Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Core / System / Linq / Parallel / QueryOperators / Inlined / NullableIntSumAggregationOperator.cs / 1305376 / NullableIntSumAggregationOperator.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== // =+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+ // // NullableIntSumAggregationOperator.cs // //[....] // // =-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=- using System.Collections.Generic; using System.Diagnostics.Contracts; using System.Threading; namespace System.Linq.Parallel { ////// An inlined sum aggregation and its enumerator, for Nullable ints. /// internal sealed class NullableIntSumAggregationOperator : InlinedAggregationOperator{ //---------------------------------------------------------------------------------------- // Constructs a new instance of a sum associative operator. // internal NullableIntSumAggregationOperator(IEnumerable child) : base(child) { } //--------------------------------------------------------------------------------------- // Executes the entire query tree, and aggregates the intermediate results into the // final result based on the binary operators and final reduction. // // Return Value: // The single result of aggregation. // protected override int? InternalAggregate(ref Exception singularExceptionToThrow) { // Because the final reduction is typically much cheaper than the intermediate // reductions over the individual partitions, and because each parallel partition // will do a lot of work to produce a single output element, we prefer to turn off // pipelining, and process the final reductions serially. using (IEnumerator enumerator = GetEnumerator(ParallelMergeOptions.FullyBuffered, true)) { // We just reduce the elements in each output partition. int sum = 0; while (enumerator.MoveNext()) { checked { sum += enumerator.Current.GetValueOrDefault(); } } return sum; } } //--------------------------------------------------------------------------------------- // Creates an enumerator that is used internally for the final aggregation step. // protected override QueryOperatorEnumerator CreateEnumerator ( int index, int count, QueryOperatorEnumerator source, object sharedData, CancellationToken cancellationToken) { return new NullableIntSumAggregationOperatorEnumerator (source, index, cancellationToken); } //--------------------------------------------------------------------------------------- // This enumerator type encapsulates the intermediary aggregation over the underlying // (possibly partitioned) data source. // private class NullableIntSumAggregationOperatorEnumerator : InlinedAggregationOperatorEnumerator { private QueryOperatorEnumerator m_source; // The source data. //---------------------------------------------------------------------------------------- // Instantiates a new aggregation operator. // internal NullableIntSumAggregationOperatorEnumerator(QueryOperatorEnumerator source, int partitionIndex, CancellationToken cancellationToken) : base(partitionIndex, cancellationToken) { Contract.Assert(source != null); m_source = source; } //--------------------------------------------------------------------------------------- // Tallies up the sum of the underlying data source, walking the entire thing the first // time MoveNext is called on this object. // protected override bool MoveNextCore(ref int? currentElement) { int? element = default(int?); TKey keyUnused = default(TKey); QueryOperatorEnumerator source = m_source; if (source.MoveNext(ref element, ref keyUnused)) { // We just scroll through the enumerator and accumulate the sum. int tempSum = 0; int i = 0; do { if ((i++ & CancellationState.POLL_INTERVAL) == 0) CancellationState.ThrowIfCanceled(m_cancellationToken); checked { tempSum += element.GetValueOrDefault(); } } while (source.MoveNext(ref element, ref keyUnused)); // The sum has been calculated. Now just return. currentElement = new int?(tempSum); return true; } return false; } //---------------------------------------------------------------------------------------- // Dispose of resources associated with the underlying enumerator. // protected override void Dispose(bool disposing) { Contract.Assert(m_source != null); m_source.Dispose(); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== // =+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+ // // NullableIntSumAggregationOperator.cs // // [....] // // =-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=- using System.Collections.Generic; using System.Diagnostics.Contracts; using System.Threading; namespace System.Linq.Parallel { ////// An inlined sum aggregation and its enumerator, for Nullable ints. /// internal sealed class NullableIntSumAggregationOperator : InlinedAggregationOperator{ //---------------------------------------------------------------------------------------- // Constructs a new instance of a sum associative operator. // internal NullableIntSumAggregationOperator(IEnumerable child) : base(child) { } //--------------------------------------------------------------------------------------- // Executes the entire query tree, and aggregates the intermediate results into the // final result based on the binary operators and final reduction. // // Return Value: // The single result of aggregation. // protected override int? InternalAggregate(ref Exception