Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / CompMod / System / Collections / Specialized / StringCollection.cs / 1 / StringCollection.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Collections.Specialized { using System.Diagnostics; using System.Collections; ////// [ Serializable, ] public class StringCollection : IList { private ArrayList data = new ArrayList(); ///Represents a collection of strings. ////// public string this[int index] { get { return ((string)data[index]); } set { data[index] = value; } } ///Represents the entry at the specified index of the ///. /// public int Count { get { return data.Count; } } bool IList.IsReadOnly { get { return false; } } bool IList.IsFixedSize { get { return false; } } ///Gets the number of strings in the /// ///. /// public int Add(string value) { return data.Add(value); } ///Adds a string with the specified value to the /// ///. /// public void AddRange(string[] value) { if (value == null) { throw new ArgumentNullException("value"); } data.AddRange(value); } ///Copies the elements of a string array to the end of the ///. /// public void Clear() { data.Clear(); } ///Removes all the strings from the /// ///. /// public bool Contains(string value) { return data.Contains(value); } ///Gets a value indicating whether the /// ///contains a string with the specified /// value. /// public void CopyTo(string[] array, int index) { data.CopyTo(array, index); } ///Copies the ///values to a one-dimensional instance at the /// specified index. /// public StringEnumerator GetEnumerator() { return new StringEnumerator(this); } ///Returns an enumerator that can iterate through /// the ///. /// public int IndexOf(string value) { return data.IndexOf(value); } ///Returns the index of the first occurrence of a string in /// the ///. /// public void Insert(int index, string value) { data.Insert(index, value); } ///Inserts a string into the ///at the specified /// index. /// public bool IsReadOnly { get { return false; } } ///Gets a value indicating whether the ///is read-only. /// public bool IsSynchronized { get { return false; } } ///Gets a value indicating whether access to the /// ////// is synchronized (thread-safe). /// public void Remove(string value) { data.Remove(value); } ///Removes a specific string from the /// ///. /// public void RemoveAt(int index) { data.RemoveAt(index); } ///Removes the string at the specified index of the ///. /// public object SyncRoot { get { return data.SyncRoot; } } object IList.this[int index] { get { return this[index]; } set { this[index] = (string)value; } } int IList.Add(object value) { return Add((string)value); } bool IList.Contains(object value) { return Contains((string) value); } int IList.IndexOf(object value) { return IndexOf((string)value); } void IList.Insert(int index, object value) { Insert(index, (string)value); } void IList.Remove(object value) { Remove((string)value); } void ICollection.CopyTo(Array array, int index) { data.CopyTo(array, index); } IEnumerator IEnumerable.GetEnumerator() { return data.GetEnumerator(); } } ///Gets an object that can be used to synchronize access to the ///. /// public class StringEnumerator { private System.Collections.IEnumerator baseEnumerator; private System.Collections.IEnumerable temp; internal StringEnumerator(StringCollection mappings) { this.temp = (IEnumerable)(mappings); this.baseEnumerator = temp.GetEnumerator(); } ///[To be supplied.] ////// public string Current { get { return (string)(baseEnumerator.Current); } } ///[To be supplied.] ////// public bool MoveNext() { return baseEnumerator.MoveNext(); } ///[To be supplied.] ////// public void Reset() { baseEnumerator.Reset(); } } }[To be supplied.] ///
Link Menu
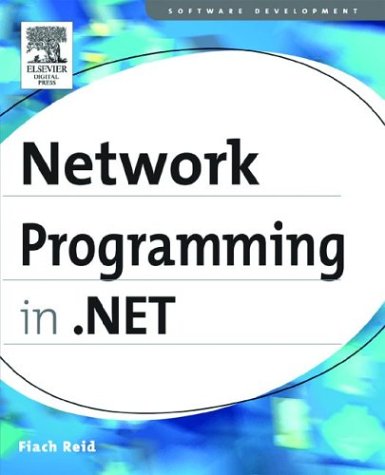
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XmlLanguage.cs
- Attributes.cs
- MemberListBinding.cs
- PrincipalPermission.cs
- ProcessInfo.cs
- ListViewUpdateEventArgs.cs
- SiteMapPath.cs
- WebPartAuthorizationEventArgs.cs
- UnsafeNativeMethods.cs
- CachedFontFamily.cs
- ByValueEqualityComparer.cs
- WebService.cs
- ActivatableWorkflowsQueryResult.cs
- CodeMemberField.cs
- Clause.cs
- StaticDataManager.cs
- HttpResponse.cs
- SemanticAnalyzer.cs
- BinaryReader.cs
- RotateTransform.cs
- rsa.cs
- BitmapDecoder.cs
- GroupBoxRenderer.cs
- MobileUserControlDesigner.cs
- TimeSpanStorage.cs
- TextMarkerSource.cs
- BindingWorker.cs
- NestedContainer.cs
- SplitterCancelEvent.cs
- HttpDictionary.cs
- RbTree.cs
- DocumentSchemaValidator.cs
- Visual3DCollection.cs
- ExpressionLexer.cs
- _SslSessionsCache.cs
- ToolStripDropDown.cs
- FragmentQueryKB.cs
- ReadOnlyNameValueCollection.cs
- DATA_BLOB.cs
- ECDiffieHellmanCngPublicKey.cs
- TypeReference.cs
- sqlser.cs
- DebugView.cs
- X509CertificateStore.cs
- XmlUrlResolver.cs
- WebPartDescription.cs
- Ray3DHitTestResult.cs
- RangeContentEnumerator.cs
- SynchronizedInputAdaptor.cs
- METAHEADER.cs
- TraceListener.cs
- DrawingBrush.cs
- ListViewHitTestInfo.cs
- Knowncolors.cs
- MSAAWinEventWrap.cs
- StringWriter.cs
- TypeToStringValueConverter.cs
- DataGridViewRowCollection.cs
- BuildProvider.cs
- PackagePartCollection.cs
- ProcessModuleCollection.cs
- DataGridViewColumnCollectionDialog.cs
- XmlNodeChangedEventArgs.cs
- ClientTarget.cs
- WebPartMinimizeVerb.cs
- SerializationHelper.cs
- elementinformation.cs
- InlineCollection.cs
- SQLResource.cs
- CodeTryCatchFinallyStatement.cs
- PeerNearMe.cs
- SqlTransaction.cs
- OneWayChannelFactory.cs
- FormViewModeEventArgs.cs
- ModifiableIteratorCollection.cs
- X509CertificateCollection.cs
- MSG.cs
- TextRange.cs
- Ray3DHitTestResult.cs
- SerialErrors.cs
- HttpPostedFile.cs
- DataBoundLiteralControl.cs
- ResourcePropertyMemberCodeDomSerializer.cs
- WebAdminConfigurationHelper.cs
- EntityDataSourceStatementEditor.cs
- RNGCryptoServiceProvider.cs
- NullableIntSumAggregationOperator.cs
- TextDecorations.cs
- MonthCalendar.cs
- TextModifierScope.cs
- SqlDependencyListener.cs
- ControlPaint.cs
- QilCloneVisitor.cs
- RuleSettings.cs
- ShellProvider.cs
- VBIdentifierNameEditor.cs
- HttpCacheVaryByContentEncodings.cs
- XamlReaderHelper.cs
- Substitution.cs
- ZipIOZip64EndOfCentralDirectoryLocatorBlock.cs