Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / Designer / Host / Serialization / ResourcePropertyMemberCodeDomSerializer.cs / 2 / ResourcePropertyMemberCodeDomSerializer.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.ComponentModel.Design.Serialization { using System; using System.CodeDom; using System.Collections; using System.ComponentModel; using System.ComponentModel.Design; using System.Diagnostics; using System.Globalization; using System.Resources; using System.Windows.Forms; using System.Windows.Forms.Design; ////// This serializer replaces the property serializer for properties when we're /// in localization mode. /// internal class ResourcePropertyMemberCodeDomSerializer : MemberCodeDomSerializer { private CodeDomLocalizationModel _model; private MemberCodeDomSerializer _serializer; private CodeDomLocalizationProvider.LanguageExtenders _extender; private CultureInfo localizationLanguage = null; internal ResourcePropertyMemberCodeDomSerializer(MemberCodeDomSerializer serializer, CodeDomLocalizationProvider.LanguageExtenders extender, CodeDomLocalizationModel model) { Debug.Assert(extender != null, "Extender should have been created by now."); _serializer = serializer; _extender = extender; _model = model; } ////// This method actually performs the serialization. When the member is serialized /// the necessary statements will be added to the statements collection. /// public override void Serialize(IDesignerSerializationManager manager, object value, MemberDescriptor descriptor, CodeStatementCollection statements) { // We push the localization model to indicate that our serializer is in control. Our // serialization provider looks for this and decides what type of resource serializer // to give us. manager.Context.Push(_model); try { _serializer.Serialize(manager, value, descriptor, statements); } finally { manager.Context.Pop(); } } private CultureInfo GetLocalizationLanguage(IDesignerSerializationManager manager) { if (localizationLanguage == null) { // Check to see if our base component's localizable prop is true RootContext rootCxt = manager.Context[typeof(RootContext)] as RootContext; if (rootCxt != null) { object comp = rootCxt.Value; PropertyDescriptor prop = TypeDescriptor.GetProperties(comp)["LoadLanguage"]; if (prop != null && prop.PropertyType == typeof(CultureInfo)) { localizationLanguage = (CultureInfo)prop.GetValue(comp); } } } return localizationLanguage; } private void OnSerializationComplete(object sender, EventArgs e) { // we do the cleanup here and clear out the cache of the localizedlanguage localizationLanguage = null; //unhook the event IDesignerSerializationManager manager = sender as IDesignerSerializationManager; Debug.Assert(manager != null, "manager should not be null!"); if(manager != null) { manager.SerializationComplete -= new EventHandler(OnSerializationComplete); } } ////// This method returns true if the given member descriptor should be serialized, /// or false if there is no need to serialize the member. /// public override bool ShouldSerialize(IDesignerSerializationManager manager, object value, MemberDescriptor descriptor) { bool shouldSerialize = _serializer.ShouldSerialize(manager, value, descriptor); if (!shouldSerialize && !descriptor.Attributes.Contains(DesignOnlyAttribute.Yes)) { switch (_model) { case CodeDomLocalizationModel.PropertyReflection : if(!shouldSerialize) { // hook up the event the first time to clear out our cache