Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / xsp / System / Web / UI / StateBag.cs / 1 / StateBag.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI { using System.Text; using System.IO; using System.Collections; using System.Collections.Specialized; using System.Reflection; using System.Web.Util; using System.Security.Permissions; /* * The StateBag class is a helper class used to manage state of properties. * The class stores name/value pairs as string/object and tracks modifications of * properties after being 'marked'. This class is used as the primary storage * mechanism for all HtmlControls and WebControls. */ ////// [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] public sealed class StateBag : IStateManager, IDictionary { private IDictionary bag; private bool marked; private bool ignoreCase; /* * Constructs an StateBag */ ///Manages the state of Web Forms control properties. This /// class stores attribute/value pairs as string/object and tracks changes to these /// attributes, which are treated as properties, after the Page.Init /// method is executed for a /// page request. ////// Only values changed after Page.Init /// has executed are persisted to the page's view state. /// ////// public StateBag() : this(false) { } /* * Constructs an StateBag */ ///Initializes a new instance of the ///class. /// public StateBag(bool ignoreCase) { marked = false; this.ignoreCase = ignoreCase; bag = CreateBag(); } /* * Return count of number of StateItems in the bag. */ ///Initializes a new instance of the ///class that allows stored state /// values to be case-insensitive. /// public int Count { get { return bag.Count; } } /* * Returns a collection of keys. */ ///Indicates the number of items in the ///object. This property is /// read-only. /// public ICollection Keys { get { return bag.Keys; } } /* * Returns a collection of values. */ ///Indicates a collection of keys representing the items in the ///object. /// This property is read-only. /// public ICollection Values { get { return bag.Values; } } /* * Get or set value of a StateItem. * A set will automatically add a new StateItem for a * key which is not already in the bag. A set to null * will remove the item if set before mark, otherwise * a null set will be saved to allow tracking of state * removed after mark. */ ///Indicates a collection of view state values in the ///object. /// This property is read-only. /// public object this[string key] { get { if (String.IsNullOrEmpty(key)) throw ExceptionUtil.ParameterNullOrEmpty("key"); StateItem item = bag[key] as StateItem; if (item != null) return item.Value; return null; } set { Add(key,value); } } /* * Private implementation of IDictionary item accessor */ ///Indicates the value of an item stored in the /// ////// object. Setting this property with a key not already stored in the StateBag will /// add an item to the bag. If you set this property to before /// the TrackState method is called on an item will remove it from the bag. Otherwise, /// when you set this property to /// the key will be saved to allow tracking of the item's state. object IDictionary.this[object key] { get { return this[(string) key]; } set { this[(string) key] = value; } } private IDictionary CreateBag() { return new HybridDictionary(ignoreCase); } /* * Add a new StateItem or update an existing StateItem in the bag. */ /// /// public StateItem Add(string key,object value) { if (String.IsNullOrEmpty(key)) throw ExceptionUtil.ParameterNullOrEmpty("key"); StateItem item = bag[key] as StateItem; if (item == null) { if (value != null || marked) { item = new StateItem(value); bag.Add(key,item); } } else { if (value == null && !marked) { bag.Remove(key); } else { item.Value = value; } } if (item != null && marked) { item.IsDirty = true; } return item; } /* * Private implementation of IDictionary Add */ ///[To be supplied.] ///void IDictionary.Add(object key,object value) { Add((string) key, value); } /* * Clear all StateItems from the bag. */ /// /// public void Clear() { bag.Clear(); } /* * Get an enumerator for the StateItems. */ ///Removes all controls from the ///object. /// public IDictionaryEnumerator GetEnumerator() { return bag.GetEnumerator(); } /* * Return the dirty flag of the state item. * Returns false if there is not an item for given key. */ ///Returns an enumerator that iterates over the key/value pairs stored in /// the ///. /// public bool IsItemDirty(string key) { StateItem item = bag[key] as StateItem; if (item != null) return item.IsDirty; return false; } /* * Return true if 'marked' and state changes are being tracked. */ ///Checks an item stored in the ///to see if it has been /// modified. /// internal bool IsTrackingViewState { get { return marked; } } /* * Restore state that was previously saved via SaveViewState. */ ///Determines if state changes in the StateBag object's store are being tracked. ////// internal void LoadViewState(object state) { if (state != null) { ArrayList data = (ArrayList)state; for (int i = 0; i < data.Count; i += 2) { #if OBJECTSTATEFORMATTER string key = ((IndexedString)data[i]).Value; #else string key = (string)data[i]; #endif object value = data[i + 1]; Add(key, value); } } } /* * Start