Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Core / CSharp / System / Windows / Media / Animation / Generated / RectKeyFrameCollection.cs / 1 / RectKeyFrameCollection.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // Please see http://wiki/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- using MS.Internal; using System; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.Diagnostics; using System.Globalization; using System.Windows.Media.Animation; using System.Windows.Media.Media3D; namespace System.Windows.Media.Animation { ////// This collection is used in conjunction with a KeyFrameRectAnimation /// to animate a Rect property value along a set of key frames. /// public class RectKeyFrameCollection : Freezable, IList { #region Data private List_keyFrames; private static RectKeyFrameCollection s_emptyCollection; #endregion #region Constructors /// /// Creates a new RectKeyFrameCollection. /// public RectKeyFrameCollection() : base() { _keyFrames = new List< RectKeyFrame>(2); } #endregion #region Static Methods ////// An empty RectKeyFrameCollection. /// public static RectKeyFrameCollection Empty { get { if (s_emptyCollection == null) { RectKeyFrameCollection emptyCollection = new RectKeyFrameCollection(); emptyCollection._keyFrames = new List< RectKeyFrame>(0); emptyCollection.Freeze(); s_emptyCollection = emptyCollection; } return s_emptyCollection; } } #endregion #region Freezable ////// Creates a freezable copy of this RectKeyFrameCollection. /// ///The copy public new RectKeyFrameCollection Clone() { return (RectKeyFrameCollection)base.Clone(); } ////// Implementation of ///Freezable.CreateInstanceCore . ///The new Freezable. protected override Freezable CreateInstanceCore() { return new RectKeyFrameCollection(); } ////// Implementation of protected override void CloneCore(Freezable sourceFreezable) { RectKeyFrameCollection sourceCollection = (RectKeyFrameCollection) sourceFreezable; base.CloneCore(sourceFreezable); int count = sourceCollection._keyFrames.Count; _keyFrames = new List< RectKeyFrame>(count); for (int i = 0; i < count; i++) { RectKeyFrame keyFrame = (RectKeyFrame)sourceCollection._keyFrames[i].Clone(); _keyFrames.Add(keyFrame); OnFreezablePropertyChanged(null, keyFrame); } } ///Freezable.CloneCore . ////// Implementation of protected override void CloneCurrentValueCore(Freezable sourceFreezable) { RectKeyFrameCollection sourceCollection = (RectKeyFrameCollection) sourceFreezable; base.CloneCurrentValueCore(sourceFreezable); int count = sourceCollection._keyFrames.Count; _keyFrames = new List< RectKeyFrame>(count); for (int i = 0; i < count; i++) { RectKeyFrame keyFrame = (RectKeyFrame)sourceCollection._keyFrames[i].CloneCurrentValue(); _keyFrames.Add(keyFrame); OnFreezablePropertyChanged(null, keyFrame); } } ///Freezable.CloneCurrentValueCore . ////// Implementation of protected override void GetAsFrozenCore(Freezable sourceFreezable) { RectKeyFrameCollection sourceCollection = (RectKeyFrameCollection) sourceFreezable; base.GetAsFrozenCore(sourceFreezable); int count = sourceCollection._keyFrames.Count; _keyFrames = new List< RectKeyFrame>(count); for (int i = 0; i < count; i++) { RectKeyFrame keyFrame = (RectKeyFrame)sourceCollection._keyFrames[i].GetAsFrozen(); _keyFrames.Add(keyFrame); OnFreezablePropertyChanged(null, keyFrame); } } ///Freezable.GetAsFrozenCore . ////// Implementation of protected override void GetCurrentValueAsFrozenCore(Freezable sourceFreezable) { RectKeyFrameCollection sourceCollection = (RectKeyFrameCollection) sourceFreezable; base.GetCurrentValueAsFrozenCore(sourceFreezable); int count = sourceCollection._keyFrames.Count; _keyFrames = new List< RectKeyFrame>(count); for (int i = 0; i < count; i++) { RectKeyFrame keyFrame = (RectKeyFrame)sourceCollection._keyFrames[i].GetCurrentValueAsFrozen(); _keyFrames.Add(keyFrame); OnFreezablePropertyChanged(null, keyFrame); } } ///Freezable.GetCurrentValueAsFrozenCore . ////// /// protected override bool FreezeCore(bool isChecking) { bool canFreeze = base.FreezeCore(isChecking); for (int i = 0; i < _keyFrames.Count && canFreeze; i++) { canFreeze &= Freezable.Freeze(_keyFrames[i], isChecking); } return canFreeze; } #endregion #region IEnumerable ////// Returns an enumerator of the RectKeyFrames in the collection. /// public IEnumerator GetEnumerator() { ReadPreamble(); return _keyFrames.GetEnumerator(); } #endregion #region ICollection ////// Returns the number of RectKeyFrames in the collection. /// public int Count { get { ReadPreamble(); return _keyFrames.Count; } } ////// See public bool IsSynchronized { get { ReadPreamble(); return (IsFrozen || Dispatcher != null); } } ///ICollection.IsSynchronized . ////// See public object SyncRoot { get { ReadPreamble(); return ((ICollection)_keyFrames).SyncRoot; } } ///ICollection.SyncRoot . ////// Copies all of the RectKeyFrames in the collection to an /// array. /// void ICollection.CopyTo(Array array, int index) { ReadPreamble(); ((ICollection)_keyFrames).CopyTo(array, index); } ////// Copies all of the RectKeyFrames in the collection to an /// array of RectKeyFrames. /// public void CopyTo(RectKeyFrame[] array, int index) { ReadPreamble(); _keyFrames.CopyTo(array, index); } #endregion #region IList ////// Adds a RectKeyFrame to the collection. /// int IList.Add(object keyFrame) { return Add((RectKeyFrame)keyFrame); } ////// Adds a RectKeyFrame to the collection. /// public int Add(RectKeyFrame keyFrame) { if (keyFrame == null) { throw new ArgumentNullException("keyFrame"); } WritePreamble(); OnFreezablePropertyChanged(null, keyFrame); _keyFrames.Add(keyFrame); WritePostscript(); return _keyFrames.Count - 1; } ////// Removes all RectKeyFrames from the collection. /// public void Clear() { WritePreamble(); if (_keyFrames.Count > 0) { for (int i = 0; i < _keyFrames.Count; i++) { OnFreezablePropertyChanged(_keyFrames[i], null); } _keyFrames.Clear(); WritePostscript(); } } ////// Returns true of the collection contains the given RectKeyFrame. /// bool IList.Contains(object keyFrame) { return Contains((RectKeyFrame)keyFrame); } ////// Returns true of the collection contains the given RectKeyFrame. /// public bool Contains(RectKeyFrame keyFrame) { ReadPreamble(); return _keyFrames.Contains(keyFrame); } ////// Returns the index of a given RectKeyFrame in the collection. /// int IList.IndexOf(object keyFrame) { return IndexOf((RectKeyFrame)keyFrame); } ////// Returns the index of a given RectKeyFrame in the collection. /// public int IndexOf(RectKeyFrame keyFrame) { ReadPreamble(); return _keyFrames.IndexOf(keyFrame); } ////// Inserts a RectKeyFrame into a specific location in the collection. /// void IList.Insert(int index, object keyFrame) { Insert(index, (RectKeyFrame)keyFrame); } ////// Inserts a RectKeyFrame into a specific location in the collection. /// public void Insert(int index, RectKeyFrame keyFrame) { if (keyFrame == null) { throw new ArgumentNullException("keyFrame"); } WritePreamble(); OnFreezablePropertyChanged(null, keyFrame); _keyFrames.Insert(index, keyFrame); WritePostscript(); } ////// Returns true if the collection is frozen. /// public bool IsFixedSize { get { ReadPreamble(); return IsFrozen; } } ////// Returns true if the collection is frozen. /// public bool IsReadOnly { get { ReadPreamble(); return IsFrozen; } } ////// Removes a RectKeyFrame from the collection. /// void IList.Remove(object keyFrame) { Remove((RectKeyFrame)keyFrame); } ////// Removes a RectKeyFrame from the collection. /// public void Remove(RectKeyFrame keyFrame) { WritePreamble(); if (_keyFrames.Contains(keyFrame)) { OnFreezablePropertyChanged(keyFrame, null); _keyFrames.Remove(keyFrame); WritePostscript(); } } ////// Removes the RectKeyFrame at the specified index from the collection. /// public void RemoveAt(int index) { WritePreamble(); OnFreezablePropertyChanged(_keyFrames[index], null); _keyFrames.RemoveAt(index); WritePostscript(); } ////// Gets