherwise, return the guid return (Guid)_CustomGuids[(int)nIndex]; } /// /// Finds a guid based on Tag /// /// ///public Guid FindGuid(KnownTagCache.KnownTagIndex tag) { if (tag < (KnownTagCache.KnownTagIndex)KnownIdCache.CustomGuidBaseIndex) { Guid guid = FindKnownGuid(tag); if (Guid.Empty != guid) return guid; return FindCustomGuid(tag); } else { Guid guid = FindCustomGuid(tag); if (Guid.Empty != guid) return guid; return FindKnownGuid(tag); } } /// /// Returns the expected size of data if it is a known guid /// /// ///public static uint GetDataSizeIfKnownGuid(Guid guid) { for (uint i = 0; i < KnownIdCache.OriginalISFIdTable.Length; ++i) { if (guid == KnownIdCache.OriginalISFIdTable[i]) { return KnownIdCache.OriginalISFIdPersistenceSize[i]; } } return 0; } /// /// Serializes the GuidList in the memory stream and returns the size /// /// If null, calculates the size only ///public uint Save(Stream stream) { // calculate the number of custom guids to persist // custom guids are those which are not reserved in ISF via 'tags' uint ul = (uint)(_CustomGuids.Count * Native.SizeOfGuid); // if there are no custom guids, then the guid list can be persisted // without any cost ('tags' are freely storeable) if (ul == 0) { return 0; } // if only the size was requested, return it if (null == stream) { return (uint)(ul + SerializationHelper.VarSize(ul) + SerializationHelper.VarSize((uint)KnownTagCache.KnownTagIndex.GuidTable)); } // encode the guid table tag in the output stream uint cbWrote = SerializationHelper.Encode(stream, (uint)KnownTagCache.KnownTagIndex.GuidTable); // encode the size of the guid table cbWrote += SerializationHelper.Encode(stream, ul); // encode each guid in the table for (int i = 0; i < _CustomGuids.Count; i++) { Guid guid = (Guid)_CustomGuids[i]; stream.Write(guid.ToByteArray(), 0, (int)Native.SizeOfGuid); } cbWrote += ul; return cbWrote; } /// /// Deserializes the GuidList from the memory stream /// /// /// ///public uint Load(Stream strm, uint size) { uint cbsize = 0; _CustomGuids.Clear(); uint count = size / Native.SizeOfGuid; byte[] guids = new byte[Native.SizeOfGuid]; for (uint i = 0; i < count; i++) { // NTRAID:WINDOWSOS#1622775-2006/04/26-WAYNEZEN, // Stream.Read could read less number of bytes than the request. We call ReliableRead that // reads the bytes in a loop until all requested bytes are received or reach the end of the stream. uint bytesRead = StrokeCollectionSerializer.ReliableRead(strm, guids, Native.SizeOfGuid); cbsize += bytesRead; if ( bytesRead == Native.SizeOfGuid ) { _CustomGuids.Add(new Guid(guids)); } else { // If Stream.Read cannot return the expected number of bytes, we should break here. // The caller - StrokeCollectionSerializer.DecodeRawISF will check our return value. // An exception might be thrown if reading is failed. break; } } return cbsize; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
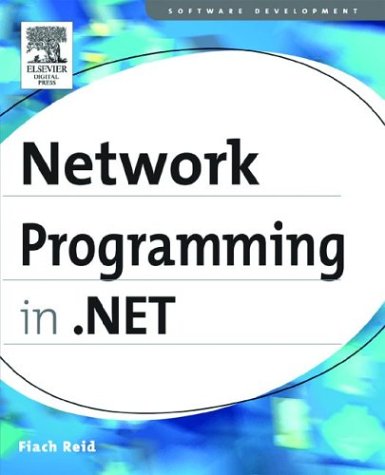
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- VisualBasicHelper.cs
- Rotation3DKeyFrameCollection.cs
- VarInfo.cs
- SupportingTokenAuthenticatorSpecification.cs
- Error.cs
- ImageAnimator.cs
- OpacityConverter.cs
- GeometryModel3D.cs
- ToolboxComponentsCreatingEventArgs.cs
- GPPOINT.cs
- CLSCompliantAttribute.cs
- ProjectionPlanCompiler.cs
- SafeFileMappingHandle.cs
- XmlMemberMapping.cs
- ExpressionBinding.cs
- WindowVisualStateTracker.cs
- SplayTreeNode.cs
- Wizard.cs
- SchemaElement.cs
- InfoCardRSAOAEPKeyExchangeFormatter.cs
- coordinatorscratchpad.cs
- ServiceOperationParameter.cs
- DBParameter.cs
- Pen.cs
- SqlGenericUtil.cs
- _TransmitFileOverlappedAsyncResult.cs
- MarkupProperty.cs
- XmlImplementation.cs
- BinaryVersion.cs
- Directory.cs
- SwitchDesigner.xaml.cs
- TextPatternIdentifiers.cs
- Typography.cs
- recordstatescratchpad.cs
- COM2ComponentEditor.cs
- IListConverters.cs
- NodeCounter.cs
- TrackingDataItem.cs
- ErrorFormatterPage.cs
- Preprocessor.cs
- Brush.cs
- SliderAutomationPeer.cs
- StorageRoot.cs
- Baml2006KnownTypes.cs
- CngUIPolicy.cs
- DataPagerField.cs
- SingleConverter.cs
- InkCanvasSelectionAdorner.cs
- RectConverter.cs
- BinaryReader.cs
- PickBranchDesigner.xaml.cs
- FastEncoder.cs
- SortDescription.cs
- CodeVariableDeclarationStatement.cs
- XmlAttributeOverrides.cs
- CrossContextChannel.cs
- AutomationPatternInfo.cs
- XmlLinkedNode.cs
- BaseDataBoundControl.cs
- ResXResourceWriter.cs
- ConversionContext.cs
- COM2ComponentEditor.cs
- PackWebRequest.cs
- ClientType.cs
- DoubleCollectionConverter.cs
- OdbcConnection.cs
- BezierSegment.cs
- ImageSourceValueSerializer.cs
- HostedNamedPipeTransportManager.cs
- TrustManagerMoreInformation.cs
- AdornerLayer.cs
- ScriptingProfileServiceSection.cs
- ConfigXmlText.cs
- AjaxFrameworkAssemblyAttribute.cs
- UiaCoreApi.cs
- LocatorPartList.cs
- StylusButtonCollection.cs
- RouteValueDictionary.cs
- WebPartConnectionsDisconnectVerb.cs
- GenerateScriptTypeAttribute.cs
- ConstructorExpr.cs
- counter.cs
- QueryableFilterUserControl.cs
- DesignerTransaction.cs
- Vector3DKeyFrameCollection.cs
- TemplateBindingExpressionConverter.cs
- MethodImplAttribute.cs
- DataGridTableCollection.cs
- FtpWebRequest.cs
- PointAnimationBase.cs
- RelationshipWrapper.cs
- FixedFlowMap.cs
- HTTPNotFoundHandler.cs
- ReadOnlyTernaryTree.cs
- TraceSource.cs
- TextModifier.cs
- PartManifestEntry.cs
- ObjectParameterCollection.cs
- WsdlImporterElementCollection.cs
- ImageIndexConverter.cs