tracking state changes after "mark". */ ///Loads the specified previously saved state information ////// internal void TrackViewState() { marked = true; } /* * Remove a StateItem from the bag altogether regardless of marked. * Used internally by controls. */ ///Initiates the tracking of state changes for items stored in the /// ///object. /// public void Remove(string key) { bag.Remove(key); } /* * Private implementation of IDictionary Remove */ ///Removes the specified item from the ///object. void IDictionary.Remove(object key) { Remove((string) key); } /* * Return object containing state that has been modified since "mark". * Returns null if there is no modified state. */ /// /// internal object SaveViewState() { ArrayList data = null; // if (bag.Count != 0) { IDictionaryEnumerator e = bag.GetEnumerator(); while (e.MoveNext()) { StateItem item = (StateItem)(e.Value); if (item.IsDirty) { if (data == null) { data = new ArrayList(); } #if OBJECTSTATEFORMATTER data.Add(new IndexedString((string)e.Key)); #else data.Add(e.Key); #endif data.Add(item.Value); } } } return data; } ///Returns an object that contains all state changes for items stored in the /// ///object. /// Sets the dirty state of all item values currently in the StateBag. /// public void SetDirty(bool dirty) { if (bag.Count != 0) { foreach (StateItem item in bag.Values) { item.IsDirty = dirty; } } } /* * Internal method for setting dirty flag on a state item. * Used internallly to prevent state management of certain properties. */ ////// /// public void SetItemDirty(string key,bool dirty) { StateItem item = bag[key] as StateItem; if (item != null) { item.IsDirty = dirty; } } ///bool IDictionary.IsFixedSize { get { return false; } } /// bool IDictionary.IsReadOnly { get { return false; } } /// bool ICollection.IsSynchronized { get { return false;} } /// object ICollection.SyncRoot { get {return this; } } /// bool IDictionary.Contains(object key) { return bag.Contains((string) key); } /// IEnumerator IEnumerable.GetEnumerator() { return ((IDictionary)this).GetEnumerator(); } /// void ICollection.CopyTo(Array array, int index) { Values.CopyTo(array, index); } /* * Return true if tracking state changes. * Method of private interface, IStateManager. */ /// bool IStateManager.IsTrackingViewState { get { return IsTrackingViewState; } } /* * Load previously saved state. * Method of private interface, IStateManager. */ /// void IStateManager.LoadViewState(object state) { LoadViewState(state); } /* * Start tracking state changes. * Method of private interface, IStateManager. */ /// void IStateManager.TrackViewState() { TrackViewState(); } /* * Return object containing state changes. * Method of private interface, IStateManager. */ /// object IStateManager.SaveViewState() { return SaveViewState(); } } }
Link Menu
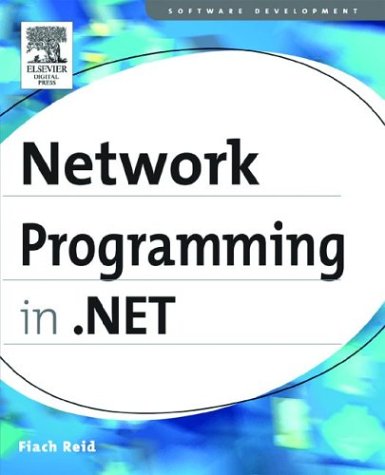
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SHA1.cs
- MemberJoinTreeNode.cs
- DrawingBrush.cs
- WithParamAction.cs
- CodeArrayIndexerExpression.cs
- FamilyMapCollection.cs
- DocumentXmlWriter.cs
- GetMemberBinder.cs
- CommandConverter.cs
- DataGridViewImageColumn.cs
- OleAutBinder.cs
- AliasExpr.cs
- FindCompletedEventArgs.cs
- PolicyLevel.cs
- XsltLoader.cs
- DataControlButton.cs
- EditorAttribute.cs
- RawTextInputReport.cs
- LocalValueEnumerator.cs
- DataSysAttribute.cs
- BaseDataBoundControlDesigner.cs
- DesignTimeParseData.cs
- Style.cs
- InputScopeAttribute.cs
- MetabaseServerConfig.cs
- SeekStoryboard.cs
- CompositionDesigner.cs
- SynchronizingStream.cs
- KerberosSecurityTokenParameters.cs
- PrtCap_Builder.cs
- DbConnectionHelper.cs
- _UriTypeConverter.cs
- OrderedDictionary.cs
- _NegoState.cs
- DataRelationCollection.cs
- StylusPointCollection.cs
- LayoutTable.cs
- HttpHandlerActionCollection.cs
- TabItemAutomationPeer.cs
- RequestCachePolicyConverter.cs
- AudioException.cs
- MenuCommand.cs
- NamespaceCollection.cs
- PipelineModuleStepContainer.cs
- WindowsClaimSet.cs
- InstancePersistenceCommandException.cs
- SpeechSynthesizer.cs
- DebugViewWriter.cs
- CryptoApi.cs
- ShaderEffect.cs
- FaultDesigner.cs
- ConnectivityStatus.cs
- Int64AnimationBase.cs
- WebPartTransformerAttribute.cs
- HMACMD5.cs
- SeekableReadStream.cs
- columnmapfactory.cs
- NavigateEvent.cs
- DataGridViewLayoutData.cs
- NCryptSafeHandles.cs
- ParameterModifier.cs
- DataGridViewSortCompareEventArgs.cs
- ValidationManager.cs
- ObjectAssociationEndMapping.cs
- ChannelPoolSettings.cs
- RTLAwareMessageBox.cs
- JsonWriter.cs
- xml.cs
- SoapAttributes.cs
- SoapFault.cs
- WebPartDescription.cs
- InfoCardServiceInstallComponent.cs
- ValidatingReaderNodeData.cs
- TrustManagerMoreInformation.cs
- DataColumnPropertyDescriptor.cs
- DbMetaDataColumnNames.cs
- WebReferenceCollection.cs
- RSATokenProvider.cs
- HelpKeywordAttribute.cs
- CodeMemberField.cs
- TrustLevel.cs
- Debug.cs
- FolderNameEditor.cs
- MediaElement.cs
- GreenMethods.cs
- XMLUtil.cs
- Attributes.cs
- HMACRIPEMD160.cs
- ISAPIWorkerRequest.cs
- PersonalizationState.cs
- OracleConnectionStringBuilder.cs
- KoreanLunisolarCalendar.cs
- IisTraceWebEventProvider.cs
- PreloadedPackages.cs
- BypassElement.cs
- WSHttpBinding.cs
- CfgParser.cs
- CrossContextChannel.cs
- ConstraintStruct.cs
- CellIdBoolean.cs