singularExceptionToThrow) { // Because the final reduction is typically much cheaper than the intermediate // reductions over the individual partitions, and because each parallel partition // will do a lot of work to produce a single output element, we prefer to turn off // pipelining, and process the final reductions serially. using (IEnumerator enumerator = GetEnumerator(ParallelMergeOptions.FullyBuffered, true)) { // We just reduce the elements in each output partition. int sum = 0; while (enumerator.MoveNext()) { checked { sum += enumerator.Current.GetValueOrDefault(); } } return sum; } } //--------------------------------------------------------------------------------------- // Creates an enumerator that is used internally for the final aggregation step. // protected override QueryOperatorEnumerator CreateEnumerator ( int index, int count, QueryOperatorEnumerator source, object sharedData, CancellationToken cancellationToken) { return new NullableIntSumAggregationOperatorEnumerator (source, index, cancellationToken); } //--------------------------------------------------------------------------------------- // This enumerator type encapsulates the intermediary aggregation over the underlying // (possibly partitioned) data source. // private class NullableIntSumAggregationOperatorEnumerator : InlinedAggregationOperatorEnumerator { private QueryOperatorEnumerator m_source; // The source data. //---------------------------------------------------------------------------------------- // Instantiates a new aggregation operator. // internal NullableIntSumAggregationOperatorEnumerator(QueryOperatorEnumerator source, int partitionIndex, CancellationToken cancellationToken) : base(partitionIndex, cancellationToken) { Contract.Assert(source != null); m_source = source; } //--------------------------------------------------------------------------------------- // Tallies up the sum of the underlying data source, walking the entire thing the first // time MoveNext is called on this object. // protected override bool MoveNextCore(ref int? currentElement) { int? element = default(int?); TKey keyUnused = default(TKey); QueryOperatorEnumerator source = m_source; if (source.MoveNext(ref element, ref keyUnused)) { // We just scroll through the enumerator and accumulate the sum. int tempSum = 0; int i = 0; do { if ((i++ & CancellationState.POLL_INTERVAL) == 0) CancellationState.ThrowIfCanceled(m_cancellationToken); checked { tempSum += element.GetValueOrDefault(); } } while (source.MoveNext(ref element, ref keyUnused)); // The sum has been calculated. Now just return. currentElement = new int?(tempSum); return true; } return false; } //---------------------------------------------------------------------------------------- // Dispose of resources associated with the underlying enumerator. // protected override void Dispose(bool disposing) { Contract.Assert(m_source != null); m_source.Dispose(); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
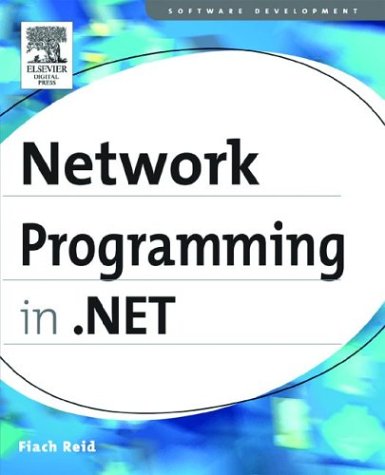
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- WebPartsPersonalizationAuthorization.cs
- ListViewContainer.cs
- BuildProvider.cs
- IOException.cs
- QilIterator.cs
- MailWebEventProvider.cs
- SizeIndependentAnimationStorage.cs
- URLEditor.cs
- DetailsViewCommandEventArgs.cs
- CqlParser.cs
- ProcessManager.cs
- DataGridComponentEditor.cs
- MoveSizeWinEventHandler.cs
- CancellationTokenSource.cs
- MsmqTransportElement.cs
- DebugManager.cs
- EnvironmentPermission.cs
- RemoteWebConfigurationHostServer.cs
- XAMLParseException.cs
- AssociationSetEnd.cs
- StopStoryboard.cs
- FileRecordSequenceHelper.cs
- securitycriticaldataClass.cs
- FactoryGenerator.cs
- BitVector32.cs
- SqlCacheDependencySection.cs
- GcHandle.cs
- PassportPrincipal.cs
- MenuCommand.cs
- XmlTextWriter.cs
- KeyFrames.cs
- _SslState.cs
- LoginView.cs
- UserControlFileEditor.cs
- _NetworkingPerfCounters.cs
- VisualStyleTypesAndProperties.cs
- ClusterRegistryConfigurationProvider.cs
- CatalogPartCollection.cs
- ZipIOLocalFileDataDescriptor.cs
- ValueChangedEventManager.cs
- _AutoWebProxyScriptEngine.cs
- AttachedPropertyBrowsableForTypeAttribute.cs
- ReferencedAssembly.cs
- DebugInfoGenerator.cs
- XXXInfos.cs
- VoiceObjectToken.cs
- XmlSchemaAny.cs
- MimeTextImporter.cs
- StatusBarItem.cs
- _IPv6Address.cs
- PackageProperties.cs
- TileBrush.cs
- TreeViewItem.cs
- SqlConnectionHelper.cs
- InputMethodStateChangeEventArgs.cs
- Menu.cs
- OracleDateTime.cs
- ContextProperty.cs
- WinInet.cs
- DBCommand.cs
- WriteTimeStream.cs
- ToolboxItem.cs
- HTMLTextWriter.cs
- comcontractssection.cs
- XmlDownloadManager.cs
- CodeIdentifiers.cs
- TTSEngineTypes.cs
- CounterSet.cs
- DetailsViewUpdateEventArgs.cs
- SchemaTableColumn.cs
- SoapCodeExporter.cs
- ProjectionPlanCompiler.cs
- ProgressBar.cs
- OleDbStruct.cs
- PropertyTab.cs
- WorkflowInstanceQuery.cs
- ITextView.cs
- PixelFormatConverter.cs
- CultureSpecificStringDictionary.cs
- State.cs
- WpfXamlLoader.cs
- RequestUriProcessor.cs
- FormViewDesigner.cs
- GridViewRowEventArgs.cs
- PropertyReference.cs
- OneWayElement.cs
- FixedElement.cs
- NetWebProxyFinder.cs
- SEHException.cs
- SchemaNames.cs
- Help.cs
- PropertyMapper.cs
- InvalidPipelineStoreException.cs
- MachinePropertyVariants.cs
- NameValuePair.cs
- DataGridViewCellCollection.cs
- PlanCompiler.cs
- SemanticKeyElement.cs
- X509Utils.cs
- PointAnimationUsingPath.cs