or sets the RectKeyFrame at a given index. /// object IList.this[int index] { get { return this[index]; } set { this[index] = (RectKeyFrame)value; } } ////// Gets or sets the RectKeyFrame at a given index. /// public RectKeyFrame this[int index] { get { ReadPreamble(); return _keyFrames[index]; } set { if (value == null) { throw new ArgumentNullException(String.Format(CultureInfo.InvariantCulture, "RectKeyFrameCollection[{0}]", index)); } WritePreamble(); if (value != _keyFrames[index]) { OnFreezablePropertyChanged(_keyFrames[index], value); _keyFrames[index] = value; Debug.Assert(_keyFrames[index] != null); WritePostscript(); } } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // Please see http://wiki/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- using MS.Internal; using System; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.Diagnostics; using System.Globalization; using System.Windows.Media.Animation; using System.Windows.Media.Media3D; namespace System.Windows.Media.Animation { ////// This collection is used in conjunction with a KeyFrameRectAnimation /// to animate a Rect property value along a set of key frames. /// public class RectKeyFrameCollection : Freezable, IList { #region Data private List_keyFrames; private static RectKeyFrameCollection s_emptyCollection; #endregion #region Constructors /// /// Creates a new RectKeyFrameCollection. /// public RectKeyFrameCollection() : base() { _keyFrames = new List< RectKeyFrame>(2); } #endregion #region Static Methods ////// An empty RectKeyFrameCollection. /// public static RectKeyFrameCollection Empty { get { if (s_emptyCollection == null) { RectKeyFrameCollection emptyCollection = new RectKeyFrameCollection(); emptyCollection._keyFrames = new List< RectKeyFrame>(0); emptyCollection.Freeze(); s_emptyCollection = emptyCollection; } return s_emptyCollection; } } #endregion #region Freezable ////// Creates a freezable copy of this RectKeyFrameCollection. /// ///The copy public new RectKeyFrameCollection Clone() { return (RectKeyFrameCollection)base.Clone(); } ////// Implementation of ///Freezable.CreateInstanceCore . ///The new Freezable. protected override Freezable CreateInstanceCore() { return new RectKeyFrameCollection(); } ////// Implementation of protected override void CloneCore(Freezable sourceFreezable) { RectKeyFrameCollection sourceCollection = (RectKeyFrameCollection) sourceFreezable; base.CloneCore(sourceFreezable); int count = sourceCollection._keyFrames.Count; _keyFrames = new List< RectKeyFrame>(count); for (int i = 0; i < count; i++) { RectKeyFrame keyFrame = (RectKeyFrame)sourceCollection._keyFrames[i].Clone(); _keyFrames.Add(keyFrame); OnFreezablePropertyChanged(null, keyFrame); } } ///Freezable.CloneCore . ////// Implementation of protected override void CloneCurrentValueCore(Freezable sourceFreezable) { RectKeyFrameCollection sourceCollection = (RectKeyFrameCollection) sourceFreezable; base.CloneCurrentValueCore(sourceFreezable); int count = sourceCollection._keyFrames.Count; _keyFrames = new List< RectKeyFrame>(count); for (int i = 0; i < count; i++) { RectKeyFrame keyFrame = (RectKeyFrame)sourceCollection._keyFrames[i].CloneCurrentValue(); _keyFrames.Add(keyFrame); OnFreezablePropertyChanged(null, keyFrame); } } ///Freezable.CloneCurrentValueCore . ////// Implementation of protected override void GetAsFrozenCore(Freezable sourceFreezable) { RectKeyFrameCollection sourceCollection = (RectKeyFrameCollection) sourceFreezable; base.GetAsFrozenCore(sourceFreezable); int count = sourceCollection._keyFrames.Count; _keyFrames = new List< RectKeyFrame>(count); for (int i = 0; i < count; i++) { RectKeyFrame keyFrame = (RectKeyFrame)sourceCollection._keyFrames[i].GetAsFrozen(); _keyFrames.Add(keyFrame); OnFreezablePropertyChanged(null, keyFrame); } } ///Freezable.GetAsFrozenCore . ////// Implementation of protected override