at the end of the serialization if(localizationLanguage == null) { manager.SerializationComplete += new EventHandler(OnSerializationComplete); } if(GetLocalizationLanguage(manager) != CultureInfo.InvariantCulture) { shouldSerialize = true; } } break; case CodeDomLocalizationModel.PropertyAssignment : // If this property contains its default value, we still want to serialize it if we are in // localization mode if we are writing to the default culture, but only if the object // is not inherited. InheritanceAttribute inheritance = (InheritanceAttribute)manager.Context[typeof(InheritanceAttribute)]; if (inheritance == null) { inheritance = (InheritanceAttribute)TypeDescriptor.GetAttributes(value)[typeof(InheritanceAttribute)]; if (inheritance == null) { inheritance = InheritanceAttribute.NotInherited; } } if (inheritance.InheritanceLevel != InheritanceLevel.InheritedReadOnly) { shouldSerialize = true; } break; default : break; } } return shouldSerialize; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
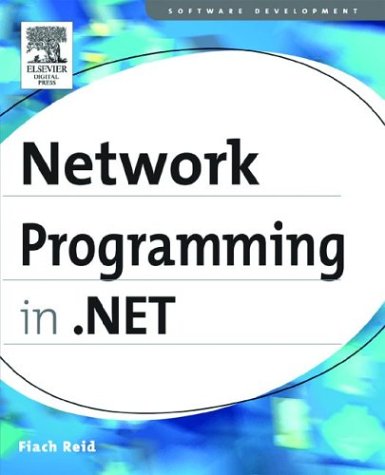
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- InputBindingCollection.cs
- ScriptMethodAttribute.cs
- ProjectionNode.cs
- GPPOINTF.cs
- FormatException.cs
- SqlConnectionPoolGroupProviderInfo.cs
- ImmutableObjectAttribute.cs
- HttpProfileGroupBase.cs
- DataFormats.cs
- DataGridClipboardHelper.cs
- DrawingVisual.cs
- Semaphore.cs
- EntityConnectionStringBuilderItem.cs
- TimeSpanSecondsOrInfiniteConverter.cs
- ObjectDataSource.cs
- RegexCompilationInfo.cs
- Sequence.cs
- ReadContentAsBinaryHelper.cs
- HttpRuntimeSection.cs
- TdsParserStaticMethods.cs
- SqlNode.cs
- wgx_commands.cs
- XPathNavigatorReader.cs
- Point3DCollectionValueSerializer.cs
- TdsParserSessionPool.cs
- Config.cs
- TableSectionStyle.cs
- AtlasWeb.Designer.cs
- dbenumerator.cs
- ImmComposition.cs
- WindowsToolbar.cs
- SessionIDManager.cs
- SQLBytes.cs
- MultipleViewProviderWrapper.cs
- OutKeywords.cs
- Int32Storage.cs
- DataServices.cs
- ExpressionBindings.cs
- MultipleViewPattern.cs
- ConsumerConnectionPointCollection.cs
- CategoryNameCollection.cs
- QuaternionAnimationBase.cs
- RuntimeHandles.cs
- Point.cs
- DSASignatureDeformatter.cs
- Subtree.cs
- QilScopedVisitor.cs
- comcontractssection.cs
- ParentControlDesigner.cs
- TemplateParser.cs
- ArrayElementGridEntry.cs
- UrlPath.cs
- WebPartConnectionsCloseVerb.cs
- Button.cs
- Int32Storage.cs
- AccessorTable.cs
- FamilyTypeface.cs
- PersonalizationProvider.cs
- ByteKeyFrameCollection.cs
- mactripleDES.cs
- ViewManager.cs
- CurrencyWrapper.cs
- __ComObject.cs
- GridItemPattern.cs
- DelayedRegex.cs
- TranslateTransform3D.cs
- HeaderedItemsControl.cs
- CalendarData.cs
- DataGridViewRowErrorTextNeededEventArgs.cs
- CompareValidator.cs
- DocumentViewerBase.cs
- GeometryHitTestParameters.cs
- BooleanAnimationUsingKeyFrames.cs
- RawStylusInput.cs
- SqlNodeTypeOperators.cs
- PreviewPageInfo.cs
- RSAProtectedConfigurationProvider.cs
- SqlCaseSimplifier.cs
- TranslateTransform3D.cs
- DataGridViewRowHeightInfoNeededEventArgs.cs
- WindowsListViewItemCheckBox.cs
- HtmlTernaryTree.cs
- AncillaryOps.cs
- ExpandCollapseProviderWrapper.cs
- Positioning.cs
- InheritanceAttribute.cs
- FixedSOMElement.cs
- TdsParserHelperClasses.cs
- GPRECTF.cs
- Repeater.cs
- FixedSOMTableCell.cs
- Scene3D.cs
- IntSecurity.cs
- CookielessHelper.cs
- GACMembershipCondition.cs
- ExpandSegment.cs
- CachedTypeface.cs
- Border.cs
- MetaType.cs
- BinarySecretSecurityToken.cs