Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / fx / src / Data / System / Data / SQLTypes / SQLResource.cs / 1 / SQLResource.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //junfang //[....] //[....] //----------------------------------------------------------------------------- //************************************************************************* // @File: SQLResource.cs // // Create by: JunFang // // Purpose: Implementation of utilities in COM+ SQL Types Library. // Includes interface INullable, exceptions SqlNullValueException // and SqlTruncateException, and SQLDebug class. // // Notes: // // History: // // 10/22/99 JunFang Created. // // @EndHeader@ //************************************************************************* namespace System.Data.SqlTypes { using System; using System.Data; using System.Globalization; internal sealed class SQLResource { private SQLResource() { /* prevent utility class from being insantiated*/ } internal static readonly String NullString = Res.GetString(Res.SqlMisc_NullString); internal static readonly String MessageString = Res.GetString(Res.SqlMisc_MessageString); internal static readonly String ArithOverflowMessage = Res.GetString(Res.SqlMisc_ArithOverflowMessage); internal static readonly String DivideByZeroMessage = Res.GetString(Res.SqlMisc_DivideByZeroMessage); internal static readonly String NullValueMessage = Res.GetString(Res.SqlMisc_NullValueMessage); internal static readonly String TruncationMessage = Res.GetString(Res.SqlMisc_TruncationMessage); internal static readonly String DateTimeOverflowMessage = Res.GetString(Res.SqlMisc_DateTimeOverflowMessage); internal static readonly String ConcatDiffCollationMessage = Res.GetString(Res.SqlMisc_ConcatDiffCollationMessage); internal static readonly String CompareDiffCollationMessage = Res.GetString(Res.SqlMisc_CompareDiffCollationMessage); internal static readonly String InvalidFlagMessage = Res.GetString(Res.SqlMisc_InvalidFlagMessage); internal static readonly String NumeToDecOverflowMessage = Res.GetString(Res.SqlMisc_NumeToDecOverflowMessage); internal static readonly String ConversionOverflowMessage = Res.GetString(Res.SqlMisc_ConversionOverflowMessage); internal static readonly String InvalidDateTimeMessage = Res.GetString(Res.SqlMisc_InvalidDateTimeMessage); internal static readonly String TimeZoneSpecifiedMessage = Res.GetString(Res.SqlMisc_TimeZoneSpecifiedMessage); internal static readonly String InvalidArraySizeMessage = Res.GetString(Res.SqlMisc_InvalidArraySizeMessage); internal static readonly String InvalidPrecScaleMessage = Res.GetString(Res.SqlMisc_InvalidPrecScaleMessage); internal static readonly String FormatMessage = Res.GetString(Res.SqlMisc_FormatMessage); internal static readonly String NotFilledMessage = Res.GetString(Res.SqlMisc_NotFilledMessage); internal static readonly String AlreadyFilledMessage = Res.GetString(Res.SqlMisc_AlreadyFilledMessage); internal static readonly String ClosedXmlReaderMessage = Res.GetString(Res.SqlMisc_ClosedXmlReaderMessage); internal static String InvalidOpStreamClosed(String method) { return Res.GetString(Res.SqlMisc_InvalidOpStreamClosed, method); } internal static String InvalidOpStreamNonWritable(String method) { return Res.GetString(Res.SqlMisc_InvalidOpStreamNonWritable, method); } internal static String InvalidOpStreamNonReadable(String method) { return Res.GetString(Res.SqlMisc_InvalidOpStreamNonReadable, method); } internal static String InvalidOpStreamNonSeekable(String method) { return Res.GetString(Res.SqlMisc_InvalidOpStreamNonSeekable, method); } } // SqlResource } // namespace System // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //junfang //[....] //[....] //----------------------------------------------------------------------------- //************************************************************************* // @File: SQLResource.cs // // Create by: JunFang // // Purpose: Implementation of utilities in COM+ SQL Types Library. // Includes interface INullable, exceptions SqlNullValueException // and SqlTruncateException, and SQLDebug class. // // Notes: // // History: // // 10/22/99 JunFang Created. // // @EndHeader@ //************************************************************************* namespace System.Data.SqlTypes { using System; using System.Data; using System.Globalization; internal sealed class SQLResource { private SQLResource() { /* prevent utility class from being insantiated*/ } internal static readonly String NullString = Res.GetString(Res.SqlMisc_NullString); internal static readonly String MessageString = Res.GetString(Res.SqlMisc_MessageString); internal static readonly String ArithOverflowMessage = Res.GetString(Res.SqlMisc_ArithOverflowMessage); internal static readonly String DivideByZeroMessage = Res.GetString(Res.SqlMisc_DivideByZeroMessage); internal static readonly String