void GetCurrentValueAsFrozenCore(Freezable sourceFreezable) { RectKeyFrameCollection sourceCollection = (RectKeyFrameCollection) sourceFreezable; base.GetCurrentValueAsFrozenCore(sourceFreezable); int count = sourceCollection._keyFrames.Count; _keyFrames = new List< RectKeyFrame>(count); for (int i = 0; i < count; i++) { RectKeyFrame keyFrame = (RectKeyFrame)sourceCollection._keyFrames[i].GetCurrentValueAsFrozen(); _keyFrames.Add(keyFrame); OnFreezablePropertyChanged(null, keyFrame); } } ///Freezable.GetCurrentValueAsFrozenCore . ////// /// protected override bool FreezeCore(bool isChecking) { bool canFreeze = base.FreezeCore(isChecking); for (int i = 0; i < _keyFrames.Count && canFreeze; i++) { canFreeze &= Freezable.Freeze(_keyFrames[i], isChecking); } return canFreeze; } #endregion #region IEnumerable ////// Returns an enumerator of the RectKeyFrames in the collection. /// public IEnumerator GetEnumerator() { ReadPreamble(); return _keyFrames.GetEnumerator(); } #endregion #region ICollection ////// Returns the number of RectKeyFrames in the collection. /// public int Count { get { ReadPreamble(); return _keyFrames.Count; } } ////// See public bool IsSynchronized { get { ReadPreamble(); return (IsFrozen || Dispatcher != null); } } ///ICollection.IsSynchronized . ////// See public object SyncRoot { get { ReadPreamble(); return ((ICollection)_keyFrames).SyncRoot; } } ///ICollection.SyncRoot . ////// Copies all of the RectKeyFrames in the collection to an /// array. /// void ICollection.CopyTo(Array array, int index) { ReadPreamble(); ((ICollection)_keyFrames).CopyTo(array, index); } ////// Copies all of the RectKeyFrames in the collection to an /// array of RectKeyFrames. /// public void CopyTo(RectKeyFrame[] array, int index) { ReadPreamble(); _keyFrames.CopyTo(array, index); } #endregion #region IList ////// Adds a RectKeyFrame to the collection. /// int IList.Add(object keyFrame) { return Add((RectKeyFrame)keyFrame); } ////// Adds a RectKeyFrame to the collection. /// public int Add(RectKeyFrame keyFrame) { if (keyFrame == null) { throw new ArgumentNullException("keyFrame"); } WritePreamble(); OnFreezablePropertyChanged(null, keyFrame); _keyFrames.Add(keyFrame); WritePostscript(); return _keyFrames.Count - 1; } ////// Removes all RectKeyFrames from the collection. /// public void Clear() { WritePreamble(); if (_keyFrames.Count > 0) { for (int i = 0; i < _keyFrames.Count; i++) { OnFreezablePropertyChanged(_keyFrames[i], null); } _keyFrames.Clear(); WritePostscript(); } } ////// Returns true of the collection contains the given RectKeyFrame. /// bool IList.Contains(object keyFrame) { return Contains((RectKeyFrame)keyFrame); } ////// Returns true of the collection contains the given RectKeyFrame. /// public bool Contains(RectKeyFrame keyFrame) { ReadPreamble(); return _keyFrames.Contains(keyFrame); } ////// Returns the index of a given RectKeyFrame in the collection. /// int IList.IndexOf(object keyFrame) { return IndexOf((RectKeyFrame)keyFrame); } ////// Returns the index of a given RectKeyFrame in the collection. /// public int IndexOf(RectKeyFrame keyFrame) { ReadPreamble(); return _keyFrames.IndexOf(keyFrame); } ////// Inserts a RectKeyFrame into a specific location in the collection. /// void IList.Insert(int index, object keyFrame) { Insert(index, (RectKeyFrame)keyFrame); } ////// Inserts a RectKeyFrame into a specific location in the collection. /// public void Insert(int index, RectKeyFrame keyFrame) { if (keyFrame == null) { throw new ArgumentNullException("keyFrame"); } WritePreamble(); OnFreezablePropertyChanged(null, keyFrame); _keyFrames.Insert(index, keyFrame); WritePostscript(); } ////// Returns true if the collection is frozen. /// public bool IsFixedSize { get { ReadPreamble(); return IsFrozen; } } ////// Returns true if the collection is frozen. /// public bool IsReadOnly { get { ReadPreamble(); return IsFrozen; } } ////// Removes a RectKeyFrame