NullValueMessage = Res.GetString(Res.SqlMisc_NullValueMessage); internal static readonly String TruncationMessage = Res.GetString(Res.SqlMisc_TruncationMessage); internal static readonly String DateTimeOverflowMessage = Res.GetString(Res.SqlMisc_DateTimeOverflowMessage); internal static readonly String ConcatDiffCollationMessage = Res.GetString(Res.SqlMisc_ConcatDiffCollationMessage); internal static readonly String CompareDiffCollationMessage = Res.GetString(Res.SqlMisc_CompareDiffCollationMessage); internal static readonly String InvalidFlagMessage = Res.GetString(Res.SqlMisc_InvalidFlagMessage); internal static readonly String NumeToDecOverflowMessage = Res.GetString(Res.SqlMisc_NumeToDecOverflowMessage); internal static readonly String ConversionOverflowMessage = Res.GetString(Res.SqlMisc_ConversionOverflowMessage); internal static readonly String InvalidDateTimeMessage = Res.GetString(Res.SqlMisc_InvalidDateTimeMessage); internal static readonly String TimeZoneSpecifiedMessage = Res.GetString(Res.SqlMisc_TimeZoneSpecifiedMessage); internal static readonly String InvalidArraySizeMessage = Res.GetString(Res.SqlMisc_InvalidArraySizeMessage); internal static readonly String InvalidPrecScaleMessage = Res.GetString(Res.SqlMisc_InvalidPrecScaleMessage); internal static readonly String FormatMessage = Res.GetString(Res.SqlMisc_FormatMessage); internal static readonly String NotFilledMessage = Res.GetString(Res.SqlMisc_NotFilledMessage); internal static readonly String AlreadyFilledMessage = Res.GetString(Res.SqlMisc_AlreadyFilledMessage); internal static readonly String ClosedXmlReaderMessage = Res.GetString(Res.SqlMisc_ClosedXmlReaderMessage); internal static String InvalidOpStreamClosed(String method) { return Res.GetString(Res.SqlMisc_InvalidOpStreamClosed, method); } internal static String InvalidOpStreamNonWritable(String method) { return Res.GetString(Res.SqlMisc_InvalidOpStreamNonWritable, method); } internal static String InvalidOpStreamNonReadable(String method) { return Res.GetString(Res.SqlMisc_InvalidOpStreamNonReadable, method); } internal static String InvalidOpStreamNonSeekable(String method) { return Res.GetString(Res.SqlMisc_InvalidOpStreamNonSeekable, method); } } // SqlResource } // namespace System // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
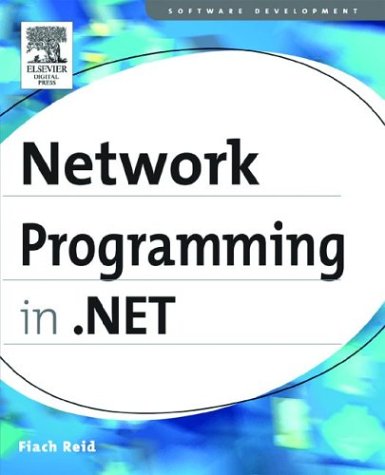
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- _ListenerRequestStream.cs
- CreateUserErrorEventArgs.cs
- PropertyConverter.cs
- ItemCollection.cs
- RuntimeVariableList.cs
- ServiceDesigner.xaml.cs
- NotImplementedException.cs
- AnnotationMap.cs
- ScriptResourceInfo.cs
- EastAsianLunisolarCalendar.cs
- Util.cs
- AnnotationAuthorChangedEventArgs.cs
- Point.cs
- QuerySettings.cs
- DbProviderFactoriesConfigurationHandler.cs
- KeyInstance.cs
- ToolStripPanelRow.cs
- GenericQueueSurrogate.cs
- TranslateTransform3D.cs
- BamlBinaryReader.cs
- ToolStripItemImageRenderEventArgs.cs
- ViewStateModeByIdAttribute.cs
- DataComponentNameHandler.cs
- ImageBrush.cs
- BitmapEffectInput.cs
- DataReceivedEventArgs.cs
- XmlProcessingInstruction.cs
- DrawingCollection.cs
- IdleTimeoutMonitor.cs
- MetabaseServerConfig.cs
- XhtmlBasicControlAdapter.cs
- ReceiveReply.cs
- KeyboardDevice.cs
- BuildProvider.cs
- ScriptingRoleServiceSection.cs
- DocumentViewerConstants.cs
- Encoding.cs
- NetworkInformationException.cs
- EnumerableRowCollectionExtensions.cs
- FontWeights.cs
- XmlCodeExporter.cs
- BooleanFunctions.cs
- WmlListAdapter.cs
- XmlNavigatorFilter.cs
- MouseEventArgs.cs
- UserUseLicenseDictionaryLoader.cs
- DataListItem.cs
- WebServiceClientProxyGenerator.cs
- WpfGeneratedKnownTypes.cs
- PtsContext.cs
- Effect.cs
- HttpPostServerProtocol.cs
- EventLogPermissionEntry.cs
- SqlDependency.cs
- RuntimeCompatibilityAttribute.cs
- ProvidePropertyAttribute.cs
- BufferedWebEventProvider.cs
- ConstraintCollection.cs
- ImageField.cs
- MediaElementAutomationPeer.cs
- ProgressBarAutomationPeer.cs
- SQLDecimal.cs
- PrintDialog.cs
- FocusTracker.cs
- ValidatorCompatibilityHelper.cs
- HwndStylusInputProvider.cs
- HttpResponseHeader.cs
- DynamicContractTypeBuilder.cs
- CompositeControl.cs
- SamlSecurityTokenAuthenticator.cs
- FormatConvertedBitmap.cs
- Compress.cs
- TextRunCacheImp.cs
- GenerateScriptTypeAttribute.cs
- SqlAliasesReferenced.cs
- ConsumerConnectionPoint.cs
- RectKeyFrameCollection.cs
- AspProxy.cs
- TaskbarItemInfo.cs
- InternalDuplexChannelListener.cs
- ConfigurationSectionGroup.cs
- TraversalRequest.cs
- SubqueryTrackingVisitor.cs
- coordinatorscratchpad.cs
- _SSPISessionCache.cs
- XmlSchemaComplexContentExtension.cs
- UserControlParser.cs
- cryptoapiTransform.cs
- BasicViewGenerator.cs
- TableCell.cs
- HelpEvent.cs
- PrintEvent.cs
- ListBindingHelper.cs
- WeakReference.cs
- ThrowOnMultipleAssignment.cs
- MetadataFile.cs
- DockAndAnchorLayout.cs
- XmlSchemaSet.cs
- HwndSubclass.cs
- WebPartTransformer.cs