from the collection. /// void IList.Remove(object keyFrame) { Remove((RectKeyFrame)keyFrame); } ////// Removes a RectKeyFrame from the collection. /// public void Remove(RectKeyFrame keyFrame) { WritePreamble(); if (_keyFrames.Contains(keyFrame)) { OnFreezablePropertyChanged(keyFrame, null); _keyFrames.Remove(keyFrame); WritePostscript(); } } ////// Removes the RectKeyFrame at the specified index from the collection. /// public void RemoveAt(int index) { WritePreamble(); OnFreezablePropertyChanged(_keyFrames[index], null); _keyFrames.RemoveAt(index); WritePostscript(); } ////// Gets or sets the RectKeyFrame at a given index. /// object IList.this[int index] { get { return this[index]; } set { this[index] = (RectKeyFrame)value; } } ////// Gets or sets the RectKeyFrame at a given index. /// public RectKeyFrame this[int index] { get { ReadPreamble(); return _keyFrames[index]; } set { if (value == null) { throw new ArgumentNullException(String.Format(CultureInfo.InvariantCulture, "RectKeyFrameCollection[{0}]", index)); } WritePreamble(); if (value != _keyFrames[index]) { OnFreezablePropertyChanged(_keyFrames[index], value); _keyFrames[index] = value; Debug.Assert(_keyFrames[index] != null); WritePostscript(); } } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
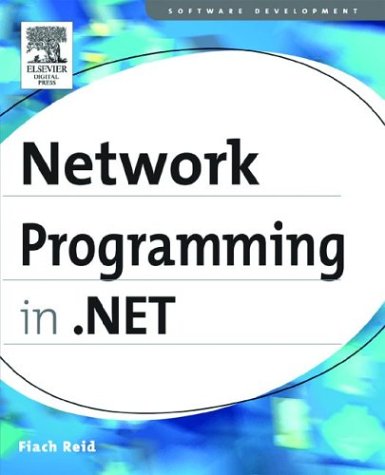
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- LogEntryDeserializer.cs
- ErrorProvider.cs
- DataException.cs
- HierarchicalDataBoundControlAdapter.cs
- OraclePermissionAttribute.cs
- ComponentChangedEvent.cs
- ObjectQueryProvider.cs
- PolicyException.cs
- _ConnectOverlappedAsyncResult.cs
- Visual.cs
- XamlTypeMapper.cs
- TemplatedEditableDesignerRegion.cs
- PageAdapter.cs
- SchemaCollectionCompiler.cs
- SqlTypeSystemProvider.cs
- FormViewCommandEventArgs.cs
- FileNotFoundException.cs
- _LocalDataStore.cs
- IdentitySection.cs
- MissingManifestResourceException.cs
- ForeignKeyFactory.cs
- assertwrapper.cs
- ArraySegment.cs
- SiteOfOriginPart.cs
- SymmetricAlgorithm.cs
- Gdiplus.cs
- DataControlImageButton.cs
- ContractUtils.cs
- BaseComponentEditor.cs
- WebPartDisplayModeCollection.cs
- ListenerConnectionDemuxer.cs
- MergeFailedEvent.cs
- ReturnValue.cs
- NumericUpDownAccelerationCollection.cs
- ArrayTypeMismatchException.cs
- Triangle.cs
- ObjectMaterializedEventArgs.cs
- TdsRecordBufferSetter.cs
- OverlappedAsyncResult.cs
- CharacterBufferReference.cs
- OracleString.cs
- Attachment.cs
- PrintDialogException.cs
- ReliableInputConnection.cs
- HttpHandlerActionCollection.cs
- Rect3D.cs
- DefaultClaimSet.cs
- X500Name.cs
- NestedContainer.cs
- EventSinkHelperWriter.cs
- X509Certificate.cs
- SignatureDescription.cs
- InputEventArgs.cs
- ControlParameter.cs
- ApplicationHost.cs
- HTTPNotFoundHandler.cs
- ToolStripItemTextRenderEventArgs.cs
- ComEventsInfo.cs
- ProxyFragment.cs
- ReadOnlyDataSource.cs
- UInt16Storage.cs
- RemoteArgument.cs
- AssemblyAssociatedContentFileAttribute.cs
- FigureParagraph.cs
- VersionValidator.cs
- PrivilegedConfigurationManager.cs
- BitmapEffectGeneralTransform.cs
- RankException.cs
- ErrorActivity.cs
- TemplateColumn.cs
- RemoteWebConfigurationHostStream.cs
- BuildProviderCollection.cs
- BoolExpr.cs
- ChildrenQuery.cs
- MouseGesture.cs
- DataGridViewRowCollection.cs
- QueryStringParameter.cs
- ClientRuntimeConfig.cs
- XmlHierarchicalEnumerable.cs
- RequestUriProcessor.cs
- WrapperSecurityCommunicationObject.cs
- FixedStringLookup.cs
- ToolStripItemImageRenderEventArgs.cs
- PrivateUnsafeNativeCompoundFileMethods.cs
- OleAutBinder.cs
- PlanCompiler.cs
- Visitors.cs
- DocumentGridContextMenu.cs
- BaseResourcesBuildProvider.cs
- MethodAccessException.cs
- WebScriptMetadataMessageEncoderFactory.cs
- ActionItem.cs
- ReadOnlyAttribute.cs
- ResourceProperty.cs
- ServiceProviders.cs
- SiteMapHierarchicalDataSourceView.cs
- CodeCatchClauseCollection.cs
- IdentityValidationException.cs
- AppDomainUnloadedException.cs
- DetailsViewDeletedEventArgs.cs